Arrays with JavaScript - 2
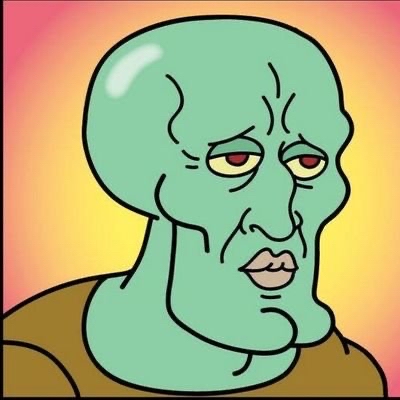

Hello there 👋. In the previous article on Arrays with JavaScript, we learnt why we use arrays, how to declare and initialize arrays, how to access elements in an array, how to add elements to an array and how to remove elements from an array. In this blog post, we will dive deeper into the world of arrays, learning about two-dimensional and multi-dimensional arrays, and JavaScript array methods among other things.
Two-Dimensional and Multi-Dimensional Arrays
Arrays are normally single-dimensional. However, some computing processes would be more efficient when the underlying data structure used is a two-dimensional or a multi-dimensional array. To implement a two-dimensional array, you just have to create an array whose primary elements are also arrays. Multi-dimensional array data structures are likened to spreadsheets, where each array in the main array is a row, and the indexes of inner arrays are columns. The code below illustrates the creation of a multi-dimensional array using a for loop.
let myArray = []
for(let i = 0; i < 5; i++){
myArray[i] = []
}
console.log(myArray); // [[],[],[],[],[]]
The code above created a two-dimensional array with 5 rows and 1 column.
We can also create a two-dimensional array, and initialize the values immediately as shown in the code below.
let myArray = [[11,23],[12,41],[45,24],[75,37],[35,87]]
Accessing Elements in a Multi-dimensional Array
Accessing elements in a multi-dimensional array is very similar to accessing elements in a regular array. The index of the array containing the element is first passed into an empty angle bracket, and then the index of the element in the array containing it is passed into another empty bracket. The code below illustrates how to access 37
in myArray
console.log(myArray[3][1]); // 37
The first angle bracket contains the index of the array containing 37
, while the second angle bracket contains the index of 37
in its array.
Working with Two-Dimensional arrays.
Two basic approaches are used to process elements of a multi-dimensional array. The first approach accesses elements by rows, while the second approach accesses elements by columns. For both approaches, a set of nested for-loops are used to iterate through the elements of the array. For this exercise, we would initialize a two-dimensional array as shown below,
let studentScores = [[11,23,56],[12,41,67],[45,24,59],[75,37,45],[35,87,50]]
Each row in the studentScores
represents a student’s scores, while each column represents the scores of all students in a particular test. To compute each student’s average score, a columnar iteration of the array can be done to add each student’s scores together and then divide the total by the total number of scores on that row.
To implement a columnar iteration, the outer loop iterates through the row, while the inner loop iterates through columns. This is illustrated in the code below.
for(let i = 0; i < studentScores.length; i++){
let studentScore = 0;
for(let j = 0; j < studentScores[i].length; j++){
studentScore += studentScores[i][j];
}
let studentAverageScore = (studentScore / studentScores[i].length).toFixed(2);
console.log(`Student ${i+1} average score is ${studentAverageScore}`);
}
/*
Student 1 average score is 30.00
Student 2 average score is 40.00
Student 3 average score is 42.67
Student 4 average score is 52.33
Student 5 average score is 57.33
*/
To perform a row-wise iteration, we just have to switch the for loops, such that the outer loop iterates through the column, while the inner loop iterates through the rows. Suppose we want to compute the average score of all the students on each test, this can be easily achieved by carrying out a row-wise iteration on the array to add the scores of each student on the test and dividing it by the number of students who took the test as shown in the code below.
for(let i = 0; i < studentScores[i].length; i++){
let totalTestScore = 0;
for(let j = 0; j < studentScores.length; j++){
totalTestScore += studentScores[j][i];
}
let averageScore = (totalTestScore / studentScores.length).toFixed(2);
console.log(`The average score for Test ${i + 1} is ${averageScore}`)
}
/*
The average score for Test 1 is 35.60
The average score for Test 2 is 42.40
The average score for Test 3 is 55.40
*/
Jagged Arrays
Jagged arrays are special kinds of multi-dimensional arrays, whose array elements don’t have equal lengths. Suppose that in the studentScores
array created above, a particular student did not sit for a test. This would reduce the length of the array representing this student’s scores by 1. A multidimensional array whose sub-arrays do not have uniform length is called a jagged array. The code below is used to illustrate a jagged array.
let studentScores = [[11,56],[12,41,67],[45],[75,37,45],[35,87,50]]
Notice that the arrays at indexes 0
and 2
have a length of 2 and 1 respectively. Working with Jagged arrays is the same as working with regular multi-dimensional arrays, however, depending on the kind of algorithm being implemented, you may need to take the length of the sub-arrays into account.
