Java OOP (Object-Oriented Programming)
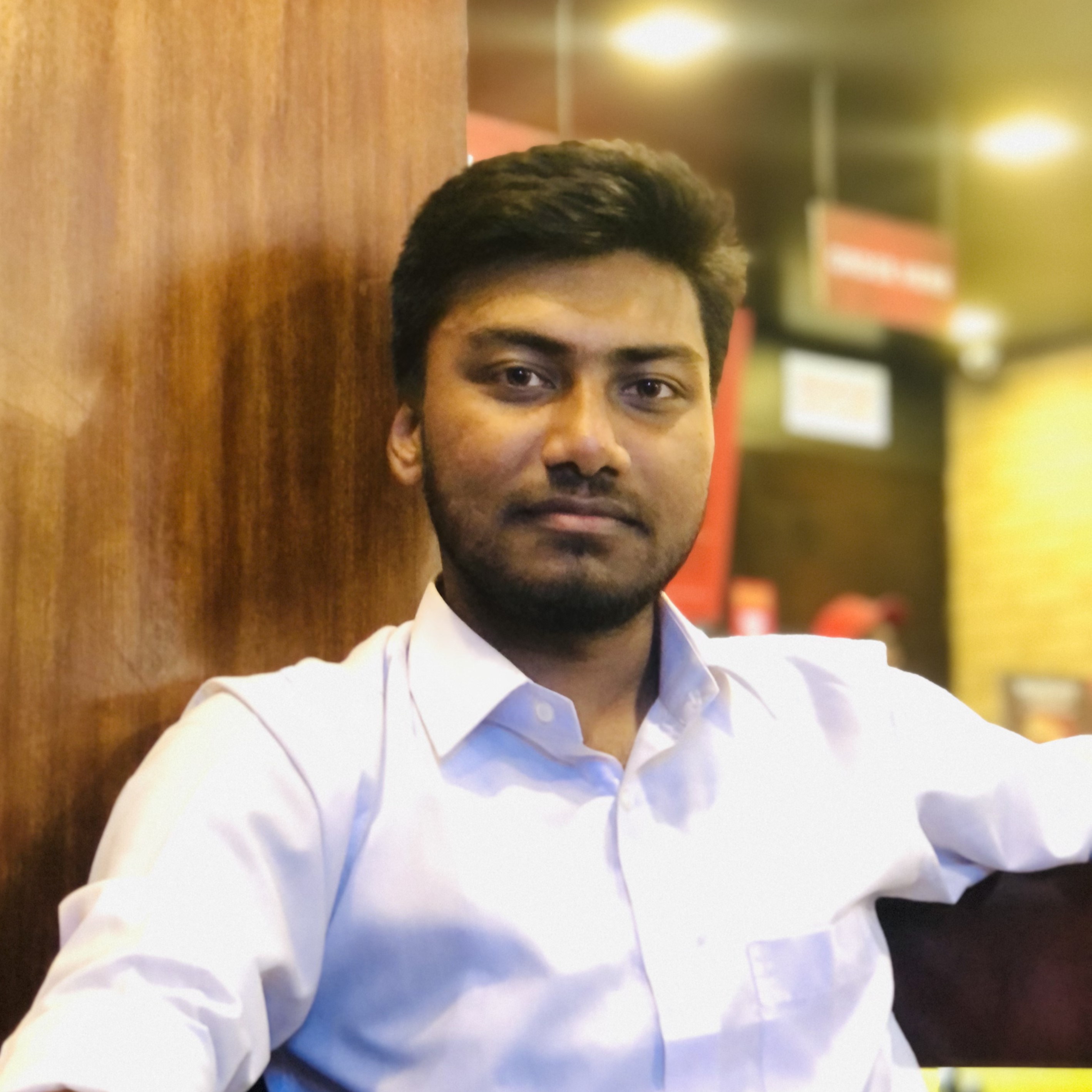
Table of contents
- Tools you need
- Java Documentation
- Book
- <mark>Things you should know</mark>
- Why Java?
