What is a Race Condition and How to Prevent it in Your Programs

Table of contents
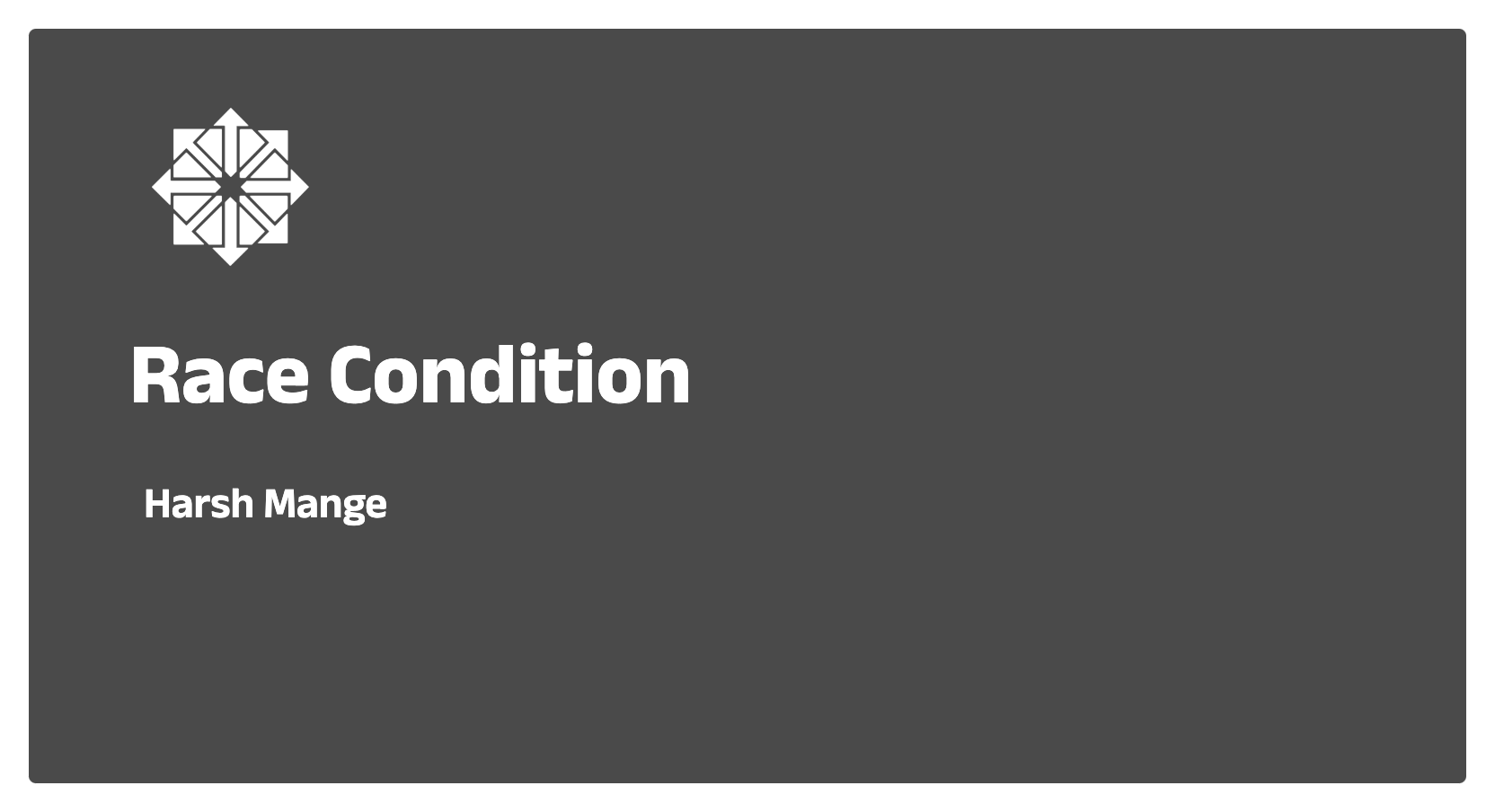
What the heck is Race Condition?
A race condition is a software bug that occurs when the correctness of a system depends on the order or timing of events that occur in the system, but the order or timing is not guaranteed. In other words, it is a situation where the behavior of a program depends on the relative timing of events that are not under its control.
Explanation
Imagine a simple program that has two threads that both increment a shared variable. The first thread reads the value of the variable, adds one to it, and writes the result back to the variable. The second thread does the same thing. If the two threads run at the same time, a race condition can occur. For example, suppose the shared variable starts with the value 0. Thread 1 reads the value and adds one, getting 1. Before it writes the value back to the variable, thread 2 reads the value, also getting 0. Thread 2 then adds one, getting 1, and writes it back to the variable. Then thread 1 writes its value, which is also 1. The result is that the variable ends up with the value 1 instead of 2, which is the expected result.
Example
Suppose we have two threads running concurrently in a Java program. Thread 1 is responsible for incrementing a shared integer variable count
by 1, while Thread 2 is responsible for printing the value of count
to the console. If both threads run simultaneously, we may encounter a race condition where the value printed is not the value incremented by Thread 1.
// Global variable
int count = 0;
// Thread 1
void incrementCount() {
count++; // increment the shared variable
}
// Thread 2
void printCount() {
System.out.println(count); // print the shared variable
}
Possible Solutions
To avoid race conditions, we can use synchronization mechanisms, such as locks, semaphores, or monitors, to ensure that only one thread at a time can access and manipulate shared resources. Here's an example of how we can use a synchronized
block to protect the critical section of the code:
// Global variable
int count = 0;
// Thread 1
void incrementCount() {
synchronized(this) { // acquire lock on this object
count++; // increment the shared variable
} // release lock on this object
}
// Thread 2
void printCount() {
synchronized(this) { // acquire lock on this object
System.out.println(count); // print the shared variable
} // release lock on this object
}
In this example, we use the synchronized
block to acquire a lock on the object that both threads share (this
), ensuring that only one thread at a time can execute the critical section of code. This guarantees that the increment operation and the print operation are performed atomically and sequentially, preventing a race condition.
Race conditions can be avoided by using synchronization techniques to ensure that only one thread can access the shared resource at a time. For example, in the above example, the program could use a lock to ensure that only one thread can access the shared variable at a time. When a thread wants to access the variable, it would acquire the lock, and release it when it was done. This would ensure that no two threads could access the variable at the same time, and therefore avoid the race condition.
Another way to avoid race conditions is to use atomic operations. An atomic operation is an operation that appears to be indivisible from the perspective of other threads. In the above example, if the program used an atomic operation to increment the shared variable, then the operation would appear to other threads as if it were indivisible, even if it was implemented using multiple low-level operations. This would ensure that no race condition could occur.
Subscribe to my newsletter
Read articles from Harsh Mange directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Harsh Mange
Harsh Mange
This is Harsh Mange, working as a Software Engineer - Backend at Argoid. I love building apps. Working on contributing to open-source projects and the dev community.