Shell Scripting (Day 4 task)
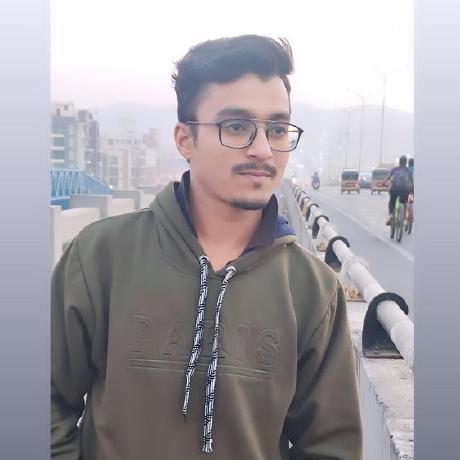
Table of contents
- 1.What is Linux Shell Scripting?
- 2.What is #!/bin/bash? can we write #!/bin/sh as well?
- 3.Write a Shell Script which prints
- I will complete #90DaysOofDevOps challenge
- 4.Write a Shell Script to take user input, input from arguments and print the variables.
- 5.Write an Example of If else in Shell Scripting by comparing 2 numbers
- Was it difficult?
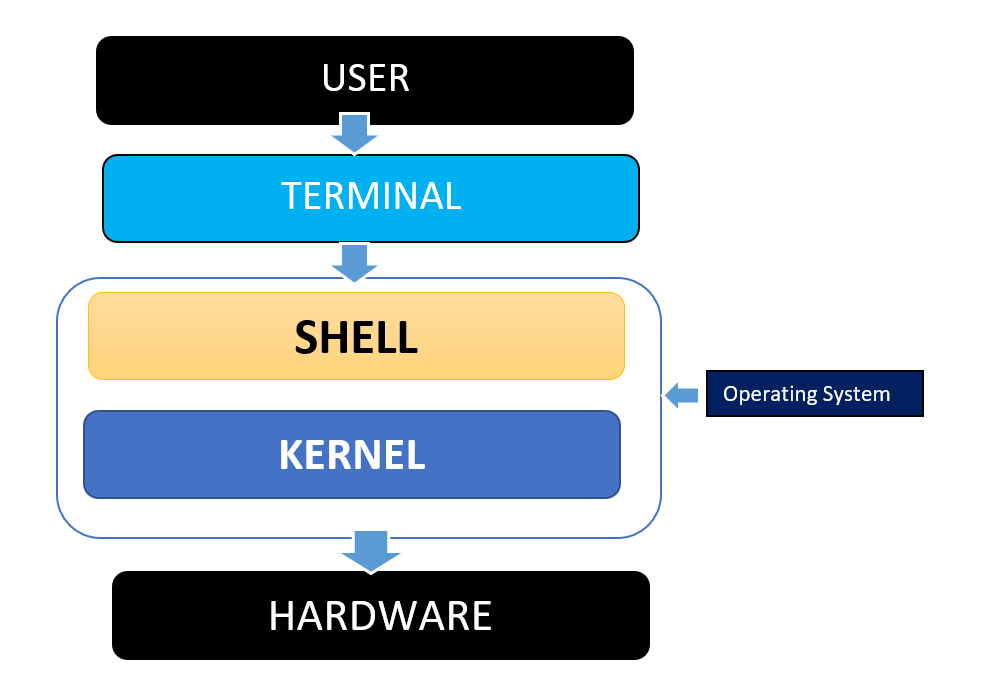
1.What is Linux Shell Scripting?
Linux shell scripting is the process of writing programs or scripts in the Linux shell (command-line interface) using various shell scripting languages such as Bash, sh, ksh, etc. These scripts can automate tasks, execute commands and programs, manipulate data, handle user inputs, and perform other system-related tasks in the Linux environment. Shell scripting is widely used by system administrators, developers, and power users to streamline their work and make it more efficient.
2.What is #!/bin/bash?
can we write #!/bin/sh
as well?
