Kubernetes 101: Kubernetes Workloads
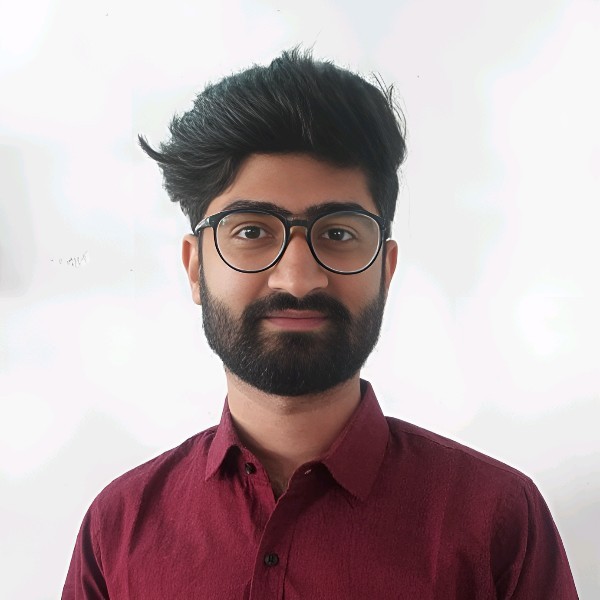
Table of contents
Kubernetes is a popular container orchestration system that enables you to automate the deployment, scaling, and management of containerized applications. One of the core concepts in Kubernetes is the concept of a "workload," which refers to the type of application that you want to run on the Kubernetes cluster. Kubernetes supports several types of workloads, each with its own characteristics and use cases.
In this article, we'll discuss the different types of Kubernetes workloads and when you might want to use each one.
Deployment
A Deployment is the most commonly used type of workload in Kubernetes. It's designed to manage the deployment and scaling of a set of identical pods. A pod is a single instance of an application, and a Deployment manages multiple replicas of the same pod to provide high availability and scalability. Deployments are ideal for stateless applications, such as web servers, where each instance is interchangeable and can be scaled up or down based on demand. Deployments provide several features, such as rolling updates, rollbacks, and automatic scaling based on resource utilization or custom metrics.
Rolling updates allow you to update your application without downtime by gradually replacing old pods with new ones. Rollbacks enable you to quickly revert to a previous version of your application if a new version causes issues. Automatic scaling enables you to scale your application up or down based on CPU or memory utilization, or based on custom metrics such as request latency or queue length.
apiVersion: apps/v1 kind: Deployment metadata: name: my-app spec: replicas: 3 selector: matchLabels: app: my-app template: metadata: labels: app: my-app spec: containers: - name: my-app-container image: my-app-image:latest ports: - containerPort: 8080 env: - name: ENV_VAR_1 value: "value1" - name: ENV_VAR_2 value: "value2"
This manifest file creates a Deployment called "my-app" with three replicas. It uses a container image called "my-app-image:latest" and exposes port 8080. It also sets two environment variables called "ENV_VAR_1" and "ENV_VAR_2".
StatefulSet
A StatefulSet is used for stateful applications that require stable, unique network identities and persistent storage. StatefulSets are typically used for databases, such as MySQL, where each instance requires its own stable network identity and persistent storage. StatefulSets guarantee that pods are deployed in a specific order, and that each pod has a unique identity that is retained even if it's rescheduled to a different node. This makes it possible to provide stable network identities and persistent storage to stateful applications, even if the underlying nodes or pods change.
StatefulSets also provide several features that are specific to stateful applications, such as ordered deployment, ordered termination, and persistent volume claims. Ordered deployment and termination ensure that pods are started and stopped in a specific order, which is important for stateful applications that require a specific initialization sequence. Persistent volume claims enable pods to request a specific volume with a specific storage class and access mode, which provides consistent, predictable storage for stateful applications.
apiVersion: apps/v1 kind: StatefulSet metadata: name: my-stateful-app spec: replicas: 3 serviceName: my-stateful-app selector: matchLabels: app: my-stateful-app template: metadata: labels: app: my-stateful-app spec: containers: - name: my-app-container image: my-stateful-app-image:latest ports: - containerPort: 8080 env: - name: ENV_VAR_1 value: "value1" - name: ENV_VAR_2 value: "value2" volumeClaimTemplates: - metadata: name: my-app-data spec: accessModes: [ "ReadWriteOnce" ] resources: requests: storage: 1Gi
This manifest file creates a StatefulSet called "my-stateful-app" with three replicas. It uses a container image called "my-stateful-app-image:latest" and exposes port 8080. It also sets two environment variables called "ENV_VAR_1" and "ENV_VAR_2". It also creates a persistent volume claim called "my-app-data" with a storage size of 1Gi.
DaemonSet
A DaemonSet ensures that a specific pod is running on every node in the cluster. DaemonSets are commonly used for system-level applications, such as log collectors or monitoring agents, that need to be deployed on every node in the cluster. DaemonSets ensure that each node has its own instance of the pod, and that the pod is automatically rescheduled if a node fails. This makes it possible to deploy system-level applications that require node-level access, such as host-level metrics or logs.
