Objects in JavaScript

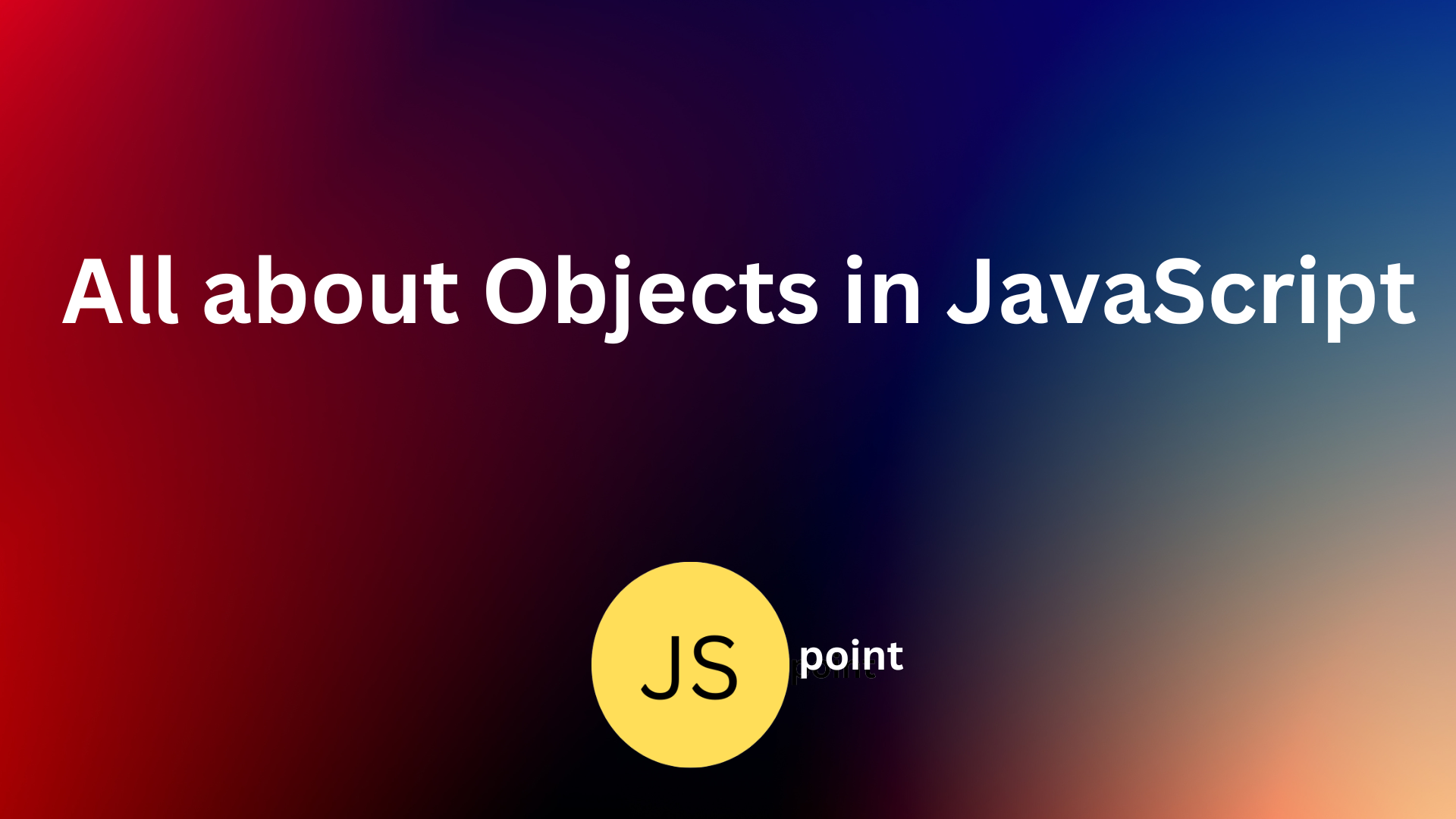
If you understand objects, you understand JavaScript.
In JavaScript, objects are a fundamental data type used to store and organize data. They are key-value pairs, where the keys are strings (or symbols) that uniquely identify the values. Objects can hold any type of value, including primitive data types (such as numbers and strings) and other objects.
Objects in JavaScript are dynamic, meaning that their properties can be added, modified, or deleted at runtime.
In JavaScript, almost "everything" is an object.
For Example
const employee = {
id: 7,
name: 'John',
dept: 'HR'
}
Here is an employee
object with three properties: id, name, and dept as keys and 7, 'John', and 'HR' as values.
Creating a JavaScript Object
Using an Object Literal
This is the easiest way to create a JavaScript Object.
const person = {
name: 'John',
age: 30,
city: 'New York'
};
In this approach, an object is defined using curly braces {}
and the properties and their values are specified within the braces using key-value pairs.
