Optional chaining and Nullish Coalescing Operator

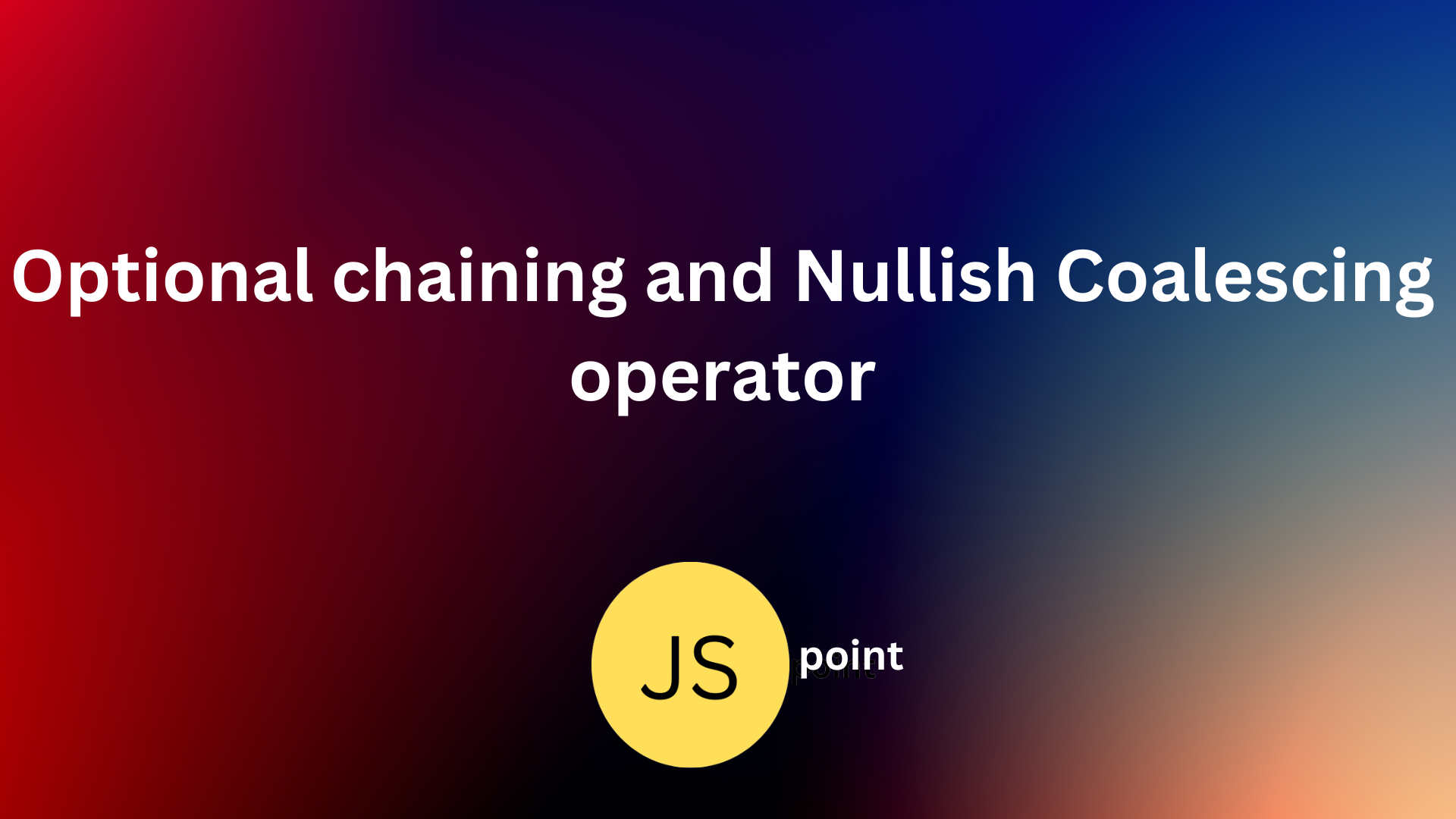
What is an Optional chaining Operator?
The Optional Chaining Operator returns
undefined
if an object isundefined
ornull
(instead of throwing an error).
The optional chaining operator (?.) enables you to read the value of a property located deep within a chain of connected objects without having to check that each reference in the chain is valid.
The optional chaining operator (?.) allows you to safely access nested properties or methods without causing an error if any intermediate property or object is null or undefined. If any property in the chain is null or undefined, the expression short-circuits and returns undefined instead of throwing an error.
For example
const user = {
name: 'John',
address: {
city: 'New York',
street: '123 ABC Street'
},
// comment out the following line to see the effect of optional chaining
// address: null,
getInfo() {
return `Name: ${this.name}, City: ${this.address.city}`;
}
};
console.log(user.addres?.city) // output: undefined
console.log(user.addres.city) // TypeError: Cannot read properties of undefined
console.log(user.address?.city); // Output: 'New York'
console.log(user.address?.zipCode); // Output: undefined
console.log(user.address?.country?.name); // Output: undefined
console.log(user.getInfo?.()); // Output: 'Name: John, City: New York'
console.log(user.unknownMethod?.()); // Output: undefined
In the example above, the optional chaining operator (?.) is used to safely access the nested properties city
, zipCode
, and country.name
without throwing an error if any of the intermediate properties are null or undefined. Similarly, it is used to safely call the getInfo()
method without causing an error if it doesn't exist.
Lets, we will learn Nullish Coalescing Operator (??)
What is Nullish Coalescing Operator (??)
The
??
operator returns the first argument if it is not nullish (null
orundefined
).Otherwise, it returns the second.
The Nullish Coalescing Operator evaluates the expression on its left-hand side and, if the result is nullish (null or undefined), it returns the value on its right-hand side. However, if the left-hand side expression is defined and not nullish, the operator returns the value of the left-hand side expression.
For Example
const foo = null;
const bar = undefined;
const baz = 0;
const qux = '';
const result1 = foo ?? 'default value';
console.log(result1); // Output: 'default value'
const result2 = bar ?? 'default value';
console.log(result2); // Output: 'default value'
const result3 = baz ?? 'default value';
console.log(result3); // Output: 0
const result4 = qux ?? 'default value';
console.log(result4); // Output: ''
In the example above, the Nullish Coalescing Operator (??) is used to provide default values for variables foo
, bar
, baz
, and qux
in case they are nullish. In the cases where the left-hand side expression is null or undefined (foo
and bar
), the operator returns the specified default value. However, for baz
and qux
, where the left-hand side expression is defined and not nullish, the operator returns the value of the left-hand side expression.
The Nullish Coalescing Operator differs from the Logical OR Operator (||) in how it handles falsy values. The Logical OR Operator considers falsy values such as 0
, empty string (''
), and false
as nullish values and returns the right-hand side expression in those cases. In contrast, the Nullish Coalescing Operator only considers null
and undefined
as nullish values.
Subscribe to my newsletter
Read articles from tarunkumar pal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

tarunkumar pal
tarunkumar pal
I'm a recent graduate with a degree in Mechanical Engineering but I have a passion for web development. I have hands on in ReactJS and I have experience building responsive, user-friendly web applications using this framework. I gained hands-on experience in web development through several projects . I'm proficient in HTML, CSS, JavaScript, and I have experience working with various front-end technologies such as ReactJS, Redux, and Bootstrap. I'm also familiar with back-end development such as Node.js and Express.js.