How to use forwardRef in react - easy explanation

Table of contents
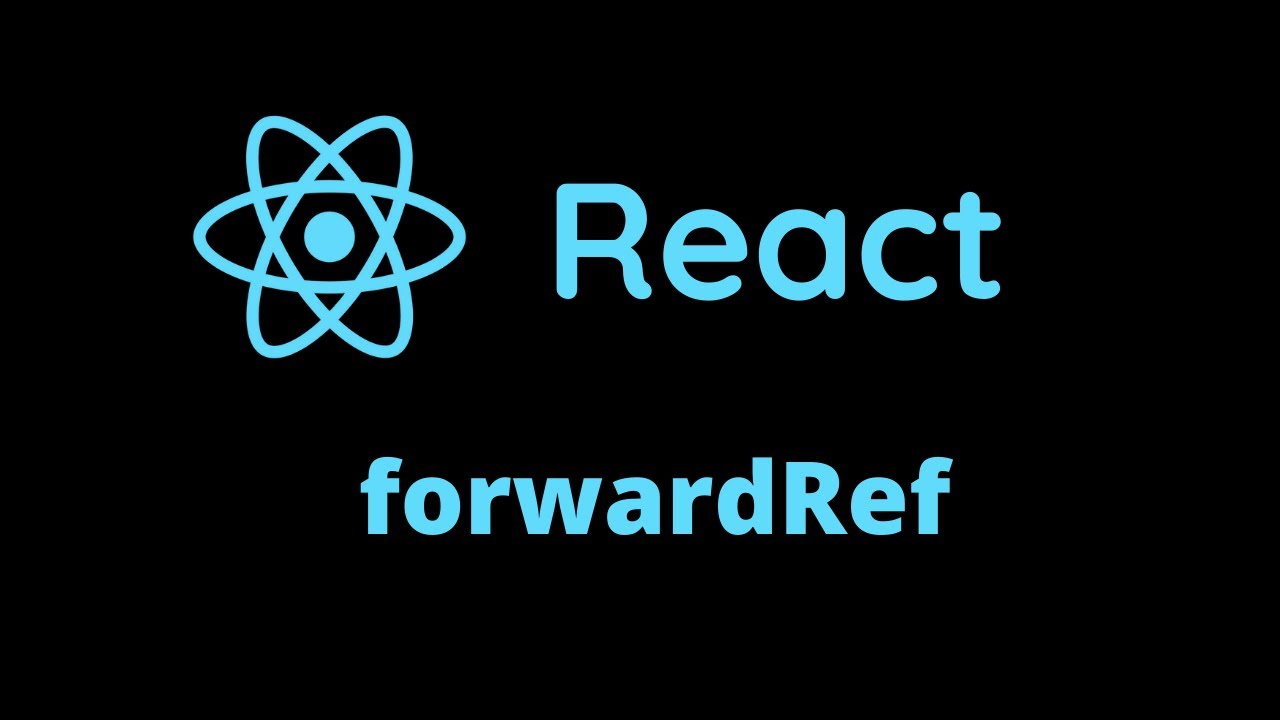
My Thoughts
After completing reading this post you will have no problem understanding the usage of forwardRef in react.
Generally, you use useRef
to refer to an HTML element in React. But what if you want to refer to an element in a child component? Passing a ref as a prop to the child won't work as React won't recognize it as a ref. This is where forwardRef
comes into play.
Step-by-Step Explanation
1. Child Component (Child.js)
First, create a child component that accepts a ref and forwards it to an internal element:
import { forwardRef } from "react";
// Define the Child component using forwardRef
const Child = forwardRef((props, ref) => {
return (
<div className="child">
<h1>This is a Child Component</h1>
<input ref={ref} type="text" /> {/* Assign the forwarded ref to the input element */}
</div>
);
});
export default Child;
forwardRef: This function allows the Child component to accept a ref from its parent and pass it to an internal element.
ref: The second argument to the function is the ref forwarded from the parent.
input ref: The forwarded ref is assigned to the
input
element.
2. Parent Component (App.js)
Next, create a parent component that uses useRef
to create a ref and passes it to the child component:
import React, { useRef } from "react";
import "./styles.css";
import Child from "./components/Child";
export default function App() {
const inputRef = useRef(null); // Create a ref using useRef
const handleFocus = () => {
inputRef.current.focus(); // Use the ref to focus the input element in Child
};
return (
<div className="App">
<h1>Hello Abeer</h1>
<h2>Start editing to see some magic happen!</h2>
<Child ref={inputRef} /> {/* Pass the ref to the Child component */}
<button onClick={handleFocus}>Focus Input</button> {/* Button to trigger the focus */}
</div>
);
}
useRef: This hook creates a mutable ref object.
inputRef: The created ref is assigned to the
input
element in the Child component.handleFocus: A function that focuses the input element using the ref.
<Child ref={inputRef} />: The ref is passed to the Child component.
How It Works
Ref Creation: In the
App
component,useRef
creates a ref (inputRef
).Forwarding Ref: The
Child
component, wrapped withforwardRef
, accepts this ref and forwards it to theinput
element.Using the Ref: When the button in the
App
component is clicked, thehandleFocus
function uses the ref to focus the input element inside theChild
component.
Key Points
Using
forwardRef
: Wrap the child component withforwardRef
to pass a ref from the parent.Ref Argument: The second argument of the
forwardRef
function is the ref.Accessing Child Ref: The parent can manipulate the child’s ref (e.g., focusing an input).
Now we are making an event happen in child component from parent’s handle function. Here is a thing to remember that you can receive only one ref in Child components
Congratulations, you now know how to use forwardRef
in React!
By the way, this is my first blog post.
Subscribe to my newsletter
Read articles from Abeer Abdul Ahad directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Abeer Abdul Ahad
Abeer Abdul Ahad
I am a Full stack developer. Currently focusing on Next.js and Backend.