Twilio API: A Reference Guide to Building Twilio Integrations and Bots
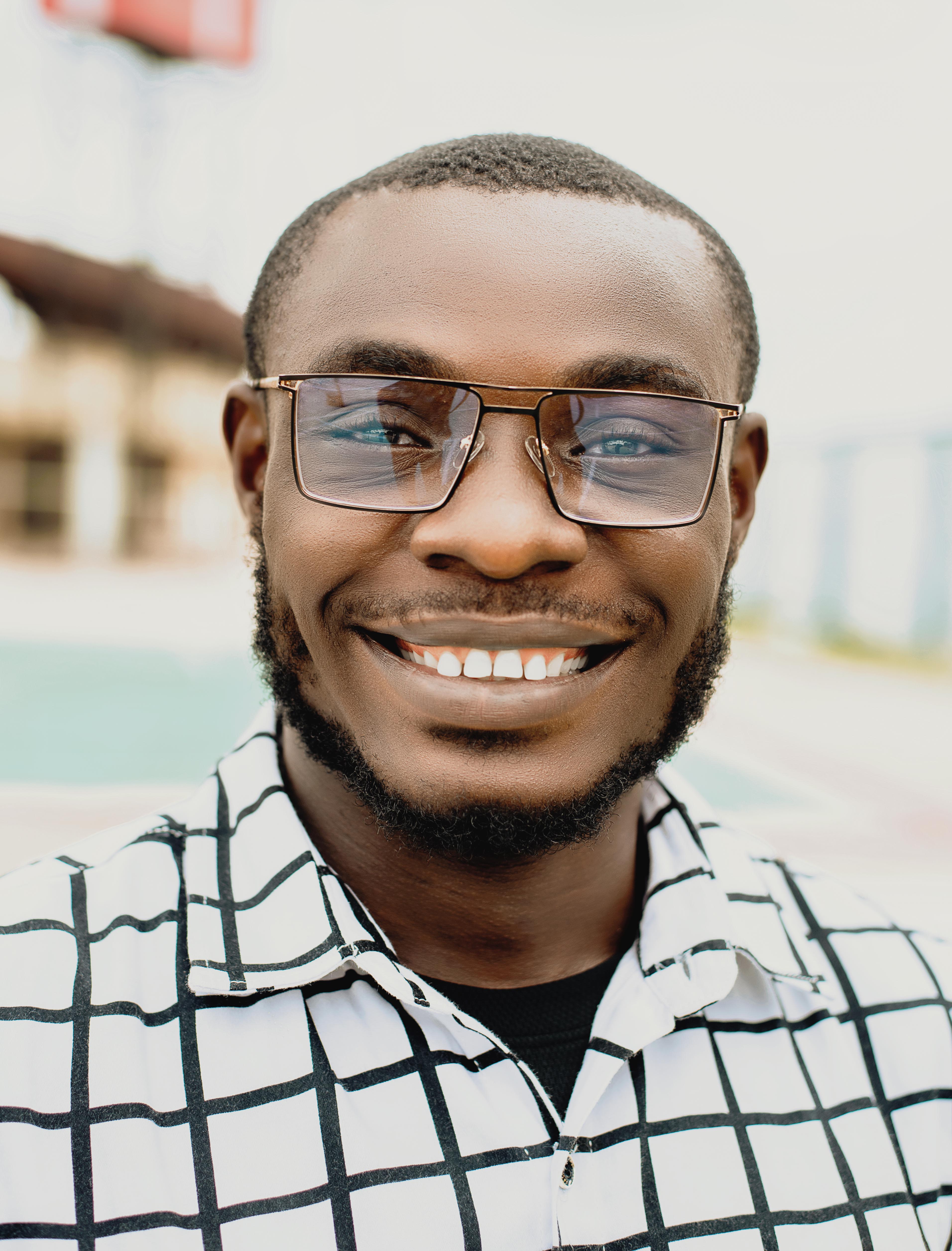
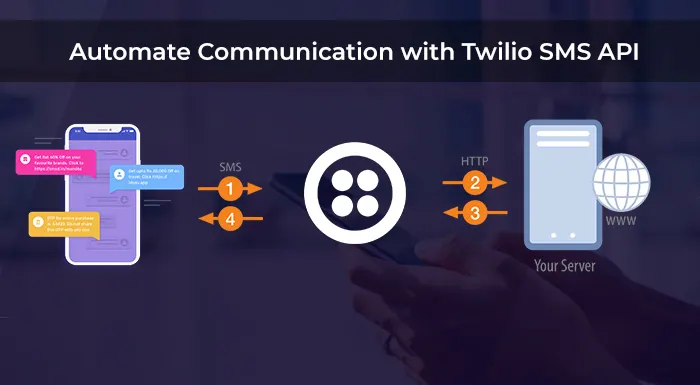
Seamless communication is one of the vital benefits of technological advancement. Organizations leverage many technologies to keep in touch with our potential users regardless of where they are, time zone or geographical location. Businesses bring their users closer now than ever, in that way real-time data is generated, informed decisions are made, and products and services are improved to give customers the best. Twilio provides a channel to achieve this feat. This is a reference guide, that looks through all you need to know in building integrations and bots with the Twilio API.
