Advanced Templates in C++: Expression Templates, Template Constraints, and Type Traits
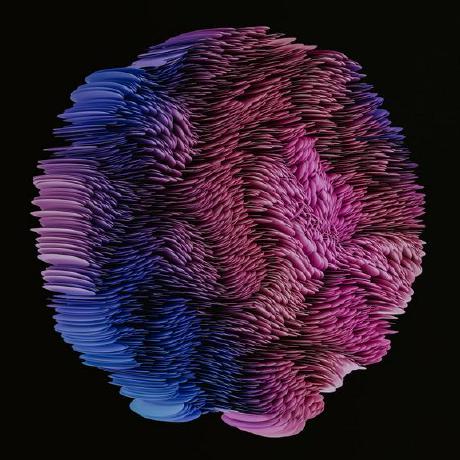
Table of contents
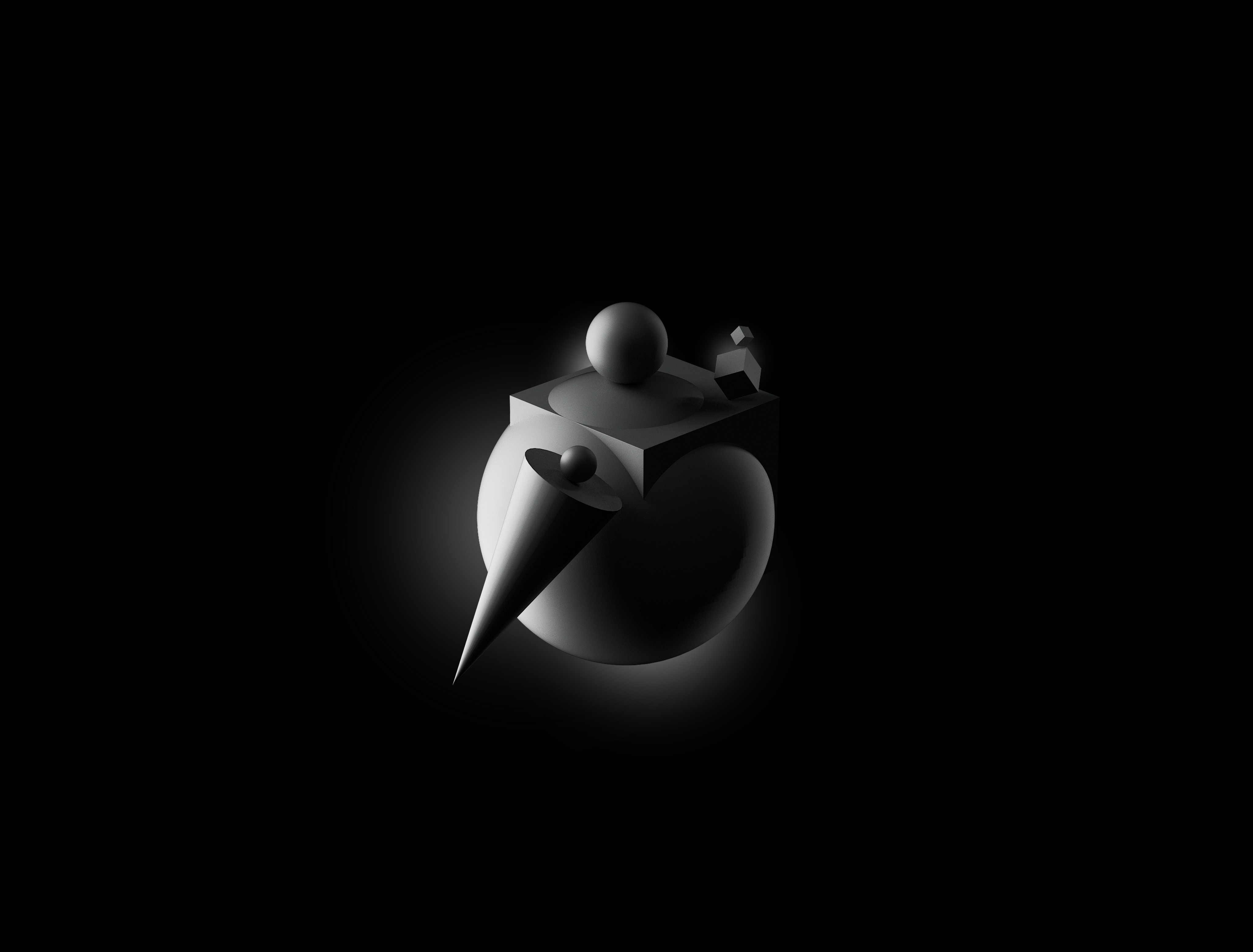
Templates in C++ allow for generic programming, enabling the creation of reusable code that can work with different data types. In this article, we will explore advanced template topics, including expression templates, template constraints, and type traits.
