Kubernetes Workloads

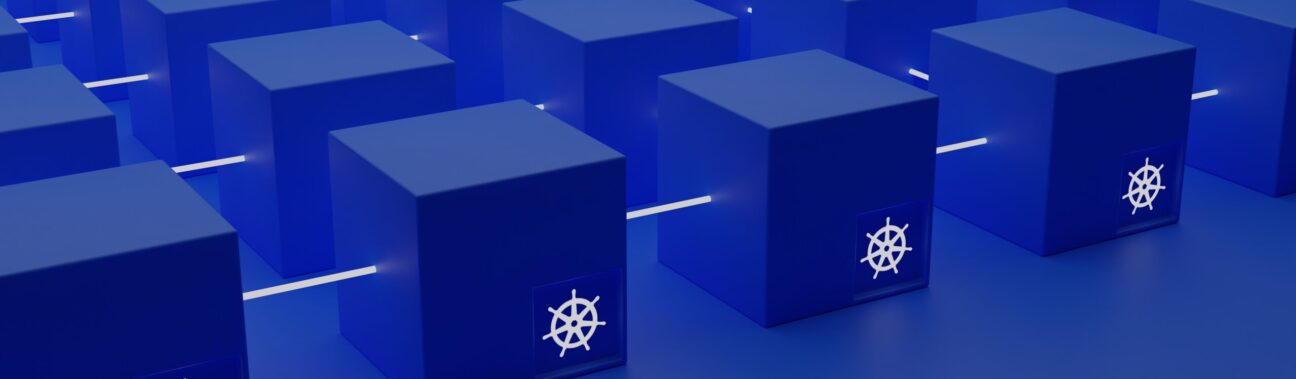
A workload refers to the type of application or service that is being deployed and managed. Workloads are defined using Kubernetes resources such as Deployments, StatefulSets, DaemonSets, Jobs, and CronJobs.
Here are some common Kubernetes workloads:
Deployments: Deployments manage a set of replica Pods and ensure that the desired number of replicas are running at any given time. Deployments are commonly used for stateless applications, such as web servers, that can scale horizontally by adding or removing replicas.
StatefulSets: StatefulSets manage a set of replica Pods, each with a unique identity and persistent storage. StatefulSets are commonly used for stateful applications, such as databases or messaging systems, that require stable network identities and persistent storage.
DaemonSets: DaemonSets ensure that a single copy of a Pod is running on each node in the cluster. DaemonSets are commonly used for system-level daemons or agents that need to run on every node in the cluster.
Jobs: Jobs manage a set of Pods that perform a specific task and then terminate. Jobs are commonly used for batch processing or one-time tasks, such as data processing or backups.
CronJobs: CronJobs manage a set of Jobs that run on a periodic schedule, similar to a Unix cron job. CronJobs are commonly used for periodic maintenance or data processing tasks.
Let's discuss them in a bit more detail.
Deployments
When you create a deployment in Kubernetes, you specify the number of replicas you want to run, as well as the container image and any other configuration options that are needed. Kubernetes then creates a specified number of replica pods and monitors them to ensure that they are running and healthy.
Here are some key features of deployments in Kubernetes, along with examples:
Scaling: Deployments can scale the number of replicas up or down automatically based on resource utilization or other factors. For example, you can scale a deployment to 3 replicas using the following command:
kubectl scale deployment my-deployment --replicas=3
This updates the deployment by increasing the number of pods to 3. This is known as scaling out the pods.
Rolling updates: Deployments allow you to perform rolling updates of your application with no downtime. For example, you can update the image of your deployment to a new version using the following command:
kubectl set image deployment/my-deployment my-container=my-image:v2
This command will update the deployment's pod template to use the new image, and then perform a rolling update, one pod at a time, until all replicas are running the new version.
Here is an example of updating a deployment using a rolling update:
apiVersion: apps/v1 kind: Deployment metadata: name: my-deployment spec: replicas: 3 selector: matchLabels: app: my-app template: metadata: labels: app: my-app spec: containers: - name: my-container image: nginx:1.15.12 ports: - containerPort: 80 strategy: type: RollingUpdate rollingUpdate: maxUnavailable: 1 maxSurge: 1
In this example, the
maxUnavailable
andmaxSurge
parameters are set to 1, which means that during the update, only one pod can be down at a time, and there will be only one extra pod beyond the desired number of replicas. This ensures that the update is applied gradually, without impacting the availability of the application.To trigger the update, you can run the following command:
kubectl apply -f my-deployment.yaml
You can monitor the progress of the update using the following command:
kubectl rollout status deployment my-deployment
If the update causes issues, you can roll back to the previous version using the following command:
kubectl rollout undo deployment my-deployment
Automatic rollout of new replicas: Deployments can automatically rollout new replicas based on changes to the pod template, such as updates to container images or environment variables. For example, if you update the image of your deployment, Kubernetes will automatically create new replicas with the updated image and terminate the old ones.
