Mastering Configuration Management: A Guide to Utilizing Spring Cloud Config for Externalizing Configuration
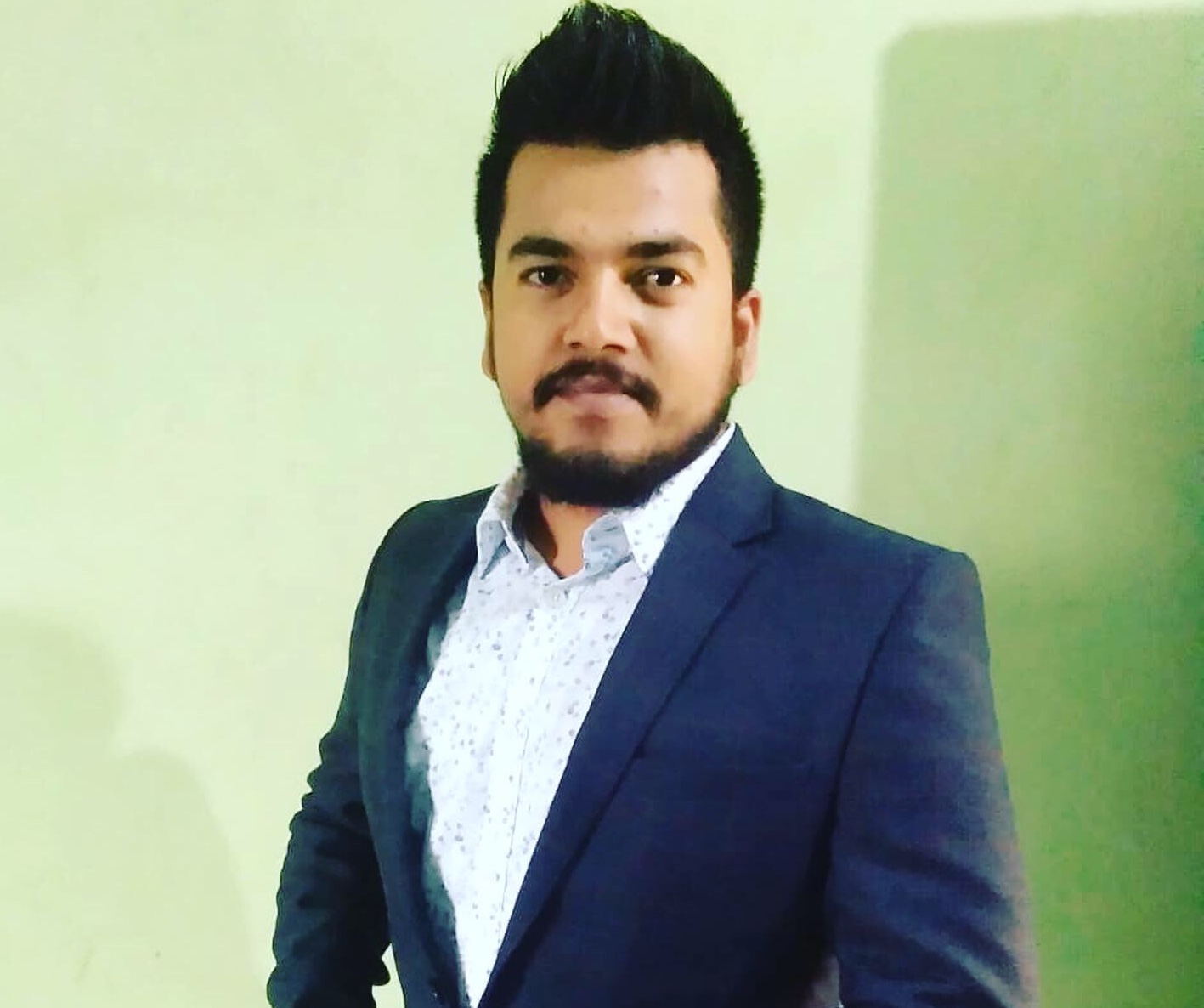
Table of contents
- Introduction
- System Requirements
- Step 1: Setting Up the Configuration Server
- Step 2: Creating the Configuration File in Git Repository
- Step 3: Creating Client Application
- Step 4: Configuring the Client to Connect with the Configuration Server