Introduction
Twilio is a platform that provides communication channels for organizations to engage with their users. These communication channels include SMS, voice, video, chat, and so on. Twilio's API aids developers in building integrations and bots that use these channels to effectively engage with users. Twilio API is easy to use, scalable, and reliable.
Features of Twilio API
Twilio API provides several features that make it attractive for building communication applications. Some of these features include:
Messaging: Twilio API provides a platform to send and receive text messages.
Voice: Twilio API provides tools for making and receiving phone calls, as well as creating voice applications.
Video: Twilio API supports video calls, video conferencing, as well as recording and live streaming.
Programmable Chat: Twilio API provides tools for building chat applications, including creating channels and messages, user authentication, and message history.
Setting up Twilio Account and API Keys
To use Twilio API, you have to create an account on their website. Create an account by following the sign-up process on their website. When you've created an account, navigate to the API key section of your Twilio console to obtain your API keys. The API key is unique and serves as a password to enable you to use the API.
Test your API key with Twilio Helper Library. The library provides functions that interact with Twilio API. Twilio Helper Library allows you to send SMS messages, make phone calls, and many more.
Here's an example of how to get your Twilio API key using the Twilio console:
from twilio.rest import Client
account_sid = 'your_account_sid'
auth_token = 'your_auth_token'
client = Client(account_sid, auth_token)
print(client.api.account.get().sid)
Twilio API authentication
Twilio uses Basic Authentication to authenticate API requests. API key and token are required in the header of each API request you make. Twilio provides client libraries for various programming languages, which make authentication easy.
Here's an example of how to authenticate your API requests using the Twilio Python client library:
from twilio.rest import Client
account_sid = 'your_account_sid'
auth_token = 'your_auth_token'
client = Client(account_sid, auth_token)
message = client.messages.create(
to='+1234567890',
from_='+19876543210',
body='Hello from Twilio!'
)
print(message.sid)
Twilio API documentation
It's the documentation that contains all the information you need to know about Twilio API. Always consult the documentation whenever you are at any point of confusion or don't know what to do. Twilio API documentation has information to help you get started, API references, code examples, and tutorials.
Here's an example of how to find the Twilio API documentation:
import webbrowser
url = 'https://www.twilio.com/docs/usage/api'
webbrowser.open_new_tab(url)
Twilio API Building Blocks
Twilio API is built on REST. REST is short for Representational State Transfer. REST API is an API that uses HTTP protocol to interact with its resources. The resources are represented at a predefined set of URLs. Each resource has its own set of HTTP methods that can interact with it.
Twilio API has a wide range of resource types, including accounts, applications, phone numbers, messages, and more. Authentication of your request using your API keys allows you to interact with these resources.
Twilio API resources and tools
Twilio offers various resources and tools to help developers build applications using its API.
Twilio Helper Libraries: Twilio Helper Library and SDKs (Software Development Kit) help you to work with Twilio API. These are client libraries that make it easy to integrate Twilio features into your application. SDK is a special set of instructions on how to use these functions.
These libraries and SDKs make it easier for developers to build integrations and bots. The library provides functions to interact with the Twilio API whilst SDK includes various programming languages such as Java, .NET, Python, Ruby, and more.
