Arrow Functions In JavaScript

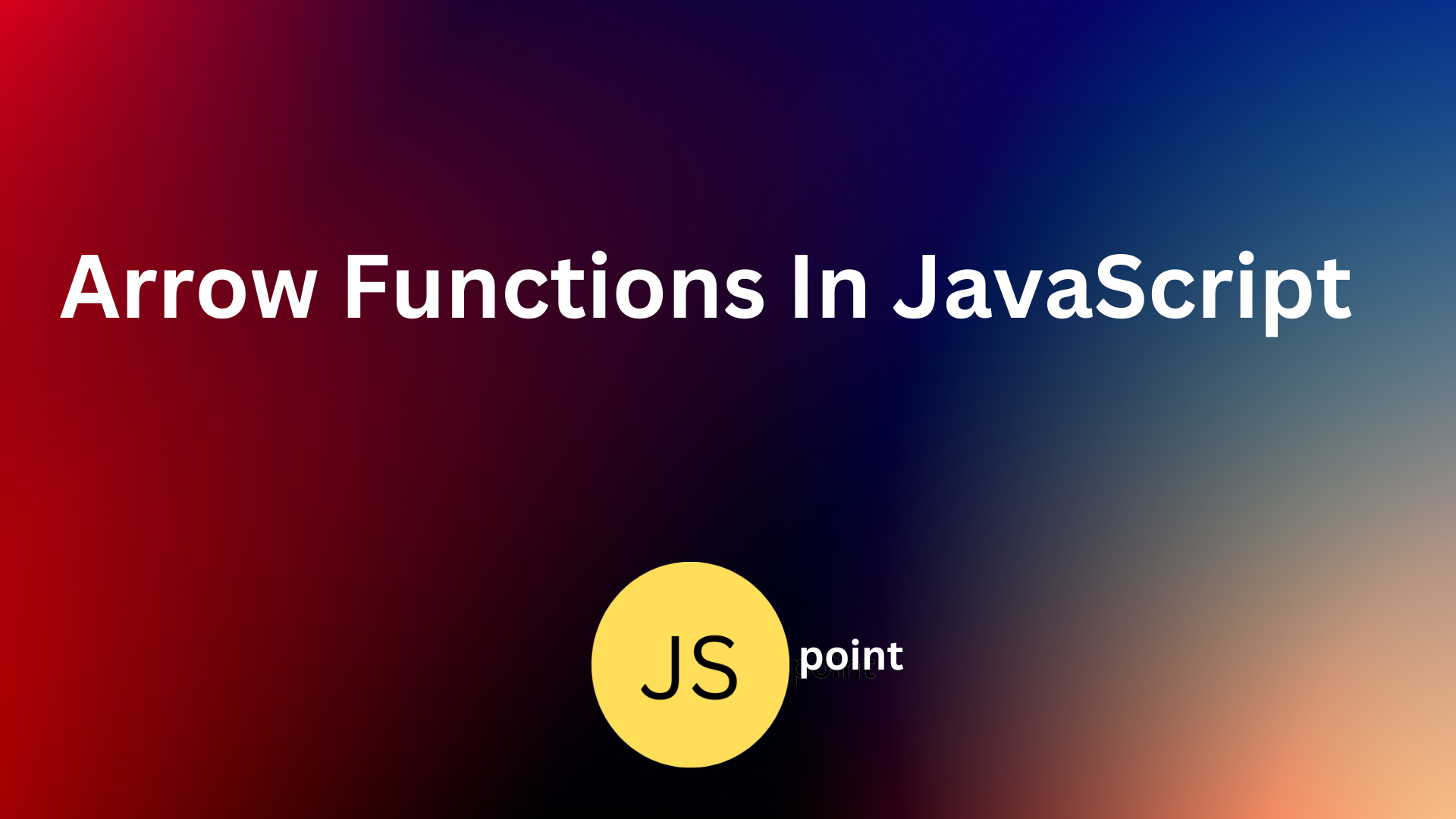
In this article, we'll learn about Arrow Functions in JavaScript in detail
What are Arrow Functions?