- Step 5: Creating a Controller in the Client Application
- Step 6: Running and Testing the Applications
- Step 7: Refreshing Configuration Properties
- Conclusion
- GitHub Repository
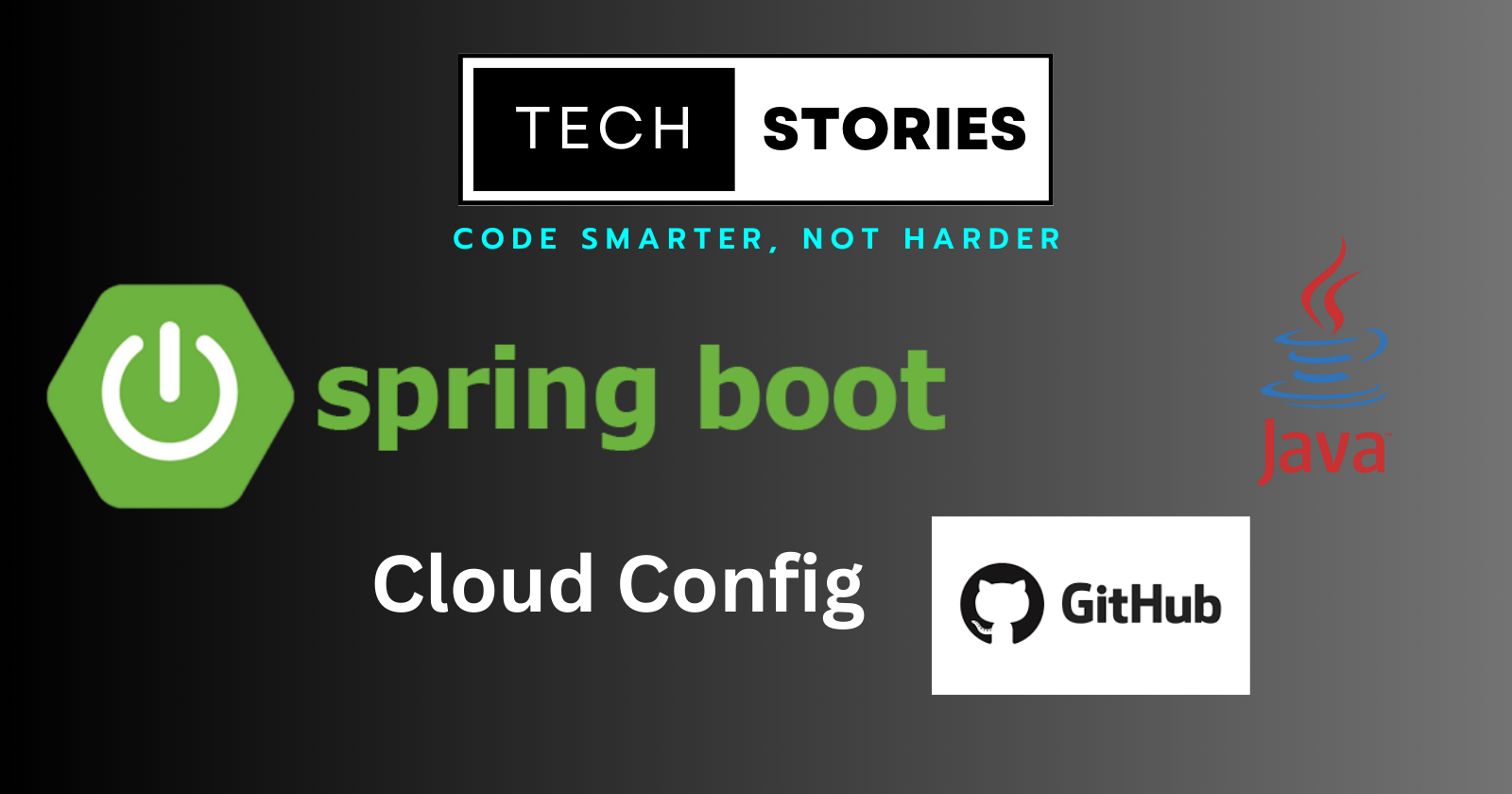
Discover the power of Spring Cloud Config in this comprehensive guide. Learn how to efficiently externalize and manage your application's configuration using Spring Cloud Config, ensuring scalability, flexibility, and ease of maintenance.
