PGDAC | Mock Test | OOPS Java | Problem #1
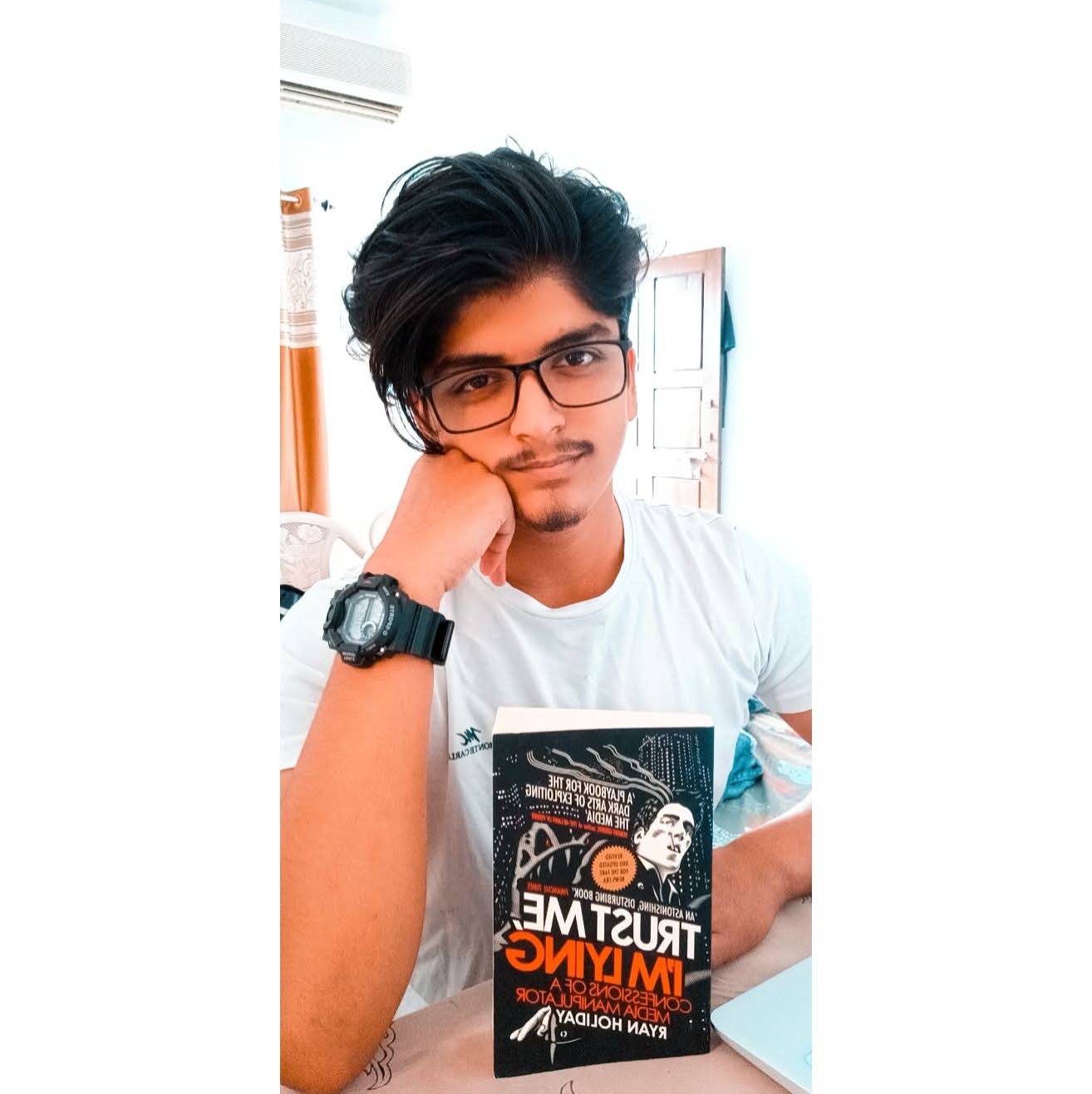

Let's begin with the Problem statement first.
Problem Statement:
Q1. Write a program to find the index of the second last occurrence of a Character in the given String.
Explanation of the Problem Statement:
The problem wants us to ask the user to input a String and then ask for a Character that is to be searched in the provided String and return the second last occurrence of that Character in that String.
