What is React Concurrent Mode?
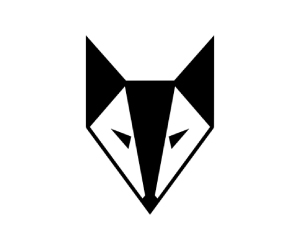
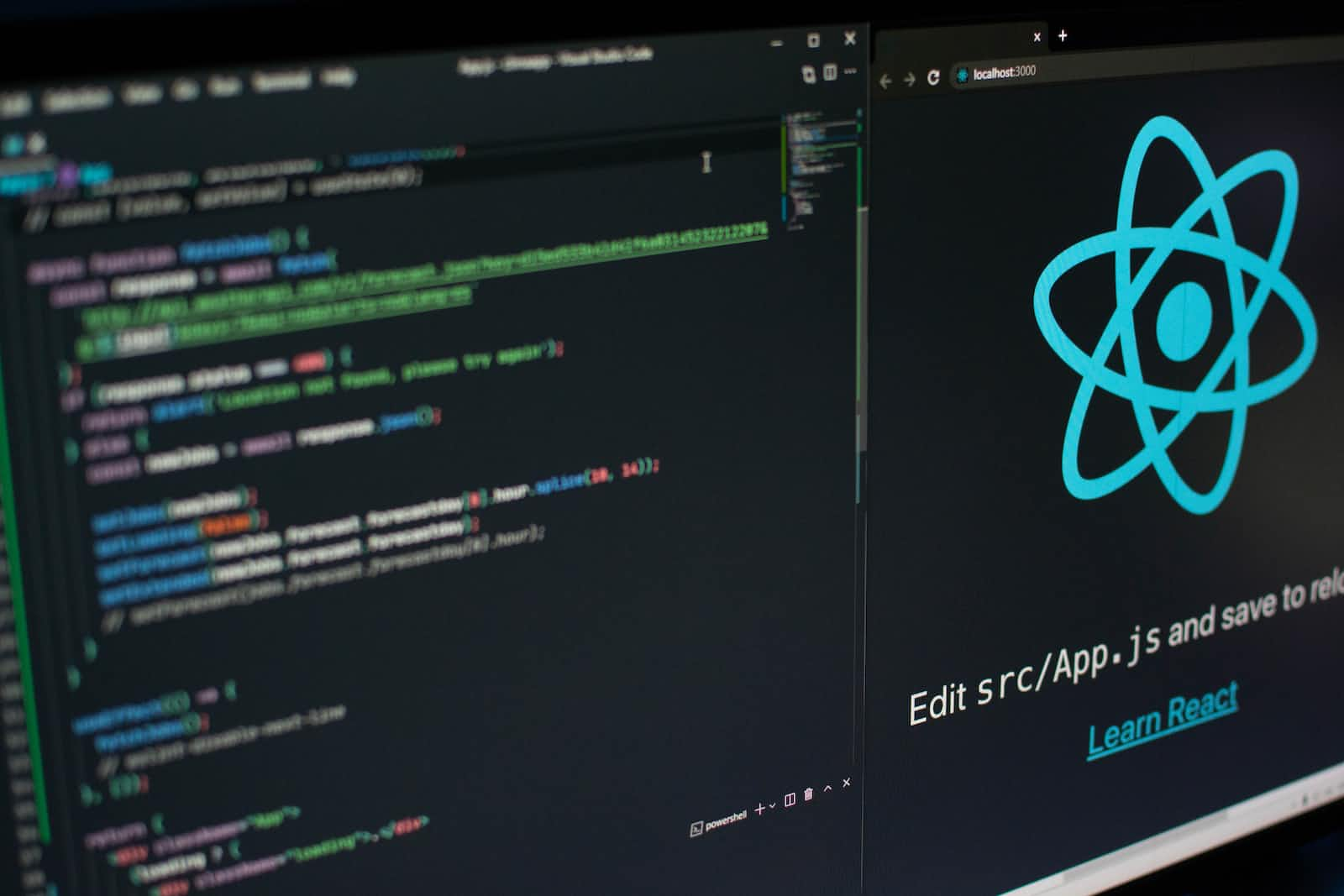
React Concurrent Mode is a feature introduced in React 18 that aims to improve the user experience by making applications more responsive and performant. It allows React to work on multiple tasks concurrently, enabling better scheduling and prioritization of rendering and updating processes.
