[JavaScript] Simple Rock Paper Scissors Game - Beginner Friendly
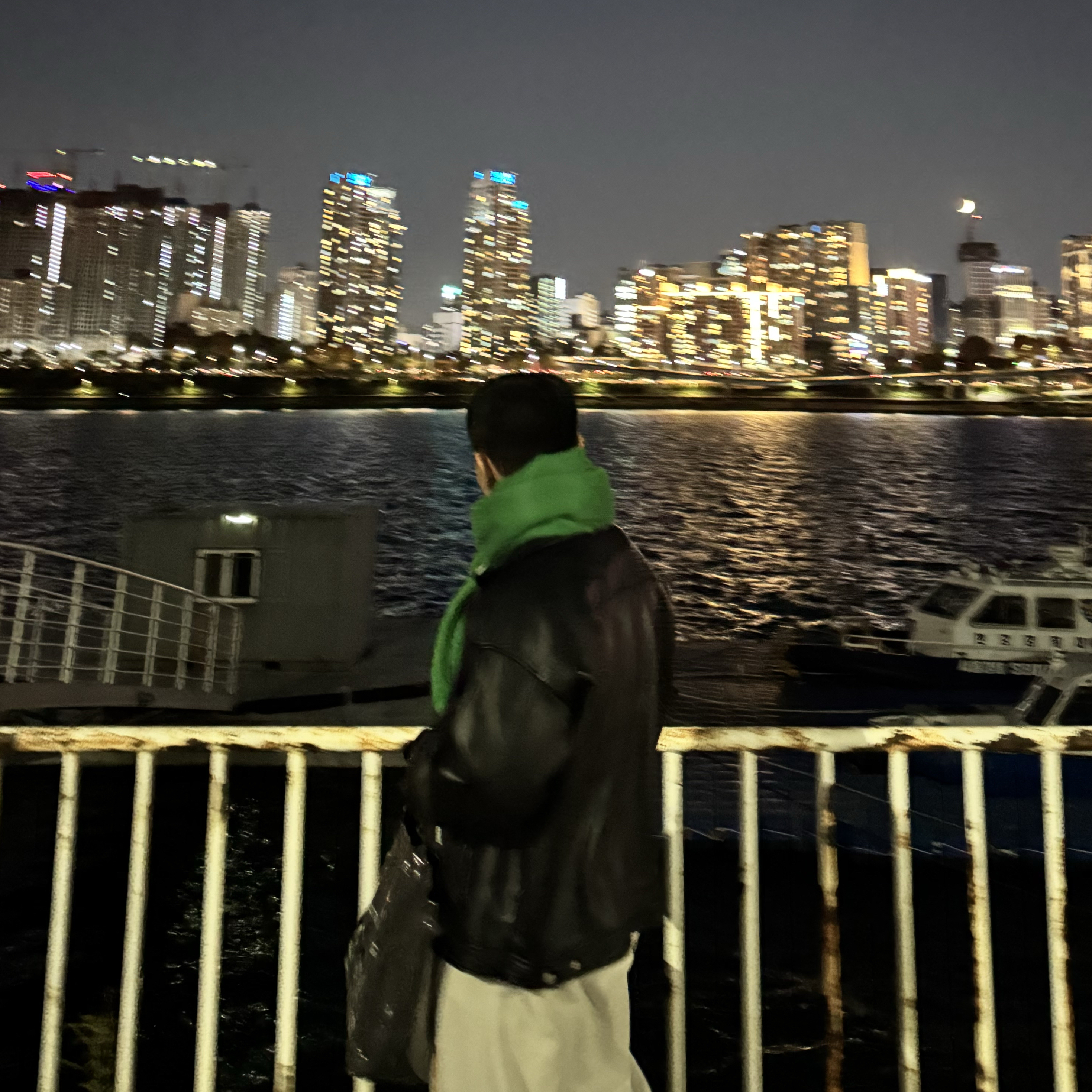
Table of contents
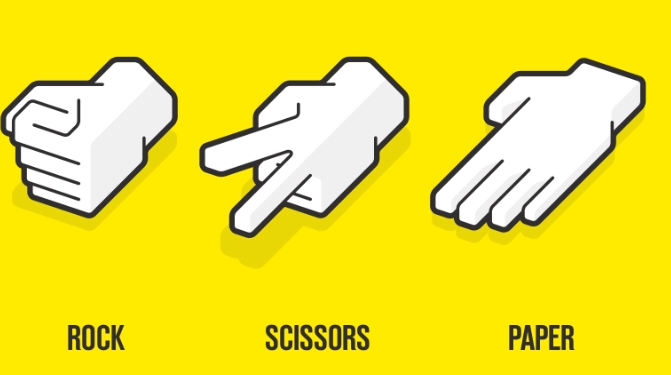
Introduction
To build a rock paper scissors game, you need to use Math.random() method to randomly generate rock, scissors and paper.
In this mini-build, we will use HTML to allow users to choose their option and print out the result if they won against the computer.
getRandomInt() Function
function getRandomInt(min, max) {
return Math.floor(Math.random() * (max - min + 1) - min);
}
This function generates a random number between min and max values.
