Demystifying Hash Tables: Boosting Data Efficiency with Smart Key-Value Pairing
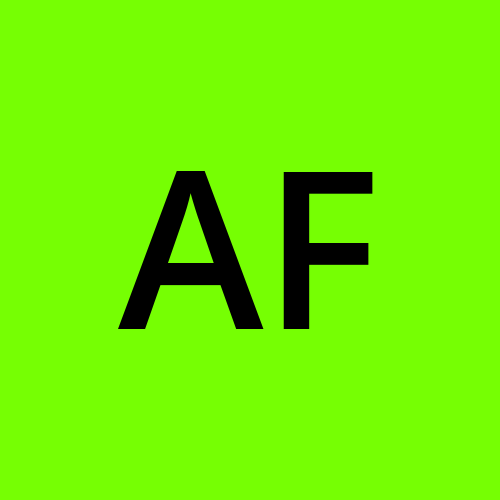
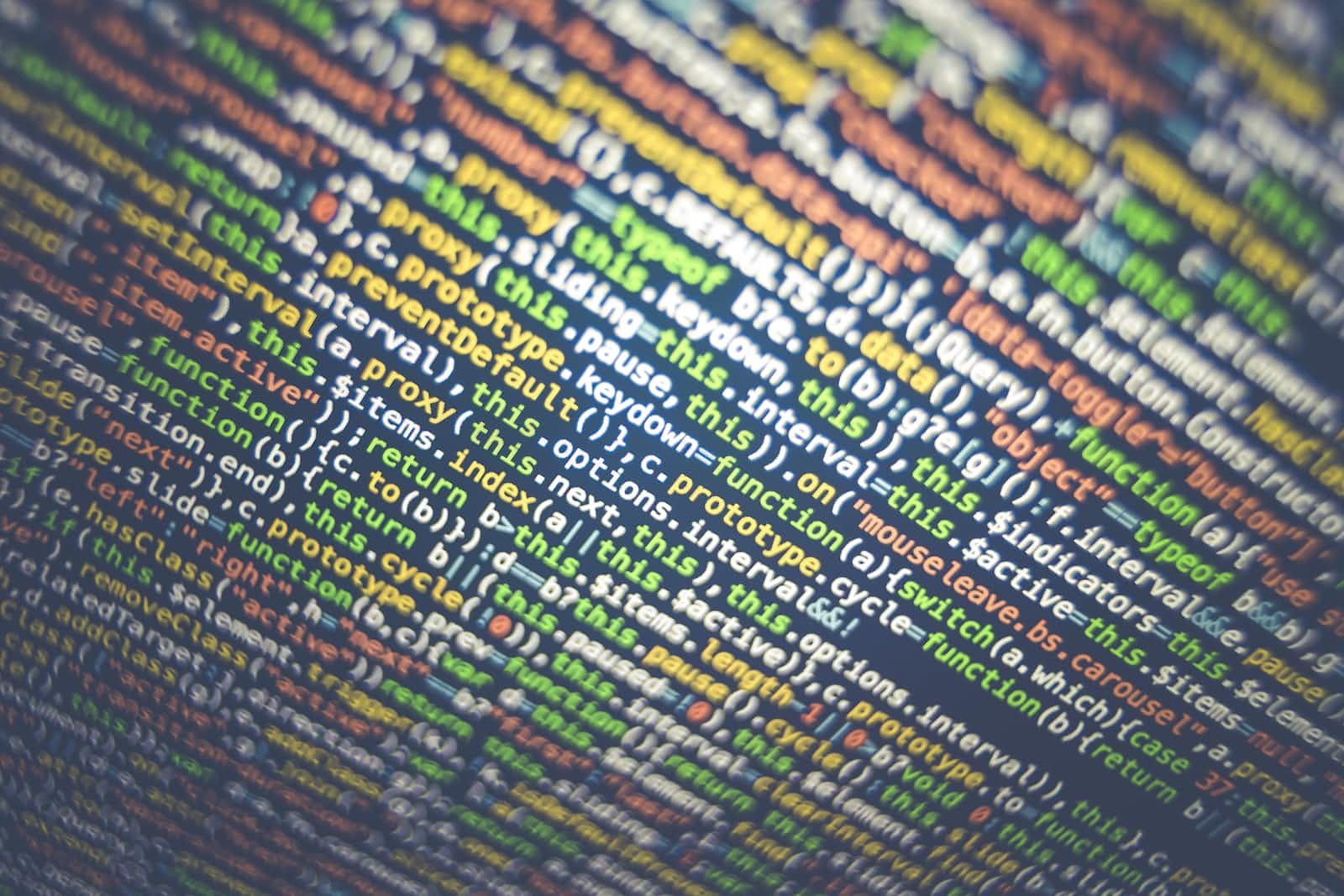
Introduction
In today's data-driven world, efficient storage and retrieval of information is crucial for optimal performance. Hash tables provide an efficient solution for storing and retrieving key-value pairs. The blog post aims to demystify hash tables, explain their inner workings, highlight their benefits, and provide guidance on implementation and optimization.
