[JavaScript] Understanding XMLHttpRequest
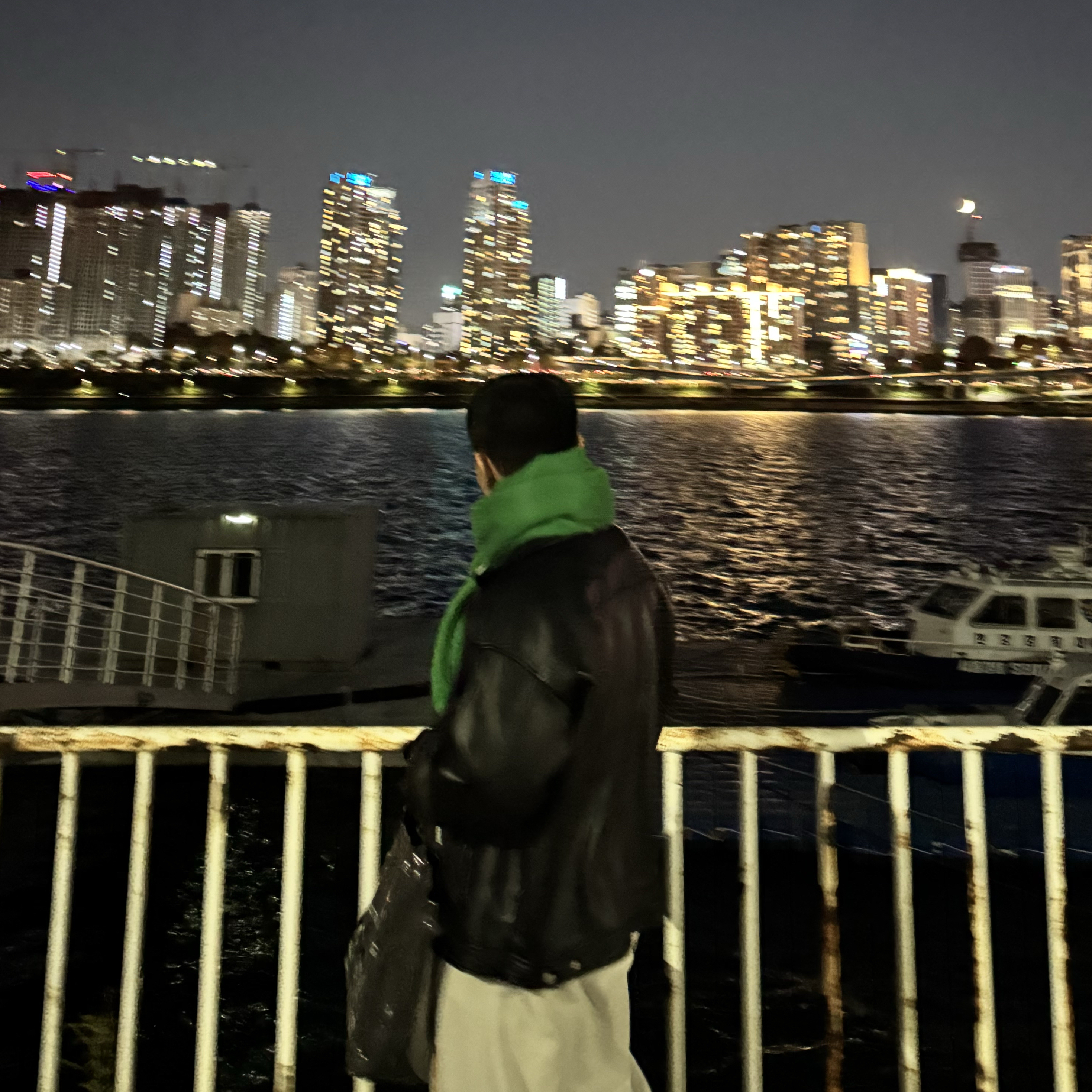
3 min read
Table of contents
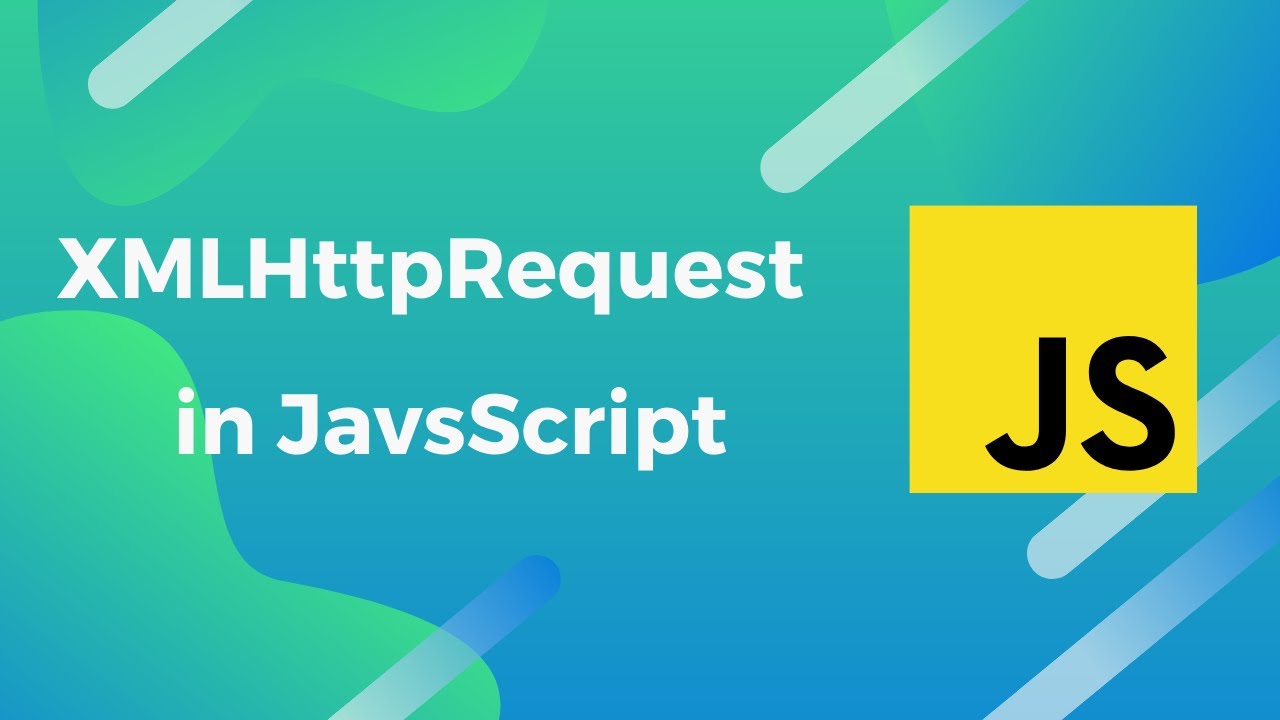
Introduction
This article will talk about:
GET to retrieve the data
POST to send the data.
PUT to replace the data.
DELETE to delete the data.
*note. I am using the JSON-server in this article.
GET Request
You can use the GET method to retrieve the data from the server.
function getData(url) {
return new Promise((resolve,reject)) => {
const xhr = new XMLHttpRequest();
xhr.open("GET", url);
xhr.setRequestHeader("content-type", "application/json");
xhr.send();
xhr.onload = () => {
// if xhr.status === 200, it means retrieving the data was successful.
if (xhr.status === 200) {
// The string type needs to be converted into JSON type.
const res = JSON.parse(xhr.response);
resolve(res);
} else {
// prints the status code and status text if failed to retrieve the data.
console.log(xhr.status, xhr.statusText)
reject(xhr.status);
};
}
}
// prints out the corresponding data.
getData("http://localhost:3000/posts").then((res) => {
console.log(res);
});
POST
You can use the POST method to send the data to the server.
function postData() {
const xhr = new XMLHttpRequest();
xhr.open("POST", "http://localhost:3000/posts");
xhr.setRequestHeader("content-type", "application/json;charset=UTF-8");
// specifies the send() because you are trying to send the data.
xhr.send(JSON.stringify(data)); // converts JSON data type to String.
