Design Patterns: Template Method

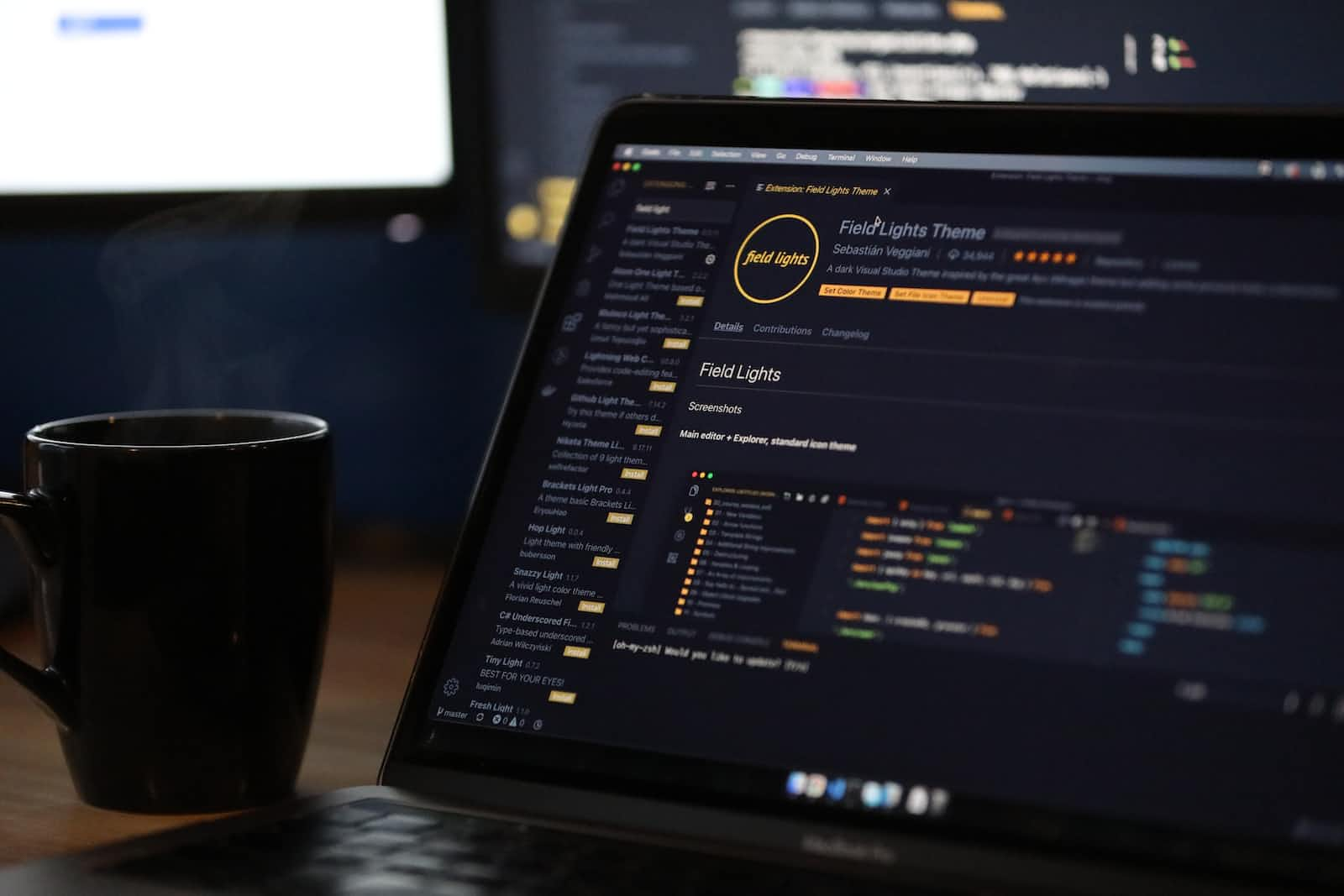
Template method
The Template method pattern is a behavioral design pattern that defines the skeleton of an algorithm in a base class while allowing subclasses to override or extend specific steps of the algorithm without changing its structure. This pattern is suitable for projects where there is a need to define a common algorithm that can be customized or specialized for different contexts or scenarios.
One example of a project where the Template method pattern is useful is in the development of a data processing pipeline that involves multiple steps, such as data ingestion, cleaning, transformation, and analysis. By using the Template method pattern, a base class can be created that defines the common algorithm for the data processing pipeline, while allowing subclasses to override or extend specific steps of the algorithm for different data sources or use cases. This can help to improve the efficiency and flexibility of the data processing pipeline and make it easier to scale and maintain over time.
Another example of a project where the Template method pattern is useful is in the development of a game engine that involves multiple stages, such as initialization, rendering, input handling, and audio processing. By using the Template method pattern, a base class can be created that defines the common algorithm for the game engine, while allowing subclasses to override or extend specific steps of the algorithm for different game modes or platforms. This can help to improve the modularity and portability of the game engine and make it easier to customize and optimize for different use cases.
In general, the Template method pattern should be used in projects where there is a need to define a common algorithm that can be customized or specialized for different contexts or scenarios. It can help to improve the flexibility and maintainability of the code, by reducing the duplication of code and providing a clear and consistent structure for the algorithm.
Let's build a coffee maker that takes advantage of the Template Method design.
class CoffeeMaker {
makeCoffee() {
this.boilWater();
this.brew();
this.pourIntoCup();
if (this.addCondiments) {
this.addCondiments();
}
}
boilWater() {
console.log('Boiling water');
}
pourIntoCup() {
console.log('Pouring into cup');
}
}
class EspressoMaker extends CoffeeMaker {
brew() {
console.log('Brewing espresso');
}
addCondiments() {
console.log('Adding sugar');
}
}
class LatteMaker extends CoffeeMaker {
brew() {
console.log('Brewing espresso');
console.log('Steaming milk');
}
addCondiments() {
console.log('Adding vanilla syrup');
}
}
// usage example
const espressoMaker = new EspressoMaker();
espressoMaker.makeCoffee();
const latteMaker = new LatteMaker();
latteMaker.makeCoffee();
In this example, we define a CoffeeMaker
class that defines a common algorithm for making coffee. The CoffeeMaker
class has three steps: boiling water, brewing coffee, and pouring coffee into a cup. The CoffeeMaker
class also defines a hook method addCondiments
that subclasses can override to customize the final step of adding condiments.
We then define two subclasses of CoffeeMaker
: EspressoMaker
and LatteMaker
. The EspressoMaker
subclass overrides the brew
method to brew espresso and overrides the addCondiments
method to add sugar. The LatteMaker
subclass overrides the brew
method to brew espresso and steam milk and overrides the addCondiments
method to add vanilla syrup.
In the usage example, we create instances of EspressoMaker
and LatteMaker
, and call their makeCoffee
methods. The makeCoffee
method follows the common algorithm defined in the CoffeeMaker
class, but specific steps are customized by the respective subclasses.
This example demonstrates how the Template pattern can be used to define a common algorithm for making coffee while allowing subclasses to customize specific steps as needed.
Subscribe to my newsletter
Read articles from Clint directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Clint
Clint
Writer, software engineer, content creator, and all-around awesome guy.