Exploring Object-Oriented Programming: Principles, Implementation, and Benefits
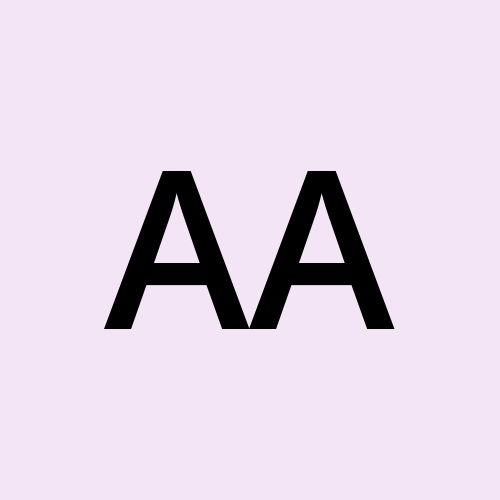
Table of contents
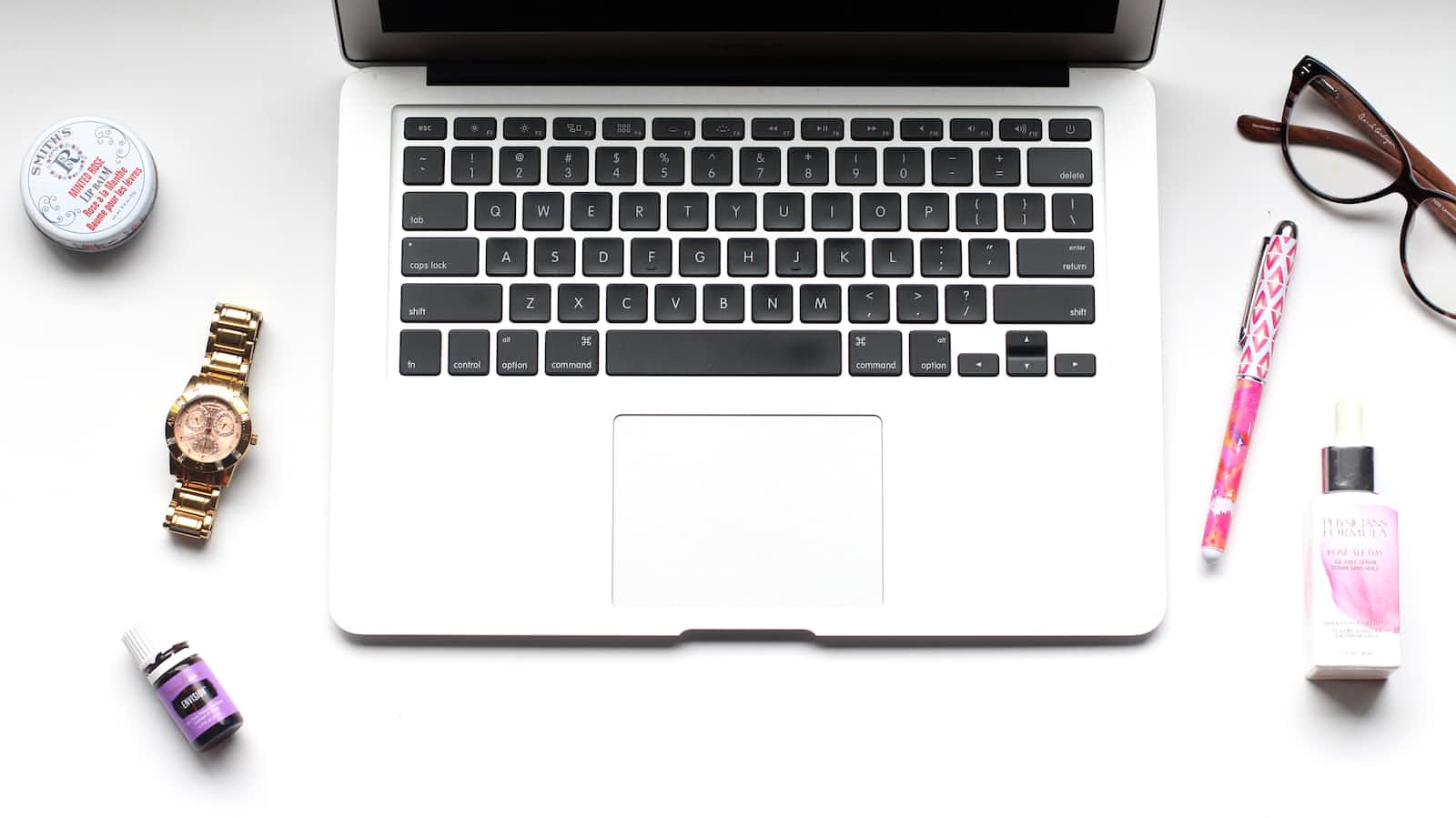
Object-oriented programming (OOP) is a programming paradigm based on the concept of objects. Each object has its own properties and methods.
In OOP, objects consist of data and functions. They both interact with one another through interfaces. "Objects are instances of classes that represent components." A class is a blueprint that defines the properties and behaviors of an object.
OOP, in software development, allows developers to organize and create reusable components. OOP achieves this by encapsulating data and code within objects, allowing them to be manipulated and modified without affecting other parts of the program. This leads to the breakdown of complex systems into smaller components. The goal is to simplify the software testing and debugging process.
Some popular programming languages that use OOP include JavaScript, Java, C#, C++, Python, and Ruby. Understanding OOP principles is an essential skill for programmers of all levels.
Key principles of OOP
This includes inheritance, encapsulation, abstraction, and polymorphism.
