The Unique Features of JavaScript Arrays
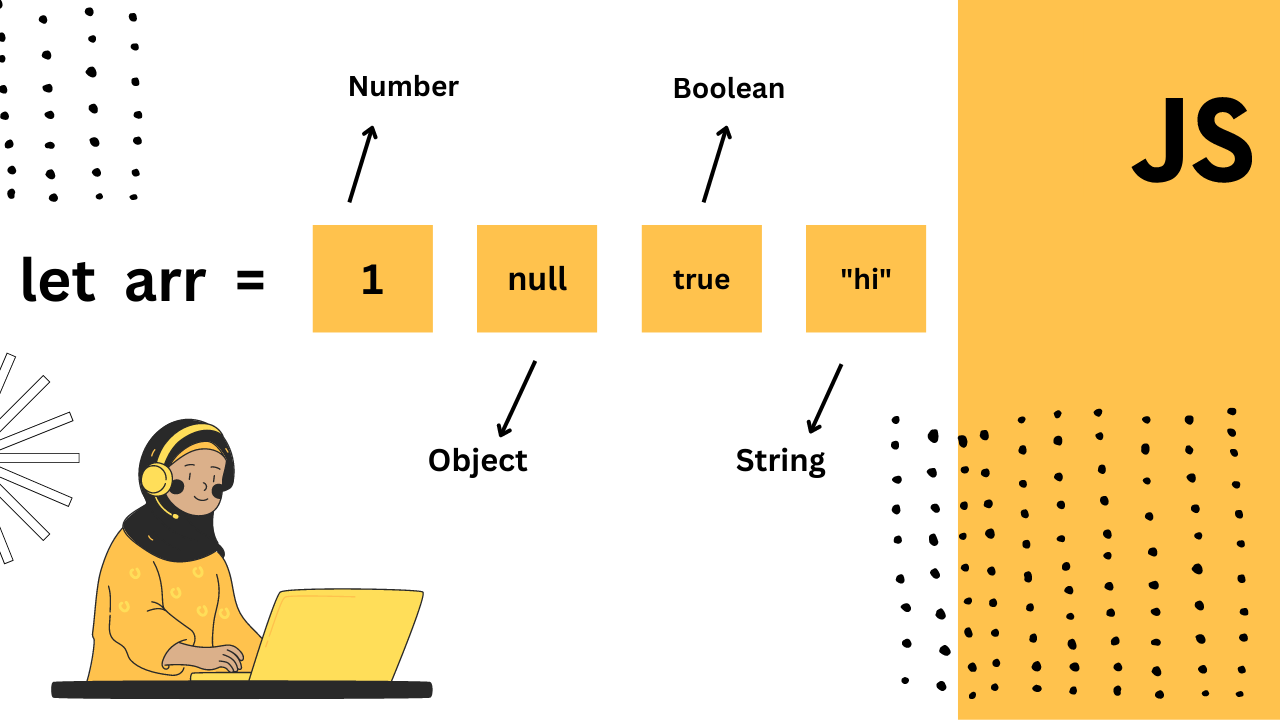
Storing elements of different types:
In JavaScript, arrays can contain different types of data types such as numbers, objects, strings, or boolean
let arr = [1, "3", "hi", true, null]
Array methods :
unlike String, Array is mutable. we can modify the array as per our requirements.
JavaScript arrays contain built-in methods by which you can easily manipulate the array. many programming languages do not have built-in methods and they have to write the code to manipulate the arrays.
built-in methods are:
There are so many array methods in JavaScript but here I listed a few common methods
Push()
pop()
shift()
unshift()
sort()
every()
Sparse Array
sparse array means an array that contains mostly undefined or empty elements with only a few non-empty elements.
Let's say you have declared an array of 10 elements, but you have only defined values for the first 4 elements.
let arr = [1, , , true,"array",null , ,] // here the array is of length 8 but we have defined values for four elements only
Dynamic Size:
In many programming languages, arrays are fixed in size, which means that once you have declared the size of an array, you cannot change it later on. However, in JavaScript, arrays are dynamic in size, which means that you can add or remove elements as needed.
let arr = [1, 2, 3]; // Declare an array with three elements
arr.push(null); // Add a fourth element to the end of the array
console.log(arr); // Output: [1, 2, 3, null]
Thank you for taking the time to read this blog. I hope that you found it informative, useful, and engaging. If you have any questions or comments, please feel free to share them in the comment section.
Subscribe to my newsletter
Read articles from misba directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
misba
misba
As someone who is passionate about technology, I have set my sights on becoming a full-stack developer. In addition to this, I am also very interested in the field of AI/ML. I am eager to learn more about this field and see how it can be applied to create innovative solutions. I am enhancing my knowledge and sharing my thoughts through blog posts.