Customization: Deployments provide a wide range of configuration options, such as liveness and readiness probes, container resource requests and limits, and environment variables. For example, you can configure a liveness probe for your deployment using the following YAML:
apiVersion: apps/v1 kind: Deployment metadata: name: my-deployment spec: replicas: 3 selector: matchLabels: app: my-app template: metadata: labels: app: my-app spec: containers: - name: my-container image: my-image:v1 livenessProbe: httpGet: path: /healthz port: 8080
This YAML configures a liveness probe for the container in the deployment, which checks the path "/healthz" on port 8080 to determine if the container is healthy. If the probe fails, Kubernetes will restart the container automatically.
Here is an example to create a simple deployment:
Create a YAML file named
my-deployment.yaml
with the following contents:apiVersion: apps/v1 kind: Deployment metadata: name: my-deployment spec: replicas: 3 selector: matchLabels: app: my-app template: metadata: labels: app: my-app spec: containers: - name: my-container image: nginx:latest ports: - containerPort: 80
This YAML file defines a deployment with the name
my-deployment
, which will create 3 replicas of a container running thenginx
image on port 80.Deploy the deployment by running the following command:
kubectl apply -f my-deployment.yaml
This command will create the deployment, which will create the specified number of replica pods running the
nginx
image.Verify that the deployment and pods are running by running the following commands:
kubectl get deployments kubectl get pods
These commands will show you the status of the deployment and the pods created by the deployment.
Statefulsets
StatefulSet is a workload API object that is used to manage stateful applications. It provides guarantees about the ordering and uniqueness of pods, which is useful for applications that require stable network identities or persistent storage.
StatefulSets are similar to Deployments, but they differ in several key ways:
Ordering: StatefulSets provide a stable network identity and ordering for their pods. This means that each pod has a unique hostname and network identity, and they are created and deleted in a specific order.
Scaling: StatefulSets can be scaled up or down, but the scaling process is more complicated than with Deployments. Because each pod has a unique identity, scaling a StatefulSet requires creating or deleting pods in a specific order.
Persistence: StatefulSets are often used for stateful applications that require persistent storage. Each pod in a StatefulSet can be associated with a unique persistent volume, which allows the pod to maintain its state even if it is rescheduled to a different node.
Here is an example of a StatefulSet configuration file in Kubernetes:
apiVersion: apps/v1
kind: StatefulSet
metadata:
name: my-statefulset
spec:
selector:
matchLabels:
app: my-app
replicas: 3
serviceName: my-service
template:
metadata:
labels:
app: my-app
spec:
containers:
- name: my-container
image: nginx:latest
ports:
- containerPort: 80
volumeMounts:
- name: my-volume
mountPath: /var/www/html
volumeClaimTemplates:
- metadata:
name: my-volume
spec:
accessModes: ["ReadWriteOnce"]
resources:
requests:
storage: 1Gi
In this example, the StatefulSet includes a template for creating pods that run the nginx container image and listen on port 80. It also includes a volumeClaimTemplate that specifies a persistent volume for each pod in the StatefulSet.
When the StatefulSet is created, Kubernetes will create three pods, each with a unique hostname and network identity. The persistent volumes will be created and mounted to the appropriate pods. If a pod is deleted or rescheduled, Kubernetes will ensure that the new pod has the same hostname and network identity as the old pod, and that it is associated with the same persistent volume.
Daemonsets and Replicasets
DaemonSet is a type of workload that ensures that a copy of a pod is running on every node in the cluster. DaemonSets are often used for system daemons, log collectors, or other background services that need to run on every node in the cluster.
ReplicaSets ensure that a specified number of replicas, or copies, of a pod are running in a cluster. They are often used to provide horizontal scaling and high availability for stateless services, such as web applications.
Use Cases of DaemonSets
DaemonSets are useful for deploying a monitoring agent, log collector, cluster storage daemon, networking solution agent, or kube-proxy on every node in a Kubernetes cluster.
DaemonSets ensure that the agent is automatically added or removed from each node in the cluster as changes occur.
Examples of monitoring agents include Prometheus Node Exporter and Datadog agent, examples of log collectors include logstash and fluentd, examples of cluster storage daemons include glusterd and ceph.
Weavenet requires a Weavenet agent running on every node in the cluster.
kube-proxy is another good use case of DaemonSets, as it is needed on every node in the cluster to run IP tables and ensure that every node can access every pod.
Use Cases of ReplicaSets
Horizontal scaling: You can use ReplicaSets to scale up or down the number of replicas of a Pod running in your Kubernetes cluster based on the demand for the application or service it provides.
High availability: You can use ReplicaSets to ensure that a specified number of replicas of a Pod are always running in your cluster, even if one or more of the replicas fail or become unavailable for any reason.
Canary deployments: You can use ReplicaSets to gradually roll out new versions of an application or service to a subset of the replicas in the cluster, and then gradually increase the number of replicas running the new version until all replicas are updated.
Blue-green deployments: You can use ReplicaSets to deploy two identical environments, one active and one inactive, and switch traffic between them by updating the labels of the ReplicaSet selectors like so shown in the gif.
A/B testing: You can use ReplicaSets to run two different versions of the same application or service in separate ReplicaSets, and then gradually shift traffic from one version to the other to compare performance or user experience.
