Function in Javascript.

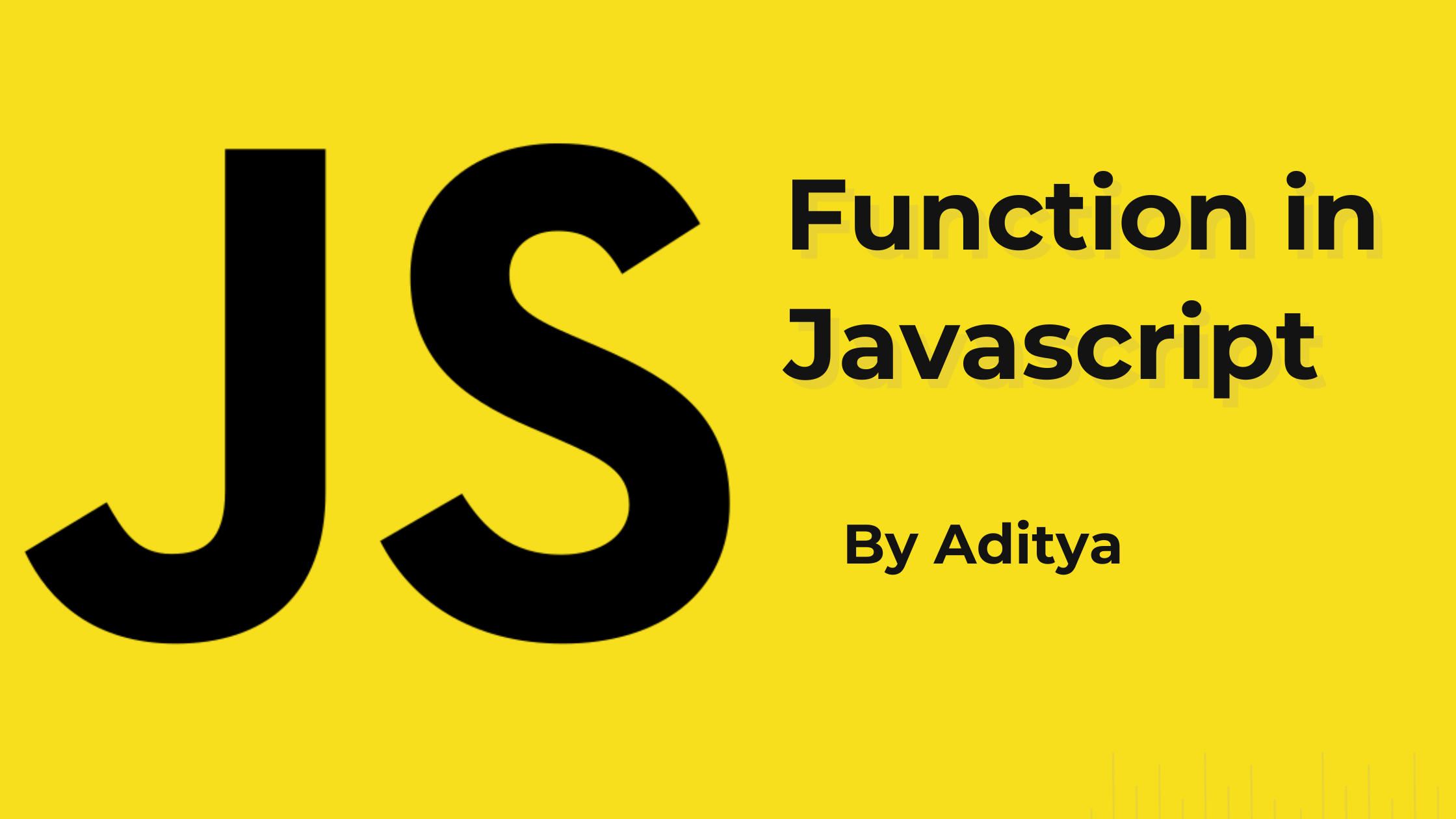
The function is one of the most important tools in JavaScript language.
A function is a reusable block of code that can perform a specific task or it is like a recipe that tells the computer. How to do something.
