PGDAC | Mock Test |OOPS Java | Problem #2
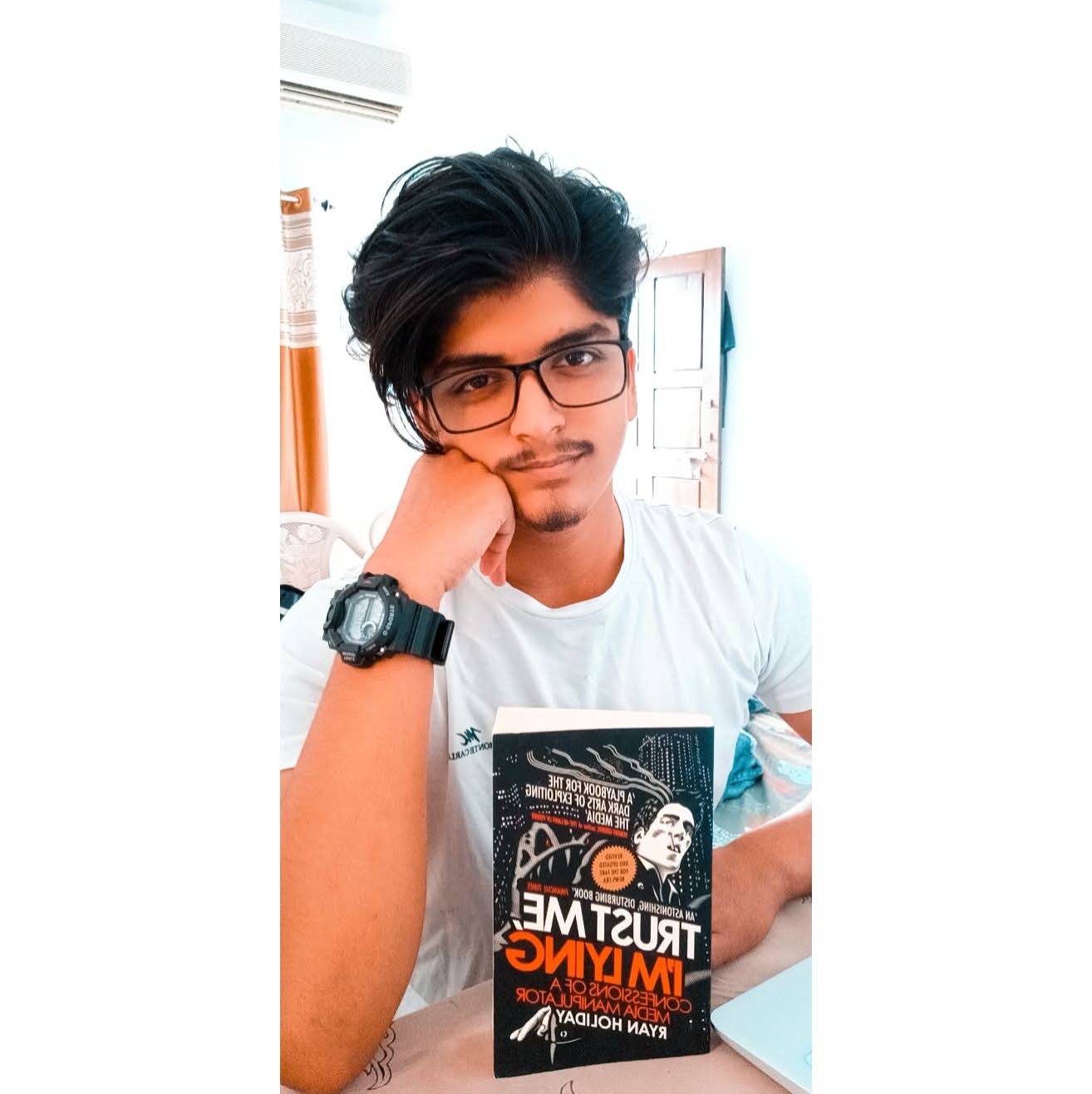

Let's begin with the Problem statement first.
Problem Statement:
Q2. Write a program to check whether 2 strings are Anagram or not
Explanation of the Problem Statement:
The problem wants us to ask the user to input two Strings and check if the Strings are Anagram.
