Preprocessor Directives in C++: Compiler Instructions and Conditional Compilation
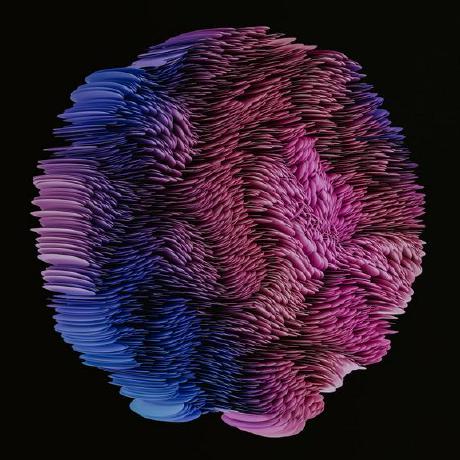
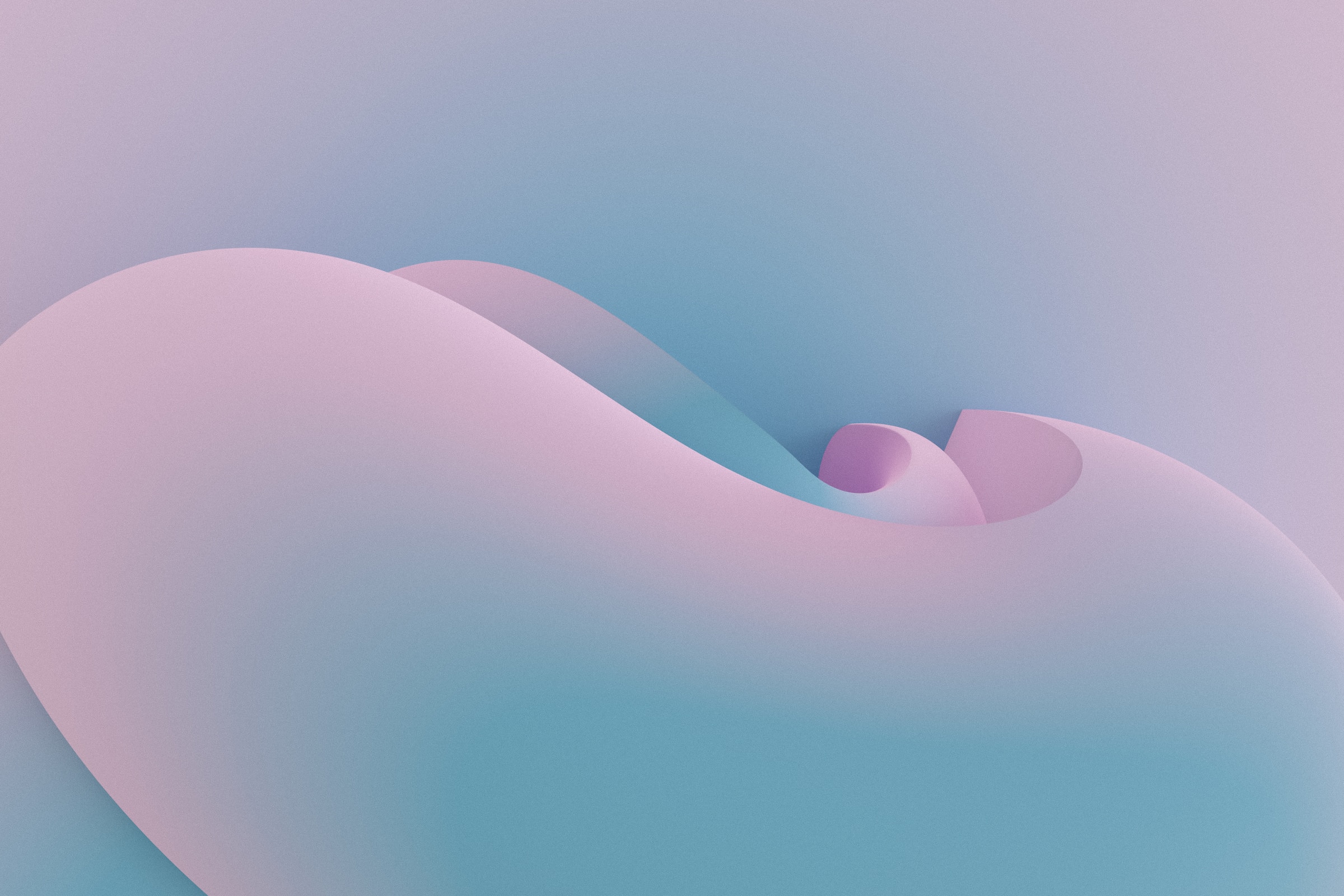
Preprocessor directives are instructions to the C++ compiler that are executed before the actual compilation process begins. They help you to manage the compilation process, include header files, define constants, and perform conditional compilation. In this article, we will discuss the most commonly used preprocessor directives in C++ and their applications.