Expression Templates
Expression templates are a technique for optimizing the evaluation of mathematical expressions involving objects of templated classes. They rely on compile-time expression manipulation to generate efficient code by delaying the evaluation of expressions until the final result is assigned. Expression templates can significantly improve the performance of mathematical operations involving objects such as matrices and vectors.
template <typename E>
class Expr {
public:
double operator[](int i) const {
return static_cast<const E&>(*this)[i];
}
};
template <typename E1, typename E2>
class AddExpr : public Expr<AddExpr<E1, E2>> {
const E1& _u;
const E2& _v;
public:
AddExpr(const E1& u, const E2& v) : _u(u), _v(v) {}
double operator[](int i) const {
return _u[i] + _v[i];
}
};
template <typename E>
class Vec : public Expr<Vec<E>> {
std::vector<double> _data;
public:
// ... Constructors, assignment operators, etc. ...
double operator[](int i) const {
return _data[i];
}
template <typename E2>
Vec& operator+=(const Expr<E2>& v) {
for (int i = 0; i < _data.size(); ++i) {
_data[i] += v[i];
}
return *this;
}
};
template <typename E1, typename E2>
AddExpr<E1, E2> operator+(const Expr<E1>& u, const Expr<E2>& v) {
return AddExpr<E1, E2>(static_cast<const E1&>(u), static_cast<const E2&>(v));
}
Template Constraints
Template constraints, introduced in C++20, allow you to specify requirements on template parameters using concepts. Constraints make your templates more robust by providing better error messages and ensuring that only the intended types can be used with your templates.
#include <concepts>
template <typename T>
concept Addable = requires(T a, T b) {
{ a + b } -> std::same_as<T>;
};
template <Addable T>
T add(const T& a, const T& b) {
return a + b;
}
Type traits
Type traits are a collection of templates that provide information about types and their properties at compile-time. They can be used to implement type-specific optimizations, static assertions, and other compile-time logic.
#include <type_traits>
template <typename T>
void foo(const T& value) {
if constexpr (std::is_integral_v<T>) {
// Perform integer-specific operations
} else if constexpr (std::is_floating_point_v<T>) {
// Perform floating-point-specific operations
} else {
// Perform generic operations
}
}
Conclusion
Advanced template topics in C++ provide powerful tools for creating efficient, flexible, and maintainable code. In this article, we explored expression templates, template constraints, and type traits. Understanding these advanced techniques is essential for mastering templates and generic programming in C++. As you continue to explore C++ programming, you will encounter even more advanced techniques that build upon these foundations.
Subscribe to my newsletter
Read articles from Enzo Hugonnier directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
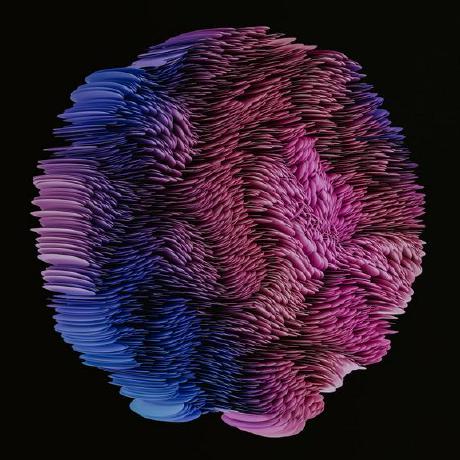