What may be the difference between the two?
ReplicaSets and DaemonSets are two different types of workloads in Kubernetes, and they serve different purposes.
Here is an example of a ReplicaSet configuration file:
apiVersion: apps/v1
kind: ReplicaSet
metadata:
name: my-replicaset
spec:
replicas: 3
selector:
matchLabels:
app: my-app
template:
metadata:
labels:
app: my-app
spec:
containers:
- name: my-container
image: nginx
ports:
- containerPort: 80
In this example, the ReplicaSet ensures that three replicas of the nginx container are running in the cluster. If a pod fails, the ReplicaSet will automatically create a new pod to replace it.
Here is an example of a DaemonSet configuration file:
apiVersion: apps/v1
kind: DaemonSet
metadata:
name: my-daemonset
spec:
selector:
matchLabels:
app: my-app
template:
metadata:
labels:
app: my-app
spec:
containers:
- name: my-container
image: nginx
ports:
- containerPort: 80
nodeSelector:
node-type: worker
In this example, the DaemonSet ensures that a single instance of the nginx container is running on every worker node in the cluster with the label of node-type "worker".
In summary, ReplicaSets ensure a specified number of replicas of a pod are running in the cluster, while DaemonSets ensure that a single instance of a pod is running on every node in the cluster.
Note that both ReplicaSets and DaemonSets use selectors to match pods based on labels. The main difference is that ReplicaSets create multiple replicas of a pod, while DaemonSets ensure that a single instance of a pod is running on every node.
Jobs
A Job is a type of workload that creates one or more pods and ensures that a specified number of them successfully complete before terminating. Jobs are often used to run batch or background tasks, such as data processing or backups, in a Kubernetes cluster.
Here is an example of a Job configuration file in Kubernetes:
apiVersion: batch/v1
kind: Job
metadata:
name: my-job
spec:
template:
metadata:
name: my-pod
spec:
containers:
- name: my-container
image: busybox
command: ["echo", "Hello, world!"]
restartPolicy: OnFailure
completions: 1
parallelism: 1
In this example, the Job creates a single pod running the busybox container, which prints "Hello, world!" to the console using the echo
command. The completions
parameter is set to 1, which means that the Job will continue to create new pods until one pod completes successfully. The parallelism
parameter is also set to 1, which means that only one pod will be created at a time.
To run the Job, you can use the following command:
kubectl apply -f my-job.yaml
Kubernetes will create a pod and monitor its progress. Once the pod completes successfully, the Job will terminate. If the pod fails, Kubernetes will create a new pod to replace it until the specified number of completions is reached.
You can monitor the progress of the Job using the kubectl describe job
command:
kubectl describe job my-job
This will show you information about the Job, including the number of pods that have completed successfully and any errors or failures. You can also view the logs of the pod using the kubectl logs
command:
kubectl logs my-job-<pod-name>
This will display the output of the echo
command in the pod.
CronJobs
A CronJob is the same as a regular Job, only it creates jobs on a schedule (with a syntax similar to the Linux cron utility). CronJobs are used to perform regularly scheduled tasks such as backups and report generation. You can define the tasks to run at specific times or repeat indefinitely (for example, daily or weekly).
Here is an example of a CronJob configuration file in Kubernetes:
apiVersion: batch/v1beta1
kind: CronJob
metadata:
name: my-cronjob
spec:
schedule: "*/5 * * * *"
jobTemplate:
spec:
template:
spec:
containers:
- name: my-container
image: nginx
command: ["/bin/sh"]
args: ["-c", "echo Hello from the Kubernetes cluster"]
restartPolicy: OnFailure
In this example, the CronJob runs a job every five minutes that runs a single container running the nginx
image. The container runs a command to echo "Hello from the Kubernetes cluster" to the console.
To run the CronJob, you can use the following command:
kubectl apply -f my-cronjob.yaml
Kubernetes will create a new job based on the template defined in the CronJob on the specified schedule. You can view the status of the CronJob and the jobs it creates using the kubectl get cronjob
and kubectl get jobs
commands:
kubectl get cronjob my-cronjob
kubectl get jobs --selector=job-name=my-cronjob-*
This will show you information about the CronJob and the jobs it has created, including their status, age, and completion time. You can also view the logs of a specific job using the kubectl logs
command:
kubectl logs my-cronjob-<job-name>
This will display the logs of the container running in the specified job.
The cron schedule syntax used by the spec.schedule
field has five characters, and each character represents one time unit.
Example 1 runs the job at 9 pm every Thursday
Example 2 runs the job every Friday at midnight and also on the 12th of each month at midnight
Example 3 runs the job every minute – the
*/n
syntax means to repeat the job every interval
Resources
https://www.leverege.com/iot-ebook/kubernetes-workloads
https://blog.learncodeonline.in/kubernetes-scheduling-daemonset
Subscribe to my newsletter
Read articles from Aditya Murali directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Aditya Murali
Aditya Murali
Dedicated and hardworking DevOps aspirant who is eager to contribute skills and experience to help organizations solve real world use cases.