- How Java works
- Java identifiers
- Data type in Java
- Class in JAVA
- Print Statement without Semicolon
- Modularization
- For Each loop
- Array in Java
- List VS ArrayList
- Equal method Override
- Object Class in JAVA
- Access Modifiers in JAVA
- Garbage Collection in JAVA
- Constructor
- Method VS Constructor
- Exception Handling (Try-Catch Finally Block)
- Method Overloading
- OOP
- Java Swing
- JavaFX
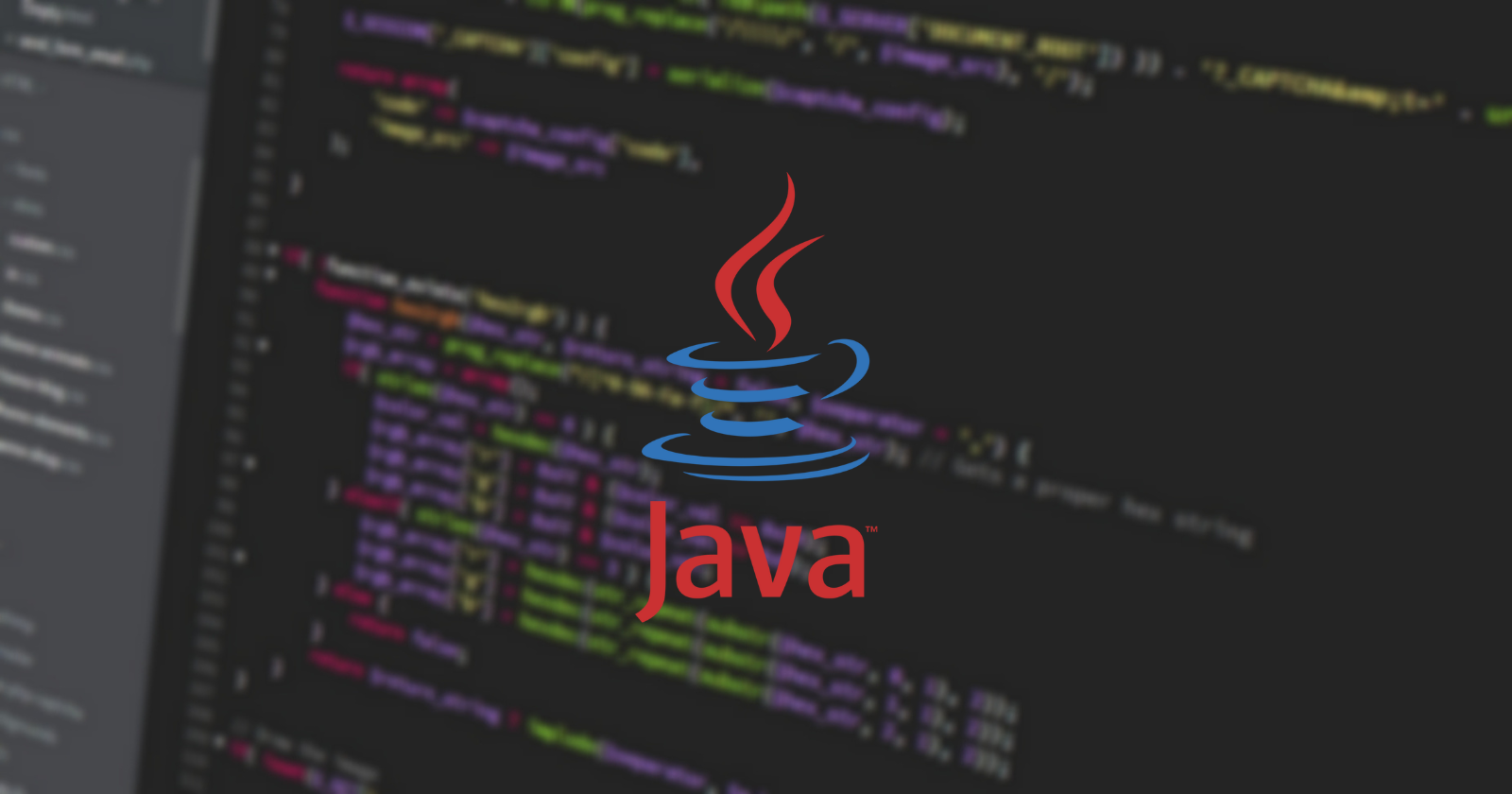
Java is a versatile and robust programming language widely used in the software development industry. It supports object-oriented programming (OOP) principles, allowing for modular and reusable code. With its rich library and extensive community support, Java is suitable for various domains such as web development, mobile app development, and enterprise software. It promotes platform independence through the Java Virtual Machine (JVM), enabling Java programs to run on different platforms. Its key features include strong type checking, automatic memory management, and exception handling. Java's popularity stems from its reliability, scalability, and wide range of applications.
