Improving Performance with React's Experimental useTransition Hook in TypeScript
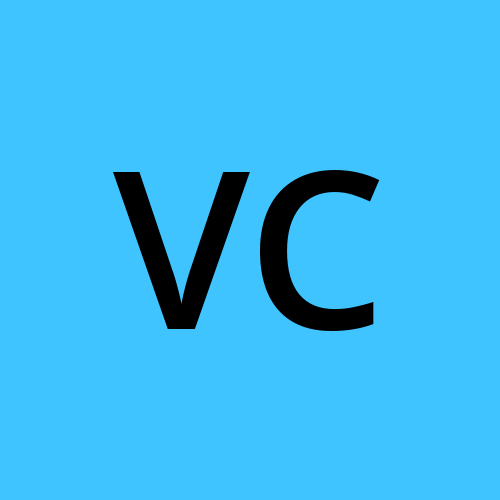
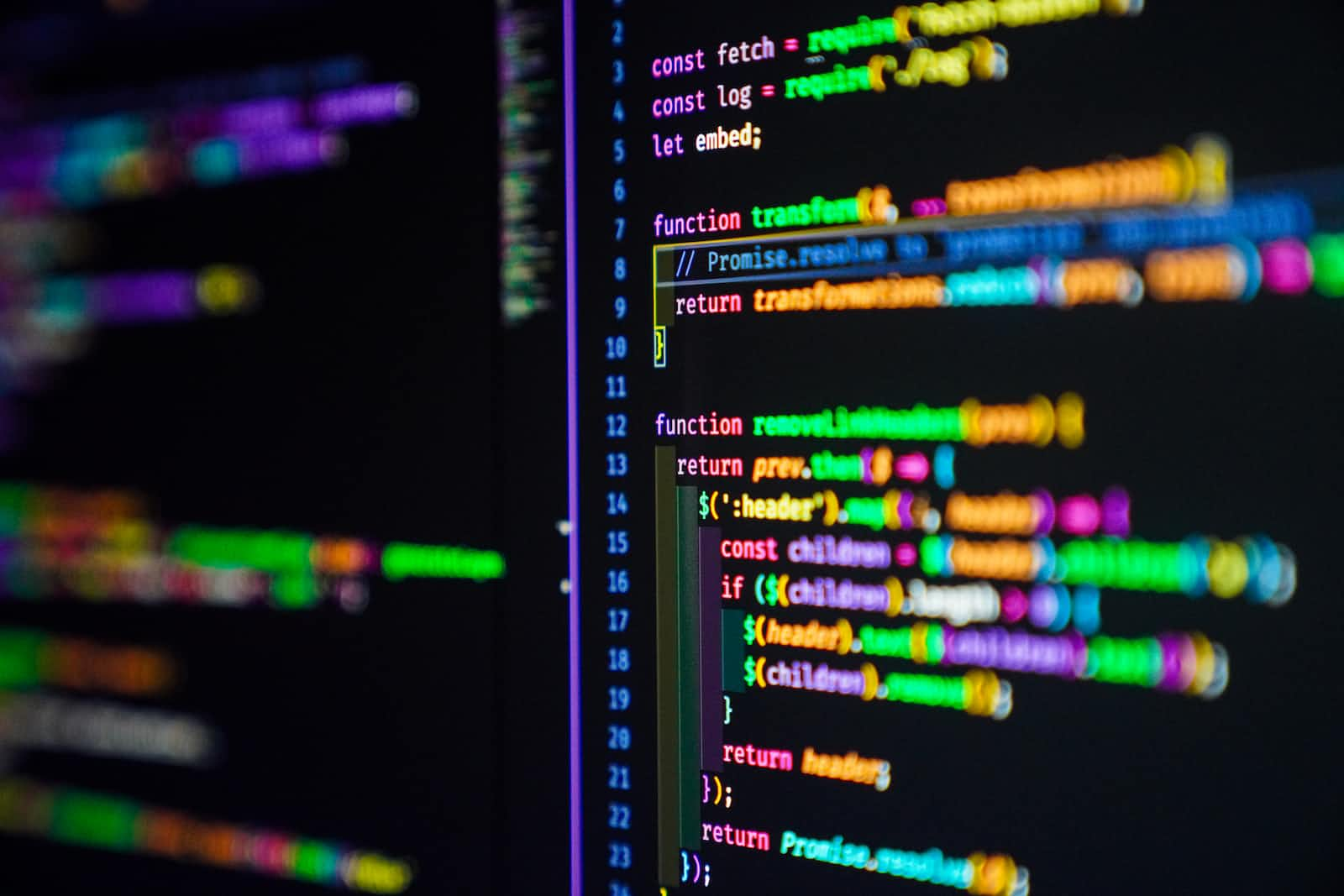
Introduction:
React is a popular JavaScript library for building user interfaces, and performance is a crucial aspect of creating responsive and engaging experiences for users. React's experimental Concurrent mode introduces the useTransition
hook, which provides a way to manage resource fetching and component rendering while keeping the UI responsive. In this blog post, we will explore how to use the useTransition
hook to optimizing performance in your React applications using TypeScript and arrow functions for components.