xhr.onload = () => {
// if xhr.status === 201, it means sending the data was successful.
if (xhr.status === 201) {
// The string type needs to be converted into JSON type.
const res = JSON.parse(xhr.response);
console.log(res);
} else {
// prints the status code and status text if failed to retrieve the data.
console.log(xhr.status, xhr.statusText);
};
}
}
PUT
You can use the PUT method to replace the data.
function postData() {
const xhr = new XMLHttpRequest();
// I put 2 at the back of the URL to specify that I want to replace the data with ID number = 2.
xhr.open("PUT", "http://localhost:3000/posts/2");
xhr.setRequestHeader("content-type", "application/json;charset=UTF-8");
// The data you want to replace with.
const data = { title: "HTML", author: "John Doe" };
// specifies the send() because you are trying to send the data.
xhr.send(JSON.stringify(data)); // converts JSON data type to String.
xhr.onload = () => {
// if xhr.status === 200, it means replacing the data was successful.
if (xhr.status === 200) {
// The string type needs to be converted into JSON type.
const res = JSON.parse(xhr.response);
console.log(res);
} else {
// prints the status code and status text if failed to retrieve the data.
console.log(xhr.status, xhr.statusText);
};
}
}
DELETE
function postData() {
const xhr = new XMLHttpRequest();
// I put 2 at the back of the URL to specify that I want to delete the data with ID number = 2.