Twilio Console: This is a web-based tool that helps you to manage your Twilio account and access various features and tools.
Twilio API Explorer: Twilio API Explorer is a web-based tool that provides the interface for exploring Twilio API. It helps you browse the Twilio API documentation, make API requests, and see the API responses.
Twilio CLI (Command Line Interface): It's a command line interface that helps you to create and manage Twilio resources, deploy Twilio applications, and more.
Twilio Marketplace: This is a marketplace of pre-built integrations and add-ons that you can use to enhance your application.
Here's an example of how to use the Twilio Python client library to send an SMS message:
from twilio.rest import Client
account_sid = 'your_account_sid'
auth_token = 'your_auth_token'
client = Client(account_sid, auth_token)
message = client.messages.create(
to='+1234567890',
from_='+19876543210',
body='Hello from Twilio!'
)
print(message.sid)
Twilio API use cases and examples
Developers across the world add messaging, voice, and video to their applications using Twilio API. Examples of applications that you can build with the Twilio API:
SMS Messaging
Twilio SMS API allows developers to add SMS messaging features to their applications.
Code sample of sending SMS messaging using Twilio's API in Python:
from twilio.rest import Client # Your Account Sid and Auth Token from twilio.com/console account_sid = 'your_account_sid' auth_token = 'your_auth_token' client = Client(account_sid, auth_token) message = client.messages.create( body='Hello from Twilio!', from_='your_twilio_number', to='recipient_phone_number' ) print(message.sid)
Voice Calls
Twilio API allows developers to add calling and receiving call features in their applications or web projects.
Code sample of making a voice call using Twilio API in Python:
from twilio.rest import Client
# Your Account Sid and Auth Token from twilio.com/console
account_sid = 'your_account_sid'
auth_token = 'your_auth_token'
client = Client(account_sid, auth_token)
call = client.calls.create(
url='http://demo.twilio.com/docs/voice.xml',
to='recipient_phone_number',
from_='your_twilio_number'
)
print(call.sid)
Video Calls
Twilio video API allows developers to add video conferencing capabilities to their applications.
Code sample for creating a video room using Twilio's API in Python:
from twilio.rest import Client
# Your Account Sid and Auth Token from twilio.com/console
account_sid = 'your_account_sid'
auth_token = 'your_auth_token'
client = Client(account_sid, auth_token)
room = client.video.rooms.create(
unique_name='my-room-name'
)
print(room.sid)
Two-factor Authentication
Twilio's Verify API allows developers to add two-factor authentication to their applications.
Code samples for sending a verification code using Twilio API in Python:
from twilio.rest import Client
# Your Account Sid and Auth Token from twilio.com/console
account_sid = 'your_account_sid'
auth_token = 'your_auth_token'
client = Client(account_sid, auth_token)
verification = client.verify.services('your_service_sid') \
.verifications \
.create(to='recipient_phone_number', channel='sms')
print(verification.status)
Chat
Twilio's Chat API allows developers to add real-time chat to their applications.
Code sample for creating a chat channel using Twilio's API in Python:
from twilio.rest import Client
# Your Account Sid and Auth Token from twilio.com/console
account_sid = 'your_account_sid'
auth_token = 'your_auth_token'
client = Client(account_sid, auth_token)
channel = client.chat.services('your_service_sid') \
.channels \
.create(unique_name='my-channel-name')
print(channel.sid)
These are just a few examples of the many use cases and capabilities of Twilio's API. To learn more, visit the Twilio documentation at https://www.twilio.com/docs.
Best practices for building Twilio integrations and bots
To effectively build high-quality Twilio integrations and bots, some practices are to be considered. The following practices ensure that your applications are secure, reliable and scalable.
Use HTTPS when making API requests
Validate user input to prevent security vulnerabilities
Use rate limiting to prevent abuse of your application
Monitor your application for errors and performance issues
Implement security features, such as two-factor authentication
Test your integrations thoroughly before deploying them to production.
Follow Twilio's best practices for performance and scalability.
Conclusion
Developers across the world add SMS messaging, voice call, and video call to their applications using Twilio API. In this reference guide, we talked about Twilio API, how to get started, best practices for building integrations and bots, and use cases and examples. I hope this guideline finds you well and will help you build secure, reliable and scalable applications.