Arrow functions were introduced with ES6 as a new way to write functions in JavaScript. The newer syntax made the code less verbose and more readable, as we don't need to write function
and return
keywords.
hey are different from traditional functions in aspects. we will discuss later.
Syntax
const functionName = (parameters) => {
// function body
};
For Example
// 1. One parameter, and a single return statement
const square = x => x*x;
// 2. Multiple parameter, and a single return expression
const sum = (x, y) => x + y;
// 3. Multiple statements in function expression
const sum = (x, y) =>{
console.log(`Adding ${x} and ${y}`);
return x + y;
}
// 4. Returning an object
const sumAndDifference = (x, y) => ({ sum: x + y, difference: x - y });
When to use Arrow Functions?
For passing anonymous callback functions to array methods
The array methods like .map()
, .filter()
, .reduce()
, etc. can look really concise and clean when arrow functions are used to pass the callback functions.
const numbers = [1, 2, 3, 4, 5];
const sum = numbers.reduce((a, b) => a + b, 0);
Promise Chaining
The promise chaining can look very confusing with repetitive function
and return
keywords. By using arrow functions, we can write promise chains with minimal code and a more readable manner.
fetch(URL)
.then(res => res.json())
.then(json => json.data)
.then(data => data.map(dataItem => console.log(dataItem)))
Callback Functions
In JavaScript, we write a lot of callback functions, and using arrow functions to do that can make it really clean
setTimeout(() => {
console.log("I'll get logged first");
setTimeout(() => {
console.log("I'll get logged later");
})
});
When to Not to use Arrow Functions?
Object Methods:
Arrow functions do not bind their own this
value, so they are not suitable for defining object methods. Object methods often require access to the object's properties and methods using this
, which arrow functions do not have. Regular functions should be used instead.
The value of this
inside an arrow function is the same as that of it's outer function. In other words, arrow function resolve the value of this
lexically and this
behavior is independent of how and where the function is executed.
For example:
// Not suitable for object methods
const obj = {
name: 'John',
greet: () => {
console.log(`Hello, ${this.name}!`); // 'this' does not refer to the object
}
};
// Preferred approach
const obj = {
name: 'John',
greet() {
console.log(`Hello, ${this.name}!`); // 'this' refers to the object
}
};
Constructors:
Arrow functions cannot be used as constructors to create new instances of objects. Regular functions should be used as constructors. For example:
// Not suitable for constructors
const Person = (name) => {
this.name = name; // Error: 'this' is not bound
};
// Preferred approach
function Person(name) {
this.name = name;
}
Methods that require arguments binding:
Arrow functions do not have their own arguments
object, which can be useful in some cases when dealing with variable-length arguments. If you need to access the arguments
object or dynamically bind arguments, a regular function should be used. For example:
// Not suitable when accessing 'arguments'
const sum = (...numbers) => {
return numbers.reduce((acc, curr) => acc + curr);
};
// Preferred approach
function sum() {
return Array.from(arguments).reduce((acc, curr) => acc + curr);
}
Function Hoisting
In regular function, function gets hoisting at top.
normalFunc()
function normalFunc() {
return "Normal Function"
}
// "Normal Function"
arrowFunc()
const arrowFunc = () => {
return "Arrow Function"
}
// ReferenceError: Cannot access 'arrowFunc' before initialization
In arrow function, function get hoisted where you define. So, if you call the function before initialisation you will get referenceError.
Subscribe to my newsletter
Read articles from tarunkumar pal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

tarunkumar pal
tarunkumar pal
I'm a recent graduate with a degree in Mechanical Engineering but I have a passion for web development. I have hands on in ReactJS and I have experience building responsive, user-friendly web applications using this framework. I gained hands-on experience in web development through several projects . I'm proficient in HTML, CSS, JavaScript, and I have experience working with various front-end technologies such as ReactJS, Redux, and Bootstrap. I'm also familiar with back-end development such as Node.js and Express.js.