#include Directive
The #include
directive is used to include the contents of a header file into your source code. This is essential for using library functions, classes, and other code components that are defined in separate files.
#include <iostream> //System headers
#include "myHeaderFile.h" //Your headers
#define Directive
The #define
directive allows you to define a constant or a macro. Constants are useful for defining values that will not change throughout the program, while macros can be used for simple text substitution.
#define PI 3.14159
#define SQUARE(x) ((x) * (x))
Notice that the function definition using the define directive is a bit different than what you've seen before.
#ifdef, #ifndef and #endif Directives
The #ifdef
, #ifndef
, and #endif
directives are used for conditional compilation. This allows you to compile specific sections of your code based on whether a certain macro is defined or not.
#define DEBUG
#ifdef DEBUG
std::cout << "Debug mode is enabled." << std::endl;
#else
std::cout << "Debug mode is disabled." << std::endl;
#endif
#if, #elif and #else Directives
The #if
, #elif
, and #else
directives are also used for conditional compilation. They allow you to test the value of a macro or a constant expression.
#define VERSION 2
#if VERSION == 1
std::cout << "Version 1" << std::endl;
#elif VERSION == 2
std::cout << "Version 2" << std::endl;
#else
std::cout << "Unknown version" << std::endl;
#endif
#undef Directive
The #undef
directive is used to undefine a macro or a constant. This can be useful in cases where you need to redefine a macro or use the same name for a different purpose.
#define PI 3.14159
#undef PI
#define PI 3.14
#pragma Directive
The #pragma
directive is a compiler-specific directive that can be used to give additional instructions to the compiler. The behavior of #pragma
directives is that they depend on the compiler being used, and not all compilers support the same set of #pragma
directives.
#pragma once // Ensures that the header file is included only once
Conclusion
Preprocessor directives in C++ are useful for managing the compilation process, including header files, defining constants, and performing conditional compilation. In this article, we covered the most commonly used preprocessor directives, such as #include, #define, #ifdef, #ifndef, #endif, #if, #elif, #else, #undef, and #pragma. Understanding and effectively using preprocessor directives can help you write more efficient and maintainable C++ code. In future articles, we will explore more advanced topics related to preprocessor directives and other important aspects of C++ programming.
Subscribe to my newsletter
Read articles from Enzo Hugonnier directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
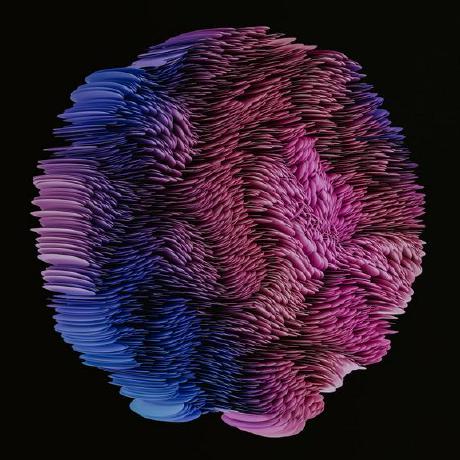