Subscribe to my newsletter
Read articles from Kelechukwu Awoke directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
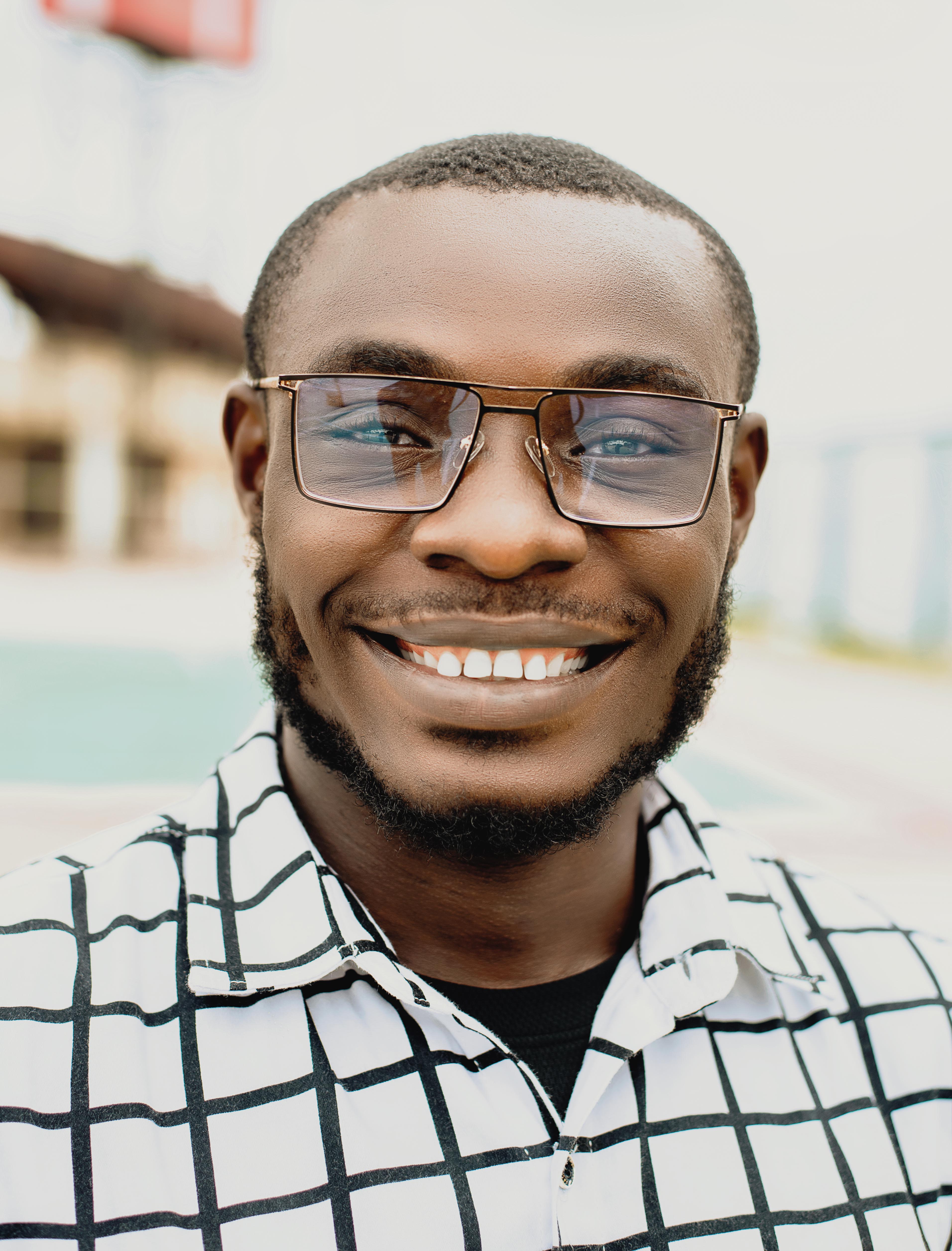
Kelechukwu Awoke
Kelechukwu Awoke
I am a Front End Developer. Technical writer. Tech enthusiast. Always optimistic!