Understanding `this` keyword in JavaScript
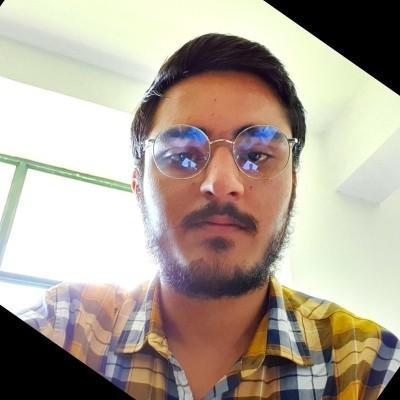
Table of contents
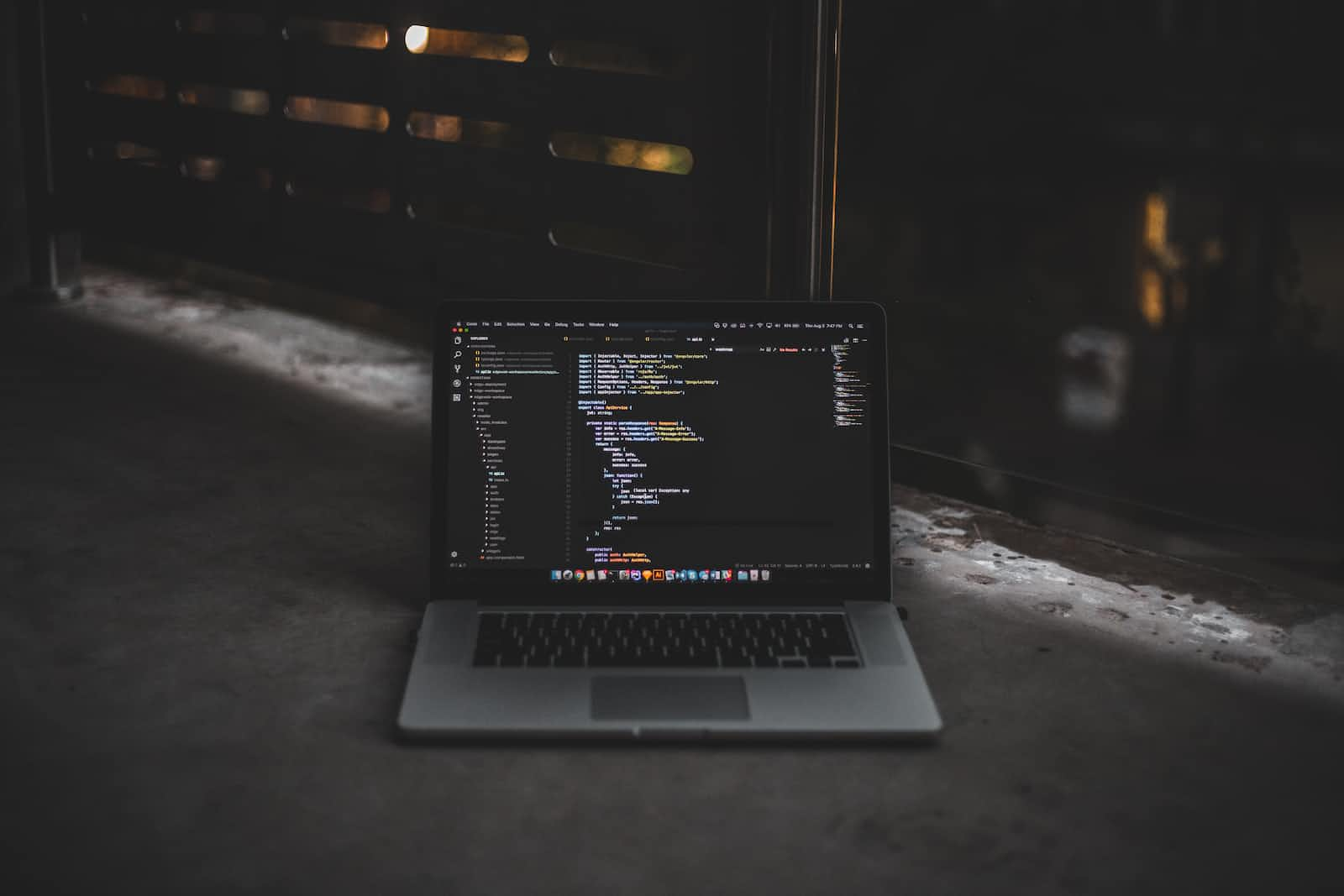
this
keyword in JavaScript is a common source of confusion for many developers, especially those who are new to the language. It can be used in a variety of contexts and can behave differently depending on the situation. In this article, we will explore this
keyword in-depth and explain how it works in various scenarios.
What is this
keyword?
In JavaScript, this
refers to the current context or object. It can be used inside a function or method to refer to the object that the function is a property of or the object that is being acted upon. It allows us to access and manipulate object properties concisely and conveniently.
How this
works
The behavior of this
keyword depends on how it is used. Here are the main ways it can be used:
1. Global context
When used in the global context (outside any function or object), this
refers to the global object. In a web browser, this is typically the window
object. For example:
console.log(this); // logs the global object (e.g. window in a web browser)
2. Function context
When used inside a function, this
refers to the object that the function is a property of. For example:
const person = {
name: 'John',
sayHi() {
console.log(`Hi, my name is ${this.name}`);
}
};
person.sayHi(); // logs "Hi, my name is John"
In this example, this
refers to the person
object, since sayHi()
is a property of that object.