- Encapsulation is the process of hiding the implementation details of an object. This helps prevent other parts of the code from modifying an object's internal state.
For example, let's say you're creating a social media platform that allows users to create posts and interact with each other. To protect the privacy and security of user data, you might encapsulate the user object to prevent other parts of the code from accessing sensitive information, such as the user's email address or password.
- Inheritance allows the creation of new classes from existing ones, which are often referred to as "parent" or "base" classes. The child classes inherit the attributes and behaviors of their parent classes. With this, developers can create reusable code and organize it.
For example, let's say you're creating a child. You might create a parent class for all children that includes properties like health, strength, and agility. You could then create subclasses for each child. Each subclass would inherit from the parent class but also have its unique attributes and behaviors.
- Polymorphism allows objects to take on multiple forms, allowing for flexibility in design and implementation. This can help create more adaptable and reusable code.
For example, you're creating a drawing program that supports different shapes. You might create a parent class for shapes such as circles, squares, and triangles. subclass would have its implementation of methods like "draw" and "calculateArea." You could then use polymorphism to create a list of different shapes and call the same "draw" method on each shape. All shapes would be drawn in their unique way, but the code that calls the "draw" method wouldn't need to know the specific type of shape.
- Abstraction is the process of simplifying complex systems by breaking them down into smaller, more manageable components. And this helps make the code easier to understand and maintain.
For example, let's say you're building a car rental application that allows users to search for and book rental cars. You might create an abstract car class to provide fundamental properties such as the automobile's make, model, and year. Then you may develop a set of interfaces, such as ICarSearch and ICarBooking, that describe how the user can search for and book rental automobiles. The parent class would abstract away the details of each car, making it easier to manage and update the code.
Implementation
To implement OOP in a programming language, you'll typically need to create classes and objects, define constructors, and use inheritance and polymorphism. Here's a breakdown of each step:
Creating classes and objects: To create a class, you use the class keyword followed by the name of the class. For example, to create the
Employee
class:public class Employee { protected string name; public Employee(string name) { this.name = name; } }
To create an object, you use the
new
keyword followed by the name of the class and the arguments for the constructor. For example, to create a newEmployee
object:
Employee employee = new Employee("John");
- Defining constructors: constructors are methods that are called when an object is created. To define a constructor, you create a method with the same name as the class. For example, the
Employee
class has a constructor that takes a name parameter:
public Employee(string name)
{
this.name = name;
}
- Using inheritance: To create a new subclass, you use the :(inheritance symbol) symbol (to inherit from the parent) followed by the name of the parent class. For example, the
FrontendDeveloper
class inherits from theEmployee
class:
public class FrontendDeveloper : Employee
{
private string programmingLanguage;
public FrontendDeveloper(string name, string programmingLanguage) : base(name)
{
this.programmingLanguage = programmingLanguage;
}
public void WriteCode()
{
Console.WriteLine("Frontend Developer " + name + " is writing code in " + programmingLanguage + ".");
}
}
- Using polymorphism: To use polymorphism, you create a parent class with abstract methods and then create subclasses that implement those methods in their unique way. For example, the
Employee
class can be made abstract with the abstract methodDoWork()
, and the subclassesFrontendDeveloper
,UIDesigner
, andBackendDeveloper
can implement the method in their unique way:
public abstract class Employee
{
protected string name;
public Employee(string name)
{
this.name = name;
}
public abstract void DoWork();
}
public class FrontendDeveloper : Employee
{
private string programmingLanguage;
public FrontendDeveloper(string name, string programmingLanguage) : base(name)
{
this.programmingLanguage = programmingLanguage;
}
public override void DoWork()
{
Console.WriteLine("Frontend Developer " + name + " is writing code in " + programmingLanguage + ".");
}
}
public class UIDesigner : Employee
{
private string designTools;
public UIDesigner(string name, string designTools) : base(name)
{
this.designTools = designTools;
}
public override void DoWork()
{
Console.WriteLine("UI Designer " + name + " is designing UI using " + designTools + ".");
}
}
public class BackendDeveloper : Employee
{
private string programmingLanguage;
public BackendDeveloper(string name, string programmingLanguage) : base(name)
{
this.programmingLanguage = programmingLanguage;
}
public override void DoWork()
{
Console.WriteLine("Backend Developer " + name + " is writing code in " + programmingLanguage + ".");
}
}
Conclusion
In conclusion, object-oriented programming (OOP) simplifies the maintenance and modification of code by allowing data and behavior to be managed through objects and classes. By using OOP features such as encapsulation, inheritance, and polymorphism, code can be organized, reduced in redundancy, and reused. Many OOP languages are popular in the industry because of OOP principles. Developing with OOP can increase developer productivity, improve code quality, and speed up a system. Developers need this tool to learn and use it effectively.
Resources
You will find the following resources helpful:
Subscribe to my newsletter
Read articles from Abdulrasheed Abdulsalam directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
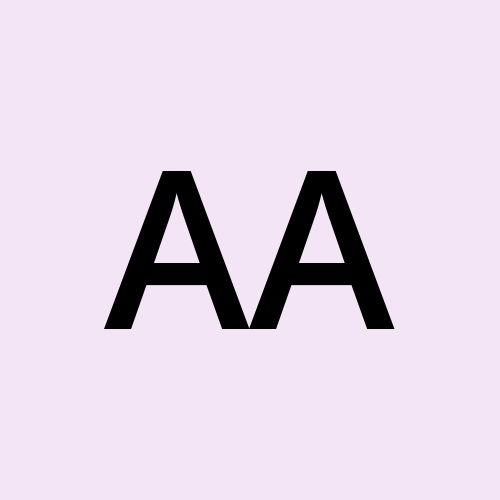