Solution:
For those who would like to figure out the approach on their own here's the solution:
// Q. Write a program to find index of second last occurrence of a character in a given string.
package oops_mock;
import java.util.Scanner;
public class Q1Occurrence {
public static void main(String[] args) {
Scanner in = new Scanner(System.in);
// Asking user for String
System.out.println("Enter your string: ");
String str = in.nextLine();
// Asking user for Character to be searched for
System.out.println("Enter the character you wish to look for: ");
char ch = in.next().charAt(0);
// Declaration of index variables
int index1 = -1, index2 = -1;
// Converting the provided String into Character Array
char[] arr = str.toCharArray();
// Using for loop to parse through the Array
for (int i=0; i<arr.length; i++){
// Using If condition to look if the Character at arr[i] matches our Character
if (ch == arr[i]){
// Storing the index values in the varibles
index2 = index1;
index1 = i;
}
}
// If the Character doesn't exist in the given String
if (index1 == -1){
System.out.println("Character not present in the given String");
}
// If the Character only exists once in the given String
if (index1 != -1 && index2 == -1) {
System.out.println("There are no two occurrence of your Character in the given String");
}
// Printing the second last index of the occurrence of the Character in the given String
if (index1 != -1 && index2 != -1){
System.out.println("The last second occurrence of your Character in the given String is at index: " + index2);
// We printed index2 as it stored the index of the second last occurrence of the Character in the given String
}
}
}
Approach to the problem:
- First things first, we will ask for inputs we will need for the problem statement:
// Asking user for String
System.out.println("Enter your string: ");
String str = in.nextLine();
// Asking user for Character to be searched for
System.out.println("Enter the character you wish to look for: ");
As we have to look for a Character in a String, we have two options:
Either we can run a for loop with an if condition looking for matches of Character ch and str.charAt(i);
Or we can convert our String into a Character Array and then look for matching values;
Both approaches should work just fine, however, due to the ease of application, we will be using the second approach here, so,
// Converting the provided String into Character Array
char[] arr = str.toCharArray();
// Using for loop to parse through the Array
for (int i=0; i<arr.length; i++){
// Using If condition to look if the Character at arr[i] matches our Character
if (ch == arr[i]){
// Storing the index values in the varibles
index2 = index1;
index1 = i;
}
}
Now the question is why index1 and index2??
Think of it this way, when we look for a single occurrence of an element in an array we store its index in a single variable and update it whenever the if case matches, however in this approach the previous value is over-written.As per the problem statement, we have to print out the index of the second last occurrence of the Character in the given String. If we follow the regular approach, we will be updating the value of our variable till the last occurrence.
So to store the previous index, we will first store the value of the index of the previous occurrence in index2 and then store the index of the current occurrence in index1. This way we will be able to store the value of the index of the second last occurrence of the Character in the String.
Another question would be why the initial values of the index1 and index2 are -1;
consider if the String is "Hello Harry Heigns!" and the Character to look for is "H". The very first occurrence of the Character "H" in our string is at the 0th index. Hence to include a case where the Character is at the 0th index, we initialized our variables from -1 as an index would never be -1.
// Declaration of index variables
int index1 = -1, index2 = -1;
Now we can have 3 cases here,
First case:
The Character does not have any occurrence in the given String:
In that case, the values of our index would never update and will remain -1, so we will write our first condition for this outcome:
// If the Character only exists once in the given String
if (index1 != -1 && index2 == -1) {
System.out.println("There are no two occurrence of your Character in the given String");
- Second case:
The Character only occurs once in the given String:
In that case, the value of index1 will change to the index of the occurrence of the Character however the value of index2 will remain -1, so we will write our second condition for this outcome:
// If the Character only exists once in the given String
if (index1 != -1 && index2 == -1) {
System.out.println("There are no two occurrence of your Character in the given String");
}
Third case:
The Character occurs twice or more times in the given String:In this case, the index1 will store the value of the index of the last occurrence of the Character in the given String and the value of the index of the second last occurrence of the Character in the given String, so we will write our final condition for this outcome
// Printing the second last index of the occurrence of the Character in the given String
if (index1 != -1 && index2 != -1){
System.out.println("The last second occurrence of your Character in the given String is at index: " + index2);
// We printed index2 as it stored the index of the second last occurrence of the Character in the given String
}
If you are looking for the solution to Problem #2 for the OOPS Java Mock Test, click on the link below:
https://ahad.hashnode.dev/iacsd-pgdac-mock-test-oops-java-problem-2
I hope this helps, if you have a better solution, please share your solution with me on my GitHub Repository, the link to the repo is mentioned below:
GitHub Repository for OOPS Java Mock Test Solution
You may wanna drop a follow to the GitHub repository as these solutions are uploaded on the repo some hours before they are shared on these blogs.
