JavaScript for ReactJS: How Much Do You Need to Learn?
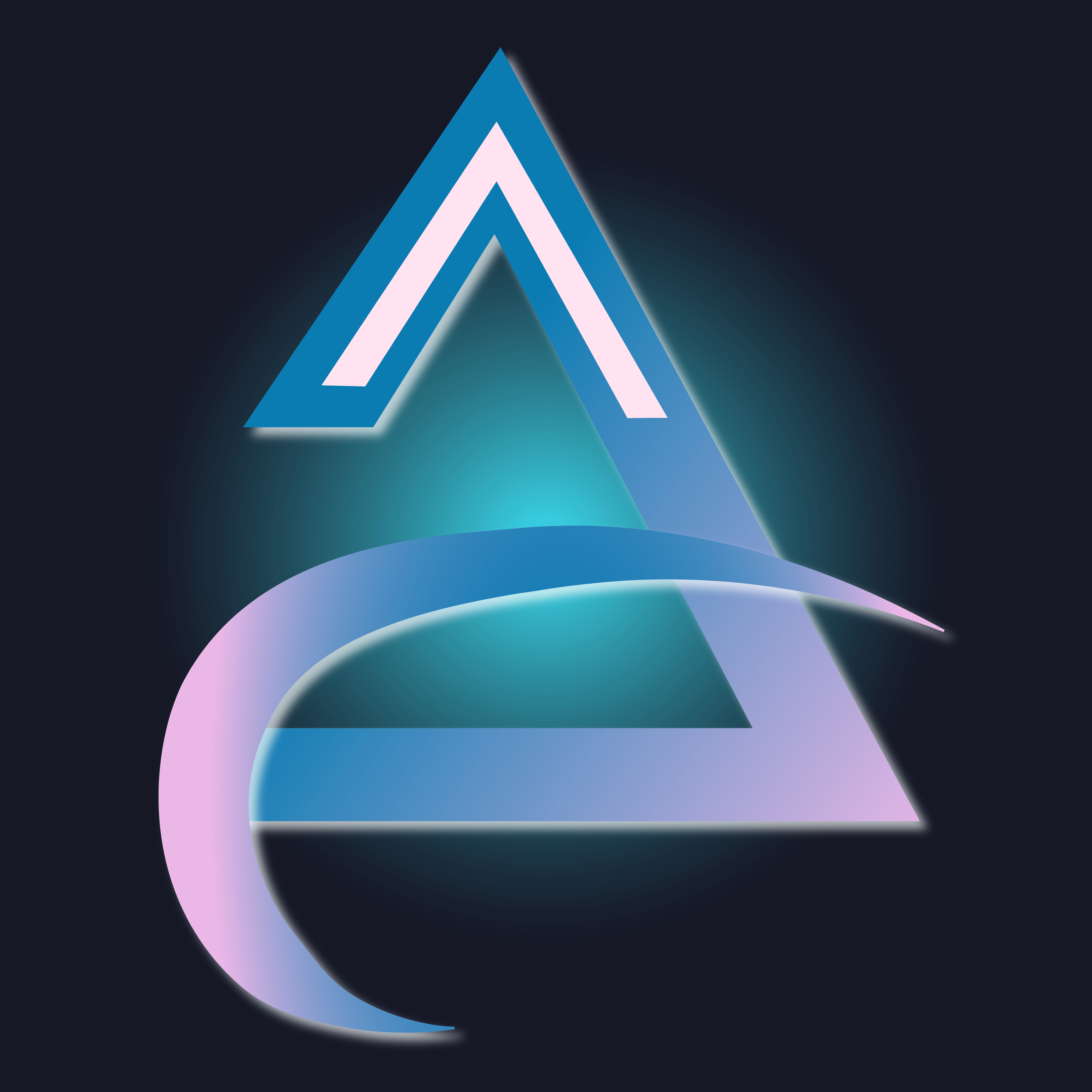
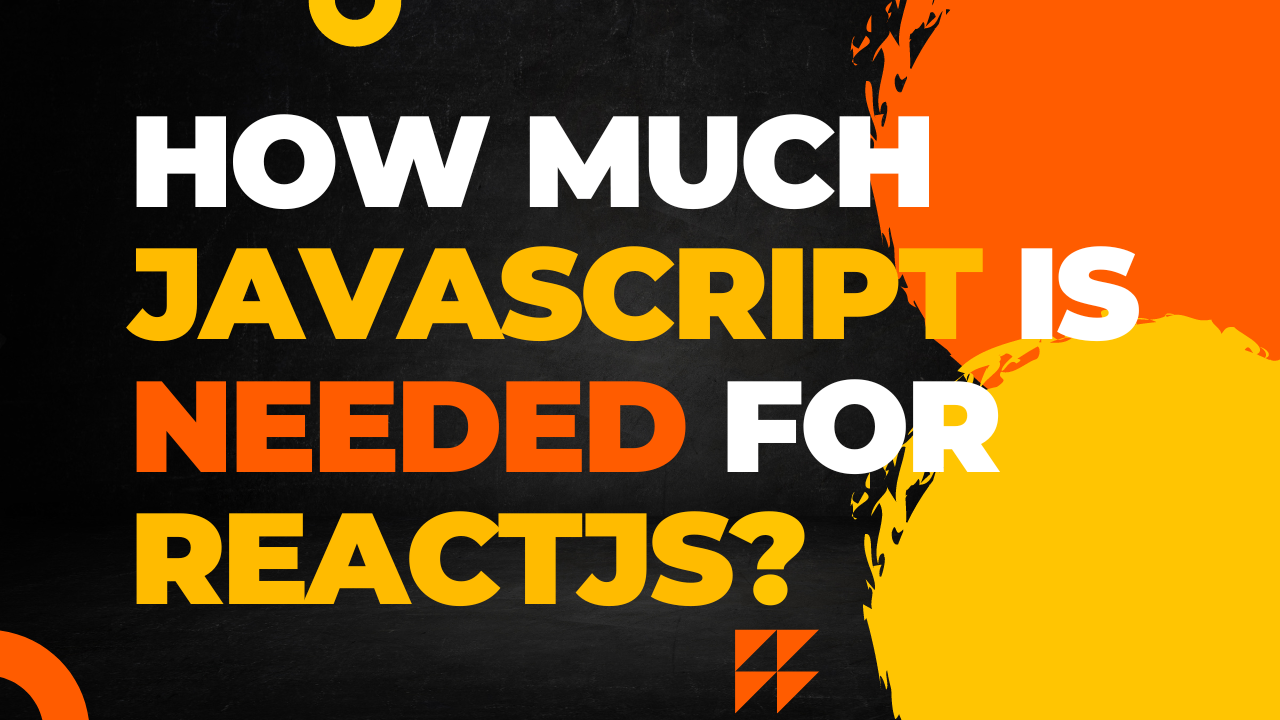
In today's blog post, we'll be discussing a common question that many aspiring React developers ask: "How much JavaScript do you need to learn for React?"
React is a popular JavaScript library used for building user interfaces. While React is written in JavaScript, it's important to note that not all JavaScript concepts are essential for working with React. However, having a solid understanding of JavaScript will undoubtedly make your journey with React much smoother.
In this blog post, we'll explore the JavaScript concepts that are essential for React development, such as Object, Functions, and Array Functions etc. We'll also touch on some advanced JavaScript concepts, such as Asynchronous Functions, Await, and Fetch API.
So, whether you're a beginner looking to start your journey with React or an experienced developer looking to brush up on your JavaScript skills, this blog post will provide you with the necessary knowledge to succeed. So, let's dive in and explore how much JavaScript you need to learn for React!
The JavaScript concepts which are important from the ReactJS point of view are:-
Ternary Operators
Arrow Functions
Map Function
Filter Function
Objects
Promise
Fetch API
Async - Await
TERNARY OPERATOR
The ternary operator, also known as the conditional operator, is a concise way to write an if...else
statement in JavaScript. It takes three operands: a condition, a value to return if the condition is true, and a value to return if the condition is false.
There are three main operators used here:-
AND Operator
{ && }
OR Operator
{ || }
IF-ELSE Operator
{ condition ? statement1 : statement2 }
If you have to execute an operation where you have to check only one condition and the condition is resulting in a true condition the AND Operator is used.
For example:-
If we have an "age" variable whose value is "10" and we have a "name" variable whose value is "Coding Adda", now we have to write a logic where it says that if the age is greater or equal to "10" then the name will be "CodingAdda17", the traditional way of writing is in if-else statement.
let age = 10;
let name = 'Coding Adda';
if ( age >= 10 ) {
name = 'CodingAdda17';
}
As you can see that it is taking three lines of code whereas it can be done in one line of code using AND Operator
.
let age = 10;
let name = age >= 10 && 'CodingAdda17';
So as you can see we wrote the logic in one line.
The working of AND Operator
is like this if the given condition is true
then and only then the statement will be executed, in our case as we are assigning a value to a variable so for the true condition
the value will be assigned or else it will return false
as the condition is false
.
If you have to execute an operation where you have to check only one condition and the condition is resulting in a false condition the OR Operator is used.
