FromDIP in wxWidgets
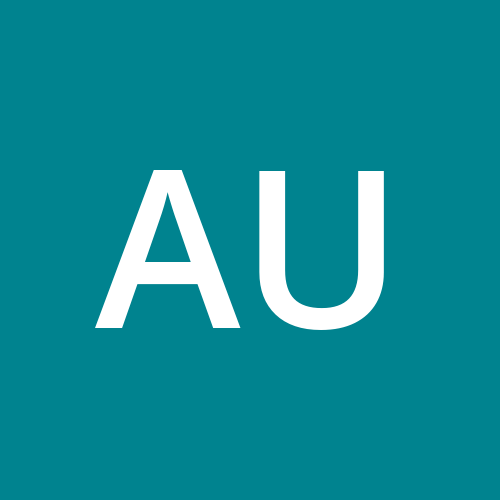
In wxWidgets, the FromDIP() function is used to convert device-independent pixels (DIPs) to device pixels.
Device-independent pixels are a unit of measurement used in modern UI design to ensure that user interfaces look consistent across different display densities and resolutions. They are defined in terms of density-independent pixels (DPs), which are then scaled based on the device's pixel density to obtain the final device-specific pixel (or point) size.
The FromDIP() function takes a DIP value as its argument and returns the corresponding value in device pixels. This function is particularly useful for calculating the correct size and position of UI elements such as buttons, labels, and text boxes.
Here's an example of how to use FromDIP() to set the size of a button in a wxWidgets application:
#include <wx/wx.h>
class MyFrame : public wxFrame
{
public:
MyFrame(const wxString& title)
: wxFrame(NULL, wxID_ANY, title)
{
// Create a button with a size of 100 DIPs by 50 DIPs
wxButton* button = new wxButton(this, wxID_ANY, "Click me!", wxDefaultPosition, wxSize( wxWindow::FromDIP(100), wxWindow::FromDIP(50) )
);
}
};
class MyApp : public wxApp
{
public:
virtual bool OnInit();
};
bool MyApp::OnInit()
{
// Create and show the main frame
MyFrame* frame = new MyFrame("My Application");
frame->Show();
return true;
}
IMPLEMENT_APP(MyApp)
In this example, we define a custom frame class MyFrame
that inherits from wxFrame
. In the constructor of this class, we create a new wxButton
object with a size of 100 DIPs by 50 DIPs.
To convert these DIP values to device pixels, we use the static FromDIP()
function of the wxWindow
class. We pass the DIP values as arguments to this function and wrap them in a wxSize
object, which is then used as the button's size.
By using the FromDIP() function in this way, we can ensure that our UI elements have a consistent size and appearance across different devices and display densities.
Subscribe to my newsletter
Read articles from Arun Udayakumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
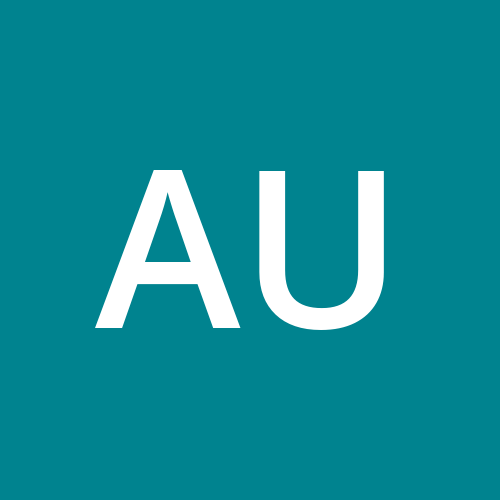