Tools you need
Java Documentation
Book
জাভা প্রোগ্রামিং (দ্বিতীয় সংস্করণ) আ ন ম বজলুর রহমান
Head First Java
Things you should know
Why Java?
Learning Java can be beneficial for several reasons:
Versatility: Java is a versatile programming language widely used in various domains, such as web development, mobile app development (Android), enterprise software, and more.
Job opportunities: Java is one of the most in-demand programming languages in the job market. Learning Java can open up a wide range of career opportunities.
Popularity and community support: Java has a vast and active community of developers, which means there are numerous resources, libraries, and frameworks available to support your learning and development.
Object-oriented programming (OOP): Java is an object-oriented language, which helps you understand key programming concepts like classes, objects, inheritance, and polymorphism, which are widely used in many other languages.
Scalability and performance: Java is known for its scalability and performance. It's used in building robust and high-performance applications, making it suitable for both small projects and large-scale enterprise systems.
How Java works
Java identifiers
Identifiers are names that are helpful in uniquely recognizing different types of content or aspects that build up language.
Here are some rules for naming Java identifiers:
Identifiers must start with a letter.
Identifiers can contain letters, digits, and underscores.
Identifiers cannot contain spaces.
Identifiers cannot start with a digit.
Identifiers cannot be the same as any Java keywords.
Here are some examples: myVar, my_var, myVar123, $myVar, etc. But these are invalid Java identifiers: 123myVar, my var, myVar-123, class, etc.
Data type in Java
Data types in Java are used to define the values that can be stored in variables. There are two types of data types in Java: primitive data types and reference data types.
Primitive data types
Primitive data types are the basic data types in Java. They are used to store basic values, such as numbers, characters, and Boolean values. There are eight primitive data types in Java:
byte
short
int
long
float
double
boolean
char
Reference data types
Reference data types are used to store references to objects. Objects are created from classes, and they can contain data and methods. There are many different types of reference data types in Java, including:
String
Arrays
Classes
Interfaces
Class in JAVA
Everything we write in Java is under a class. In Java, a class is a blueprint for creating objects. It defines the properties and behaviors of an object. A class can contain Fields, Methods, Constructors, Blocks, Nested classes, Interfaces, etc.
To understand more about the class: Class & Object
Print Statement without Semicolon
int i = 0;
if(System.out.printf("Sanfoundary!! ") == null) {}
for(i = 1; i < 2; System.out.println("Hello World."))
{
i++;
}
Scan One char from input (Char Input)
scan*.next
().charAt(0);
Char to string
Modularization
These are only a few of the 98 platform modules. The diagram is a dependency graph. Each box indicates a module, and if a module has an arrow pointing to another module, it means that the module where the hand originates needs the module it is pointing at to perform its functions.
For Each loop
Array in Java
To define an array in Java, you need to specify the type of elements it will hold, followed by the array name and the size of the array (number of elements) in square brackets []. Here's the general syntax:
dataType[] arrayName = new dataType[arraySize];
To input values into the array, you can use a loop or assign values individually to each element. Here's an example of how you can input values into an array using a loop:
Scanner scanner = new Scanner(System.in);
int[] numbers = new int[5];
System.out.println("Enter 5 numbers:");
for (int i = 0; i < numbers.length; i++) {
numbers[i] = scanner.nextInt();
}
scanner.close();
To print the array, you can use a loop to iterate over the elements and print each one individually. Here's an example:
int[] numbers = {1, 2, 3, 4, 5};
System.out.println("Array elements:");
for (int i = 0; i < numbers.length; i++) {
System.out.println(numbers[i]);
}
List VS ArrayList
The List is an interface, and the ArrayList is a class of the Java Collection framework. The List creates a static array, and the ArrayList creates a dynamic array for storing the objects.
Equal method Override
Overriding the equals() method in Java is important because it allows us to define custom equality for objects. The default implementation of equals() compares object references, which may not reflect the desired equality criteria for certain classes. By overriding equals(), we can compare the internal state of objects and determine if they are equal based on our own criteria, providing more meaningful and accurate comparisons when needed.
Object Class in JAVA
In Java, the Object class is the root class for all other classes. It serves as the base class from which all other classes are derived. The Object class provides fundamental methods such as toString(), equals(), and hashCode(), which can be overridden by subclasses to customize their behavior. It also acts as a reference type for objects of any class, allowing them to be treated uniformly and enabling features like polymorphism in Java.
