PGDAC | OOP Java | Collections Framework
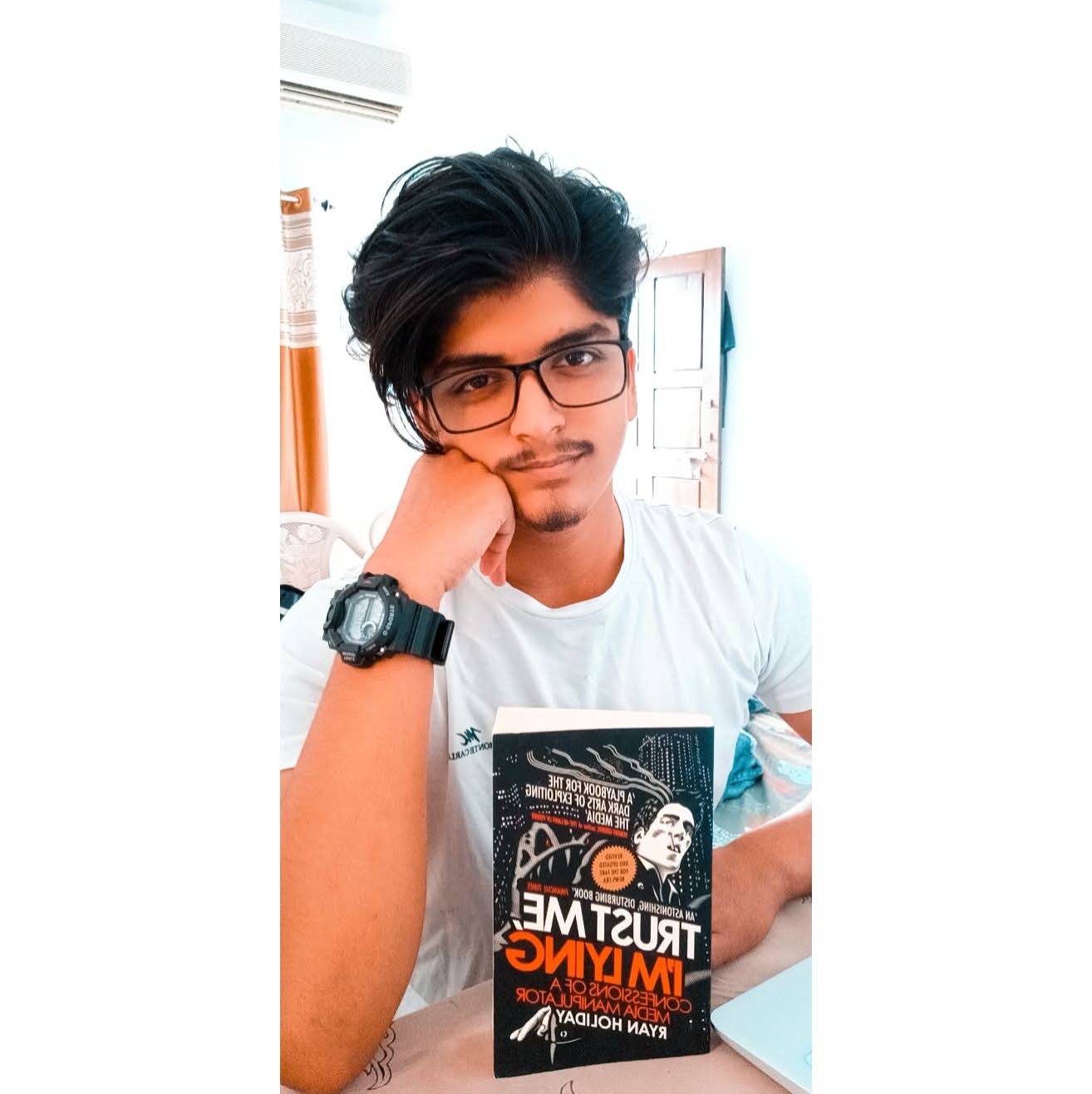

What are Collections?
Any group of individual objects which are represented as a single unit is known as a collection of objects. In Java, a separate framework named the “Collection Framework” has been defined in JDK 1.2 which holds all the collection classes and interface in it.
The Collection interface (java.util.Collection) and Map interface (java.util.Map) are the two main “root” interfaces of Java collection classes.
What is a Framework?
A framework is a set of classes and interfaces which provide a ready-made architecture. In order to implement a new feature or a class, there is no need to define a framework. However, an optimal object-oriented design always includes a framework with a collection of classes such that all the classes perform the same kind of task.
