Exception Handling In JavaScript

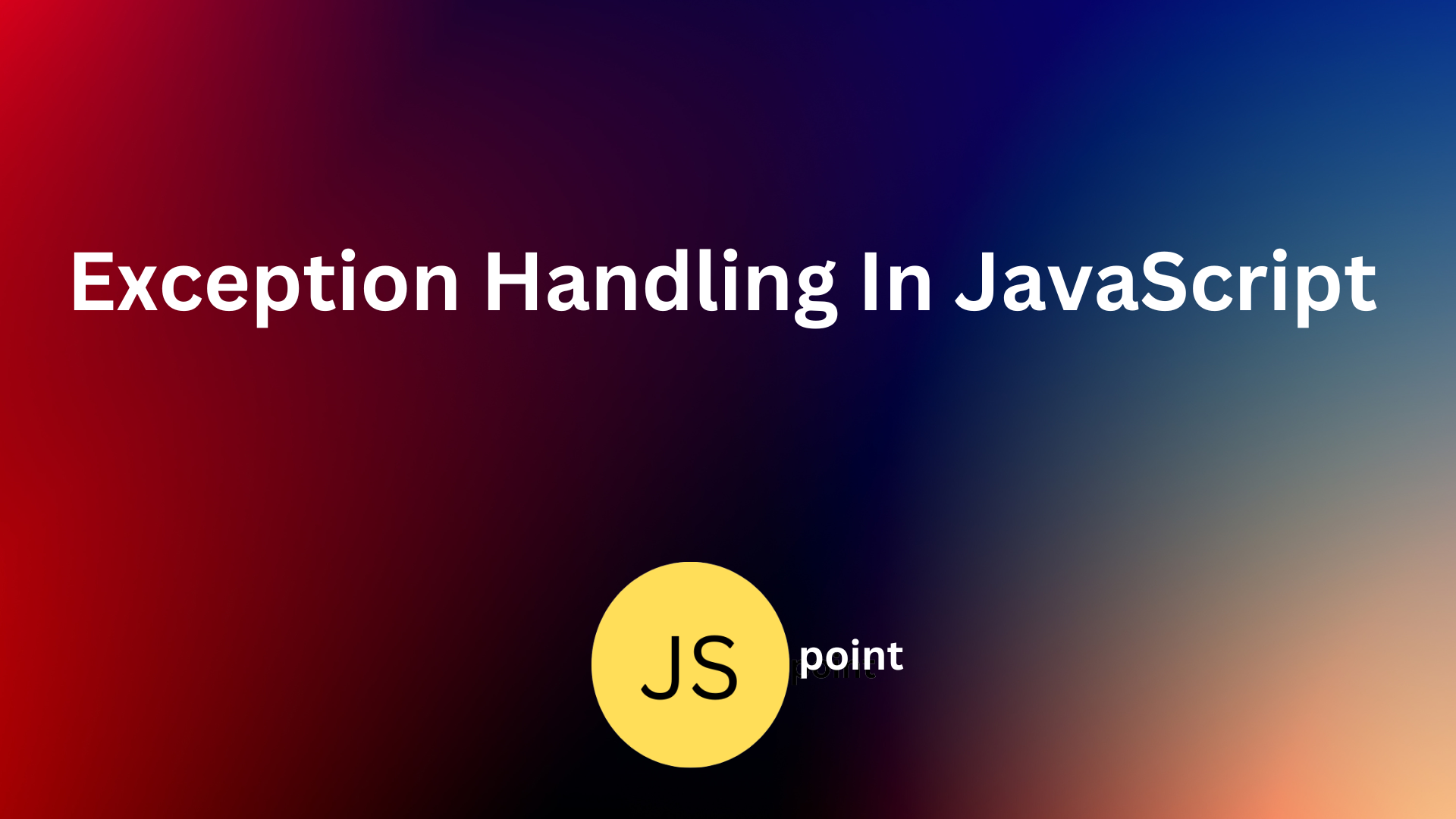
In this article, we will learn about how to handle unconditional errors in the program.
Types of Errors
Understanding the different types of errors can help you diagnose and handle them appropriately. Here are some common types of errors in JavaScript:
Syntax Errors: Syntax errors occur when the JavaScript engine encounters code that violates the language syntax rules. These errors prevent the code from being parsed and executed. Examples of syntax errors include missing semicolons, unmatched braces or parentheses, and incorrect variable declarations.
