HashMap Data Structure

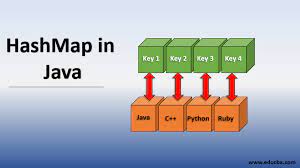
HashMap is a class in Java that implements the Map interface and is part of the Java Collections Framework. It is used to store and manipulate key-value pairs, where each key is unique within the map. It uses an array and LinkedList to store key-value pairs.
Here are some important features and characteristics of HashMap in Java:
Key-Value Pairs: Each element in a HashMap is stored as a key-value pair, where the key is used to retrieve the corresponding value.
Unique Keys: Keys in a HashMap must be unique. Duplicate keys are not allowed, and if you attempt to insert a duplicate key, the existing value will be overwritten.
Null Keys and Values: HashMap allows null keys and null values. You can have a key-value pair with a null key or a null value, but there can be at most one entry with a null key.
Hashing: HashMap uses the hash code of the key to determine the index of the corresponding value in an internal array. It employs a hashing technique to efficiently store and retrieve elements.
Performance: The performance of HashMap operations, such as adding, removing, and retrieving elements, is generally constant time (O(1)), assuming a good hash function and an evenly distributed set of keys. However, in the case of collisions (when different keys have the same hash code), the performance can degrade to O(n), where n is the number of elements in the collision chain.
Iteration Order: The iteration order of elements in a HashMap is not guaranteed. If you need a specific order, you can use the LinkedHashMap class, which maintains the insertion order.
Thread Safety: By default, HashMap is not thread-safe. If you need to use it in a concurrent environment, you should consider using the ConcurrentHashMap class or applying proper synchronization mechanisms.
Declaration of Hashmaps-
public class HashMapExample1{
public static void main(String args[]){
HashMap<Integer,String> map=new HashMap<Integer,String>();//Creating HashMap
map.put(1,"Jay"); //Put elements in Map
map.put(2,"Veeru");
map.put(3,"Gabbar");
map.put(4,"Thakur");
System.out.println("Iterating Hashmap...");
for(Map.Entry m : map.entrySet()){
System.out.println(m.getKey()+" "+m.getValue());
}
}
}
Internal working of hashmaps-
Before understanding the internal working of HashMap, you must be aware of the hashCode() and equals() methods.
equals(): It checks the equality of two objects. It compares the keys, whether they are equal or not. It is a method of the Object class. It can be overridden. If you override the equals() method, then it is mandatory to override the hashCode() method.
hashCode(): This is the method of the object class. It returns the memory reference of the object in integer form. The value received from the method is used as the bucket number. The bucket number is the address of the element inside the map. The hash code of the null Key is 0.
Buckets: The array of the node is called buckets. Each node has a data structure like a LinkedList. More than one node can share the same bucket. It may be different in capacity.
Important methods -
put(key, value)
: Inserts a key-value pair into the map.get(key)
: Retrieves the value associated with a given key.containsKey(key)
: Checks if the map contains a specific key.containsValue(value)
: Checks if the map contains a specific value.remove(key)
: Removes the key-value pair associated with a given key.size()
: Returns the number of key-value pairs in the map.isEmpty()
: Checks if the map is empty.keySet()
: Returns a set of all the keys in the map.values()
: Returns a collection of all the values in the map.entrySet()
: Returns a set of all the key-value entries in the map.clear()
: Removes all the key-value pairs from the map.Uses of Hashmaps-
Data storage and retrieval: HashMaps provide an efficient way to store and retrieve data using key-value pairs. They are often used to store and retrieve data based on unique identifiers (keys) such as user IDs, product codes, or names.
Caching: HashMaps can be used as a cache to store frequently accessed data, avoiding the need to retrieve the data from expensive or slow sources repeatedly. This can improve the performance of applications by reducing the response time.
Indexing and searching: HashMaps are suitable for indexing and searching data based on keys. They allow for quick lookup operations, making them useful for scenarios such as indexing documents, implementing dictionaries, or building search engines.
Counting occurrences: HashMaps can be used to count the frequency of occurrences of elements in a collection. For example, you can use a HashMap to count the number of times each word appears in a text document.
Implementing associations: HashMaps are used to establish associations between related objects. For example, in a social networking application, a HashMap can be used to associate a user's profile with their friends or followers.
Memoization: HashMaps can be employed to implement memoization, which is a technique for caching function results based on the input parameters. This can optimize the performance of functions that are computationally expensive or have repetitive calculations.
Configurations and settings: HashMaps are useful for storing and managing configuration settings or properties. Key-value pairs can represent various settings, and HashMaps provides a flexible way to access and modify these settings.
These are just a few examples of the many use cases for HashMaps. HashMaps provide a versatile and efficient data structure for various applications that require fast and convenient storage, retrieval, and manipulation of key-value pairs.
Happy Coding
Subscribe to my newsletter
Read articles from Anuj Rathour directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
