Handling props and state in ReactJS classbassed component

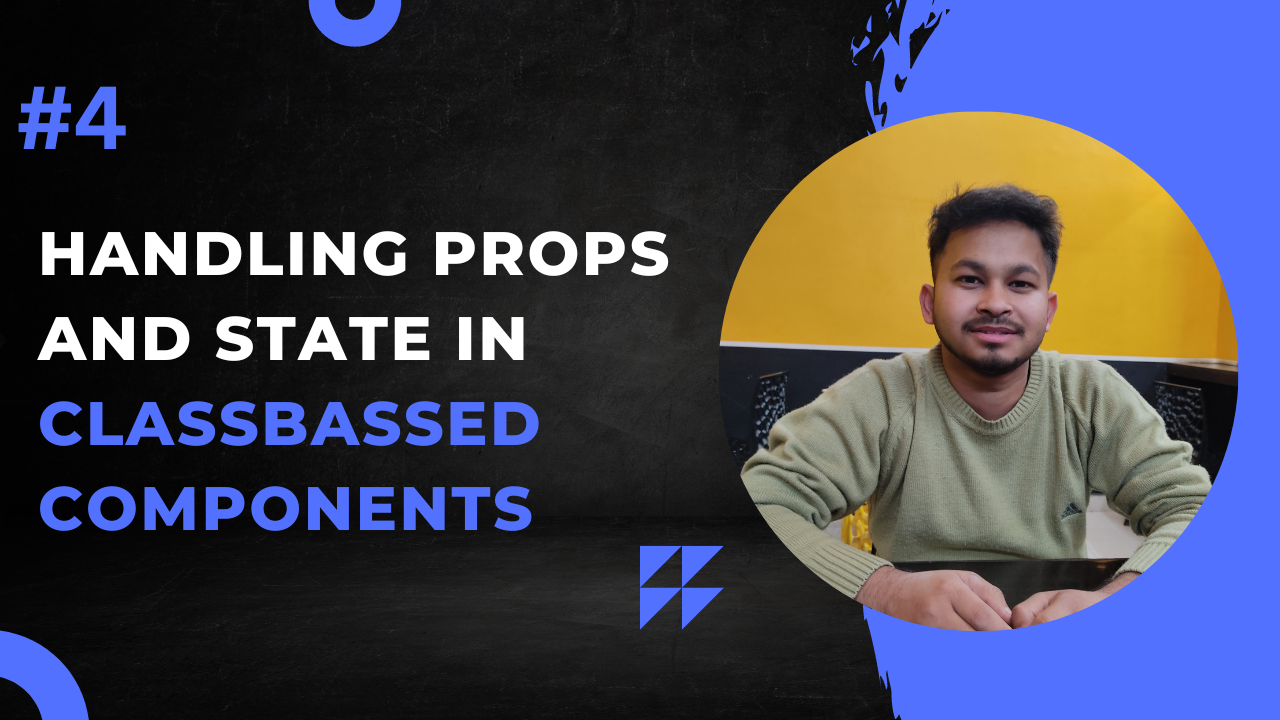
Here is the article for showing the state and props management in class-based components. Chances are less anybody is creating a new project in class-based components. Functional components are more popular than class-based components. Because to learn class-based components a programmer should have a good understanding of OOPs concepts. OOPs makes some difficulty with the beginner-to-learn class component.
handling State with Class component
If you are familiar with functional components we can use hooks there to create a state variable. here we do not have hooks but we can directly create a state variable in the constructor. We don't need to create it by calling hooks.
type P = {};
type S = {
userName: string;
};
class Welcome extends Component<P, S> {
constructor(props: P) {
super(props);
this.state = {
userName: "Himanshu",
};
}
render(): ReactNode {
return <div>Welcome, {this.state.userName}</div>;
}
}
export default Welcome;
In the above example, there is a component named Welcome. It is to show the welcome message to a new user. Managing the state in the class component is different from the functional component. here we need to pass an object to a nonstatic variable state. we can create multiple states just using this.
the code is in Typescript If not comfortable then you can ignore the typings in it.
And for setting a new value in the state react provides us a function that takes a new object and a new state value in it. Only an updated state key should be added to it. we don't need to spread all values in it.
function changeState(){
this.setState({userName:"Akhil Tiwari"})
}
This function is to update the user name in it. if there is more than one state Like userId or userPhone then also we don't need to spread all the values in the setState function.
handling Props with Class component
Here we can get props in a props object. In the class component, there is no such big difference in using props. here just we don't need to take from parameters we can just call it with this function.
type P = {
userName:string
};
type S = {};
class Welcome extends Component<P, S> {
constructor(props: P) {
super(props);
}
render(): ReactNode {
return <div>Welcome, {this.props.userName}</div>;
}
}
export default Welcome;
In the above example, we are getting the props and just showing them.
Passing the props are same as the function-based component. we just need to write the name as a tag in the parent component and pass the props in attributes.
import { Component, ReactNode } from "react";
import Welcome from "./Welcome"
class App extends Component{
render(): ReactNode {
return <div>
<Welcome userName="Himanshu Chauhan" />
</div>
}
}
export default App;
In this example, I am passing the username as a prop in the Welcome component.
Thank you for the reading๐๐๐. I hope this will help you a lot. Please comment with suggestions or queries.
Subscribe to my newsletter
Read articles from Himanshu Chauhan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Himanshu Chauhan
Himanshu Chauhan
A coder how works for excellence...