"#!/bin/bash" is known as the shebang or hashbang and is the first line of a shell script. It tells the system which interpreter to use to execute the script. In this case, it indicates that the script is a Bash shell script and should be executed using the Bash shell interpreter located at "/bin/bash". When the script is executed, this first line is read by the system and the specified interpreter is launched to execute the remaining lines of the script.
Yes, it is possible to use "#!/bin/sh" in place of "#!/bin/bash" as the shebang line in a shell script. In this case, the script will be executed using the "sh" shell interpreter, which is a simpler and more basic shell compared to Bash. However, please note that Bash is a superset of sh and includes additional features and functionality, which might not be available in sh. As a result, using "#!/bin/bash" might be preferred for more complex scripts that require additional features provided by Bash.
3.Write a Shell Script which prints
I will complete #90DaysOofDevOps challenge
Here is a short Bash shell script that prints the text "I will complete #90DaysOofDevOps challenge" when executed:
#!/bin/bash
echo "I will complete #90DaysOofDevOps challenge"
To execute this script, you would first need to save it to a file (e.g., "script.sh") and make it executable using the following command:
chmod +x script.sh
Then, you can run the script by typing its name:
./script.sh
This will execute the script and print the text "I will complete #90DaysOofDevOps challenge" to the terminal.
4.Write a Shell Script to take user input, input from arguments and print the variables.
To take user input and input from arguments in a shell script and print the variables, we can use the read
command to read user input and the $1
, $2
, etc. .Here's an example shell script that takes user input and input from arguments and prints the variables:
#!/bin/bash
# Read user input
echo "Enter a value:"
read user_input
# Read input from arguments
arg1=$1
arg2=$2
# Print the variables
echo "User input: $user_input"
echo "Argument 1: $arg1"
echo "Argument 2: $arg2"
In this script, the read
command is used to read user input and save it to the user_input
variable. The $1
and $2
variables are used to read input from the first and second arguments, respectively. The variables are then printed using the echo
command.To run this script, save it to a file (e.g., script.sh
), make it executable (chmod +x
script.sh
), and then run it with arguments (e.g., ./
script.sh
arg1 arg2
) or without arguments (e.g., ./
script.sh
).For example, if we run the script with the command ./
script.sh
hello world
, the output will be:
Enter a value:
User input:
Argument 1: hello
Argument 2: world
If we run the script without arguments (./
script.sh
), the output will be:
If we run the script without arguments (./
script.sh
), the output will be:
Enter a value:
hello
Argument 1:
Argument 2:
5.Write an Example of If else in Shell Scripting by comparing 2 numbers
Was it difficult?
Here is an example of how to use if-else statements in a Bash shell script to compare two numbers:
#!/bin/bash
# Assign two integer values
num1=10
num2=20
# Compare the values using conditional statements
if [ $num1 -gt $num2 ]
then
echo "$num1 is greater than $num2"
else
echo "$num2 is greater than $num1"
fi
In this script, two integer values are assigned to the variables "num1" and "num2". Then, an if-else statement is used to compare the values. The "-gt" operator.
Thank you,
Subscribe to my newsletter
Read articles from mehboob shaikh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
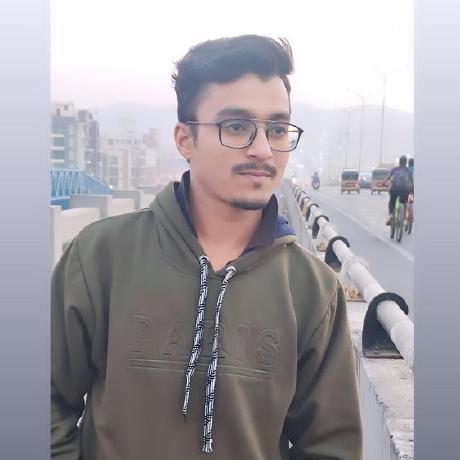