Building a Real-Time Chat App with Node.js and Socket.io

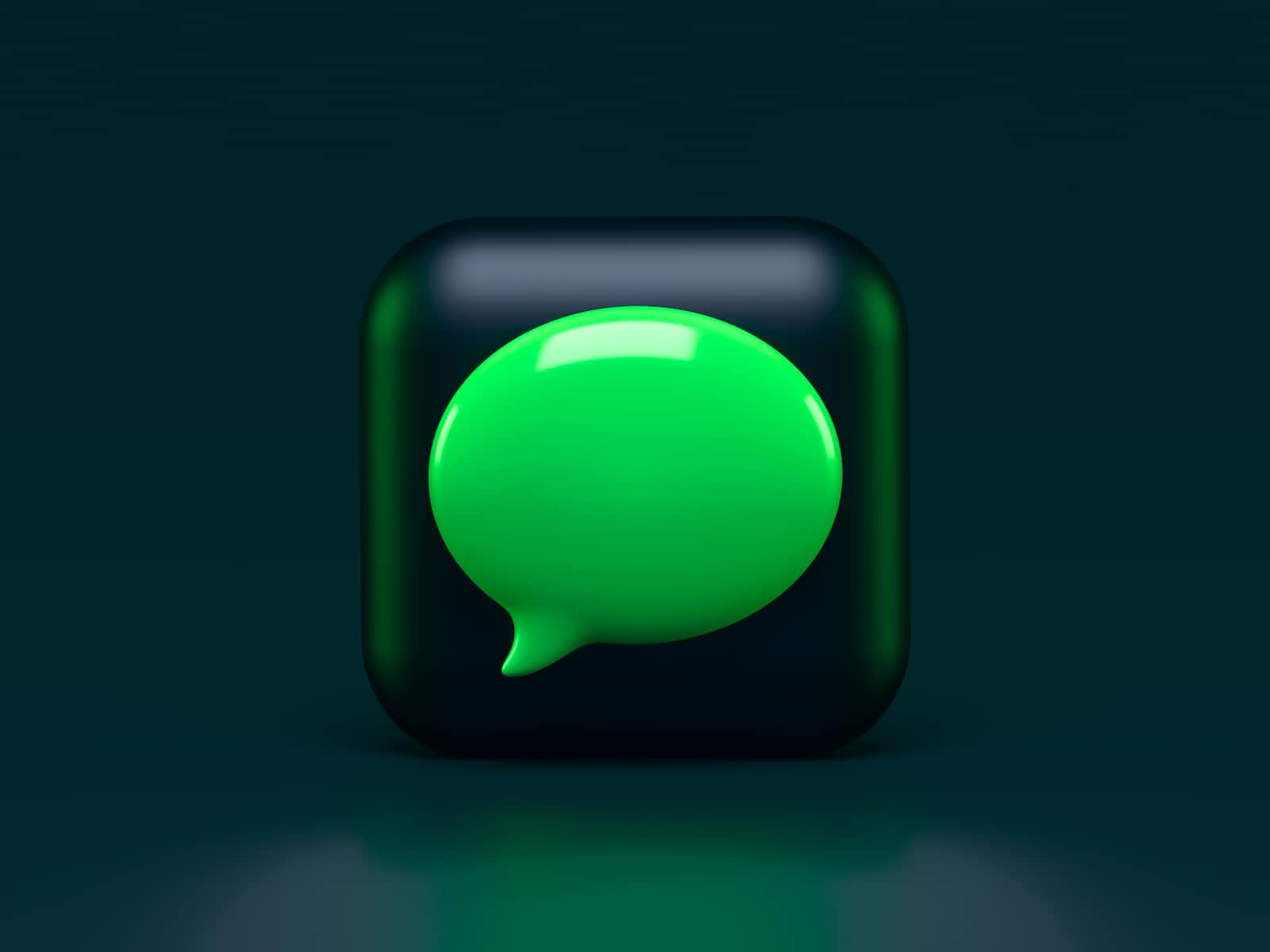
Real-time chat applications are becoming increasingly popular in today's world, and there are several ways to build one. In this article, we will explore how to build a real-time chat application using Socket.io and Node.js. Socket.io is a JavaScript library that enables real-time communication between the client and the server, while Node.js is a runtime environment that enables the development of server-side applications using JavaScript. Together, they provide an excellent platform for building real-time chat applications.
Setting Up the Project
To start building our real-time chat application, we need to set up the project. First, we need to create a new directory and initialize a new Node.js project. We can use the following commands to achieve this:
mkdir real-time-chat-app
cd real-time-chat-app
npm init -y
The npm init -y
command initializes a new Node.js project with default settings.
