Callback Functions In JavaScript

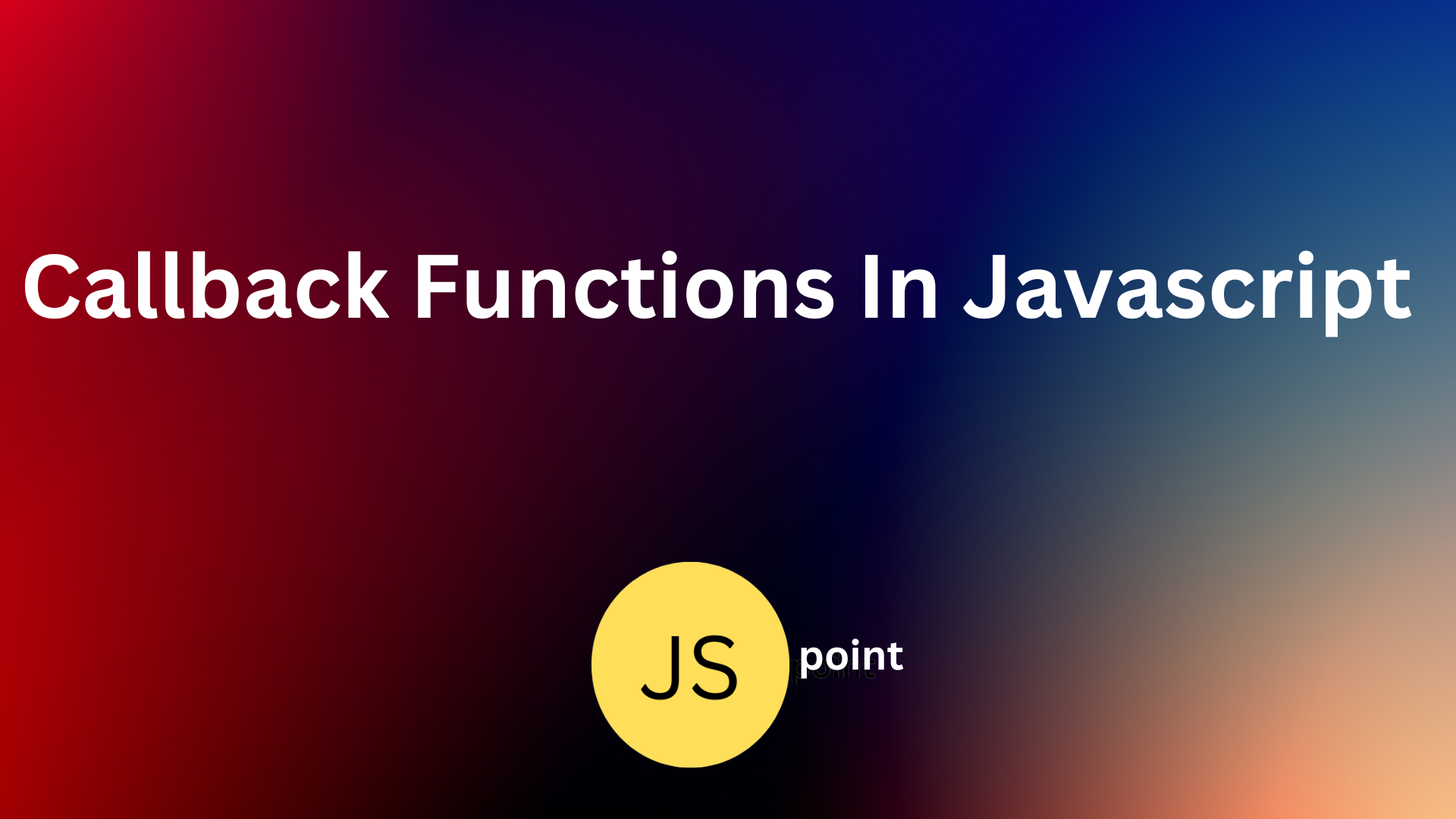
In this article, we will discuss the callback functions in detail.
What is a Callback Functions?
A callback function is a function that is passed as an argument to another function and is executed at a later time or after a certain event occurs.
"I will call back later!"
function outer(callbackFn){
//...
}
outer(function inner(){
console.log("I am a callback function")
});
In the above code snippet, inner()
function is known as callback function.
Where to use the Callback function?
Callbacks make sure that a function is not going to run before a task is completed but will run right after the task has completed. It helps us develop asynchronous JavaScript code and keeps us safe from problems and errors.
Asynchronous functions:
function fetchData(callback) {
// Simulating an asynchronous operation
setTimeout(function() {
const data = { name: 'John', age: 30 };
callback(data);
}, 2000);
}
function processData(data) {
console.log('Received data:', data);
}
fetchData(processData);
// Received data: { name: 'John', age: 30 }
In this example, the fetchData
function acts as a asynchronous operation using setTimeout
. It takes a callback function callback
as a parameter. After a delay of 2000 milliseconds, it creates an object data
and invokes the callback
function with the data
as an argument.
The processData
function is defined separately and serves as the callback function. It receives the data
object as an argument and logs a message to the console, indicating that data has been received.
When we call fetchData(processData)
, we pass the processData
function as the callback. This means that when the data is retrieved after the delay, the processData
function is invoked with the received data as its argument.
As a result, when the asynchronous operation completes, the callback function is triggered, and we see the message "Received data: { name: 'John', age: 30 }" logged to the console. This demonstrates the use of a callback function to handle the retrieved data asynchronously.
Event listeners:
document.addEventListener('click', function() {
console.log('Button clicked!');
});
In this example, the callback function is triggered when a click event occurs on the document. The function logs a message to the console, providing a way to handle the event.
Promises:
function fetchData() {
return new Promise(function(resolve, reject) {
// Simulating an asynchronous operation
setTimeout(function() {
const data = { name: 'Jane', age: 25 };
resolve(data);
}, 2000);
});
}
fetchData()
.then(function(data) {
console.log('Received data:', data);
})
.catch(function(error) {
console.error('Error:', error);
});
In this example, the fetchData
function returns a Promise
object. Inside the Promise
constructor, an asynchronous operation is simulated using setTimeout
. After a delay of 2000 milliseconds, it creates an object data
and resolves the Promise
with the data
.
The fetchData
function can be called without passing a callback. Instead, it returns a Promise
that can be used to handle the asynchronous result. We can use the .then()
method on the Promise
to specify what should happen when the promise is resolved. The .catch()
method is used to handle any errors that may occur during the asynchronous operation.
In this example, the .then()
method is used to define a callback function that receives the resolved data as its argument. The callback function logs a message to the console, indicating that data has been received. If any errors occur, they are handled by the .catch()
method, which logs an error message to the console.
When we call fetchData()
, it returns a Promise
object. We can chain the .then()
and .catch()
methods to handle the asynchronous result. When the data is retrieved after the delay, the .then()
callback is triggered with the received data as its argument, and the message "Received data: { name: 'Jane', age: 25 }" is logged to the console.
Subscribe to my newsletter
Read articles from tarunkumar pal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

tarunkumar pal
tarunkumar pal
I'm a recent graduate with a degree in Mechanical Engineering but I have a passion for web development. I have hands on in ReactJS and I have experience building responsive, user-friendly web applications using this framework. I gained hands-on experience in web development through several projects . I'm proficient in HTML, CSS, JavaScript, and I have experience working with various front-end technologies such as ReactJS, Redux, and Bootstrap. I'm also familiar with back-end development such as Node.js and Express.js.