Traditionally, React operates in a single-threaded manner, where it performs rendering and updates in a synchronous and sequential manner. This means that if there is a long-running task or a large component tree to render, it can block other updates and result in a less responsive user interface.
With Concurrent Mode, React introduces a new rendering strategy that breaks down work into smaller units called "tasks" or "priorities". It enables React to pause, resume, and prioritize tasks based on their importance, allowing for more fine-grained control over rendering and responsiveness.
Concurrent Mode brings several benefits to React applications:
Improved responsiveness: By breaking down work into smaller units, Concurrent Mode allows the browser to handle other tasks and user interactions alongside React's rendering and updating processes. This leads to a more responsive user interface, as the application can respond to user input and keep the interface interactive even during heavy computations.
Smoother user experience: With Concurrent Mode, React can prioritize rendering and updates based on the urgency of the task. It ensures that high-priority updates, such as user interactions or animations, are processed quickly, while lower-priority updates are processed in a more relaxed manner. This helps prevent UI jank and provides a smoother user experience.
Incremental rendering: Concurrent Mode introduces the concept of incremental rendering, where React can render and update components in multiple passes. It prioritizes rendering of visible and interactive parts of the UI first, allowing the user to see and interact with the application faster. Then, it gradually fills in the rest of the UI, providing a perceived performance improvement.
Graceful loading and error handling: Concurrent Mode enables React to handle loading states and errors more gracefully. It allows developers to define fallback UIs or loading indicators that are shown to users while the main content is being loaded or in case of errors. This helps create a better user experience by providing feedback and visual cues during asynchronous operations.
How does React Concurrent Mode work?
React Concurrent Mode works by using a new feature called task scheduling. Task scheduling allows React to run multiple tasks at the same time, each in its own task queue. This means that React can continue rendering the UI even if it is waiting for a task to finish.
There are two types of tasks in React Concurrent Mode: urgent tasks and non-urgent tasks. Urgent tasks are tasks that need to be completed immediately, such as responding to user input. Non-urgent tasks are tasks that can be completed at a later time, such as fetching data from the server.
By default, React will only run urgent tasks. However, you can use the useDeferredValue hook to mark a task as non-urgent. This will allow React to run the task at a later time, even if it is currently rendering the UI.
How to use React Concurrent Mode
To use React Concurrent Mode, you will need to upgrade your React and ReactDOM packages to React 18. You will also need to use the useDeferredValue hook to mark tasks as non-urgent.
For example, the following code shows how to use the useDeferredValue hook to mark a task as non-urgent:
const [data, setData] = useState(null);
const fetchData = async () => { const response = await fetch("https://example.com/data"); const data = await response.json(); setData(data); };
const useDeferredData = () => { const [isLoading, setIsLoading] = useState(true); const [data, setData] = useDeferredValue(null);
useEffect(() => { fetchData().then(() => { setIsLoading(false); setData(data); }); }, []);
return { isLoading, data, }; };
The above code will fetch data from the server and then render it to the UI. However, if the user starts interacting with the UI while the data is still loading, the UI will not freeze. Instead, the UI will continue to be responsive and the data will be rendered as soon as it is available.
It's important to note that while Concurrent Mode brings significant performance improvements, it also requires React developers to write their components in a way that is compatible with the new rendering model. Certain patterns and best practices may need to be followed to ensure optimal performance and behavior in Concurrent Mode.
React Concurrent Mode is a powerful new feature that can help you make your React applications more responsive. By using Concurrent Mode, you can improve the overall responsiveness of your application, especially on devices with slower processors.
Subscribe to my newsletter
Read articles from Nickelfox directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
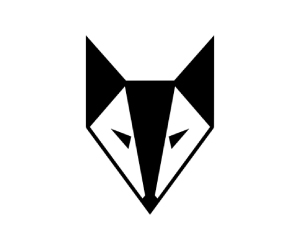
Nickelfox
Nickelfox
Nickelfox is one of the leading design & development studios in app development space. We define the digital experience of Startups & Fortune 500 firms through robust, scalable, customized software solutions. We offer Product Consultancy Services, MVP Services, Staff Augmentation Services, App and Web Design & Development Services.