DaemonSets also provide several features that are specific to system-level applications, such as host-level access and node affinity. Host-level access enables system-level applications to access host-level resources, such as CPU or memory usage, which provides more granular monitoring and management capabilities. Node affinity enables DaemonSets to be scheduled on specific nodes, based on node labels or other criteria, which provides more control over where system-level applications are deployed.
apiVersion: apps/v1 kind: DaemonSet metadata: name: my-daemon spec: selector: matchLabels: app: my-daemon template: metadata: labels: app: my-daemon spec: hostNetwork: true containers: - name: my-daemon-container image: my-daemon-image:latest volumeMounts: - name: var-log mountPath: /var/log volumes: - name: var-log hostPath: path: /var/log
This manifest file creates a DaemonSet called "my-daemon" that runs on each node in the Kubernetes cluster. It uses a container image called "my-daemon-image:latest" and mounts the host's "/var/log" directory to the container's "/var/log" directory.
Job
A Job is used to run a single instance of a task to completion. Jobs are commonly used for batch processing or other short-lived tasks. Jobs guarantee that a task runs to completion, and they provide mechanisms for handling errors and retries. This makes it possible to run batch jobs or other short-lived tasks on the Kubernetes cluster, without having to manually manage the lifecycle of each task.
Jobs also provide several features that are specific to batch processing or short-lived tasks, such as parallelism and backoff limits. Parallelism enables multiple instances of a job to run in parallel, which can speed up the processing of large data sets or other compute-intensive tasks. Backoff limits enable you to specify the number of times a job should be retried if it fails, which provides greater resiliency and reliability for batch processing tasks.
apiVersion: batch/v1 kind: Job metadata: name: my-job spec: backoffLimit: 3 template: spec: containers: - name: my-job-container image: my-job-image:latest args: ["--arg1=value1", "--arg2=value2"] restartPolicy: Never
This manifest file creates a Job called "my-job" that runs a container image called "my-job-image:latest" with arguments "--arg1=value1" and "--arg2=value2". It specifies a backoff limit of 3 retries and a restart policy of "Never". Once the Job completes, the pod will be terminated.
CronJob
A CronJob is used to run a job on a scheduled basis, similar to a cron job in a Linux system. CronJobs are commonly used for tasks that need to be run at regular intervals, such as backups, data cleaning, or report generation. CronJobs provide the ability to specify a schedule, such as every hour or every day, and they automatically create and manage jobs based on that schedule.
CronJobs also provide several features that are specific to scheduled jobs, such as job history and concurrency policies. Job history enables you to view the status and results of previous job runs, which can be useful for troubleshooting and auditing purposes. Concurrency policies enable you to specify how CronJobs should handle overlapping job runs, such as whether to allow concurrent runs or to limit the number of concurrent runs.
apiVersion: batch/v1beta1 kind: CronJob metadata: name: my-cronjob spec: schedule: "*/5 * * * *" jobTemplate: spec: template: spec: containers: - name: my-cronjob-container image: my-cronjob-image:latest args: ["--arg1=value1", "--arg2=value2"] restartPolicy: OnFailure
This manifest file creates a CronJob called "my-cronjob" that runs a container image called "my-cronjob-image:latest" with arguments "--arg1=value1" and "--arg2=value2" every 5 minutes. It specifies a restart policy of "OnFailure", meaning that the Job will be restarted if it fails.
In conclusion, Kubernetes provides several types of workloads to support different types of applications and tasks. By understanding the characteristics and use cases of each workload, you can choose the appropriate workload for your application or task, and take advantage of the advanced features provided by Kubernetes to deploy, manage, and scale your application with ease.
Subscribe to my newsletter
Read articles from Vipul Vyas directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
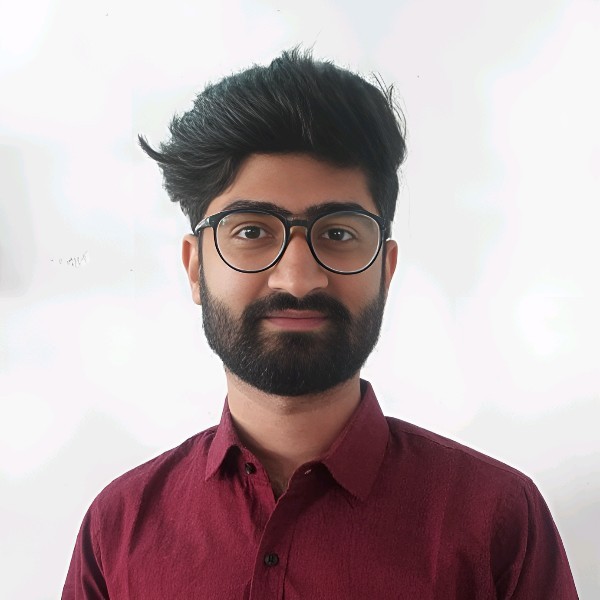