Object Destructuring in JavaScript

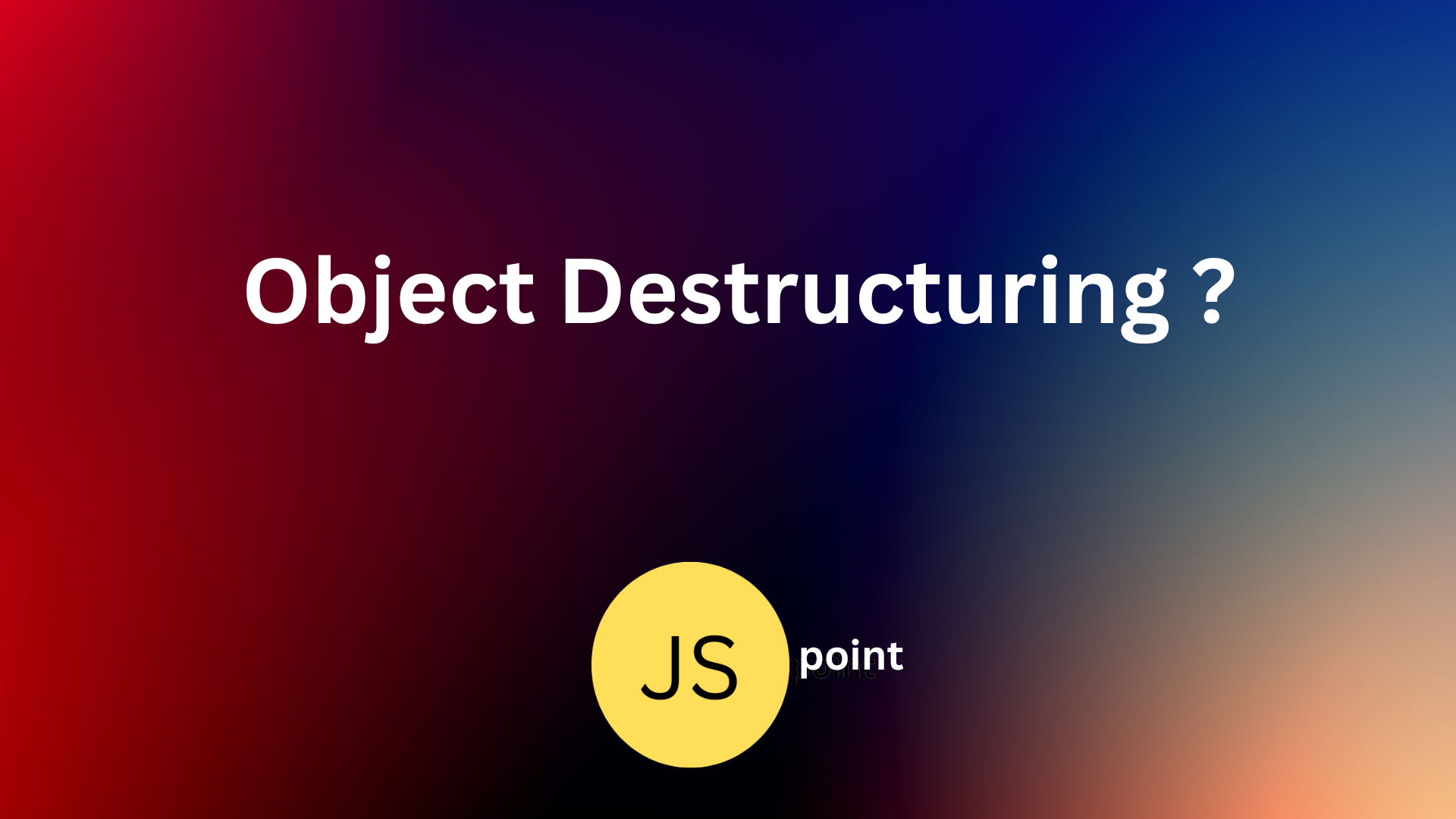
We use JavaScript objects to store data and retrieve it later. We store data in key-value
pairs. The key-value pair is also known as the object properties
.
What is Destructuring ?
Destructuring is a feature in JavaScript that allows you to extract values from objects or arrays and assign them to variables in a more concise and convenient way. It provides a shorthand syntax to extract values from an object and use them directly.
Object Destructuring
When destructuring objects, you can extract specific properties and assign them to variables with the same name as the property.
Use destructuring to extract values from an object
Let's take an example to understand
const person = {
name: 'John',
age: 30,
city: 'New York',
};
const name=person.name;
const age=person.age;
console.log(name); // John
console.log(age); // 30
In this example, we are retrieving the value of age
and name
from the person
object using the dot notation.
To destructure a value from an object, you use a syntax like this,
const { name , age } = person;
const person = {
name: 'John',
age: 30,
city: 'New York',
};
const { name , age } = person;
console.log(name); // John
console.log(age); // 30
In complex applications this game changer. we have to write less code and get the same result.
Use destructuring to extract values from an nested object
const person= {
name: 'John',
age: 30,
dept: {
id: 'D001',
address: {
street: '30 Wellington Square',
city: 'New York'
}
}
}
const street = person.dept.address.street;
console.log(street);
// { street: '30 Wellington Square', city: 'New York' }
const street = person.dept.address.street;
console.log(street); // 30 Wellington Square
const { dept: { address } } = person;
console.log(address);
// { street: '30 Wellington Square', city: 'New York' }
const { dept: { address: { street } } } = person;
console.log(street);
// 30 Wellington Square
Use destructuring aliases
const person = {
name: 'John',
age: 30,
city: 'New York',
};
const { name: myName , age: myAge } = person;
console.log(name); // ReferenceError: name is not defined
console.log(age); // ReferenceError: name is not defined
console.log(myName); // John
console.log(myAge ); // 30
Destructuring objects in the function argument and return value
You can use object destructuring to pass the property values as arguments to the function.
const person = {
name: 'John',
age: 30,
city: 'New York',
};
function logPerson({name, age}) {
console.log(`${name} is ${age}`);
}
You don't need to take the entire object as an argument and pick the required property values. You pass the values directly as function arguments and use them inside.
You can now call the function,
logPerson(person ); // John is 30
If a function returns an object, you can destructure the values directly into variables. Let's create a function that returns an object.
function getUser() {
return {
'name': 'Alex',
'age': 45
}
}
const { age } = getUser();
console.log(age); // 45
Use object destructuring in loops
const person= [
{
'name': 'john',
'address': 'Park Avenue',
'age': 55
},
{
'name': 'Tkp',
'address': 'Delhi',
'age': 30
},
{
'name': 'Raju',
'address': 'Bangalore',
'age': 16
}
];
for(let {name, age} of person) {
console.log(`${name} is ${age} years old!!!`);
}
// output
// john is 55 years old!!!
// Tkp is 30 years old!!!
// Raju is 16 years old!!!
Subscribe to my newsletter
Read articles from tarunkumar pal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

tarunkumar pal
tarunkumar pal
I'm a recent graduate with a degree in Mechanical Engineering but I have a passion for web development. I have hands on in ReactJS and I have experience building responsive, user-friendly web applications using this framework. I gained hands-on experience in web development through several projects . I'm proficient in HTML, CSS, JavaScript, and I have experience working with various front-end technologies such as ReactJS, Redux, and Bootstrap. I'm also familiar with back-end development such as Node.js and Express.js.