For example, getRandomInt(1, 10) will give a random number between 1 and 10.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Document</title>
<button onclick="rspGame('rock');">rock</button>
<button onclick="rspGame('paper');">paper</button>
<button onclick="rspGame('scissors');">scissors</button>
</head>
<body>
<script>
function rspGame(userInput) {
computerRsp = ["rock", "paper", "scissors"];
computerOutput = computerRsp[getRandomInt(0, 2)]
userWinValue = {
rock: "scissors",
paper: "rock",
scissors: "paper"
};
console.log("User Side:", userInput);
console.log("Computer Side:", computerOuput);
return userWinValue[userInput] === computerOutput
? "User Wins!"
: userInput = comptuerOutput
? "Draw!"
: "Computer Wins!";
}
function getRandomInt(min, max) {
return Math.floor(Math.random() * (max - min + 1) - min);
}
</script>
</body>
</html>
The portion below lets the user makes their choice.
If a click on the button is detected, the computer generates its output (one of rock, paper, scissors), then prints out the results.
<button onclick="rspGame('rock');">rock</button>
<button onclick="rspGame('paper');">paper</button>
<button onclick="rspGame('scissors');">scissors</button>
Subscribe to my newsletter
Read articles from Lim Woojae directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
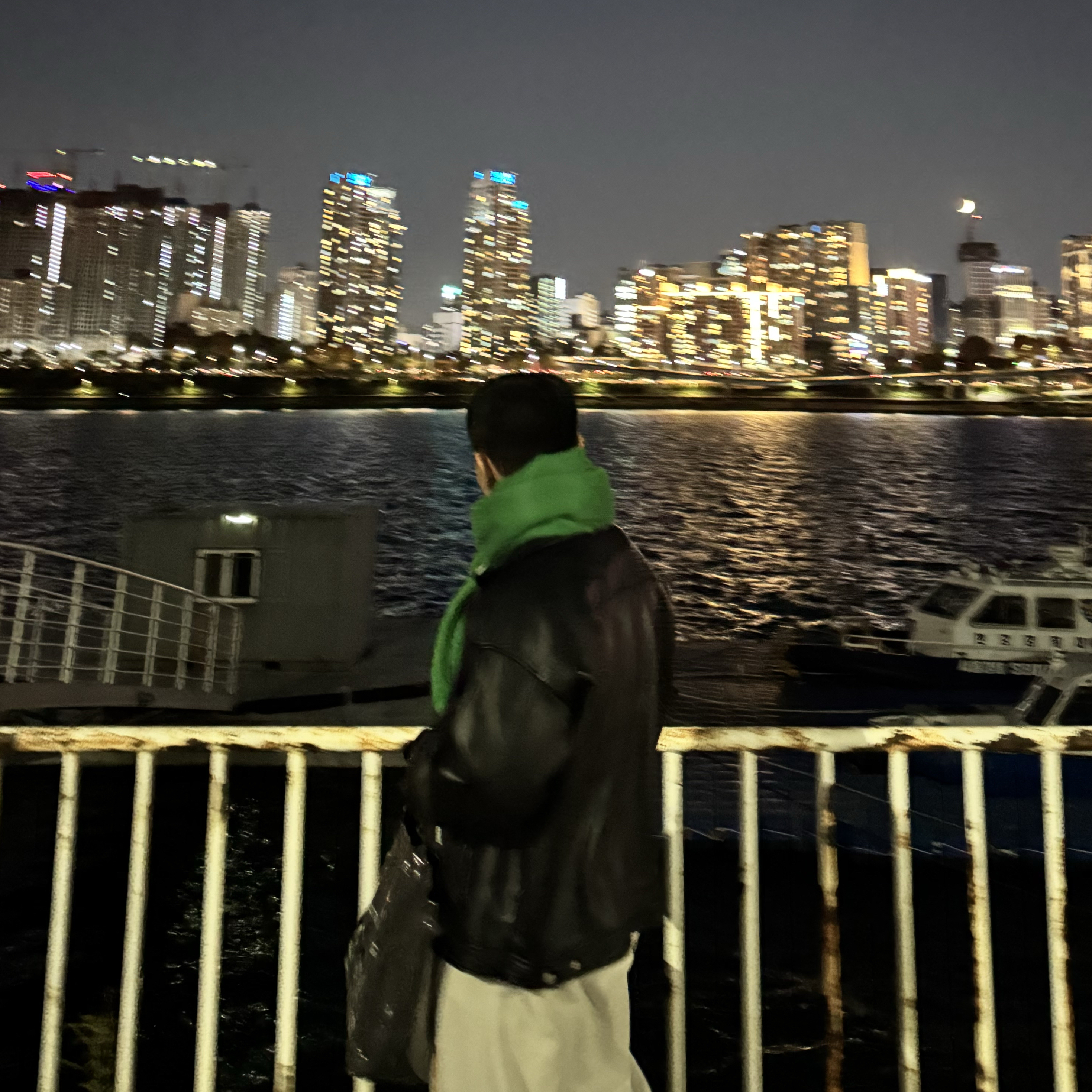
Lim Woojae
Lim Woojae
Computer Science Enthusiast with a Drive for Excellence | Data Science | Web Development | Passionate About Tech & Innovation