Access Modifiers in JAVA
Garbage Collection in JAVA
Java has no destructor because Java has Garbage Collection.
Constructor
Constructors in Java are special methods used to initialize objects of a class. They have the same name as the class and are called automatically when an object is created. Constructors can be used to set initial values for object attributes and perform any necessary setup tasks.
a special method responsible for initializing objects
Method VS Constructor
Exception Handling (Try-Catch Finally Block)
Method Overloading
Method overloading in Java allows the creation of multiple methods with the same name but different parameters within a class. It enables the flexibility of using the same method name for similar operations with varying input types or quantities. Method overloading is resolved at compile-time based on the arguments used during method invocation.
OOP
Encapsulation
Encapsulation in Java is the principle of bundling data and methods within a class, allowing for data hiding and abstraction. It ensures that the internal state of an object is protected and can only be accessed through defined methods, enhancing security and maintainability of the code.
Polymorphism
Polymorphism in Java refers to the ability of an object to take on many forms. It allows a single method or class to have different implementations, depending on the context in which it is used. Polymorphism enables code reusability, flexibility, and extensibility in object-oriented programming.
polymorphism in Java allows us to perform the same action in many different ways.
means more than one form
Inheritance
Inheritance in Java allows for the creation of new classes based on existing classes. It enables the reuse of code and the establishment of hierarchical relationships between classes, where the derived class inherits the properties and methods of the parent class. Inheritance promotes code reusability, modularity, and abstraction.
Abstraction
Abstraction is one of the fundamental concepts of object-oriented programming. It is a way of hiding the implementation details of a class and only exposing the essential features to the user. This makes the code more modular and reusable, and it also makes it easier to understand and maintain.
Abstract Class in Java
An abstract class in Java serves as a blueprint for other classes. It cannot be instantiated and may contain both abstract and non-abstract methods. It provides a way to define common methods and attributes that can be shared among multiple subclasses while enforcing implementation requirements.
Interface
An interface in Java is a contract that defines a set of methods that a class implementing the interface must implement. It allows for multiple inheritances and supports the concept of loose coupling. Interfaces are used to achieve abstraction and provide a way to achieve polymorphism in Java.
Java Swing
JavaFX
Practice Java:
CodingBat Java
Hacker RankSome Youtube Channels to learn JAVA
APNA College
Code with harry.
Abdul Bari
Zulkarnine Mahmud
Code with MOSH
NESO Accademy
Great learning
Telusko
Coding with John
Derek Banas
Useful Shortcuts for Intellij
Ctrl + SideArrows(→) To move Word by word
Ctrl + up and down key work as muse scroll
Alt+ SideArrows(→) To move another class
Ctrl + Shift + Side arrows to select word by word
Alt + Shift + UpDown Keys to move the line up or down
Ctrl + W to select code block by block
Shift + ctrl + enter to end line with a semicolon
Alt+ ctrl + enter to index code
ctrl + click to see the prebuild methods body
ctrl + / for comment
ctrl+ alt +L formatting lines
Shift + F10 Run code
Java interview questions you need to know: Link
Java Project Ideas
Bank Management Software.
Temperature Converter.
Electricity Billing System.
Supermarket Billing Software.
Memory Game.
Link Shortener.
Chatting Application.
Digital Clock.
Note: Some used pictures are taken form google and some websites.