xhr.open("DELETE", "http://localhost:3000/posts/2");
xhr.setRequestHeader("content-type", "application/json;charset=UTF-8");
// The data you want to replace with.
const data = { title: "HTML", author: "John Doe" };
// specifies the send() because you are trying to send the data.
xhr.send(JSON.stringify(data)); // converts JSON data type to String.
xhr.onload = () => {
// if xhr.status === 200, it means deleting the data was successful.
if (xhr.status === 200) {
// The string type needs to be converted into JSON type.
const res = JSON.parse(xhr.response);
console.log(res);
} else {
// prints the status code and status text if failed to retrieve the data.
console.log(xhr.status, xhr.statusText);
};
}
}
0
Subscribe to my newsletter
Read articles from Lim Woojae directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
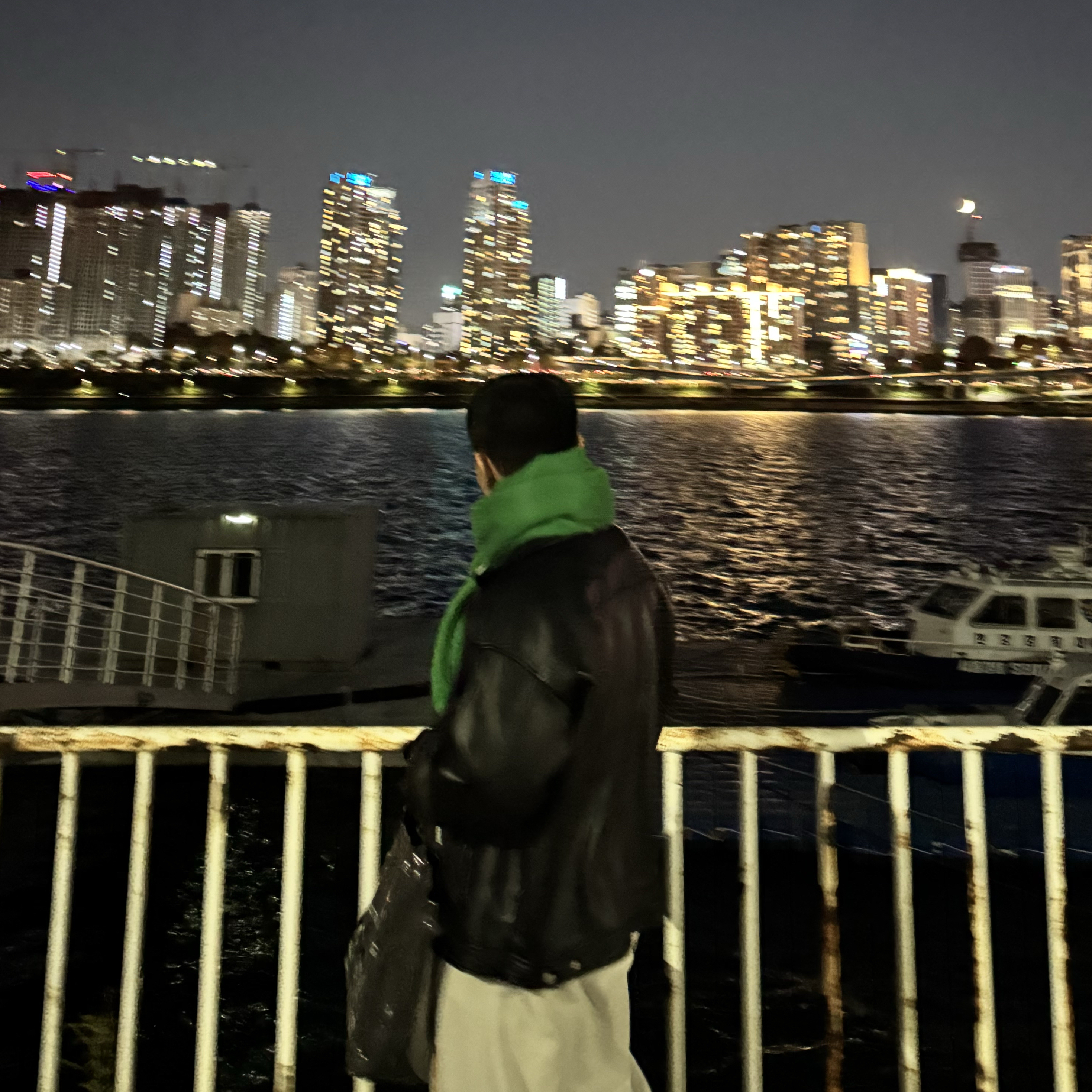
Lim Woojae
Lim Woojae
Computer Science Enthusiast with a Drive for Excellence | Data Science | Web Development | Passionate About Tech & Innovation