Hierarchy of the Collection Framework
The Collection Framework Hierarchy is shown below:
Before understanding the different components in the above framework, let’s first understand a class and an interface and basic definitions of ArrayList, Vector, Stack, LinkedList, HashMap, Queue and Dequeue
Class:
- A class is a user-defined blueprint or prototype from which objects are created. It represents the set of properties or methods that are common to all objects of one type.
Interface:
- Like a class, an interface can have methods and variables, but the methods declared in an interface are by default abstract (only method signature, nobody). Interfaces specify what a class must do and not how. It is the blueprint of the class.
ArrayList:
- The ArrayList class is a resizable array, which can be found in java.util package. The difference between a built-in array and an ArrayList in Java is that the size of an array cannot be modified (if you want to add or remove elements to/from an array, you have to create a new one). While elements can be added and removed from an ArrayList whenever you want.
Vector:
- The Vector class implements a growable array of objects. Vectors fall in legacy classes, but now it is fully compatible with collections. It is found in the java.util.package and implements the List interface. Vector implements a dynamic array which means it can grow or shrink as required. Like an array, it contains components that can be accessed using an integer index. They are very similar to ArrayList, but Vector is synchronized and has some legacy methods that the collection framework does not contain. It also maintains an insertion order like an ArrayList. Still, it is rarely used in a non-thread environment as it is synchronized, and due to this, it gives a poor performance in adding, searching, deleting, and updating its elements. The Iterators returned by the Vector class are fail-fast. In the case of concurrent modification, it fails and throws the ConcurrentModificationException.
Stack:
- Java Collection framework provides a Stack class that models and implements a Stack data structure. The class is based on the basic principle of last-in-first-out. In addition to the basic push and pop operations, the class provides three more functions empty, search, and peek. The class can also be said to extend Vector and treats the class as a stack with the five mentioned functions. The class can also be referred to as the subclass of Vector.
LinkedList:
- Linked List is a part of the Collection framework present in java.util package. This class is an implementation of the LinkedList data structure which is a linear data structure where the elements are not stored in contiguous locations and every element is a separate object with a data part and address part. The elements are linked using pointers and addresses. Each element is known as a node. Due to the dynamicity and ease of insertions and deletions, they are preferred over the arrays. It also has a few disadvantages like the nodes cannot be accessed directly instead we need to start from the head and follow through the link to reach a node we wish to access.
HashMap:
- HashMap<K, V> is a part of Java’s collection since Java 1.2. This class is found in java.util package. It provides the basic implementation of the Map interface of Java. It stores the data in (Key, Value) pairs, and you can access them by an index of another type (e.g. an Integer). One object is used as a key (index) to another object (value). If you try to insert the duplicate key, it will replace the element of the corresponding key.
Queue:
- The Queue interface is present in java.util package and extends the Collection interface is used to hold the elements about to be processed in FIFO(First In First Out) order. It is an ordered list of objects with its use limited to inserting elements at the end of the list and deleting elements from the start of the list, (i.e.), it follows the FIFO or the First-In-First-Out principle.
Deque:
- Deque interface present in java.util package is a subtype of the queue interface. The Deque is related to the double-ended queue that supports the addition or removal of elements from either end of the data structure. It can either be used as a queue(first-in-first-out/FIFO) or as a stack(last-in-first-out/LIFO). Deque is the acronym for double-ended queue. The Deque (double-ended queue) interface in Java is a subinterface of the Queue interface and extends it to provide a double-ended queue, which is a queue that allows elements to be added and removed from both ends. The Deque interface is part of the Java Collections Framework and is used to provide a generic and flexible data structure that can be used to implement a variety of algorithms and data structures.
Methods of the Collection Interface
This interface contains various methods which can be directly used by all the collections which implement this interface. Some of them are:
Method | Description |
add(Object) | This method is used to add an object to the collection. |
addAll(Collection c) | This method adds all the elements in the given collection to this collection. |
clear() | This method removes all of the elements from this collection. |
contains(Object o) | This method returns true if the collection contains the specified element. |
containsAll(Collection c) | This method returns true if the collection contains all of the elements in the given collection. |
equals(Object o) | This method compares the specified object with this collection for equality. |
hashCode() | This method is used to return the hash code value for this collection. |
isEmpty() | This method returns true if this collection contains no elements. |
iterator() | This method returns an iterator over the elements in this collection. |
max() | This method is used to return the maximum value present in the collection. |
parallelStream() | This method returns a parallel Stream with this collection as its source. |
remove(Object o) | This method is used to remove the given object from the collection. If there are duplicate values, then this method removes the first occurrence of the object. |
removeAll(Collection c) | This method is used to remove all the objects mentioned in the given collection from the collection. |
removeIf(Predicate filter) | This method is used to remove all the elements of this collection that satisfy the given predicate. |
retainAll(Collection c) | This method is used to retain only the elements in this collection that are contained in the specified collection. |
size() | This method is used to return the number of elements in the collection. |
spliterator() | This method is used to create a Spliterator over the elements in this collection. |
stream() | This method is used to return a sequential Stream with this collection as its source. |
toArray() | This method is used to return an array containing all of the elements in this collection. |
In the upcoming blogs, we will discuss the classes that implement the Interfaces of the Collections Framework
As always thank you so much for reading
I hope this helps
Happy Learning!