For example:-
Same as before but now we have to write a logic where it says that the age is less than 10 i.e. the condition will be false, and if the condition is false then the value of "name"
variable will be 'Coding-Adda'
, the traditional way of writing is an if-else statement.
let age = 9;
let name = 'Coding Adda';
if ( age >= 10 ) {
name = 'Coding-Adda';
}
As you can see that it is taking three lines of code whereas it can be done in one line of code using OR Operator
.
let age = 9;
let name = age >= 10 || 'Coding-Adda';
So as you can see we wrote the logic in one line.
The working of OR Operator
is like if the given condition is false
then and only then the statement will be executed, in our case as we are assigning a value to a variable so for the false condition
the value will be assigned or else it will return true
as the condition is true
.
If you have to execute and traditional If-Else Statement in Ternary Operator or Conditional Operator then we can use if-else operator.
For Example:-
Taking the same example as above, but we have to write a logic where it says that if the condition is True then then the value of the "name"
variable should be equal to "CodingAdda"
and if the condition is false then the value of the "name"
variable should be equal to "Coding_Adda"
, the traditional way of writing is in if-else statement.
let age = 10;
let name = 'Coding Adda';
if ( age >= 10 ) {
name = 'CodingAdda';
} else {
name = "Coding_Adda"
}
As you can see that it is taking five lines of code whereas it can be done in one line of code using IF-ELSE Operator
.
let age = 10;
let name = age >= 10 ? 'CodingAdda' : "Coding_Adda";
So as you can see we wrote the logic in one line.
The working of IF-ELSE Operator
is like if the given condition is true
then the first section
of the statement will be executed and if the condition is false
then the second section
of the statement will be executed, in our case we are assigning the value to the variable so if the condition is true then the first value will be assigned and if the condition is false the second value will be assigned.
ARROW FUNCTION
An arrow function in JavaScript is a concise way to define a function using a new syntax introduced in ES6 (ECMAScript 2015). It's also known as a "fat arrow" function, due to the use of the =>
arrow symbol.
Traditional defination :
function addNum(a, b) {
return a + b;
}
Arrow Function Defination :
const addNum = (a, b) => {
return a + b;
}
In this example, addNum
is an arrow function that takes two parameters a
and b
and returns their sum. The function is assigned to a const
variable, but it could also be assigned to a let
or var
variable also.
Exporting the traditional function :
export default function addNum(a, b) {
return a + b;
}
Exporting the arrow function :
export const addNum = (a, b) => {
return a + b;
}
MAP FUNCTION
The map()
function is an array method that allows you to apply a function to each element in an array and return a new array with the transformed elements. The map()
function takes a single argument, which is the function that should be applied to each element.
The map()
function is a powerful tool for working with arrays in JavaScript, as it allows you to apply a function to each element in a concise and readable way. It can be used for a wide variety of tasks, such as filtering, sorting, and formatting data.
For Example :
const numbers = [1, 2, 3, 4, 5];
const printNumbers = numbers.map((number) => {
return number;
});
console.log(printNumbers); // Output: [2, 4, 6, 8, 10]
In this example, the map()
function is used for printing the elements of the array of numbers. The function takes a single parameter number
, which represents each element of the array in turn. The function returns the transformed value, which is then collected into a new array printNumbers
.
FILTER FUNCTION
The filter()
function is an array method that allows you to create a new array with all the elements that pass a certain condition. The filter()
function takes a single argument, which is a function that tests each element in the array.
The filter()
function is a useful tool for working with arrays in JavaScript, as it allows you to selectively extract elements that meet certain criteria. It can be used for a wide variety of tasks, such as searching, sorting, and formatting data.
For Example :
const numbers = [1, 2, 3, 4, 5];
const evenNumbers = numbers.filter((number) => {
return number % 2 === 0;
});
console.log(evenNumbers); // Output: [2, 4]
In this example, the filter()
function is used to create a new array evenNumbers
that only include the even numbers from the original array numbers
. The function takes a single parameter number
, which represents each element of the array in turn. The function returns a boolean value indicating whether the element should be included in the new array.
OBJECTS
An object is a collection of related data and/or functionality that can be accessed and manipulated as a single unit. Objects in JavaScript are similar to objects in real life, in that they have properties and methods that define their characteristics and behavior.
Objects in JavaScript can be very powerful and flexible and are used extensively in many JavaScript programs. They can be used to represent complex data structures, create reusable code modules, and provide encapsulation and abstraction for code organization and security.
