Concatenation Of Array
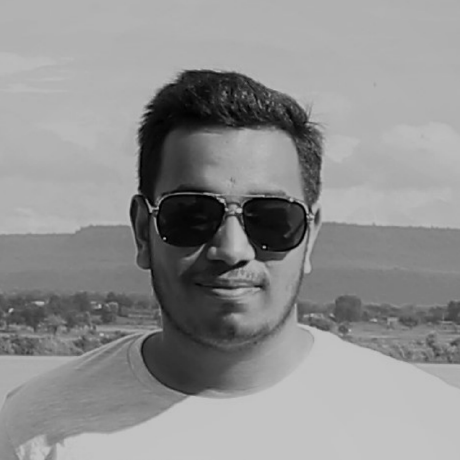
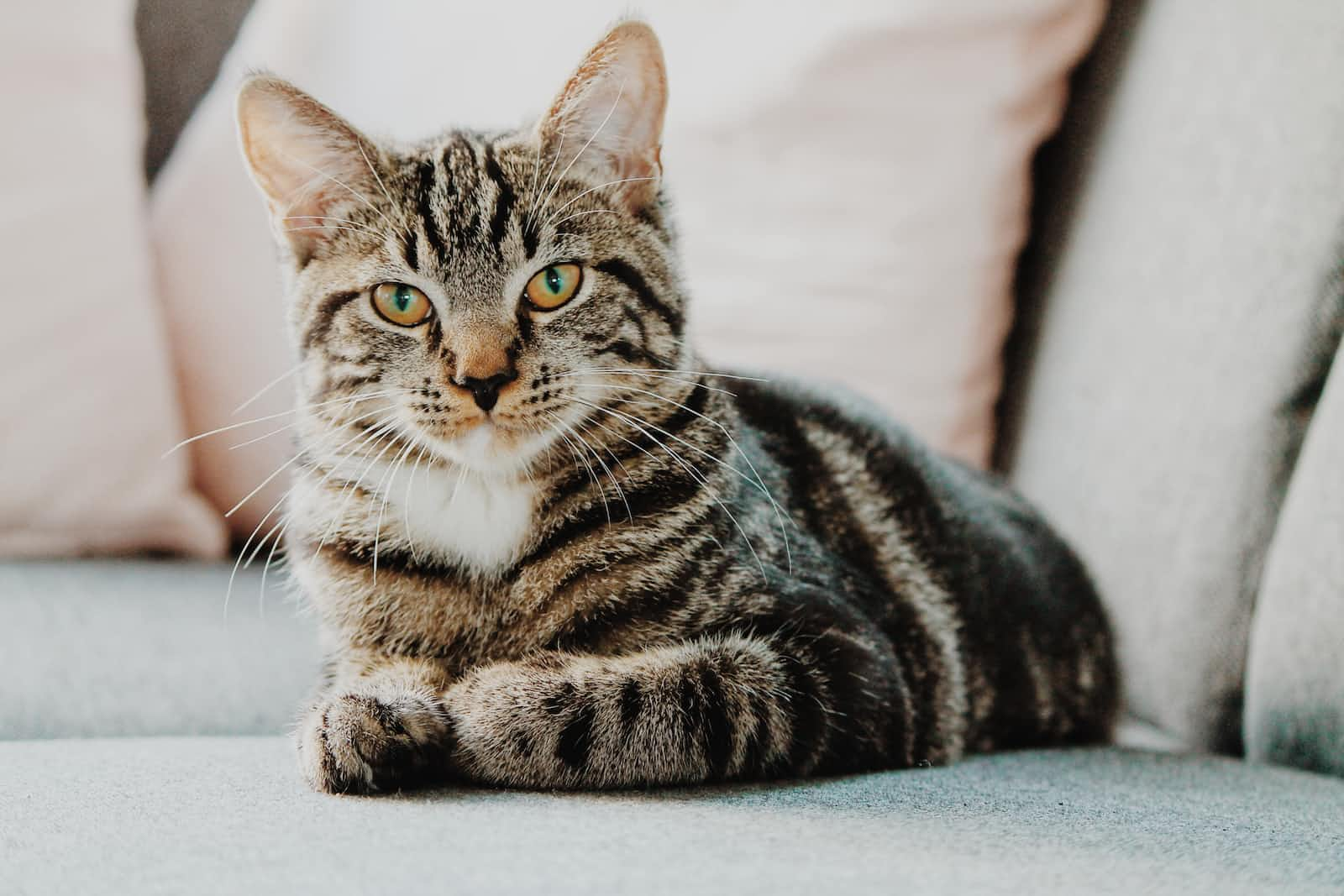
Problem Statement:
Given an integer array
num
of lengthn
, you want to create an arrayans
of length2n
whereans[i] == nums[i]
andans[i + n] == nums[i]
for0 <= i < n
(0-indexed). Specifically,ans
is the concatenation of twonums
arrays.
In JavaScript, the concatenation of two arrays is the process of merging the elements of two arrays into a single array. This can be done using the concat()
method, which returns a new array consisting of the elements of the original array followed by the elements of the argument array(s).
Approach 1: Arrays are dynamic in JavaScript
Declare an empty array
result
.Iterate through the array
arr
and for each element assign the same to theresult[i]
andresult[i + arr.length]
.
// ES6 Arrow Function
const concatenationArray = arr => {
let result = [];
for(let i = 0; i < arr.length; i++) {
result[i] = arr[i];
result[i + arr.length] = arr[i];
}
return result;
}
Time Complexity: O(N)
Space Complexity: O(N)
Approach 2: Constant space
- Store the length of the array
arr
in a variablen
and then run a loop n times and push the elements in the same array.
// ES6 Arrow Function
const concatenationArray = arr => {
let n = arr.length;
for(let i = 0; i < n; i++) {
arr.push(arr[i]);
}
return arr;
}
Time Complexity: O(n)
Space Complexity: O(1)
Note: Here are some fun ways to concatenate the array using the built in functions and operators in JavaScript like concat and spread.
Note: Time and Space for these are going to be linear.
// ES6 Arrow Function
const concatenationArray = arr => [...arr, ...arr];
// ES6 Arrow Function
const concatenationArray = arr => arr.concat(arr);
I hope this article has provided you with valuable insights and helped you better understand the different approaches to solve this problem. Happy Coding!
Problem - Leetcode 1929
Subscribe to my newsletter
Read articles from Nilesh Saini directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
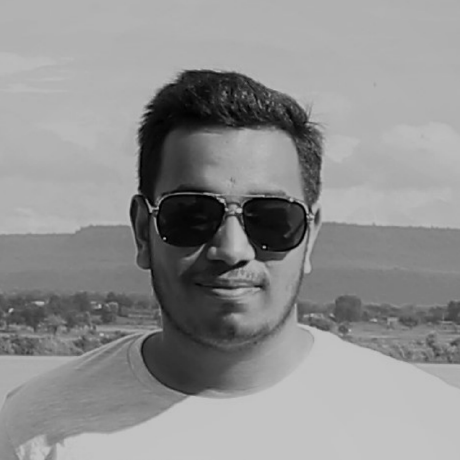