7 <git> commands every coder should know ๐คทโโ๏ธ
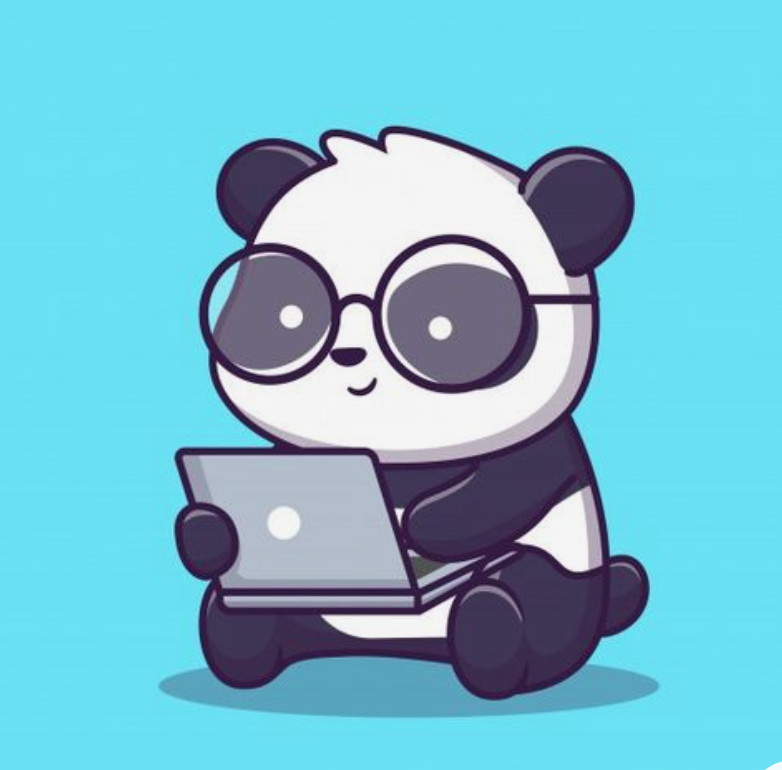
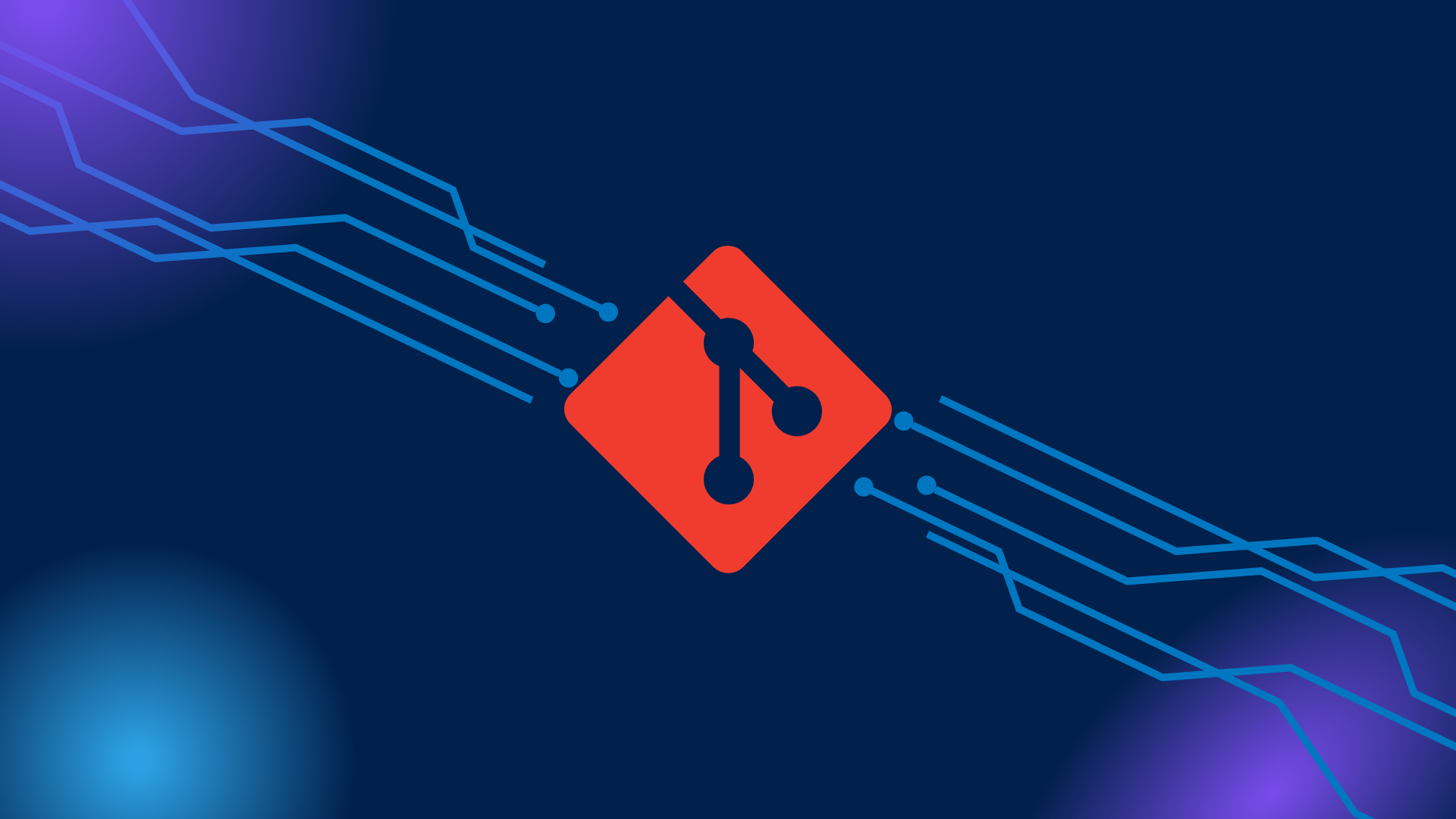
init ๐ค
When you use the git init command, you're telling Git to set up a new place to save all the changes you make to your project. This special place is called a "repository".