Subscribe to my newsletter
Read articles from Abdul Ahad Sheikh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
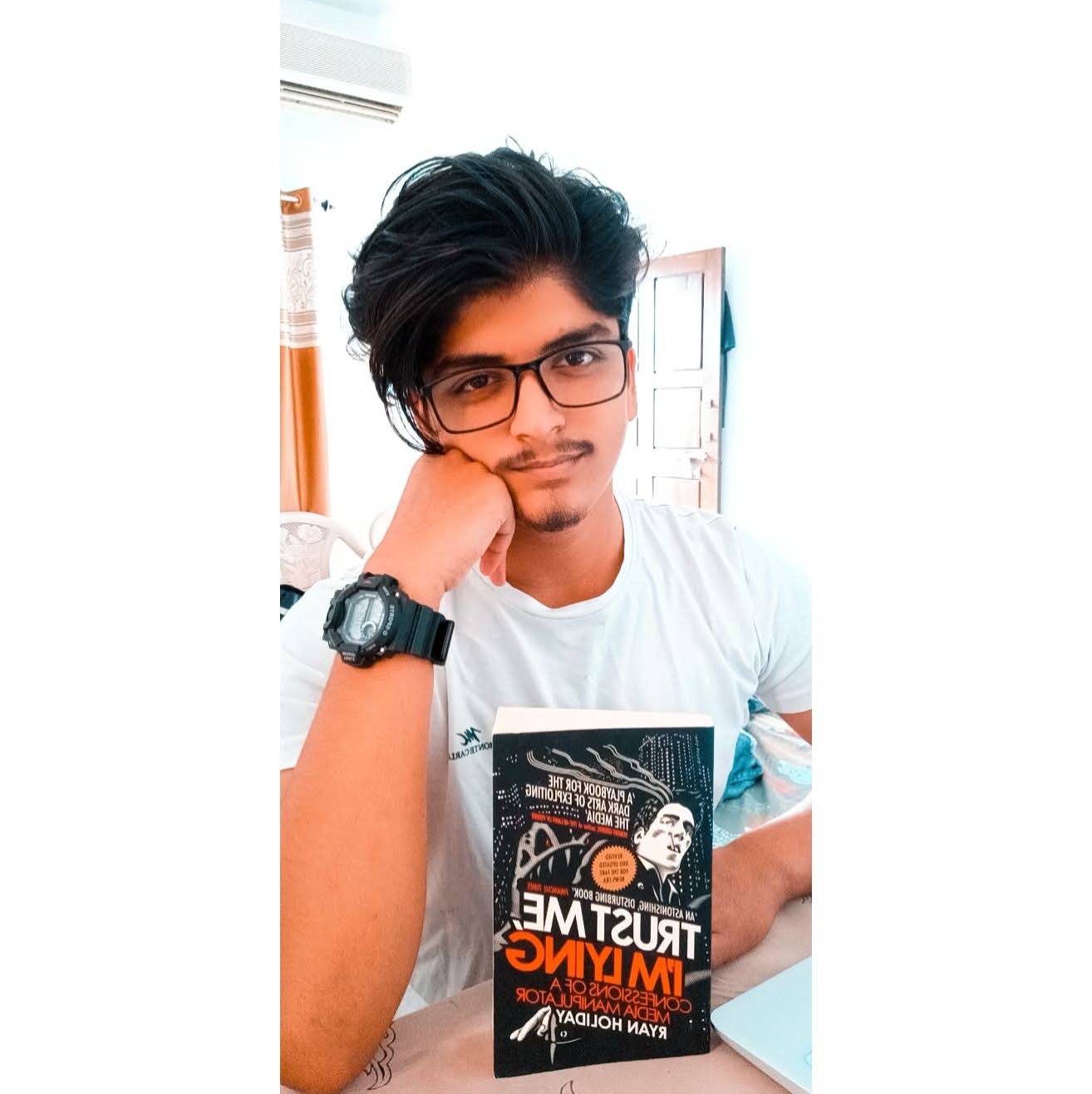
Abdul Ahad Sheikh
Abdul Ahad Sheikh
Learning Fullstack Development using Java and .Net Aspiring Public Speaker Cofounder @BookBrotherhood Part-time Musician🎶 and Writer✒️ Live😊 | Love❤️ | Laugh😁