However, if the function is called in the global context, this
will again refer to the global object:
const sayHi = person.sayHi;
sayHi(); // logs "Hi, my name is undefined"
In this case, this
refers to the global object (window
in a browser), because the function is being called in the global context, not as a property of the person
object.
3. Method context
When used inside a method of an object, this
refers to the object that the method is a property of. For example:
const person = {
name: 'John',
address: {
city: 'New York',
getCity() {
console.log(this.city);
}
}
};
person.address.getCity(); // logs "New York"
In this example, this
refers to the address
object, since getCity()
is a property of that object.
4. Constructor context
When used inside a constructor function, this
refers to the instance of the object being created. For example:
function Person(name) {
this.name = name;
this.sayHi = function() {
console.log(`Hi, my name is ${this.name}`);
};
}
const john = new Person('John');
john.sayHi(); // logs "Hi, my name is John"
In this example, this
refers to the instance of the Person
object being created (john
), since sayHi()
is a method of that object.
5. Arrow Function context
In contrast to regular functions, arrow functions do not have their own this
value. Instead, this
value of the enclosing lexical scope is used. In other words, the value of this
inside an arrow function is determined by the context in which it is defined, not where it is called.
Here's an example:
const obj = {
name: "John",
greet() {
setTimeout(() => {
console.log(`Hello ${this.name}`);
}, 1000);
}
};
obj.greet(); // Output: Hello John
In this example, the greet
method of the obj
object defines an arrow function inside the setTimeout
method. When the setTimeout
method is called, the arrow function is executed, and it logs the name property of the obj
object to the console.
Because the arrow function is defined inside the greet
method, it inherits this
value of its parent function, which is the obj
object. Therefore, the output of the above code is Hello John
.
Common Pitfalls with this
Keyword
this
keyword can be quite tricky to understand and use properly, and there are a few common pitfalls that developers might encounter when working with it. Here are some of the most common issues:
1. Losing the Context
One of the most common pitfalls with this
is losing the context or the value of this
within a function. This usually happens when a function is called without its context, which causes this
to be bound to the global object (e.g., the window
object in a web browser). For example:
const person = {
name: 'John',
sayHello() {
console.log(`Hello, my name is ${this.name}`);
}
};
// This will print "Hello, my name is John"
person.sayHello();
// However, if we assign the sayHello function to a variable,
// and then call it without its context, "this" will be bound
// to the global object, and the output will be "Hello, my name is undefined"
const hello = person.sayHello;
hello();
To avoid this issue, you can use the bind
, call
, or apply
methods to explicitly set the context of the function.
2. Confusion with Arrow Functions
Arrow functions are a relatively new addition to JavaScript, and they behave differently from regular functions when it comes to this
. Arrow functions do not have their own this
value, but instead, inherit the value of this
from the surrounding context. This can lead to confusion when using arrow functions within objects, as the value of this
may not be what you expect. For example:
const person = {
name: 'John',
sayHello: () => {
console.log(`Hello, my name is ${this.name}`);
}
};
// This will print "Hello, my name is undefined"
person.sayHello();
3. Using this
in Callback Functions
Callback functions are commonly used in JavaScript, but they can also cause issues with this
if not used properly. When a callback function is invoked, the value of this
is often different from what you might expect, as it is determined by the function that calls the callback, rather than the callback function itself. For example:
const person = {
name: 'John',
friends: ['Alice', 'Bob', 'Charlie'],
listFriends() {
this.friends.forEach(function(friend) {
console.log(`${friend} is a friend of ${this.name}`);
});
}
};
// This will print "undefined is a friend of Alice", "undefined is a friend of Bob", and "undefined is a friend of Charlie"
person.listFriends();
To avoid this issue, you can use the bind
method to explicitly set the context of the callback function, or use arrow functions, which inherit the context from the surrounding context.
Avoiding this
confusion
The behavior of the this
keyword can be tricky to understand, especially in complex code. Here are a few tips for avoiding this
confusion:
Use
bind()
,call()
, orapply()
to explicitly set the value ofthis
when calling a function.Use arrow functions, which have a lexical
this
binding and always refer to thethis
value of their surrounding context.
Conclusion
The this
keyword in JavaScript can be a source of confusion and frustration for many developers, especially those who are new to the language. However, once you understand how it works, it can be a powerful tool for writing more flexible and reusable code.
Remember that the value of this
is determined by the context in which a function is called, not where it is defined. Use the call
, apply
, and bind
methods to set this
value explicitly, and be careful when using arrow functions.
I hope this article has helped you gain a better understanding of this
keyword in JavaScript. Happy coding!
Subscribe to my newsletter
Read articles from Shivangam Soni directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
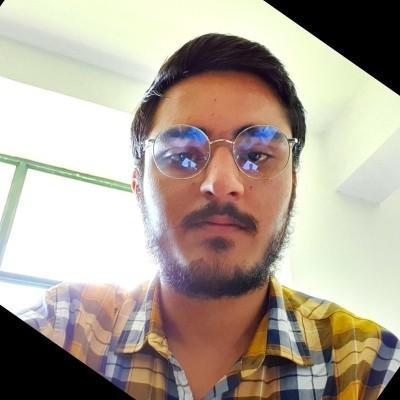
Shivangam Soni
Shivangam Soni
I'm an Aspiring Web Developer who enjoys writing code. I have strong Technical Skills & an Academic Background in Computer Science Engineering. My Focus is to be a Full Stack Web Developer & following that goal I've acquired skills in MERN Stack & I'm constantly working on it.