When you run git init, Git will create a new folder called ".git" inside the folder you're currently in. This folder is where Git keeps track of everything it needs to know about your project. It will contain all the files and information Git needs to manage your project's version control.
So, running git init is like telling Git, "Hey, I want to use version control for this project, so please create a special folder to help me keep track of all the changes I make."
git init
After running git init, you can start adding files to your repository and commit changes to keep track of changes to your project's code.
status โน๏ธ
When you're working on a project with Git, you can use the git status command to check what's going on. It's like asking Git, "Hey, what's up? What changes have been made?"
When you run git status, Git will tell you if any files have been changed, added, or deleted since the last time you checked. It will also let you know if there are any files that you haven't told Git to keep track of yet.
For example, imagine you're working on a website and you've made changes to the homepage. When you run git status, Git might tell you something like:
git status
Here are the most common statuses that you might see:
Changes not staged for commit: These are changes that have been made to tracked files, but have not been staged to be committed yet.
Changes to be committed: These are changes that have been staged to be committed in the next commit.
Untracked files: These are files in your project directory that are not being tracked by Git yet.
add โ
So, let's say you're working on a project in Git, and you've made some changes to the files. Before you can actually save those changes, you need to tell Git which ones you want to keep track of. That's where the git add
command comes in.
Using git add
is like telling Git, "Hey, I want to save these changes!" You can add specific files or add all changes at once using git add .
command.
git add .
So, let's say you've made some changes to a single file in your project, and you want to stage those changes using git add
.
To add a single file, you'll need to use the git add
command followed by the file name.
git add 'filename'
to add all the files
ignore ๐ซ
When you're working on a Git project, there might be some files that you don't want to track with Git. Maybe they're temporary files, log files, or files that contain sensitive information like passwords.
That's where the git ignore
command comes in handy. Basically, you create a file called .gitignore
in the root directory of your Git project. In this file, you list the names of all the files or directories that you want Git to ignore.
For example, let's say you don't want to track any files with the extension .log
. You would create a .gitignore
file and add the following line:
*.log
This tells Git to ignore all files that end in .log. You can also ignore entire directories by adding their names to the .gitignore file.
Once you've created your .gitignore file, Git will automatically ignore any files or directories that match the patterns you've specified. This means that they won't show up in your git status output, and they won't be included in any commits or pushes.
Using git ignore is a great way to keep your Git project clean and organized, and to avoid accidentally committing or pushing sensitive information.
branch ๐
git branch
So, let's say you're working on a project in Git and you want to start experimenting with some new features without affecting the code in your main branch. That's where the git branch
command comes in.
When you run git branch
, Git will show you a list of all the branches that currently exist in your repository. The current branch you're working on will be highlighted with an asterisk.
You can also use git branch
to create a new branch. This is like making a copy of your current codebase, so you can make changes without affecting the main branch.
git branch 'newBranchName'
This would create a new branch called "newBranchName", which would be a copy of your current branch. You could then switch to that branch using git checkout newBeanchName
, and start making changes without worrying about breaking anything in the main branch.
git checkout 'newBranchName'
This would switch your working directory to the "newBranchName" branch, allowing you to make changes and commit them to that branch.
Commit, did I just say that, well, not to worry, I will come to that in a while.
Before that, let me explain the git branch -d
command briefly :
So, you know how Git allows you to create different branches for your project? Well, sometimes you might want to delete one of those branches that you don't need anymore. That's where the git branch -d
command comes in handy!
git branch -d 'branchName'
This will delete the branch and remove it from your Git repository.
clone ยฉ
The git clone
command is like making a photocopy of someone else's project. You can make a copy of the entire project, including all of its history and branches, and start working on it on your own computer.