Introduction
In today's rapidly evolving technology landscape, building scalable, flexible, and easily maintainable applications is crucial. One of the critical aspects of achieving these goals is effective configuration management. The ability to externalize and manage application configuration centrally can greatly simplify the deployment and maintenance of distributed systems and microservices. This is where Spring Cloud Config comes into play. In this comprehensive guide, we will explore the power of Spring Cloud Config and learn how to leverage it to externalize and manage configuration in your Spring Boot applications.
What is Spring Cloud Config?
Spring Cloud Config is a powerful module within the Spring ecosystem that provides a centralized configuration management solution. It allows you to store application configuration in a Git, SVN, or any other version control system. It provides a configuration server that can serve configuration properties to multiple client applications.
Benefits of Externalizing Configuration
Externalizing configuration offers several advantages for modern application development:
Scalability: With externalized configuration, scaling your application by simply modifying the configuration properties becomes easier. There's no need to redeploy the entire application when a configuration change is required.
Flexibility: Configuration changes can be made dynamically without requiring application restarts, making it possible to adapt to changing environments and business requirements on the fly.
Maintenance Ease: By centralizing configuration, it becomes simpler to manage and version control application settings across different environments, reducing the risk of configuration drift and making troubleshooting easier.
Now let's dive into the practical implementation of Spring Cloud Config.
System Requirements
To follow along with this guide, ensure that you have the following system requirements:
JDK 11 or higher
Maven as the build tool
Git for version control
Postman for API testing
Visual Studio Code (VSCode) or any preferred IDE
Step 1: Setting Up the Configuration Server
To get started, let's use Spring Initializr to create a Spring Boot project for the configuration server.
Visit start.spring.io in your web browser.
Set the following project configurations:
Project: Maven Project
Language: Java
Spring Boot: Choose the latest stable version.
Group: online.techstories.demo
Artifact: config-server
Name: springboot-cloud-config-server
Java: 11
Dependencies: Select "Config Server" and "Spring Web"
Click the "Generate" button to download the project.
Extract the downloaded project and open it in Visual Studio Code (or your preferred IDE).
Open the
ConfigServerApplication.java
file and ensure it contains the following code:@SpringBootApplication @EnableConfigServer public class ConfigServerApplication { public static void main(String[] args) { SpringApplication.run(ConfigServerApplication.class, args); } }
The
@EnableConfigServer
annotation enables the configuration server functionality.Configure the server's properties by adding the following lines to your application's
application.properties
file:spring.application.name=config-server server.port=8888 spring.cloud.config.server.git.uri=<URL to your Git repository> spring.cloud.config.server.git.username=<Git username> spring.cloud.config.server.git.password=<Git password>
Note
Spring Cloud Config supports various configuration sources like Git, SVN, local file systems, and more. In this example, we are using Git as the configuration source.
Step 2: Creating the Configuration File in Git Repository
To store and manage configuration files, we'll use a Git repository. Follow these steps to initialize and create the configuration file in a Git repository:
Install Git on your machine if you haven't already.
Create a new Git repository either locally or on a remote Git hosting platform (e.g., GitHub, GitLab, Bitbucket).
Initialize the Git repository by navigating to the desired directory and running the following command:
git init
Create a configuration file named
my-app.properties
in the Git repository directory. This file will contain the application-specific configuration properties. Add the following sample properties to the file:# Sample Configuration Properties my.property=Hello, World!