Thank you so much for reading
Happy Learning!
Subscribe to my newsletter
Read articles from Abdul Ahad Sheikh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
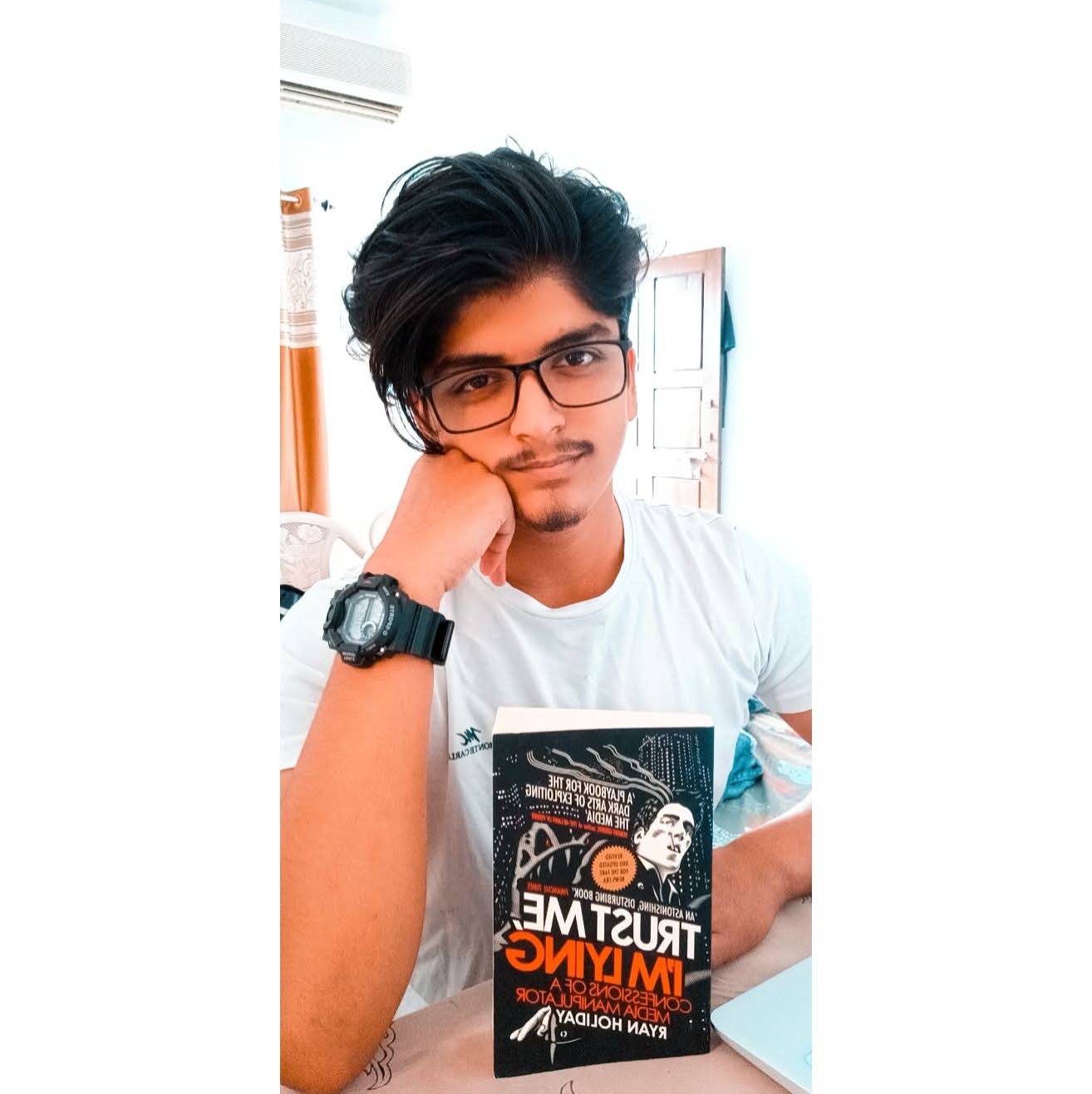
Abdul Ahad Sheikh
Abdul Ahad Sheikh
Learning Fullstack Development using Java and .Net Aspiring Public Speaker Cofounder @BookBrotherhood Part-time Musician🎶 and Writer✒️ Live😊 | Love❤️ | Laugh😁