Next, we need to install the required dependencies, including Express, Socket.io, and nodemon. Express is a popular Node.js framework for building web applications, while nodemon is a tool that helps to monitor changes to the project and restart the server automatically. We can install the dependencies using the following command:
npm install express socket.io nodemon
Building the Server
Once we have set up the project and installed the dependencies, we can now start building the server. In this example, we will use Express to create the server and Socket.io to enable real-time communication between the client and the server.
// Import required modules
const express = require('express');
const http = require('http');
const socketio = require('socket.io');
// Create a new instance of the Express app
const app = express();
// Create a new HTTP server using the Express app
const server = http.createServer(app);
// Create a new instance of Socket.io and attach it to the HTTP server
const io = socketio(server);
// Start the server
const PORT = process.env.PORT || 3000;
server.listen(PORT, () => {
console.log(`Server started on port ${PORT}`);
});
In the above code, we first import the required modules, including Express, http, and Socket.io. We then create a new instance of the Express app, a new HTTP server using the Express app, and a new instance of Socket.io attached to the HTTP server. Finally, we start the server on the specified port using the server.listen
method.
Building the Client
Once we have built the server, we can now build the client-side of the application. In this example, we will create a simple HTML file with a form to submit messages to the server.
<!DOCTYPE html>
<html>
<head>
<title>Real-Time Chat App</title>
</head>
<body>
<form id="message-form">
<input type="text" name="message" placeholder="Enter message">
<button>Send</button>
</form>
<ul id="messages"></ul>
<script src="/socket.io/socket.io.js"></script>
<script src="/js/chat.js"></script>
</body>
</html>
In the above code, we first create a form with an input field to enter messages and a button to submit the messages to the server. We also create an unordered list to display the messages received from the server.
We then include the Socket.io client library and a separate JavaScript file that contains the client-side code for the chat application. The Socket.io client library allows the client to connect to the server and send and receive messages in real-time.
Handling Socket Connections
Once we have built the client-side of the application, we need to handle socket connections on the server-side. Socket.io provides a set of events that allow us to handle socket connections, including the connection
event, which is emitted when a client connects to the server.
// Handle socket connections
io.on('connection', (socket) => {
console.log(`New user connected: ${socket.id}`);
});
Here, we use the io.on
method to handle socket connections. When a client connects to the server, the connection
event is emitted, and we log a message to the console with the socket ID.
Handling Chat Messages
Once a client has connected to the server, we need to handle chat messages sent from the client. Socket.io provides a socket.emit
method that allows us to emit custom events from the client to the server. We can use this method to emit a sendMessage
event with the message content when the user submits the form.
<form id="message-form">
<input type="text" name="message" placeholder="Enter message">
<button>Send</button>
</form>
<script>
const form = document.querySelector('#message-form');
const input = form.querySelector('input');
form.addEventListener('submit', (e) => {
e.preventDefault();
const message = input.value;
socket.emit('sendMessage', message);
input.value = '';
input.focus();
});
</script>
In the above code, we first select the form and input field using the querySelector
method. We then add an event listener to the form to listen for the submit
event. When the user submits the form, we prevent the default form submission behavior using the e.preventDefault
method.
We then get the message content from the input field, emit a sendMessage
event with the message content using the socket.emit
method, clear the input field, and set the focus back to the input field.
On the server-side, we can handle the sendMessage
event using the socket.on
method.
// Handle chat messages
io.on('connection', (socket) => {
console.log(`New user connected: ${socket.id}`);
socket.on('sendMessage', (message) => {
io.emit('message', message);
});
});
In the above code, we use the socket.on
method to handle the sendMessage
event. When a sendMessage
event is emitted from the client, we emit a message
event to all connected clients using the io.emit
method.
Displaying Chat Messages
Finally, we need to display the chat messages received from the server on the client-side. We can handle the message
event emitted from the server using the socket.on
method.
// Handle chat messages
socket.on('message', (message) => {
const messages = document.querySelector('#messages');
const li = document.createElement('li');
li.innerText = message;
messages.appendChild(li);
});
In the above code, we select the unordered list element using the querySelector
method and create a new list item element using the createElement
method. We then set the inner text of the list item to the message content and append the list item to the unordered list using the appendChild
method.
Keep in Mind
It's important to note that this is just a basic example of what you can achieve with Socket.io and Node.js. There are many other features that you can add to your chat application, such as user authentication, message history, and user presence. You can also incorporate other technologies such as databases to persist the chat messages and user data.
Overall, building a real-time chat application with Socket.io and Node.js can seem daunting at first, but with the right guidance and approach, it can be a fun and rewarding experience. With the knowledge gained from this article, you can begin exploring the possibilities of Socket.io and Node.js to build real-time chat applications that meet the needs of your users.
Subscribe to my newsletter
Read articles from Clint directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Clint
Clint
Writer, software engineer, content creator, and all-around awesome guy.