Subscribe to my newsletter
Read articles from Tashin Parvez directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
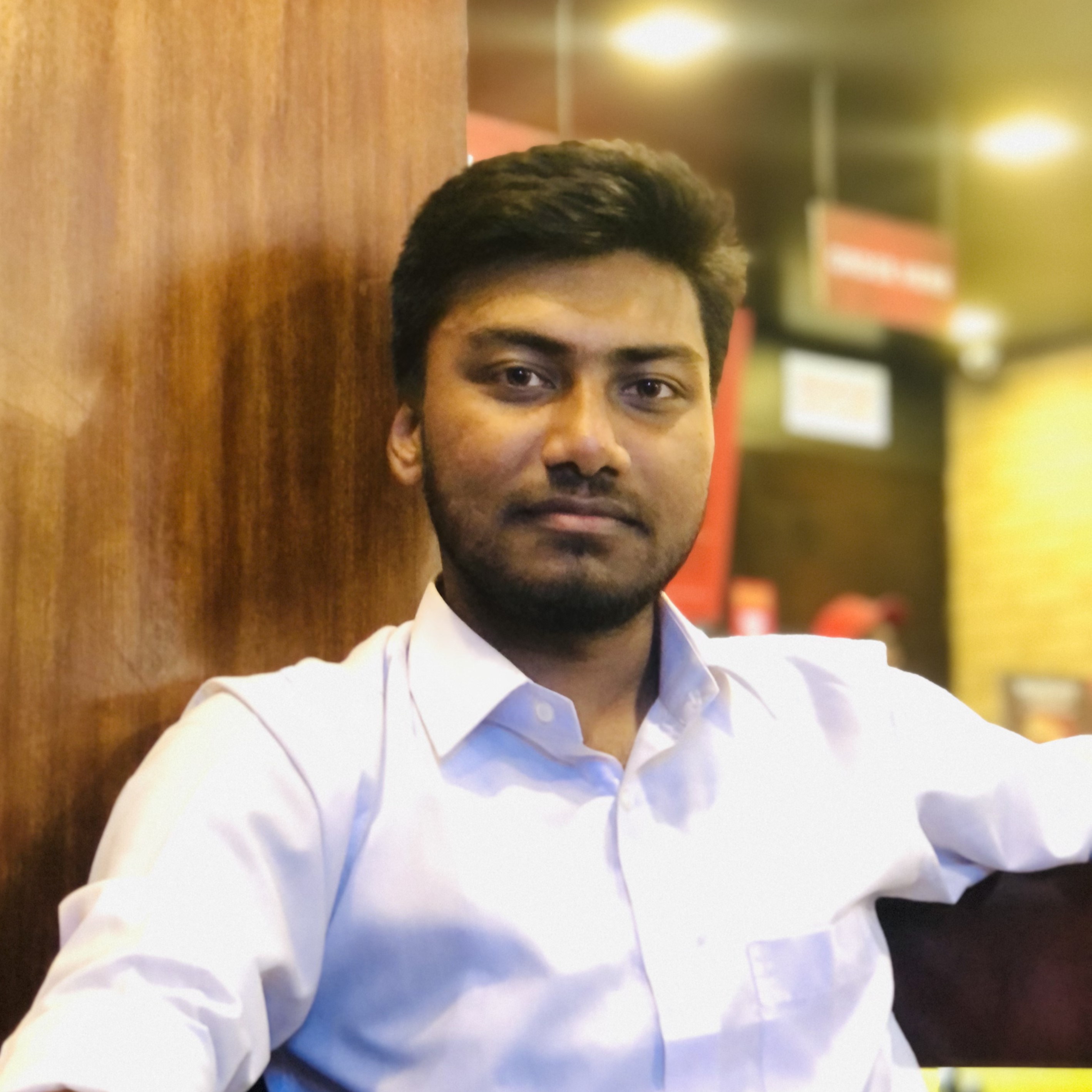
Tashin Parvez
Tashin Parvez
Hey there! I'm Tashin Parvez, currently pursuing my undergraduate degree in Computer Science and Engineering at UIU (United International University). Programming is not just a subject for me; it's a deep passion that drives me. I absolutely love diving into the world of code and technology, constantly seeking new challenges to push my limits. Competitive programming is my jam! I find myself thrilled by coding contests and challenges that test my problem-solving skills. There's something incredibly satisfying about unraveling complex problems and crafting elegant solutions using algorithms and data structures. I'm a huge movie buff, exploring different genres and narratives to transport myself to another world. From mind-bending science fiction to heartfelt dramas, I appreciate the art of storytelling and the power of cinema. Photography is another passion of mine. I love capturing moments and freezing them in time through the lens of my camera. It's amazing how a single photograph can convey so much emotion and tell a unique story. I enjoy experimenting with various techniques and playing with light, composition, and perspective to create visually captivating images. Of course, life isn't all about solitary pursuits. I believe in the importance of friendship and spending quality time with loved ones. Whether it's engaging in deep conversations or embarking on exciting adventures, I cherish the bonds I've formed and the memories we create together. Overall, I consider myself a well-rounded individual, eager to excel in both my academic pursuits and personal interests. I'm confident that my passion for programming will lead me to a successful and fulfilling career in the dynamic tech industry. That's a glimpse into who I am.