[JavaScript] Date Object Methods & Usage in Real Life - Explained with Examples.
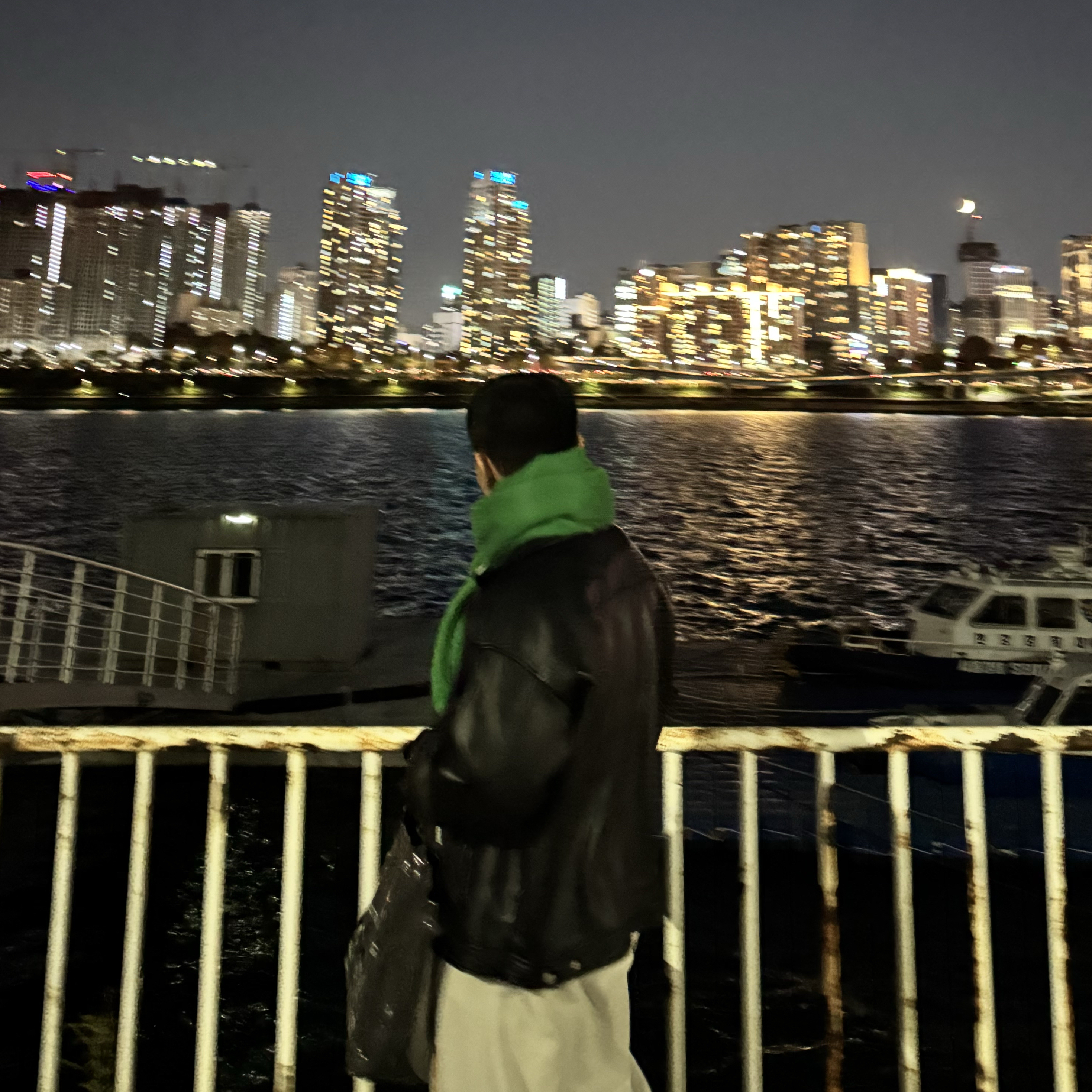
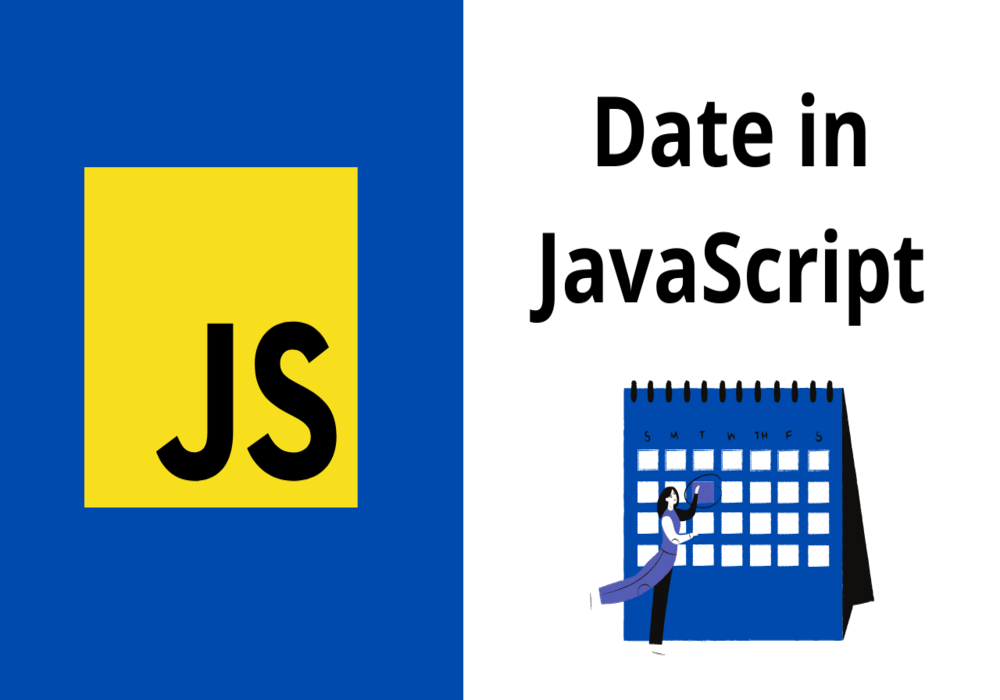
Introduction
Date Object in JavaScript is useful when you want to display a date and time on your web page.
If your website requires users to select the date, a method that will be introduced in this article will help you to improve the UI of your website.
Basic Methods That Will be Covered
getFullYear().
getMonth().
getDate().
getDay().
getHours().
getMinutes().
getSeconds().
getMilliseconds
let curDate = new Date(); // creates a date object.
// let's say current date is 2022-04-29 Monday 8h, 30min, 00sec, 00millisec
console.log(curDate.getFullYear()); // 2022
console.log(curDate.getMonth()); // 3
console.log(curDate.getDate()); // 29
console.log(curDate.getDay()); // 1
console.log(curDate.getHours()); // 8
console.log(curDate.getMinutes()); // 30
console.log(curDate.getSeconds()); // 0
console.log(curDate.getMilliseconds()); // 0
Please take note that 0 refers to January, 1 refers to February and so on. That's why curDate.getMonth() is 3 when it is April.
Also take note that getDate(), 0 refers to Sunday, 1 refers to Monday and so on. Therefore, 1 is printed.
Using new Date() to Assign Values
You can add the date when creating the date object.
let d = new Date(2022, 3, 29, 19, 34, 20, 0);
Method to Compute the Date Without Getting Affected by the Leap Year
We can use milliseconds to calculate the date after or before a certain amount of days.
JavaScript Code:
function getIntervalDateFormat(day, format) {
let curDate = new Date();
let dayMilliseconds = 24 * 60 * 60 * 1000;
let curMilliseconds = curDate.getTime();
let intervalDate = curMilliseconds + day * dayMilliseconds;
let d = new Date(intervalDate);
let year = d.getFullYear();
let month = (d.getMonth() + 1).toString().padStart(2, "0");
let date = d.getDate().toString().padStart(2, "0");
return
format.replace("YYYY", year)
.replace("MM", month)
.replace("DD", day);
}
// calling the function
let before7Days = getIntervalDateFormat(-7, "MM-DD-YYYY");
document.getElementById("startDate").value = before7Days;
// you can set an endDate as well.