Introduction to hash tables as a fundamental data structure: Hash tables provide an efficient solution for storing and retrieving key-value pairs. They are used in a wide variety of applications, including databases, caching systems, and symbol tables for programming languages.
The blog post aims to demystify hash tables by explaining their inner workings, highlighting their benefits, and providing guidance on implementation and optimization.
Understanding Hash Tables
Hash tables, known by different names like hash maps, maps, unordered maps, dictionaries, or objects in JavaScript, are indispensable data structures in the field of computer science. They serve as a powerful tool for efficient data organization and retrieval. The versatility of hash tables is reflected in their widespread implementation across different areas, including databases and caching systems. Due to their ability to provide fast and convenient access to data, hash tables have become an essential component in numerous applications, demonstrating their significance and practical utility.
Hash tables are data structures that use a hash function to map keys to indices for efficient retrieval. Key-value pairs allow for the association of data with unique identifiers for easy retrieval. Hash functions take input keys and transform them into numerical indices within the table.
The Inner Workings of Hash Tables
The hash function plays a vital role in generating a unique hash code from the given key. This hash code is then transformed into a table index by using the modulus operation. As an example, we can take a look at a basic hash function that is implemented in JavaScript. It takes a string key as input and returns the sum of its character codes. Then it performs the modulus operation on the sum using the table size to ensure that it falls within the range of table indices.
function simpleHash(key, tableSize) {
let hash = 0;
for (let i = 0; i < key.length; i++) {
hash += key.charCodeAt(i);
}
return hash % tableSize;
}
Collision resolution is required when two keys are hashed to the same index. Separate chaining handles collisions by using linked lists to store multiple key-value pairs at the same index. Open addressing resolves collisions by finding alternative positions within the table. For example, the following code implements open addressing with linear probing:
class HashTable {
constructor(size = 16) {
this.size = size;
this.table = new Array(size).fill(null);
}
set(key, value) {
let index = this.hash(key);
while (this.table[index] !== null && this.table[index].key !== key) {
index = (index + 1) % this.size;
}
this.table[index] = { key, value };
}
get(key) {
let index = this.hash(key);
while (this.table[index] !== null && this.table[index].key !== key) {
index = (index + 1) % this.size;
}
return this.table[index] !== null ? this.table[index].value : null;
}
hash(key) {
let hash = 0;
for (let i = 0; i < key.length; i++) {
hash += key.charCodeAt(i);
}
return hash % this.size;
}
}
Separate chaining allows for flexibility but may have higher memory overhead, while open addressing minimizes memory usage but can lead to more collisions.
Benefits of Hash Tables
Efficiency advantages of hash tables over other data structures for key-value storage
Hash tables offer several efficiency advantages compared to other data structures when it comes to storing and retrieving key-value pairs.
Constant-time average-case complexity: One of the primary benefits of hash tables is their ability to provide constant-time average-case complexity for operations such as insertion, retrieval, and deletion. This means that regardless of the number of elements stored in the hash table, the time required to perform these operations remains relatively constant. The use of hash functions and direct indexing allows for efficient access to the desired values.
Fast retrieval: Hash tables excel at retrieving values based on their keys. By employing a hash function, keys are mapped to specific indices within the underlying array, enabling direct access to the associated values. Unlike other data structures that may require iterating through the entire collection, hash tables allow for rapid and efficient retrieval.
Analysis of time complexity for common operations such as insertion, retrieval, and deletion
Insertion: In well-implemented hash tables, the average-case time complexity for insertion is O(1), indicating constant time. When inserting a key-value pair, the hash function calculates the index where the pair will be stored, and the value is placed at that index. If collisions occur, appropriate collision resolution techniques are employed to ensure efficient insertion.
Retrieval: Hash tables offer fast retrieval with an average-case time complexity of O(1). Using the hash function, the index corresponding to the key is determined, allowing for direct access to the desired value. This direct mapping eliminates the need for searching or iterating through the entire data structure, resulting in swift retrieval.
Deletion: Similar to insertion and retrieval, deletion in hash tables also has an average-case time complexity of O(1). The hash function is used to identify the index of the key-value pair, and the value at that index is efficiently removed. However, if collisions exist, appropriate collision resolution techniques are applied to ensure accurate deletion.