For Example :
const person = {
name : "Harikrishnan",
age : 21,
isMarried : False,
};
We can access the "key-value" of the above "Object" in many ways. The default way is to access using the Dot Operator.
const person = {
name : "Rahul",
age : 21,
isMarried : False,
};
const name = person.name;
const age = person.age;
const isMarried = person.isMarried;
As we can see in the above code block we are accessing the value of the three keys present in the "Object" which are name
, age
and isMarried
. The more efficient way of accessing the key-value
is by "Destructuring" the object. By "Destructing" instead of creating different variables for different "keys" we can just reduce the code to one line and have the same result.
const person = {
name : "Rahul",
age : 21,
isMarried : False,
};
// Destructuring the above Object
const { name, age, isMarried } = person;
As we can see we just reduced the lines of code using "Destructuring". The concept of Destructuring is important from the ReactJS point of view.
If we have declared a variable at the very top of the code and we want to use that value in the object then there is no need to create an object and manually put the value of that particular variable in the object. Instead what we can do is to put the "Variable Name" in the place of that key's value.
const name = "Rahul";
const age = 21;
const person = {
name : name,
age : age,
isMarried : False,
}
There is also a way of putting the same value instead of putting the variable name in the place of that key's value we can just write the name of that variable.
const name = "Rahul";
const age = 21;
const person = {
name,
age,
isMarried : False,
}
This way we can use a variable as the value of any particular object.
If we have an object in our code and want to create another object where we want to have the same keys and values as the previous object then we can approach it with the traditional method by creating a new object and manually adding the keys and values it the newly created object.
const person1 = {
name : "Rahul",
age : 21,
isMarried : False,
}
const person2 = {
name : "Rohan",
age : 21,
isMarried : False,
}
Another way is to use the Spread Operator and create a new object, using Spread Operator we can create a new object and specify which key-value to modify or which key-value to add. Spread Operator is "...
" .
const person1 = {
name : "Rahul",
age : 21,
isMarried : False,
}
const person2 = { ...person1, name : "Rohan"};
As we can see using Spread Operator add the keys and values of "person1" to "person2" and changed the name from "Rahul" to "Rohan".
Spread Operator can be used in arrays also.
For Example :
const names = ['Rahul', 'Rohan', 'Abdul'];
const new_names = [ ...names, 'Hari', 'Adi'];
PROMISE
A promise is an object that represents the eventual completion (or failure) of an asynchronous operation and its resulting value. A promise can be in one of three states: pending
, fulfilled
, or rejected
.
Promises are used extensively in modern JavaScript programming to handle asynchronous operations such as network requests, file I/O, and database queries. Promises provide a way to chain asynchronous operations together and handle success or error conditions in a more organized and consistent way.
function fetchData() {
return new Promise((resolve, reject) => {
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => resolve(data))
.catch(error => reject(error));
});
}
fetchData()
.then(data => console.log(data))
.catch(error => console.error(error));
As you can see the fetchData()
function returns a new promise that wraps a network request made using the fetch()
method. The resolve()
function is called with the resulting data when the request is successful, and the reject()
function is called with an error when the request fails.
The returned promise can be clubbed together with additional then()
and catch()
methods to handle success or error conditions further down the chain.
FETCH API
The Fetch API is a modern interface for making network requests, allowing you to fetch resources from a server or API endpoint using a simple and flexible syntax.
The Fetch API provides a global fetch()
method that returns a Promise object, which can be used to handle the response from a network request. The fetch()
method takes a single argument, which is the URL of the resource you want to fetch.
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
As you can see the fetch()
method is used to make a GET request to the https://api.example.com/data
endpoint. The then()
method is used to parse the response as JSON, and the resulting data is logged to the console. The catch()
method is used to handle any errors that might occur during the request.
ASYNC - AWAIT
async/await
is a pair of keywords used to handle asynchronous operations in a more synchronous-like fashion.
async/await
is built on top of promises, which are a way to handle asynchronous operations in JavaScript. With promises, you can chain together operations and handle success or error conditions using the then()
and catch()
methods.
async/await
simplifies the syntax for working with promises, making the code more readable and easier to follow. With async/await
, you can write asynchronous code that looks and behaves like synchronous code.
For Example :
async function fetchData() {
try {
const response = await fetch('https://api.example.com/data');
const data = await response.json();
console.log(data);
} catch (error) {
console.error(error);
}
}
As you can see the fetchData()
function is marked as async
, indicating that it will contain asynchronous operations. The await
keyword is used to wait for the completion of each asynchronous operation, in this case, a network request is made using the fetch()
method. The try/catch
block is used to handle any errors that might occur during the execution of the asynchronous code.
To get a more clear idea of the implementation, you can check out our video on "JavaScript for ReactJS: How Much Do You Need to Learn?" which is live on Youtube.
