Sorting
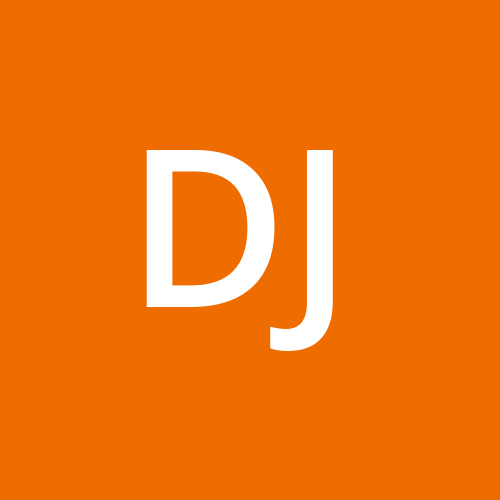
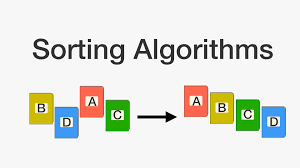
First, what's sorting, Sorting is a way in which data can be in ascending(small to big) or descending(big to small) order it reduces the human effort and time to get the smallest or largest value according to need.
There are various methods to do the sorting of the data. But the finest way is which we get the data in minimum time complexity (time complexity - time taken to perform the particular task).
There are the most popular types of sorting techniques:-
a.) Bubble sort
b.) Selection sort
c.) Merge sort
d.) Insertion sort
e.) Quick sort
Firstly let's talk about bubble sort
Bubble sort is a simple sorting algorithm that works by repeatedly swapping adjacent elements if they are in the wrong order. It gets its name from the way the smaller elements "bubble" to the top of the list, much like bubbles rising to the surface of the water.
The basic idea behind bubble sort is to start at the beginning of the list and compare each pair of adjacent elements. If they are in the wrong order (i.e., the first element is greater than the second), they are swapped. This process is repeated over and over again until no more swaps are needed.
Now let's take a look at the selection sort
Selection sort is a simple sorting algorithm that works by repeatedly finding the minimum element from an unsorted portion of the list and moving it to the beginning of the list.
Here's how selection sort works:
Set the first element of the list as the minimum value.
For each element from the second element to the last element of the list, compare it with the current minimum value.
If the current element is smaller than the minimum value, set it as the new minimum value.
After comparing all elements, swap the minimum value with the first unsorted element (i.e., the first element that comes after the sorted portion of the list).
Repeat steps 2-4 for the remaining unsorted portion of the list.
Thirdly Merge sort
Merge sort is a divide-and-conquer sorting algorithm that works by dividing the input list into two halves, sorting each half separately, and then merging the two sorted halves into a single sorted list.
Here's how merge sort works:
Divide the input list into two halves, roughly equal in size.
Recursively sort each of the two halves by applying the same divide-and-conquer strategy.
Merge the two sorted halves into a single sorted list. This is done by repeatedly comparing the first elements of each half and selecting the smaller one to be the next element of the merged list.
Repeat step 3 until all elements of both halves have been merged into the final sorted list.
Fourthly Insertion sort
Insertion sort is a simple sorting algorithm that works by repeatedly inserting each element of an unsorted list into its proper place in a sorted portion of the list.
Here's how insertion sort works:
Start with the second element of the list.
Compare the second element with the first element. If the second element is smaller, swap them.
Move to the third element of the list.
Compare the third element with the second element. If the third element is smaller, swap them.
Compare the third element with the first element. If the third element is smaller, swap them.
Continue this process for each element in the list, comparing it with the sorted elements that come before it and inserting it in its proper place in the sorted portion of the list.
When you reach the end of the list, you will have a fully sorted list.
Lastly Quick sort
Quick sort is a popular and efficient sorting algorithm that works by partitioning an array into two sub-arrays, one with elements less than a chosen pivot value and the other with elements greater than the pivot value. The algorithm then recursively sorts each sub-array until the entire array is sorted.
Here's how quick sort works:
Choose a pivot element from the array. This element can be any element of the array but is often chosen to be the first or last element of the array.
Partition the array into two sub-arrays, one with elements less than the pivot and the other with elements greater than the pivot. This is done by iterating through the array and moving elements smaller than the pivot to the left of the pivot and elements greater than the pivot to the right of the pivot. The pivot element itself is now in its final sorted position.
Recursively apply the above steps to the sub-arrays on either side of the pivot until the entire array is sorted.
here are some of the ways to sort the data.
In general, merge sort and quick sort are considered to be more efficient than bubble sort, selection sort, and insertion sort for large datasets.
Merge sort has a time complexity of O(n log n) in the worst-case scenario, where n is the number of elements in the array, while quick sort has an average case time complexity of O(n log n) and a worst-case time complexity of O(n^2). However, quick sort is often faster than merge sort in practice because it has a better cache locality and requires less memory.
It's important to note that the actual performance of a sorting algorithm depends on a variety of factors, such as the size and nature of the dataset, the computer hardware used, and the implementation of the algorithm. Therefore, it's often a good idea to benchmark and compare different sorting algorithms on the specific dataset and hardware in question to determine which one is the most efficient for that particular scenario.
Subscribe to my newsletter
Read articles from Dheeraj Jha directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
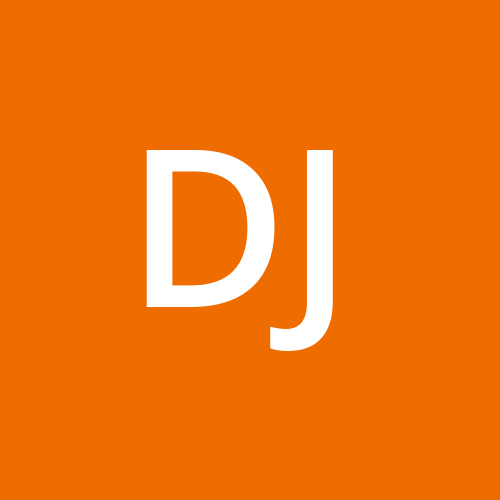