Object in JavaScript.

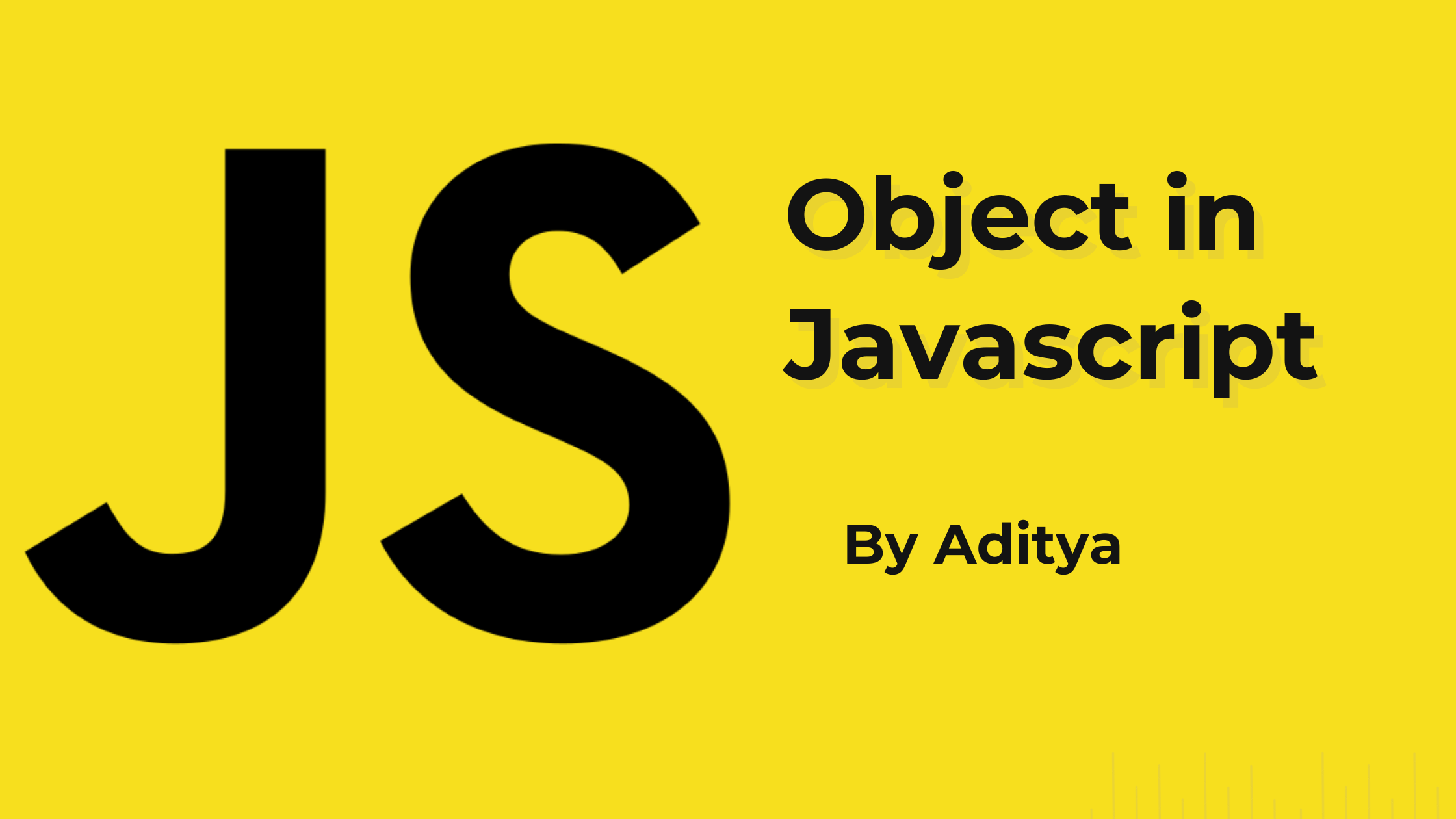
What is an object in javascript?
The object is a datatype that allows you to store and organize data in key-value pairs. It is similar to a real-life object. which has properties that describe its characteristics.
To create an object in javascript you can use {} curly braces.
let's create an object.
let car = {
name: "Mahindra XUV500"
length: 4cm,
price: $5000,
companyName: "Mahindra"
}
consol.log(car) // We get all the property of object.
// access the property of object
consol.log(car.length) // result 4cm
consol.log(car.name) // Mahindra xub500
consol.log(car.companyName) // Mahindra
consol.log(car.price) // $5000
In this example, the name of the object is car and the property of the object is name,
length,
compamyName,
price
and the name of the car and the name of the company the length of the car and the price of the car are property value.
let's create another object.
let person = {
firstName: "Raj",
lastName: "Pandey",
fullName: "Raj Pandey",
gender: "male",
age: 25,
city: "Delhi"
}
console.log(person) // We get object person and all property of person.
//We can access the property of object like this
console.log(person.firstName); // result "Raj"
console.log(person.lastName); // result "Pandey"
console.log(person.fullName); // result "Raj Pandey"
console.log(person.gender); // result "male"
console.log(person.age); // result 25
console.log(person.city); // result "Delhi"
Let's see another example of an object.
let person = {
name: 'Aditya Singh',
age: 30,
address: {
street: '123 Main',
city: 'Lucknow',
state: 'UP',
zip: '110027'
}
};
In this example, person
is an object that has three properties: name
, age
, and address
. The address
property is itself an object that has four properties. street, city,
state
, zip.
We can add a property to an object using dot notation: In this example, we add the address
property to a person
object using dot notation.
let person = {
name: 'John',
age: 30
};
person.address = '123 Main St';
console.log(person); // Outputs: { name: 'John', age: 30, address: '123 Main St' }
We can also add a property to an object using bracket [] notation.. let's see the example.
let person = {
name: 'John',
age: 30
};
person['address'] = '123 Main St';
console.log(person); // Outputs: { name: 'John', age: 30, address: '123 Main St' }
To remove a property from an object in JavaScript, you can use the delete
operator. let's see the example.
let person = {
name: 'John',
age: 30,
address: '123 Main St'
};
delete person.address;
console.log(person); // Outputs: { name: 'John', age: 30 }
In this example, we use the delete
the operator to remove the address
property from the person
object.
Thank You
Subscribe to my newsletter
Read articles from Aditya Pandey directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
