State Management RTK.
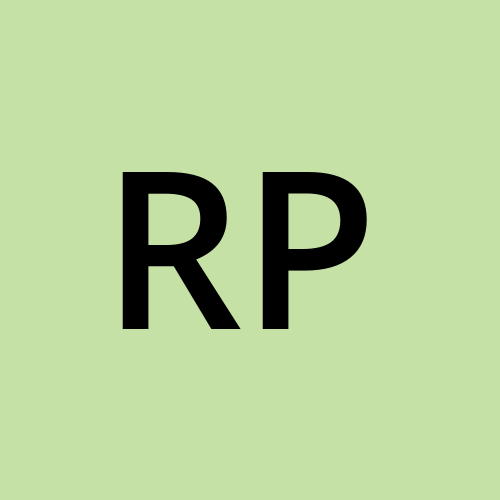
Here's a step-by-step tutorial on state management using Redux Toolkit (RTK) in a React application:
Step 1: Set up a new React project Create a new React project using Create React App or any other method you prefer. Open your project in your favorite code editor.
Step 2: Install Redux Toolkit In your terminal, navigate to your project directory and run the following command to install Redux Toolkit:
bashCopy codenpm install @reduxjs/toolkit
Step 3: Create a Redux slice Create a new file called counterSlice.js
in a folder called features
(you can choose any name) in the root of your project. This file will contain the logic for managing the counter state.
javascriptCopy code// features/counterSlice.js
import { createSlice } from '@reduxjs/toolkit';
const counterSlice = createSlice({
name: 'counter',
initialState: 0,
reducers: {
increment: (state) => state + 1,
decrement: (state) => state - 1,
},
});
export const { increment, decrement } = counterSlice.actions;
export default counterSlice.reducer;
Step 4: Create the Redux store Create a new file called store.js
in the root of your project. This file will configure and export the Redux store.
javascriptCopy code// store.js
import { configureStore } from '@reduxjs/toolkit';
import counterReducer from './features/counterSlice';
const store = configureStore({
reducer: {
counter: counterReducer,
},
});
export default store;
Step 5: Wrap your app with the Redux Provider In your index.js
file, import the Redux Provider component from react-redux
and wrap your app with it. Also, import the Redux store from store.js
and pass it as a prop to the Provider component.
javascriptCopy code// index.js
import React from 'react';
import ReactDOM from 'react-dom';
import { Provider } from 'react-redux';
import store from './store';
import App from './App';
ReactDOM.render(
<Provider store={store}>
<App />
</Provider>,
document.getElementById('root')
);
Step 6: Use the counter state in your component In your component file, import the necessary hooks and functions from Redux Toolkit to access the counter state and dispatch actions.
javascriptCopy code// Counter.js
import React from 'react';
import { useSelector, useDispatch } from 'react-redux';
import { increment, decrement } from './features/counterSlice';
const Counter = () => {
const counter = useSelector((state) => state.counter);
const dispatch = useDispatch();
return (
<div>
<h1>Counter: {counter}</h1>
<button onClick={() => dispatch(increment())}>Increment</button>
<button onClick={() => dispatch(decrement())}>Decrement</button>
</div>
);
};
export default Counter;
Step 7: Use the Counter component in your app In your App.js file or any other component where you want to use the counter, import the Counter component and use it in your JSX.
javascriptCopy code// App.js
import React from 'react';
import Counter from './Counter';
const App = () => {
return (
<div>
<h1>My App</h1>
<Counter />
</div>
);
};
export default App;
That's it! You've now implemented state management using Redux Toolkit in your React application. You can create additional slices and reducers following the same pattern to manage different parts of your application's state.
Remember to run your React app using `npm
Subscribe to my newsletter
Read articles from Rajmani Prasad directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
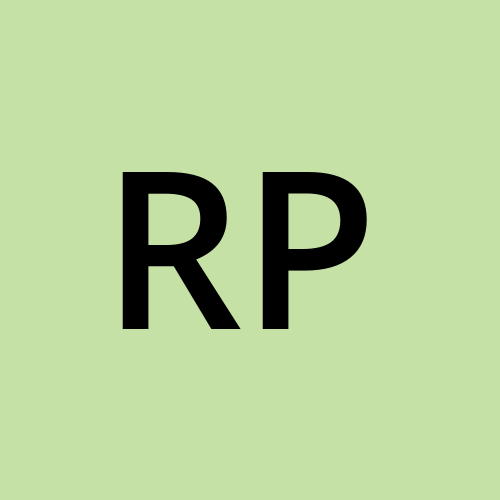
Rajmani Prasad
Rajmani Prasad
Front end developer with 4+ years of experience. Angular2+| React | AWS | HTML | CSS | JS