Object Constructor
const person = new Object();
person.name = 'John';
person.age = 30;
person.city = 'New York';
In this approach, an object is created using the Object
constructor, and properties are added using dot notation or bracket notation.
Factory Function
A factory function is a function that returns an object. It allows you to create multiple objects with the same structure and behavior.
function createPerson(name, age, city) {
return {
name: name,
age: age,
city: city,
sayHello: function() {
console.log('Hello, my name is ' + this.name);
}
};
}
const person = createPerson('John', 30, 'New York');
Object.create()
The Object.create()
method creates a new object and sets the prototype of that object to the specified object.
const person = Object.create({
name: 'John',
age: 30,
city: 'New York'
});
ES6 Classes
ES6 introduced the class
syntax, which provides a more concise and structured way to define objects and their behavior.
class Person {
constructor(name, age, city) {
this.name = name;
this.age = age;
this.city = city;
}
sayHello() {
console.log('Hello, my name is ' + this.name);
}
}
const person = new Person('John', 30, 'New York');
JavaScript Objects are Mutable
Objects are mutable. They are addressed by reference, not by value.If person is an object, the following statement will not create a copy of person.The object x is not a copy of person. It is person. Both x and person are the same object. Any changes to x will also change person, because x and person are the same object.
const person = {
firstName:"John",
lastName:"Doe",
age:50, eyeColor:"blue"
}
const x = person;
x.age = 10;
console.log(person); // { firstName: 'John', lastName: 'Doe', age: 10, eyeColor: 'blue' }
Accessing JavaScript Properties
The syntax for accessing the property of an object is:
objectName.property // person.age
or
objectName["property"] // person["age"]
or
objectName[expression] // x = "age"; person[x]
Adding New Properties
person.nationality = "English";
Deleting Properties
The delete
keyword deletes a property from an object:
const person = {
firstName: "John",
lastName: "Doe",
age: 50,
eyeColor: "blue"
};
delete person.age;
or
delete person["age"];
console.log(person); // { firstName: 'John', lastName: 'Doe', eyeColor: 'blue' }
Nested Objects
myObj = {
name:"John",
age:30,
cars: {
car1:"Ford",
car2:"BMW",
car3:"Fiat"
}
}
console.log(myObj.cars.car2); // BMW
console.log(myObj.cars["car2"]); // BMW
console.log(myObj["cars"]["car2"]); // BMW
Object Methods
A JavaScript method is a property containing a function definition.
const person = {
firstName: "John",
lastName: "Doe",
id: 5566,
fullName: function() {
return this.firstName + " " + this.lastName;
}
};
Accessing Object Methods
name = person.fullName();
console.log(name); // John Doe
Adding a Method to an Object
person.name = function () {
return this.firstName + " " + this.lastName;
};
How to Display JavaScript Objects?
const person = {
name: "John",
age: 30,
city: "New York"
};
document.getElementById("demo").innerHTML = person;
Displaying a JavaScript object will output [object Object] in the browser
Some common ways to display JavaScript objects are
Displaying the Object Properties by name
const person = {
name: "John",
age: 30,
city: "New York"
};
document.getElementById("demo").innerHTML =
person.name + "," + person.age + "," + person.city;
// John,30,New York
Displaying the Object in a Loop
const person = {
name: "John",
age: 30,
city: "New York"
};
let txt = "";
for (let x in person) {
txt += person[x] + " ";
};
document.getElementById("demo").innerHTML = txt; // John 30 New York
for(let x in person){
console.log(person[x]);
}
output
//John
//30
//New York
Using Object.values()
Any JavaScript object can be converted to an array using Object.values()
:
const person = {
name: "John",
age: 30,
city: "New York"
};
const myArray = Object.values(person);
console.log(myArray );
// [ 'John', 30, 'New York' ]
document.getElementById("demo").innerHTML=myArray;
// John,30,New York
Using JSON.stringify()
The JSON.stringify()
method converts a JavaScript object into a JSON string representation.
JSON.stringify
will not stringify functions:
const person = {
name: "John",
age: 30,
city: "New York"
};
let myString = JSON.stringify(person);
console.log(myString); // {"name":"John","age":30,"city":"New York"}
document.getElementById("demo").innerHTML = myString;
// {"name":"John","age":30,"city":"New York"}
#
Subscribe to my newsletter
Read articles from tarunkumar pal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

tarunkumar pal
tarunkumar pal
I'm a recent graduate with a degree in Mechanical Engineering but I have a passion for web development. I have hands on in ReactJS and I have experience building responsive, user-friendly web applications using this framework. I gained hands-on experience in web development through several projects . I'm proficient in HTML, CSS, JavaScript, and I have experience working with various front-end technologies such as ReactJS, Redux, and Bootstrap. I'm also familiar with back-end development such as Node.js and Express.js.