Add and commit the configuration file to the Git repository:
git add my-app.properties git commit -m "Initial configuration file"
Create a remote Git Repository and push the local to the remote Git Repository
git remote add origin https://github.com/<username>/<REPOSITORY>.git git push origin master
Step 3: Creating Client Application
Now, let's create a sample client application to retrieve configuration properties from the configuration server.
Using Spring Initializr, create a new Spring Boot project for the client application with the following dependencies:
Config Client
Spring Web
Spring Boot Actuator
Extract the downloaded project and open it in your IDE.
Step 4: Configuring the Client to Connect with the Configuration Server
To connect your client application with the configuration server, you need to specify the necessary configurations.
Open the
application.properties
file in your client application.Add the following properties to connect with the configuration server and specify the name of your client application:
# Client Application Name spring.application.name=my-app # Configuration Server Enabled spring.cloud.config.enabled=true # Spring Config Import, Optional spring.config.import=optional:configserver:http://localhost:8888 #Enable Actuator Refresh Endpoint management.endpoints.web.exposure.include=*
In the above code,
spring.application.name
represents the name of your client application, andspring.cloud.config.uri
specifies the URL of the configuration server.
Step 5: Creating a Controller in the Client Application
Now, let's create a controller in the client application to expose an endpoint for retrieving configuration properties.
Create a file named
MyController.java
under the default package.Add the following code in the controller class:
@RestController public class MyController { @Value("${my.property}") private String myProperty; @GetMapping("/my-property") public String getMyProperty() { return myProperty; } }
The
@Value
annotation is used to inject the value of themy.property
configuration property.
Step 6: Running and Testing the Applications
Now, let's run the applications and test the /my-property
endpoint to retrieve the configuration property.
Run the server application.
Run the client application.
Open a web browser or an API testing tool like Postman.
Send a GET request to
http://localhost:8080/my-property
.You should see the value of the
my.property
configuration property printed on the screen.
Step 7: Refreshing Configuration Properties
Spring Cloud Config allows for dynamic configuration updates without requiring a restart of the client applications. To enable this feature, you need to add the @RefreshScope
annotation to the beans that should be refreshed when the configuration changes.
To demonstrate the dynamic refresh of configuration properties, let's update the property file in the Git repository and see the changes reflected in the client application.
Modify the
MyController.java
class as follows:@RestController @RefreshScope public class MyController { @Value("${my.property}") private String myProperty; @GetMapping("/my-property") public String getMyProperty() { return myProperty; } }
Open the
my-app.properties
file in the Git repository.Update the value of the
my.property
property to a new value.# Sample Configuration Properties my.property=Hello, India!
Commit and push the changes to the Git repository:
git commit -m "Updated configuration property" git push origin master
Make a POST request to the
/actuator/refresh
endpoint of the client application using Postman or any API testing tool.Send a GET request to
/my-property
and observe that the updated value of themy.property
property is retrieved.
Conclusion
In this guide, we explored the power of Spring Cloud Config for externalizing and managing configuration in Spring Boot applications. By leveraging Spring Cloud Config, you can achieve scalability, flexibility, and ease of maintenance in your distributed systems and microservices.
We learned how to set up a configuration server, configure client applications to connect with the server, retrieve configuration properties, and dynamically refresh configurations. By following these steps, you can easily manage and update your application's configuration without requiring redeployment.
Effective configuration management is a vital aspect of modern application development, and Spring Cloud Config provides an excellent solution to simplify this process. By externalizing your configuration, you can adapt to changing requirements, reduce maintenance overhead, and ensure the scalability of your applications.
Remember, mastering configuration management is key to building robust and maintainable systems. So, embrace the power of Spring Cloud Config and elevate your application's configuration management capabilities.
Happy coding!
GitHub Repository
Please refer to the below GitHub location for the completed project.