Prerequisites:
Before diving into the useTransition
hook, make sure you have the following:
A basic understanding of React, its Hooks, and TypeScript.
A development environment with React Concurrent mode enabled. Please note that this feature is experimental and not yet recommended for production use.
Using the useTransition Hook:
The useTransition
hook allows you to delay the rendering of a component while fetching data, providing a smoother user experience by preventing the UI from freezing or showing a loading state.
Let's see how it works with a simple example using a long array:
First, we need to import
useTransition
andSuspense
from React:import React, { useState, Suspense } from 'react'; import { useTransition } from 'react';
Let's create a simple component that fetches a long array of data:
const fetchData = (): Promise<string[]> => new Promise((resolve) => setTimeout( () => resolve( Array.from({ length: 1000 }, (_, i) => `Item ${i + 1}`) ), 3000 ) ); const DataComponent: React.FC = () => { const data = fetchData(); return ( <div> {data.map((item) => ( <div key={item}>{item}</div> ))} </div> ); };
Now, we will use the
useTransition
hook to manage the rendering of theDataComponent
:const App: React.FC = () => { const [isPending, startTransition] = useTransition(); const [showData, setShowData] = useState(false); const handleClick = () => { startTransition(() => { setShowData(!showData); }); }; return ( <div> <button onClick={handleClick}>Toggle Data</button> <div> <Suspense fallback={<div>Loading...</div>}> {showData && <DataComponent />} </Suspense> {isPending && <div>Fetching data...</div>} </div> </div> ); }; export default App;
In this example, we used the
useTransition
hook to manage the rendering of theDataComponent
. ThestartTransition
function allows us to delay the rendering of the component while the data is being fetched, and theisPending
boolean lets us know if the transition is still in progress. TheSuspense
component wraps theDataComponent
and displays a loading state until the data is ready.
Conclusion:
The experimental useTransition
hook in React Concurrent mode provides a powerful way to manage resource fetching and component rendering, improving performance and providing a smoother user experience. While this feature is still experimental and not recommended for production use, it offers a glimpse into the future of React and the exciting possibilities it holds for building more responsive and engaging applications.
Subscribe to my newsletter
Read articles from vikash choudhary directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
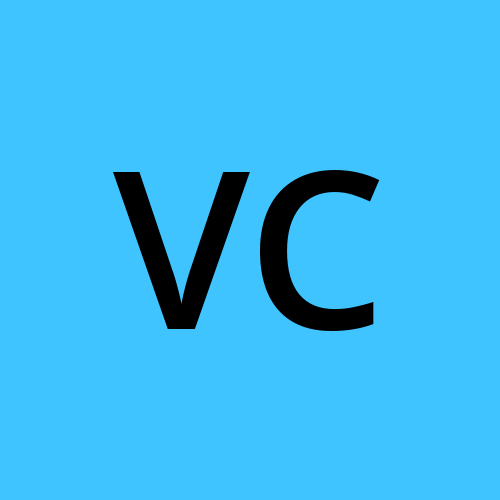