Array Iterator Methods
Iterator methods are used to apply a function to every element of an array either to return a value, a set of values or a new array after the function’s complete execution. For the following examples, we will initialize an array x
as shown below.
let x = [1,2,3,4,5,6]
The forEach()
method
The forEach()
method can be likened to a simple for loop. It is used to iterate through the elements of an array and perform an operation for each element. The example below demonstrates how to log the elements of array x
above using the forEach()
method.
x.forEach(e => {
console.log(e);
}
/*
1
2
3
4
5
6
*/
The argument e
passed to the function above represents the element on the current iteration of the function.
The some()
method
The some()
method takes a boolean function/expression as an argument and returns true
if at least one of the elements in the array satisfies the truthy condition of the function/expression but returns false if none of the elements in the array satisfies this condition. The example below demonstrates how to make use of the some()
method to determine if array x
contains any even number.
const isEven = (num) =>{
return (num % 2) === 0
}
console.log(x.some(isEven)); // true
The every() method
The every()
is quite similar to the some()
method. However, this only returns true if all elements of the array satisfy the truthy condition of the expression/function passed to the every()
method. The example below demonstrates how to make use of the every()
method to check if all elements of the x
array are even.
const isEven = (num) =>{
return (num % 2) === 0
}
console.log(x.every(isEven)); // false
The reduce() method
The reduce()
method is used to reduce an array to a single value. It applies a function to each element of the array from left to right while accumulating its return values as it traverses the array. The example below demonstrates how to use the reduce()
method to calculate the sum of all elements in the x
array.
console.log(x.reduce((a,b) => a + b)); // 21
It is important to note that a concatenation would be done if the elements of the x
array were strings rather than integers.
The map() method
The map()
method is very similar to the forEach()
method, as they both iterate through the array and carry out a function. However, the map()
method returns an array containing the results of its operation. The following example demonstrates how to use the map()
method to find the square of all elements of the x
array.
const getSquare = x => {
return x * x;
}
console.log(x.map(getSquare)); // [ 1, 4, 9, 16, 25, 36 ]
The filter() method
The filter()
method is very similar to the every()
method. However, the filter()
method does not return true
if all the elements in the array satisfy the truthy condition. It instead returns an array containing all the elements which satisfy the truthy condition of the function passed to it. The example below demonstrates how to use the filter()
method to get all even elements of the x
array.
const isEven = (num) =>{
return (num % 2) === 0
}
console.log(x.filter(isEven)); // [ 2, 4, 6 ]
Other Array Operations
Creating an array from strings
The split()
method on JavaScript strings allows us to create arrays from strings, by breaking up the string at a common delimiter. The created array takes the broken parts of the string as its elements. The code below demonstrates the use of the split()
method to create an array.
const x = "I am a boy."
const x1 = x.split("");
console.log(x1); // ['I', ' ', 'a', 'm',' ', 'a', ' ', 'b', 'o', 'y', '.']
const y= "I am a boy."
const y1 = y.split(" ");
console.log(y1); // [ 'I', 'am', 'a', 'boy.' ]
Notice the x1 = x.split("")
splits up every character in the string as the delimiter provided to the method is an empty string. However, y1 = y.split(" ")
splits up the strings into the individual words of the string because the delimiter given to the method is an empty space. Hence it breaks the string at every occurrence of a space.
Assigning an array to another array
Assigning an array to another array is as simple as initiating the value of a variable to be a variable which holds an array as its value, as shown below.
const array1 = [1,2,3,4,5];
const array2 = array1;
However, the approach used above is called shallow copy, as the value that array2
holds is the memory address of array1
in memory. Hence any changes made to array1
would affect the values gotten from array2
, an alternative to this is called deep copy which can be achieved by implementing a function as shown below.
function deepCopy(array1){
let array2 = [];
for(let i = 0; i < array1.length; i++){
array2[i] = array1[i];
}
return array2
}
Searching for a value
The indexOf()
method of the JavaScript arrays allows us to get the index of the first occurrence of the element passed to it and returns -1 if the element does not exist in the array. For this exercise, we would be making use of array1
created above. The code below demonstrates the use of the indexOf()
method.
console.log(array1.indexOf(2)); // 1
console.log(array1.indexOf(7)); // -1
To search for the last occurrence of an element in an array, the lastIndexOf()
method is used as shown below.
console.log(array1.lastIndexOf(5)); // 4
Note that if there is another occurrence of 5
after the index 4
the index of that occurrence would be returned instead.
Converting arrays to strings
Two array methods in JavaScript can be used to convert arrays to a string representation of the array of which it is called upon.
