How to convert hexadecimal to decimal and binary with only using character input in scanf, loops and conditional statement.

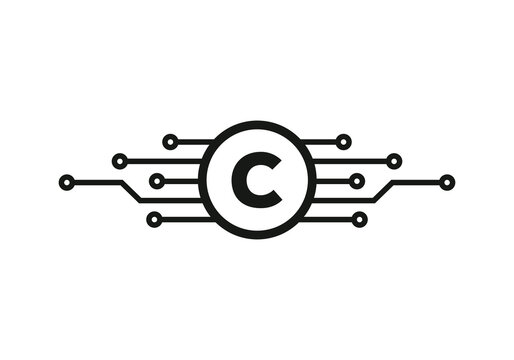
This C program converts a hexadecimal input into its equivalent decimal and binary values. The program reads the user's input character by character until the user hits the enter key. This code can be helpful in developing programs that require conversion between different number systems.
Here's a detailed explanation of the code:
#include<stdio.h>
The above line includes the standard input-output header file.
int main() {
The program begins by defining the main function, which returns an integer value.
char hex;
int dec = 0;
int d=0;
These lines declare three integer variables, 'dec', 'd' and a character variable 'hex'.
printf("Enter a hexadecimal number: ");
scanf("%c", &hex);
This code prompts the user to input a hexadecimal number and reads the input character by character using scanf() function.
while (hex != '\n')
{
if (hex >= '0' && hex <= '9') {
d = (hex - '0');
}
else if (hex >= 'A' && hex <= 'F') {
d = (hex - 'A' + 10);
}
else if (hex >= 'a' && hex <= 'f') {
d = (hex - 'a' + 10);
}
dec= dec * 16+d;
scanf("%c", &hex);
}
This block of code converts the hexadecimal input to decimal by iterating over each character in the input using a while loop. If the character is a digit from 0 to 9, its value is assigned to the variable 'd' using the expression (hex - '0')
. If the character is a letter from A to F or a to f, its value is assigned to 'd' using the expression (hex - 'A' + 10)
or (hex - 'a' + 10)
, respectively. The final decimal value is computed by multiplying the previous value by 16 and adding the new value.
int n,r=0,m=1,bin=0;
n=dec;
while(n!=0)
{
r=n%2;
bin=bin+(r*m);
m=m*10;
n=n/2;
}
This code block converts the decimal value obtained from the previous step to its equivalent binary value. The while loop runs until the decimal value is not zero. Inside the loop, the remainder of dividing the decimal value by 2 is calculated using the expression n%2
, which is stored in 'r'. 'r' is then added to the binary value by multiplying it with the appropriate power of 10 and adding it to the 'bin' variable. The power of 10 is stored in the 'm' variable, which is initialized to 1 and multiplied by 10 in each iteration. The decimal value is then divided by 2 to obtain the next digit in the binary value.
printf("\nBinary Value : %d",bin);
return 0;
}
Finally, the binary value is printed on the screen using printf() function, along with an appropriate message. The main function then returns 0 to indicate successful completion of the program.
In conclusion, the above code is a C program that converts a hexadecimal input into its equivalent decimal and binary values. Understanding this code can be helpful in developing programs that require conversion between different number systems.
Subscribe to my newsletter
Read articles from Swapnil Sinha directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