A function is just a block of code that you can call by name. and it may or may not take input or (arguments) and may or may not return a value.
Syntax:
function functionName(parameters) {
// code to be executed
}
Let's break down the code:
function
is the keyword used to define a function in JavaScript.functionName
is the name you choose for your function. choose the very wisely, such asmyFunction
,calculate
, oraddNumbers
.parameters
are optional inputs that the function can accept. These are specified inside the parentheses, separated by commas. If the function doesn't take any parameters, you can leave the parentheses empty.Inside the function body, you write the code that will be executed when the function is called.
You can call the function by the name of the function followed by parentheses.
Example:
// we want to print the Hello
function sayHello(){
console.log("Hello");
}
// call the function
sayHello(); // result Hello
In this example, we write the function using the function
keyword and sayHello
is the Name of the function and we won't print Hello
in the console.log
after that we call the function using the function name. and We get the result Hello.
- A function can also take input which allows us to write more generic and reusable code.
Example:
function greet(name){
console.log(`Hello, ${name}!`)
}
greet("Aditya") // result Hello Aditya
greet("Anjali") // result Hello Anjali
- A function can also return value. which allows them to produce output that can be used in another part of your program.
Example:
function square(x){
return x * x;
}
let result = square(5);
console.log(result); // result 25
Function name square
takes a single argument called x
. and we create a variable result
for storing the function value then we print the value.
- A function can also be assigned to variables which allows us to pass them around and use them as arguments for other functions.
Example:
let add = function(x, y){
return = x + y;
}
let result = add(3, 4) // result is now 7
console.log(result) // log 7
function expression that takes two arguments and return their sum. we assign this function expression to variable call add then we call the function by using the variable name followed by parentheses
Arrow function
An arrow function in JavaScript is a shorthand syntax for defining a function. It was introduced in ECMAScript 6 (ES6) and provides a more concise way to define functions compared to the traditional function syntax.
Syntax of an arrow function.
(parameters) => {
// function body
}
parameters
are the input arguments to the function, enclosed in parentheses.=>
is the arrow operator, which separates the parameters from the function body.{}
is an optional block of code that contains the function body. If the function has only one statement in the body, the braces can be omitted.
Example of a traditional function and its equivalent arrow function:
Traditional Function:
function add(a, b) {
return a + b;
}
Arrow Function:
const add = (a, b) => a + b;
Arrow functions have a few other notable differences compared to traditional functions. For example:
Arrow functions do not have their own value. Instead,
this
refers to the enclosing lexical context (i.e., the function that contains the arrow function).Arrow functions cannot be used as constructors and do not have a
prototype
property.Arrow functions cannot be named, and they are always anonymous functions.
Arrow functions provide a simpler and more concise way to define functions in JavaScript.So I recommend that use the arrow function.
Let's see more examples of arrow functions.
const add = (a, b) => {
return a + b;
}
let result = add(2, 3);
console.log(result); // Outputs: 5
In this example, add
is an arrow function that takes two parameters a
and b
and returns their sum. The result
variable is assigned the value returned by the add
function when called with arguments 2
and 3
.
Arrow functions have a few key features:
Concise syntax: Arrow functions have a shorter syntax than traditional functions, making them quicker and easier to write.
Implicit return: If the function body contains a single expression, the return statement is implicit, and the expression is automatically returned.
const square = (n) => n * n;
console.log(square(2)); // Outputs: 4
- Lexical
this
: In arrow functions, thethis
keyword refers to the surrounding context where the function is defined, rather than the context where it is called.
let person = {
name: 'John',
age: 30,
greet: function() {
setTimeout(() => {
console.log(`Hello, my name is ${this.name} and I'm ${this.age} years old`);
}, 1000);
}
};
person.greet(); // Outputs: "Hello, my name is John and I'm 30 years old" after a 1-second delay
In this example, the arrow function used as the argument to setTimeout
has access to the person
object's name
and age
properties, even though it is executed in a different context.
Arrow functions are a powerful tool in JavaScript, and their concise syntax and lexical this
makes them particularly useful in modern web development.
Thank You
Subscribe to my newsletter
Read articles from Aditya Pandey directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