Now, What are Anagrams?
Anagrams simply mean that the characters in The Strings are the same however their arrangement is different
For example: "the morse code" and "here come dots" are Anagrams because the characters in both the Strings are same.
Solution:
For those who would like to figure out the approach on their own here's the solution:
// Q. Write a program to check whether two strings are Anagram or not.
package oops_mock;
import java.util.*;
public class Q2Anagram {
public static void main(String[] args) {
Scanner in = new Scanner(System.in);
// Asking user for 1st String
System.out.println("Enter the first String: ");
String str1 = in.nextLine();
// Asking user for 2nd String
System.out.println("Enter the second String: ");
String str2 = in.nextLine();
// Initializing a flag checker
boolean flag = true;
// First check condition
if (str1.length() != str2.length()){
System.out.println("Your Strings are not Anagram");
}
else{
// Converting Strings to respecting Character Array
char [] arr1 = str1.toCharArray();
char [] arr2 = str2.toCharArray();
// Using Array.sort() method to sort the array Alphabetically
Arrays.sort(arr1);
Arrays.sort(arr2);
// Using for loop to match the elements of both the Arrays
for (int i=0; i<arr1.length; i++){
// If the condition fails, changing the state of flag to false
if (arr1[i] != arr2 [i]) {
flag = false;
break;
}
}
// if the state of flag is true
if (flag){
System.out.println("Your Strings are Anagram");
}
// if the state of flag is false
else {
System.out.println("Your Strings are not Anagram");
}
}
}
}
Approach to the problem:
As we have to match each and every character in the String it would be simpler if we just convert both the String into Character Arrays, sort them in alphabetical order and then look if all the Characters in both the Strings are matching or not. This is an easy and simple approach to check for Anagrams. So let's get coding.
- First things first, we will ask for inputs we will need for the problem statement:
// Asking user for 1st String
System.out.println("Enter the first String: ");
String str1 = in.nextLine();
// Asking user for 2nd String
System.out.println("Enter the second String: ");
String str2 = in.nextLine();
- We will be working with some check conditions so we will initialize a flag
// Initializing a flag checker
boolean flag = true;
- Our first condition is to check if the length of both strings is the same or not. If the length of both the strings isn't the same, we can be certain that they are not Anagrams, so,
// First check condition
if (str1.length() != str2.length()){
System.out.println("Your Strings are not Anagram");
}
- Now, if the length of both the strings are equal, let's convert them into Character arrays for ease of processing, and then we will sort these Arrays in alphabetical order using the Arrays.sort() method
// Converting Strings to respecting Character Array
char [] arr1 = str1.toCharArray();
char [] arr2 = str2.toCharArray();
// Using Array.sort() method to sort the array Alphabetically
Arrays.sort(arr1);
Arrays.sort(arr2);
- Now that we have 2 alphabetically sorted arrays, we just have to run a for loop and look for matches. If the Characters don't match, our Strings are not Anagram, else, they are Anagram. So, let's code the last part of our solution
// Using for loop to match the elements of both the Arrays
for (int i=0; i<arr1.length; i++){
// If the condition fails, changing the state of flag to false
if (arr1[i] != arr2 [i]) {
flag = false;
break;
}
}
// if the state of flag is true
if (flag){
System.out.println("Your Strings are Anagram");
}
// if the state of flag is false
else {
System.out.println("Your Strings are not Anagram");
}
With this, we have solved the Anagram problem too...
If you are looking for the solution to Problem #1 for the OOPS Java Mock Test, click on the link below:
https://ahad.hashnode.dev/iacsd-pgdac-mock-test-oops-java-problem-1
I hope this helps, if you have a better solution, please share your solution with me on my GitHub Repository, the link to the repo is mentioned below:
GitHub Repository for OOPS Java Mock Test Solution
You may wanna drop a follow to the GitHub repository as these solutions are uploaded on the repo some hours before they are shared on these blogs.
Thank you so much for reading
Happy Learning!