To use git clone
, you just need to know the URL of the repository you want to clone. Once you have that, you can run git clone
followed by the repository URL, and Git will create a new directory with the same name as the repository and copy everything from the original repository to your computer.
git clone 'url of the remote git repo'
The cool thing about git clone is that it creates a complete copy of the project, so you can start working on it right away. You can even switch to any of the existing branches and continue working on the project from there.
So, if you want to work on someone else's project, or create a copy of your own project to work on from a different computer, git clone is the way to go!
pull,commit & push ๐
pull
So, you know how sometimes you're working on a project with other people, and they make changes to the project that you want to include in your own copy? That's where the git pull
command comes in handy!
Basically, git pull
is like grabbing the latest version of the project from the internet and merging it with your own copy. It's kind of like updating your phone's apps โ you know how you get notifications for updates, and then you tap the "Update" button to get the latest version? That's kind of like what git pull
does.
git pull 'remote repo'
If already on the branch you want to take latest of, do:
git pull
When you run git pull, Git will automatically fetch any new changes from the internet (from the remote repository), and merge them with your own copy of the project. That way, you have the latest version of the project with all the changes that other people have made.
It's a good idea to run git pull pretty frequently when you're working on a project with other people, just to make sure you have the latest changes. That way, you can avoid conflicts and keep working smoothly.
commit
Have you ever made changes to a project and wanted to save them in a way that you can easily access them later on? Well, that's where git commit comes in!
Think of git commit as taking a snapshot of your changes at a specific point in time. It lets you save your changes to the Git repository and create a new commit that represents your changes.
To use git commit, you first need to stage the changes that you want to include in your commit using git add. Once you've staged your changes, you can use git commit to create a new commit with a message describing your changes.
For example, you might run a command like this:
git commit -m "your commit message"
push
So you've made some changes to your local Git repository and now you want to share those changes with your team or collaborators on a remote server. That's where the git push
command comes in!
git push
is used to send your local commits to a remote repository, such as on GitHub or Bitbucket. This will update the remote repository with your changes so that others can see them.
To use git push
, you first need to commit your changes using git commit
. Once you have committed your changes, you can use git push
to upload those changes to the remote repository.
For example, if you have a remote repository called "origin" and a branch called "main", you might run a command like this:
git push
So, git push
is used to send your local changes to a remote repository so that others can access them. It's a crucial command for collaborating with others and sharing your work.
Always remember this:
In case of any emergency ๐
git commit โ
git push ๐
git out ๐โโ๏ธ
A Basic Flow
To make changes or add a new feature to a project, you should first take a pull from the main branch to get the latest version. Then, create a new branch and switch to it. This is where you'll make your changes. Once you're done, commit your changes and push them to the remote repository. Finally, you can delete the branch you created earlier. This helps keep the project organized and makes it easier to manage changes over time.
Subscribe to my newsletter
Read articles from NAVJOT SINGH directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
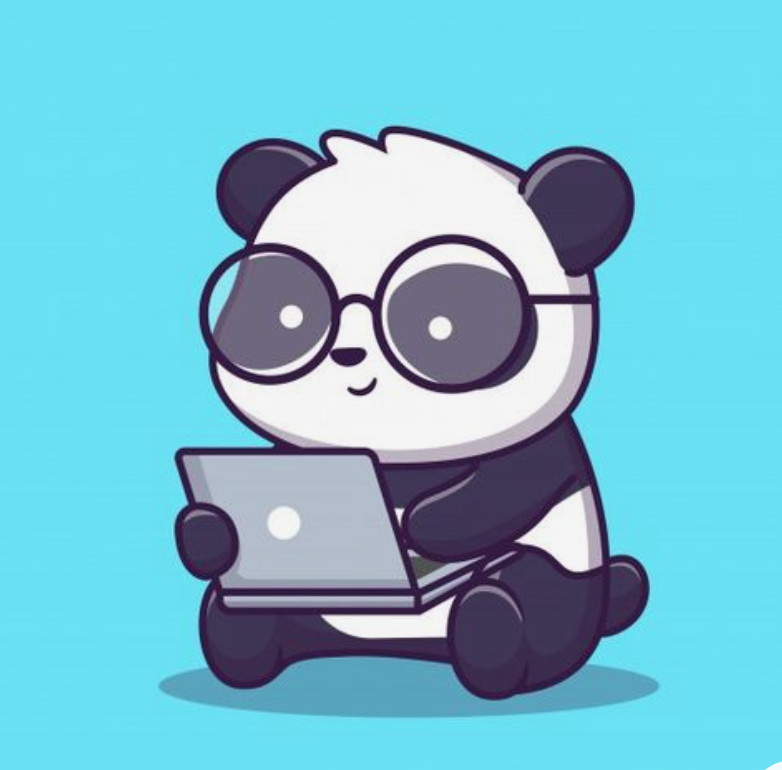