In conclusion, while it's true that learning JavaScript is crucial for becoming a proficient React developer, you don't need to be an expert in every aspect of the language. Understanding the fundamentals such as variables, functions, arrays, and objects, as well as the DOM and AJAX, is a great place to start. As you delve deeper into React, you'll naturally pick up more advanced JavaScript concepts and techniques along the way. So, don't feel overwhelmed by the vastness of JavaScript, just focus on what you need to know and keep learning as you go. Happy coding!
Adios Amigos ๐๐
Subscribe to my newsletter
Read articles from Coding Adda directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
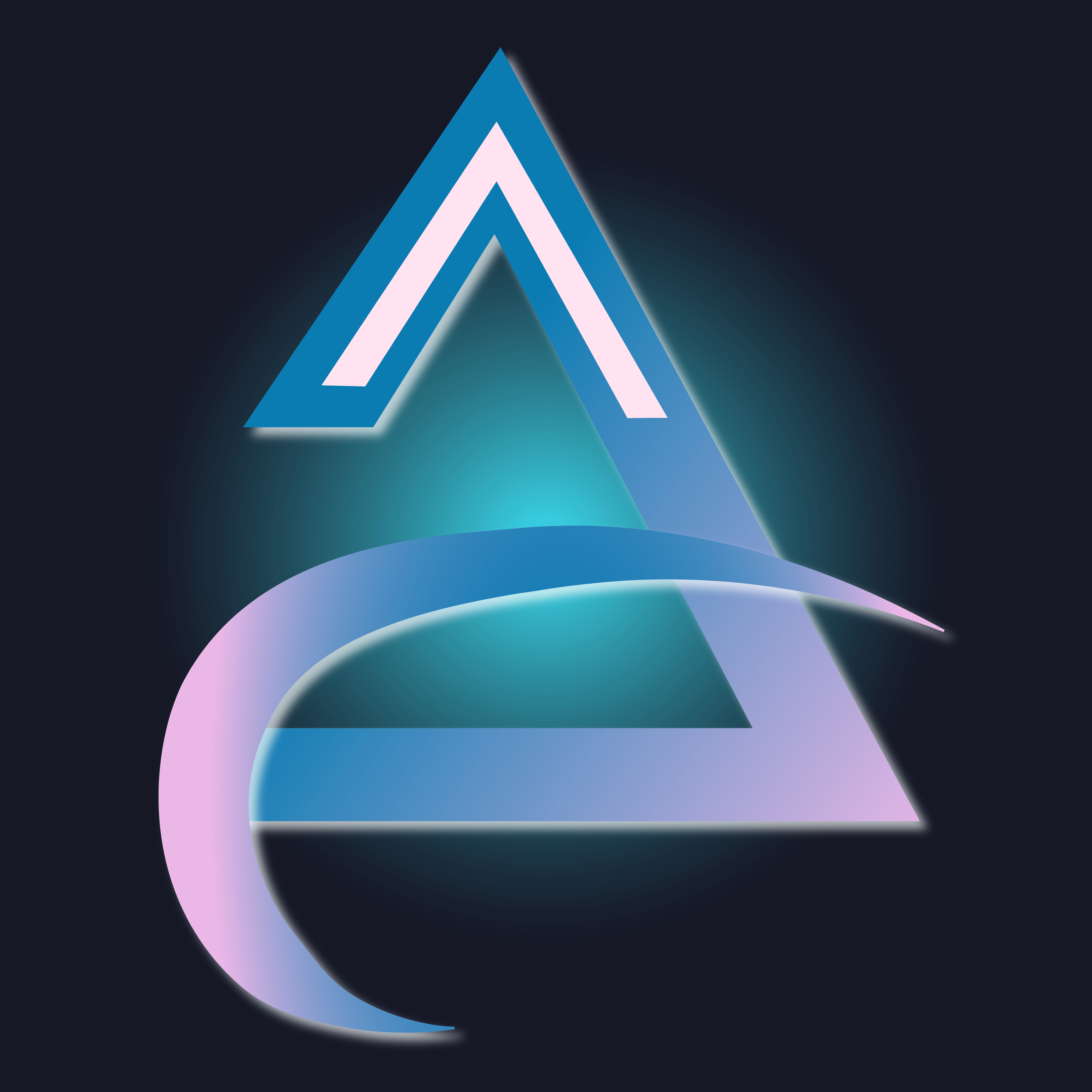
Coding Adda
Coding Adda
Hello Everyone, Presenting you CODING ADDA, We at Coding Adda will help you solve problems in Coding. We at Coding Adda try to clarify the concepts of Coding through short videos and real-life examples. Do support us in our journey. Like, Share and Subscribe to the Channel.