Real-world applications and use cases where hash tables excel
Hash tables find widespread use in various real-world applications due to their efficiency in storing and retrieving key-value pairs.
Databases: Hash tables are commonly employed in databases for indexing purposes. They enable rapid data retrieval based on primary keys, significantly enhancing query performance and overall database efficiency.
Caching Systems: Hash tables are extensively used in caching systems to store frequently accessed data. Due to their fast lookup and retrieval capabilities, hash tables enhance the overall performance of applications and systems reliant on caching mechanisms.
Symbol Tables in Programming Languages: Hash tables serve as symbol tables in programming languages to store variable names and their corresponding values. This allows for efficient name lookup during program execution, contributing to the smooth functioning of programming language interpreters and compilers.
In JavaScript, you can leverage the built-in Map
object to implement hash table functionality. Here's an example:
// Creating a hash table using Map
const hashTable = new Map();
// Inserting key-value pairs
hashTable.set('key1', 'value1');
hashTable.set('key2', 'value2');
// Retrieving values based on keys
const value1 = hashTable.get('key1'); // returns 'value1'
const value2 = hashTable.get('key2'); // returns 'value2'
// Deleting a key-value pair
hashTable.delete('key1');
// Checking if a key exists in the hash table
const hasKey = hashTable.has('key1'); // returns false
The Map
object in JavaScript provides efficient key-value storage and retrieval, making it suitable for implementing hash tables.
Implementing and Optimizing Hash Tables
Step-by-step guide on implementing a hash table from scratch:
To implement a hash table from scratch, follow these steps:
Design the underlying data structure: Begin by outlining the data structure that will serve as the foundation for the hash table. A popular choice is an array of linked lists, where each array index represents a bucket capable of holding multiple key-value pairs.
Create the HashTable class: Construct a HashTable class that encapsulates the functionalities of the hash table. This class should include methods for inserting, retrieving, and deleting key-value pairs.
Implement the hash function: Develop a hash function that accepts a key as input and produces an index within the array's range. The goal is to create a hash function that evenly distributes keys across the array, minimizing collisions. For instance, in JavaScript, you can employ the following hash function:
hash(key) {
let hashValue = 0;
for (let i = 0; i < key.length; i++) {
hashValue += key.charCodeAt(i);
}
return hashValue % this.buckets;
}
Handle collisions: When two different keys produce the same hash value (a collision), you need to handle it appropriately. One common method is separate chaining, where each bucket contains a linked list of key-value pairs. Alternatively, you can use open-addressing techniques like linear probing or quadratic probing.
Insertion: Implement the insert method, which takes a key-value pair as input. Apply the hash function to the key to determine the bucket index, then add the pair to the corresponding linked list or find the next available spot in the case of open addressing.
insert(key, value) {
const index = this.hash(key);
if (!this.table[index]) {
this.table[index] = [];
}
this.table[index].push({ key, value });
}
- Retrieval: Implement the get method, which takes a key and returns the associated value. Apply the hash function to find the bucket index and search the linked list or probe through the array until you find the matching key.
get(key) {
const index = this.hash(key);
if (!this.table[index]) {
return undefined;
}
for (const pair of this.table[index]) {
if (pair.key === key) {
return pair.value;
}
}
return undefined;
}
- Deletion: Implement the delete method, which takes a key and removes the corresponding key-value pair from the hash table. Apply the hash function to find the bucket index and traverse the linked list or probe through the array to locate the key and remove it.
delete(key) {
const index = this.hash(key);
if (!this.table[index]) {
return;
}
for (let i = 0; i < this.table[index].length; i++) {
if (this.table[index][i].key === key) {
this.table[index].splice(i, 1);
return;
}
}
}
Optimization techniques for hash table performance:
To optimize the performance of hash tables, consider the following techniques:
Choosing an appropriate load factor: The load factor represents the ratio of the number of key-value pairs to the total number of buckets. A higher load factor reduces memory usage but increases the likelihood of collisions. Find the right balance based on your specific use case.
Rehashing: Rehashing involves resizing the hash table and redistributing the key-value pairs when the load factor exceeds a certain threshold. This helps maintain a low collision rate and keeps the hash table efficient.
Advanced Concepts and Variations
Introduction to advanced hash table concepts, such as dynamic resizing and load factors
When it comes to hash tables, there are advanced concepts that can significantly enhance their functionality and performance. Let's explore two of these concepts: dynamic resizing and load factors.