Config Server Project:
Config Client Project:
Demo Config Repository:
https://github.com/BeingSujeetK/springboot-cloud-config-server.git
Subscribe to my newsletter
Read articles from Sujeet Kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
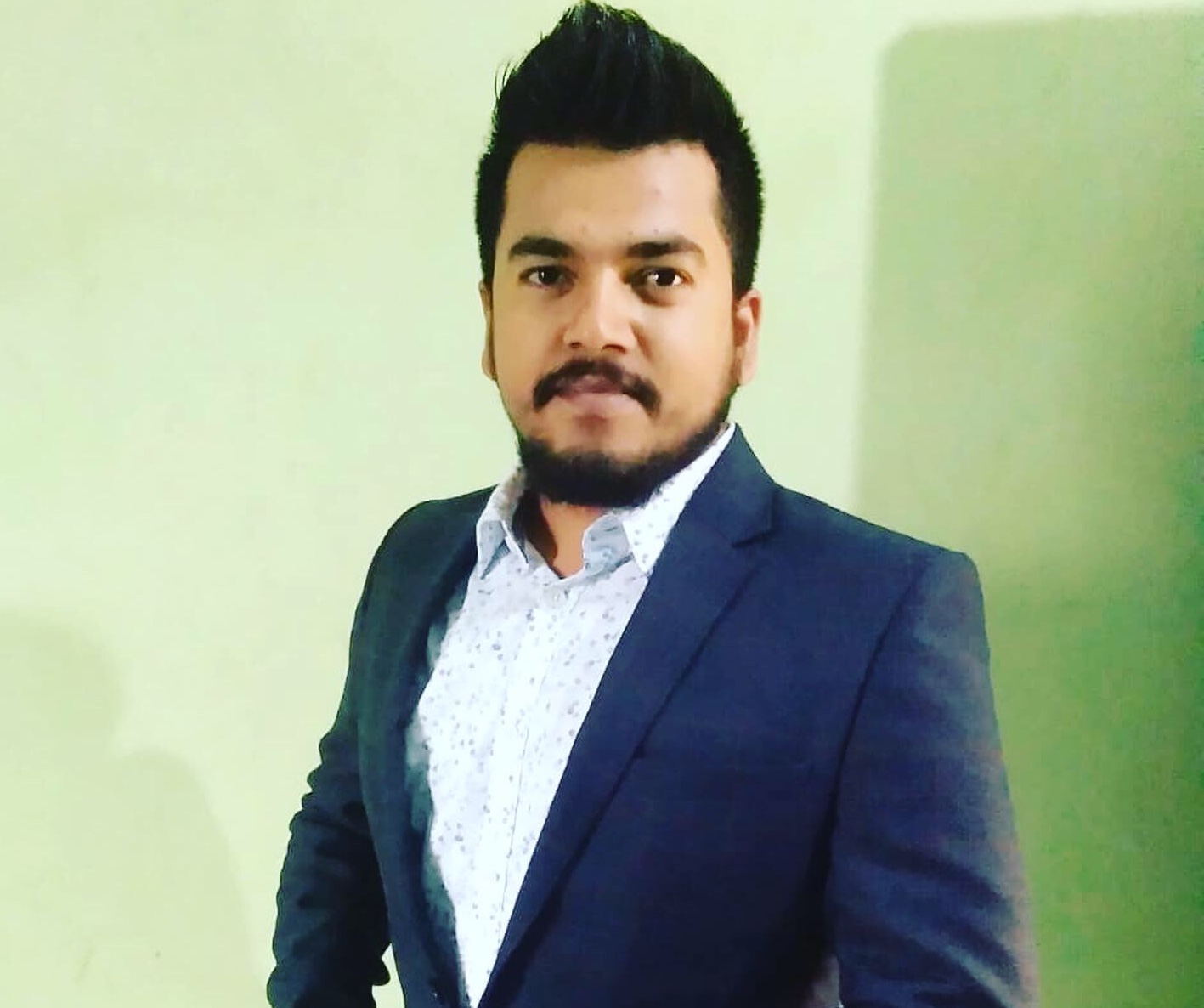
Sujeet Kumar
Sujeet Kumar
As a software developer with over 7 years of experience in the IT industry, I have worked extensively with various programming languages including Java, Spring Boot, JavaScript, and React. I also have strong knowledge and experience in Web Design and Development using tools like Figma and Webflow.