Using LMS the fastest way
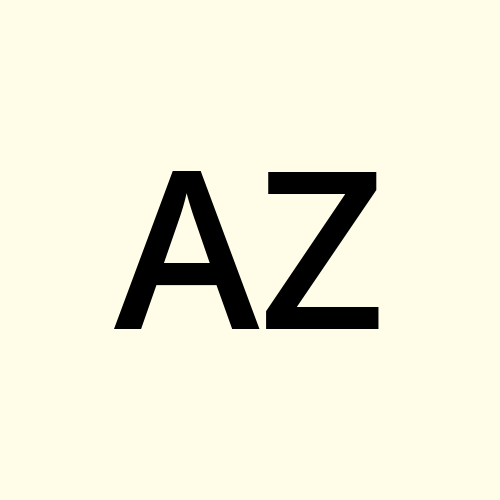
Lightning Message Service (LMS) is a robust platform event-driven messaging service in Salesforce that enables real-time communication between different components within the ecosystem.
It provides a simple and efficient way for components to communicate with each other, without having to rely on server-side controllers or complex APIs. With LMS, components can send and receive messages to and from one another, regardless of whether they are on the same Lightning page, or different pages.
This makes it easier for developers to build modular and scalable Lightning components, and create richer, more dynamic user experiences for their end-users. LMS is a key feature of the Lightning Experience platform, and it's an important tool for any Salesforce developer looking to build modern, responsive, and highly interactive applications.
Code space
Deploy Message Channel
First, we'll create a channel.
We don't have any UI in Salesforce for creating a channel.
Navigate to force-app/main/default/
and then create a folder named messageChannels(if not present, if present then just open it)
create a file in the messageChannels folder named
ABeautifulNameForChannel.messageChannel-meta.xml
<?xml version="1.0" encoding="UTF-8"?>
<LightningMessageChannel xmlns="http://soap.sforce.com/2006/04/metadata">
<masterLabel>ABeautifulNameForChannel</masterLabel>
<isExposed>true</isExposed>
<description>Description about the channel</description>
<lightningMessageFields>
<fieldName>fieldName1</fieldName>
<description>description aboy the field</description>
</lightningMessageFields>
</LightningMessageChannel>
Open this in VS Code and right-click to deploy it to the org.
How VF Page, LWC, and Aura will PUBLISH on the channel
Publishing from VF
// keep this inside the script tag of the vf page
var channel = "{!$MessageChannel.ABeautifulNameForChannel__c}";
const message = { fieldName1: "some value" };
sforce.one.publish(channel, message);
You can have this code onload of a VF page or on click of action etc.
Publishing from AURA
<!-- keep this in the component file after the attribute declation-->
<lightning:messageChannel
type="ABeautifulNameForChannel__c"
aura:id="MessageChannel"
/>
Have this in the component
// publish the message on any action you want
var message = {
fieldName1: "some value"
};
component.find("MessageChannel").publish(message);
Have this in the javascript controller
Publishing from LWC
// import these on top of the js file
import Channel from '@salesforce/messageChannel/ABeautifulNameForChannel__c';
import {publish, MessageContext} from 'lightning/messageService'
Imports
// setup this wire call anywhere inside the export section of the js file
@wire(MessageContext)
messageContext;
Have this wire method set the context
// invoke it whenever you want to publish a message maybe from a button handler
let message = {fieldName1: "some value"};
publish(this.messageContext, Channel, message);
Have this on any action to publish the message on the channel
How VF Page, LWC, and Aura will SUBSCRIBE to the channel
Subscribing from VF
// declaration maybe on the top of the script tag
var Channel = "{!$MessageChannel.ABeautifulNameForChannel__c}";
var subscription;
// keep it onload ideally but you can invoke it anytime you want
if (!subscription) {
subscription = sforce.one.subscribe(Channel, functionToHandleMessage);
}
// declare a handler that will handle the message from the channel
function functionToHandleMessage(message){
console.log("message : ", message);
// you can do anything with the message now
}
Subscribing from AURA
<!-- keep this in the component file after the attribute declation-->
<lightning:messageChannel
type="ABeautifulNameForChannel__c"
onMessage="{!c.handleMessage}"
/>
// keep this in controller
handleMessage: function(comp, event, helper) {
if (event != null && event.getParams() != null) {
var params = event.getParams();
console.log("message : ", JSON.stringify(params));
}
}
Subscribing from LWC
// import these on top of the js file
import Channel from '@salesforce/messageChannel/ABeautifulNameForChannel__c';
import { subscribe, MessageContext } from 'lightning/messageService';
// declare a variable for subscription
subscription = null;
// keep this anywhere inside the export section of the js file
@wire(MessageContext)
messageContext;
connectedCallback() {
this.handleSubscribe();
}
// method to handle subscription
handleSubscribe() {
if (this.subscription) {
return;
}
this.subscription = subscribe(this.messageContext, Channel, (message) => {
console.log("message : ", message);
});
}
Subscribe to my newsletter
Read articles from Akmal Ziyad directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
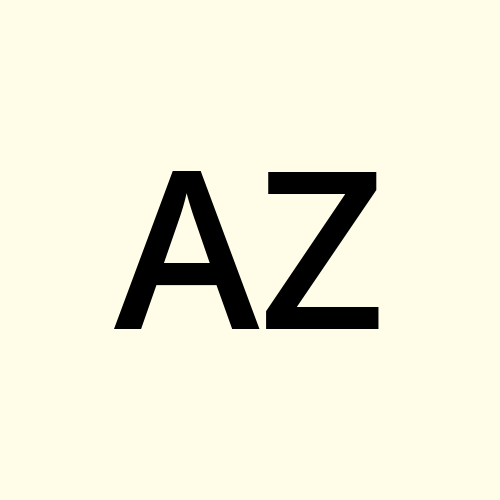