The first method we would be considering is the toString()
method. The toString()
method takes no argument and returns the elements of the array delimited with a comma as a string. The code below demonstrates the behaviour of the toString()
method.
const dummyArray = ["i", "am", "a", "master", "of", "arrays", "in", "javascript"];
const dummyArrayToString = dummyArray.toString();
console.log(dummyArrayToString); // i,am,a,master,of,arrays,in,javascript
The alternative to the toString()
method is the join()
method, which comes in handy if you wish to separate the elements of the array by a delimiter other than the default comma. The join()
method takes an optional argument which acts as a delimiter to the elements of the array. The code below demonstrates the use of the join()
method.
const dummyArray = ["i", "am", "a", "master", "of", "arrays", "in", "javascript"];
const dummyArrayToString = dummyArray.join();
console.log(dummyArrayToString); // i,am,a,master,of,arrays,in,javascript
const dummyArrayToStringWithSpaces = dummyArray.join(" ");
console.log(dummyArrayToStringWithSpaces); // i am a master of arrays in javascript
Notice that dummyToString
above does not pass any argument to the join()
method hence the default comma delimiter is used in the resulting string. While a space delimiter is passed to the join()
method used in dummyArrayToStringWithSpaces
hence space is used as a delimiter in the resulting string.
Sorting an array
The sort()
method of JavaScript arrays is used to put the elements of JavaScript arrays in order. The code below illustrates the use of the sort()
method.
const dummyArray = ["i", "am", "a", "master", "of", "arrays", "in", "javascript"];
const sortedDummyArray = dummyArray.sort();
console.log(sortedDummyArray); // [ 'a', 'am', 'arrays', 'i', 'in', 'javascript', 'master', 'of' ]
It is important to note that the sort()
method does not work well with numbers because it sorts data lexicographically like the data are strings. The code below demonstrates how the sort()
method works with numbers normally.
const numArray = [50,45,350,2,100];
const sortNumArray = numArray.sort();
console.log(sortNumArray) // [100,2,350,45,50]
The behaviour of the sort()
method can be corrected by passing a function to the sort()
method to specify how the data should be sorted. This is illustrated in the code below.
const numArray = [50,45,350,2,100];
const sortNumArrayInAscendingOrder = numArray.sort((a,b) => a - b);
console.log(sortNumArrayInAscendingOrder) // [2,45,50,100,350]
const sortNumArrayInDescendingOrder = numArray.sort((a,b) => b - a);
console.log(sortNumArrayInDescendingOrder) // [350,100,50,45,2]
Creating New Arrays from Existing Arrays
There are two methods of JavaScript arrays which allows us to form new arrays from already existing arrays. They are the concat()
and the splice()
methods. The concat()
method is used to form a new array by joining two arrays together, while the splice()
method is used to create an array by taking a portion of an already existing array. The code example below is used to illustrate how the concat()
method works.
const firstArray = ["i", "am", "a", "master"]
const secondArray = ["of", "arrays", "in", "javascript"]
const thirdArray = firstArray.concat(secondArray);
console.log(thirdArray); // ["i", "am", "a", "master", "of", "arrays", "in", "javascript"]
The splice()
method takes one compulsory argument which specifies the index at which the splice operation should start and an optional argument which specifies the number of elements to be taken. Note that if the optional argument is omitted, the operation would take all elements after the starting position up to the end of the array. The code example below illustrates how the splice
method works.
const thirdArray = ["i", "am", "a", "master", "of", "arrays", "in", "javascript"]
const fourthArray = thirdArray.splice(0,4);
console.log(fourthArray); // ["i", "am", "a", "master"]
console.log(thirdArray); // ["of", "arrays", "in", "javascript"]
const fifthArray = thirdArray.splice(0);
console.log(fifthArray) // ["of", "arrays", "in", "javascript"]
console.log(thirdArray) // []
It is important to note that the splice()
method can also be used to add and remove elements from specific positions in an array as explained in the previous article on Arrays with JavaScript.
Congratulations on getting here. This article brings us to the end of Arrays with JavaScript. By now, you should feel confident about the use of array data structure, as you have now become a Master 🙇♂️🙇♀️. I wish you success in your software engineering career.
Subscribe to my newsletter
Read articles from Hussein Tijani directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
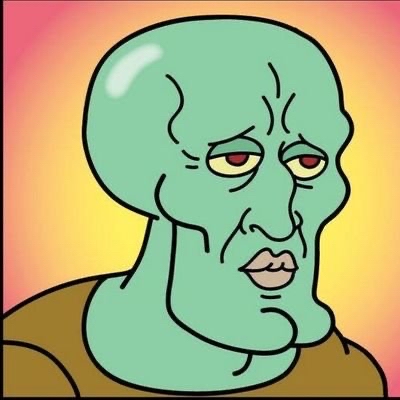
Hussein Tijani
Hussein Tijani
Hussein Tijani is a Software Engineer based in Lagos, Nigeria. His area of expertise encompasses the entire product development cycle, API design and development and third-party integrations. He is passionate about sharing his Software Engineering knowledge through technical articles and verbal conversations.