Serialization & Deserialization of JSON using Jackson
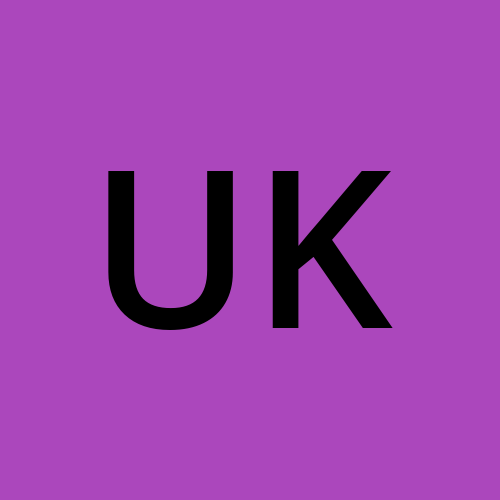
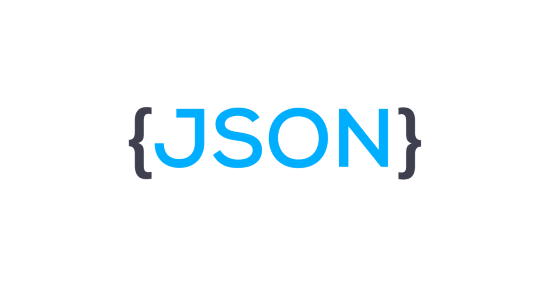
What is JSON?
How will a Python application communicate with a SpringBoot server? They need a common language to convey information, right? For this purpose, there are standard formats like JSON, XML etc, made use of for transferring data across systems.
JSON is a readable format with key-value pairs
[
{
"symbol": "ATL",
"quantity": 25,
"purchaseDate": "2023-01-02"
},
{
"symbol": "SFT"
"quantity": 10,
"purchaseDate": "2023-01-12",
}
]
The values can be any object, including String, Integer, Boolean, Array, or even another JSON structure, whereas the keys must be Strings.
Deserialization is the process of converting JSON into a Java object for processing, as opposed to serialization, which entails changing the Java object into JSON.
Reading JSON Jacksomatically
To parse JSON and store each stock data(mentioned in the above code snippet) as an object and print out the contents in a specific format. The key names have to be hardcoded, spaces have to be ignored, and not everything is inside quotes so you can’t go on vaguely finding quotes and getting stuff inside them. Consider a Trade class having trade objects.
ObjectMapper objectMapper = new ObjectMapper();
Trade[] trades = objectMapper.readValue(file, Trade[].class);
for (Trade trade : trades) {
System.out.println(trade);
}
Reading contents of the JSON file, store them as Trade
objects and print out the values as required. The readValue
method takes in the File
object for our JSON file as well as the class to which we need to Object Map the JSON contents to. We’ll read each stock data as a Trade
object to an Array
, which is why the second parameter is Trade[].class
Jackson ObjectMapper
provides a writeValue()
method to write Java objects back to JSON (serialization). The arguments are the deserialized data & the File
object to write it to.
Mapping JSON keywords
How does Jackson know which variable to map a JSON key to?
The variable names have to be the same as the key names.
public class Trade {
private String symbol;
private int quantity;
private String purchaseDate;
....
}
[
{
"symbol": "ATL",
"quantity": 25,
"purchaseDate": "2023-01-02"
},
{
"symbol": "SFT"
"quantity": 10,
"purchaseDate": "2023-01-12",
}
]
Jackson & Getters/Setters
Jackson by default doesn’t see variables with non-public access modifiers. We’ll have to provide getter & setter methods. This is how we add a getter & setter for the symbol
variable. Jackson uses the setter methods to deserialize JSON for non-public variables.
public String getSymbol() {
return symbol;
}
public void setSymbol(String symbol) {
this.symbol = symbol;
}
JACKSON Annotations
JACKSON Serialization Annotations
@JsonAnyGetter
The @JsonAnyGetter annotation allows for the flexibility of using a Map field as standard property.
@JsonGetter
The @JsonGetter annotation is an alternative to the @JsonProperty annotation, which marks a method as a getter method.
@JsonPropertyOrder
@JsonPropertyOrder annotation is used to specify the order of properties on serialization.
@JsonRawValue
@JsonRawValue annotation can instruct Jackson to serialize a property exactly as is.
@JsonValue
@JsonValue indicates a single method the library will use to serialize the entire instance.
@JsonRootName
The @JsonRootName annotation is used, if the wrapping is enabled, to specify the name of the root wrapper to be used.
@JsonSerialize
@JsonSerialize indicates a custom serializer to use when marshaling the entity.
JACKSON Deserialization Annotations
@JsonCreator
We can use the @JsonCreator annotation to tune the constructor/factory used in deserialization.
@JacksonInject
@JacksonInject indicates that a property will get its value from the injection and not from the JSON data.
@JsonAnySetter
@JsonAnySetter allows us the flexibility of using a Map as standard properties. On deserialization, the properties from JSON will simply be added to the map.
@JsonSetter
@JsonSetter is an alternative to @JsonProperty that marks the method as a setter method.
@JsonDeserialize
@JsonDeserialize indicates the use of a custom deserializer.
@JsonAlias
The @JsonAlias defines one or more alternative names for a property during deserialization.
JACKSON Property Inclusion Annotations
@JsonIgnoreProperties
@JsonIgnoreProperties is a class-level annotation that marks a property or a list of properties that Jackson will ignore.
@JsonIgnore
In contrast, the @JsonIgnore annotation is used to mark a property to be ignored at the field level.
@JsonIgnoreType
@JsonIgnoreType marks all properties of an annotated type to be ignored.
@JsonInclude
We can use @JsonInclude to exclude properties with empty/null/default values.
@JsonIncludeProperties
@JsonIncludeProperties used to mark a property or a list of properties that Jackson will include during serialization and deserialization.
@JsonAutoDetect
@JsonAutoDetect can override the default semantics of which properties are visible and which are not.
Subscribe to my newsletter
Read articles from Uday Kommireddy directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
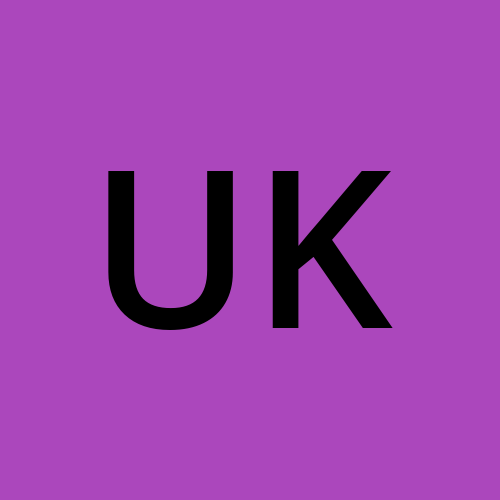