Dynamic resizing: One limitation of hash tables is their fixed size, determined during initialization. However, as the number of key-value pairs grows or shrinks, this fixed size may no longer be optimal. Dynamic resizing addresses this issue by allowing the hash table to grow or shrink based on the number of entries it stores. By periodically checking the load factor (the ratio of filled slots to the total number of slots), the hash table can decide whether to resize itself. This ensures a balanced load factor, minimizing collisions and maximizing efficiency.
Load factors: Load factors play a crucial role in hash table performance. They determine how full the hash table can become before triggering a resize operation. A load factor of 1.0 means the hash table is full, while a load factor of 0.5 means it's only half full. Selecting an appropriate load factor is essential because it affects both memory usage and performance. A higher load factor results in more memory-efficient hash tables but increases the likelihood of collisions. Conversely, a lower load factor reduces collisions but consumes more memory. Finding the right balance depends on your specific use case.
Overview of variations of hash tables
Hash tables come in various flavors, each designed to address specific requirements and challenges. Let's explore two popular variations: perfect hashing and cuckoo hashing.
Perfect hashing: In a traditional hash table, collisions occur when two different keys map to the same hash value, requiring collision resolution techniques. Perfect hashing eliminates collisions entirely by constructing a hash function that guarantees unique hash values for each key in the table. This approach achieves constant-time operations without the need for collision resolution. However, constructing perfect hash functions can be computationally expensive and may require knowing all the possible keys in advance.
Cuckoo hashing: Cuckoo hashing is an alternative approach that utilizes multiple hash functions and multiple arrays or buckets. When a collision occurs during insertion, cuckoo hashing employs the alternate hash functions to find an empty slot in the other arrays. If necessary, it displaces the existing key-value pairs in a "cuckoo-like" manner until an empty spot is found. Cuckoo hashing provides efficient worst-case time complexity for lookup and insertion operations, making it a compelling choice for scenarios where low-latency operations are critical.
Exploration of modern developments in hash table research and innovations:
Hash tables continue to be an active area of research, leading to modern developments and innovations that further enhance their capabilities. Here are a few notable advancements:
Concurrent hash tables: With the rise of multi-threaded and parallel computing, concurrent hash tables have emerged to handle simultaneous access from multiple threads or processes. These hash tables incorporate synchronization mechanisms, such as locks or atomic operations, to ensure thread safety and maintain data integrity during concurrent operations.
Cache-conscious hash tables: CPU cache hierarchy plays a vital role in system performance, and cache-conscious hash tables aim to optimize memory access patterns accordingly. By organizing the hash table in a cache-friendly manner, such as using linear probing or open addressing, cache-conscious hash tables reduce cache misses and exploit the CPU cache hierarchy effectively, leading to improved overall performance.
GPU-accelerated hash tables: Graphics Processing Units (GPUs) have gained popularity not only for graphics-intensive tasks but also for general-purpose computing. Researchers have explored leveraging the massive parallelism offered by GPUs to accelerate hash table operations. GPU-accelerated hash tables can deliver significant speedups.
Subscribe to my newsletter
Read articles from Abi Farhan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
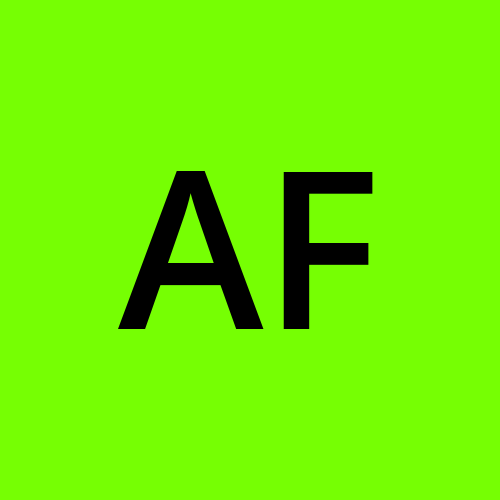
Abi Farhan
Abi Farhan
Working professionally with high passion and curiosity about Entrepreneurship, Tech, and Coffee. I am willing to work hard, learn faster, and extremely fast adaptation to new tech stacks. I have more 3 years of experience as a Software Engineer in the Industry world and academic world, I believe with my experience combined with my abilities as an Entrepreneur and Software Engineer with a deep understanding of data structure and algorithms also as strong skills for problem-solving would make me a valuable asset too much technology-oriented business seeking the talent with always hungry for knowledge and always eager to learn new things. For now, I work as Software Engineer in Aleph-Labs which develop the MyXL Ultimate app also I develop my own business Gayo Coffee Engaged in B2B and B2C. I am also Bilingual Communicator (English, Indonesia) with experience public speaker and leadership. I also experiece management project using Jira and Notion, also strong skills for teamwork including deep knowledge about Version Control System like Git.