Reference Errors: Reference errors occur when you try to access a variable or function that is not defined or is out of scope. This can happen when using an undeclared variable, referencing a variable before it is assigned a value, or accessing properties or methods of undefined or null values.
Type Errors: Type errors occur when you perform an operation on a value of an incompatible type. For example, trying to call a non-function as a function, performing arithmetic operations on non-numeric values, or accessing properties or methods of non-object types.
Range Errors: Range errors occur when you use a value that is outside the range of acceptable values. For example, using negative values with functions or methods that expect positive values, or exceeding the maximum length of an array or string.
How to handle errors
try...catch Statement
statement is used for exception handling in JavaScript. The general syntax is as follows:
try {
// Code that may throw an exception
} catch (error) {
// Code to handle the exception
}
Here's an example that demonstrates exception handling in JavaScript:
try {
// Code that may throw an exception
const result = 10 / 0; // Division by zero
console.log(result);
} catch (error) {
// Code to handle the exception
console.error('An error occurred:', error);
}
In the above example, the code attempts to divide 10
by 0
, which is an invalid operation. This would normally result in an exception being thrown and the program crashing. However, by enclosing the code within a try
block and providing a catch
block, we can handle the exception gracefully. If an exception occurs, the code execution jumps to the catch
block, and the error
object contains information about the exception. In this case, we log an error message to the console.
throw Statement
The throw
statement in JavaScript is used to throw custom errors or exceptions. When a throw
statement is executed, it interrupts the normal flow of the program and transfers control to the nearest enclosing try...catch
block.
Here's an example that demonstrates how to use the throw
statement:
function divide(a, b) {
if (b === 0) {
throw new Error("Cannot divide by zero");
}
return a / b;
}
try {
let result = divide(10, 0);
console.log(result); // This line will not be executed
} catch (error) {
console.log(error.message); // Output: "Cannot divide by zero"
}
In the example, the divide
function checks if the divisor b
is zero. If it is, a new Error
object is thrown with a custom error message. The try...catch
block is used to catch the thrown error, and the error message is logged to the console.
The throw
statement allows you to create custom error conditions in your code and handle them appropriately using try...catch
blocks. It's useful for signaling exceptional situations and providing descriptive error messages to aid in debugging and error handling.
finally Block
The finally
block in JavaScript is used in conjunction with the try...catch
statement to specify a block of code that will be executed regardless of whether an exception is thrown or not. The code inside the finally
block will always be executed, regardless of whether an exception is caught or not.
Here's the syntax of the try...catch...finally
statement:
try {
// Code that may throw an exception
} catch (error) {
// Code to handle the exception
} finally {
// Code that will always be executed
}
The finally
block is optional and can be used along with the try...catch
block. The purpose of the finally
block is to specify a set of statements that will be executed regardless of whether an exception is thrown or caught.
Here's an example that demonstrates the use of the finally
block:
function divide(a, b) {
try {
if (b === 0) {
throw new Error("Cannot divide by zero");
}
return a / b;
} catch (error) {
console.log(error.message);
return null;
} finally {
console.log("Division operation completed");
}
}
let result = divide(10, 2); // Output: Division operation completed
console.log(result); // Output: 5
result = divide(10, 0); // Output: Cannot divide by zero, Division operation completed
console.log(result); // Output: null
In the example, the divide
function attempts to divide two numbers a
and b
. If b
is zero, an error is thrown. The catch
block handles the exception and logs the error message. The finally
block is executed regardless of whether an exception is thrown or caught, and it logs a completion message indicating that the division operation is completed.
Subscribe to my newsletter
Read articles from tarunkumar pal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

tarunkumar pal
tarunkumar pal
I'm a recent graduate with a degree in Mechanical Engineering but I have a passion for web development. I have hands on in ReactJS and I have experience building responsive, user-friendly web applications using this framework. I gained hands-on experience in web development through several projects . I'm proficient in HTML, CSS, JavaScript, and I have experience working with various front-end technologies such as ReactJS, Redux, and Bootstrap. I'm also familiar with back-end development such as Node.js and Express.js.