Subscribe to my newsletter
Read articles from Abdul Ahad Sheikh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
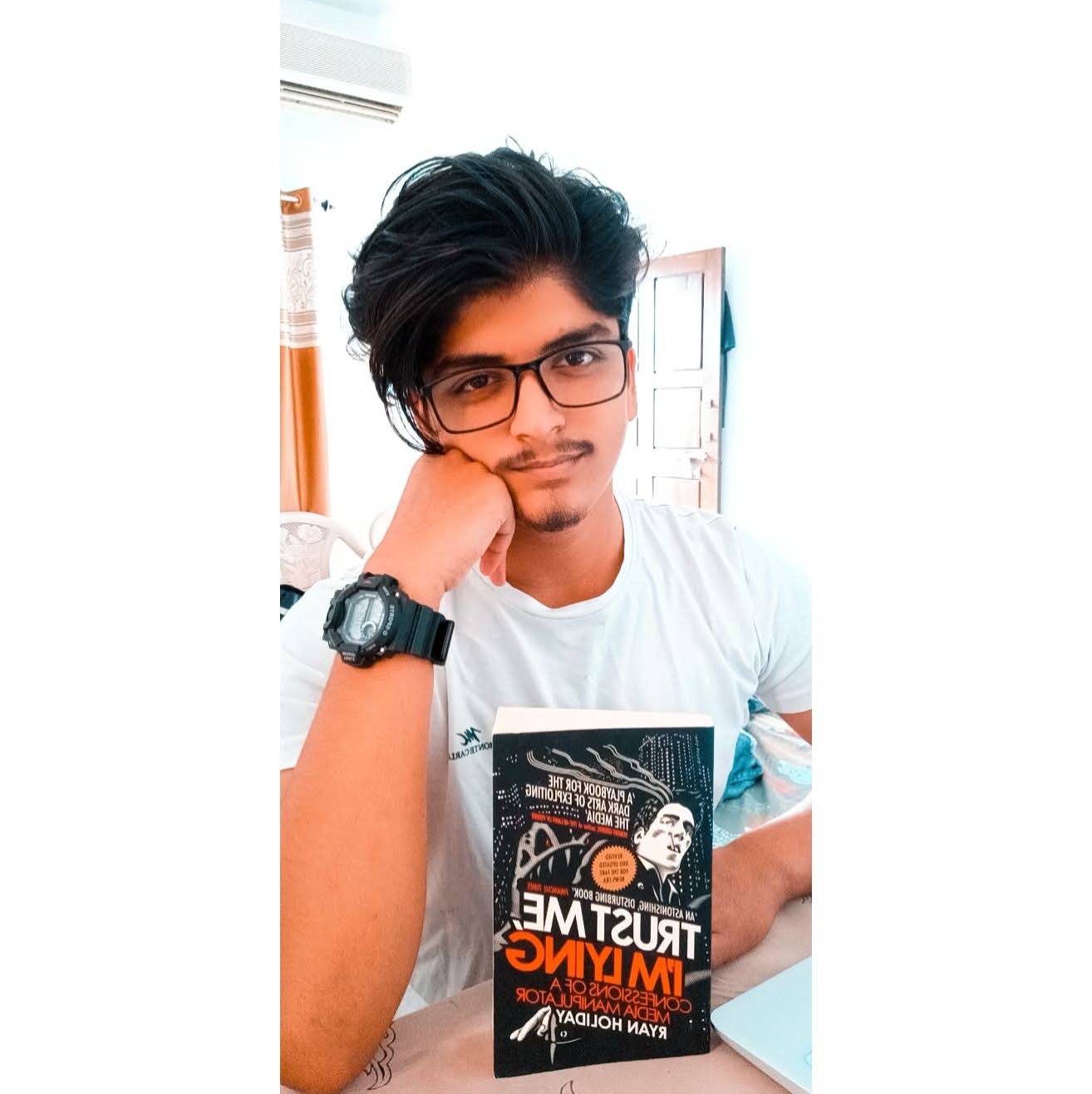
Abdul Ahad Sheikh
Abdul Ahad Sheikh
Learning Fullstack Development using Java and .Net Aspiring Public Speaker Cofounder @BookBrotherhood Part-time Musician🎶 and Writer✒️ Live😊 | Love❤️ | Laugh😁