HTML Code:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Document</title>
</head>
<body>
<input type="date" name="" id="startDate" /> ~
<input type="date" name="" id="endDate" />
<button>button</button>
</body>
</html>
Subscribe to my newsletter
Read articles from Lim Woojae directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
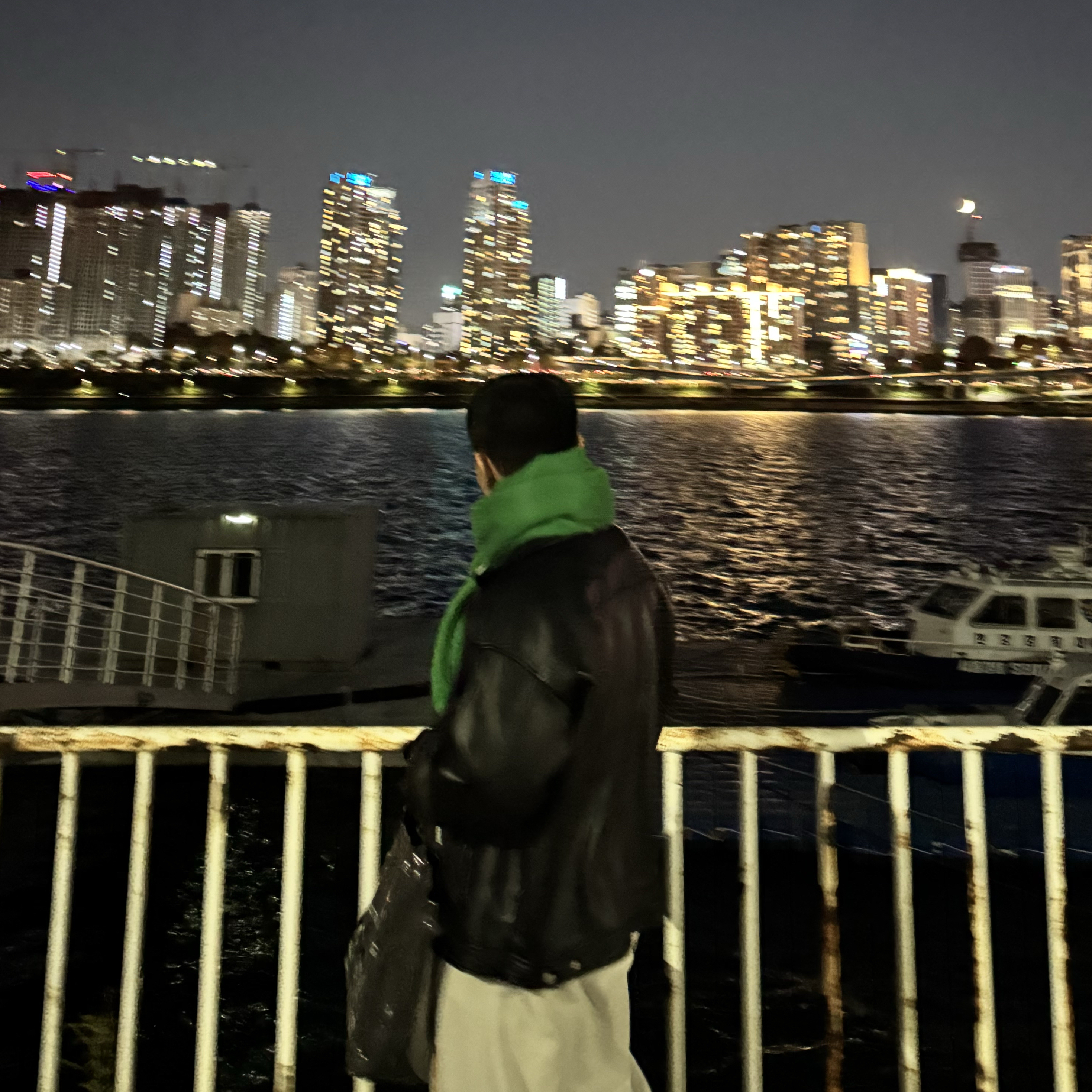
Lim Woojae
Lim Woojae
Computer Science Enthusiast with a Drive for Excellence | Data Science | Web Development | Passionate About Tech & Innovation