JavaScript Strings

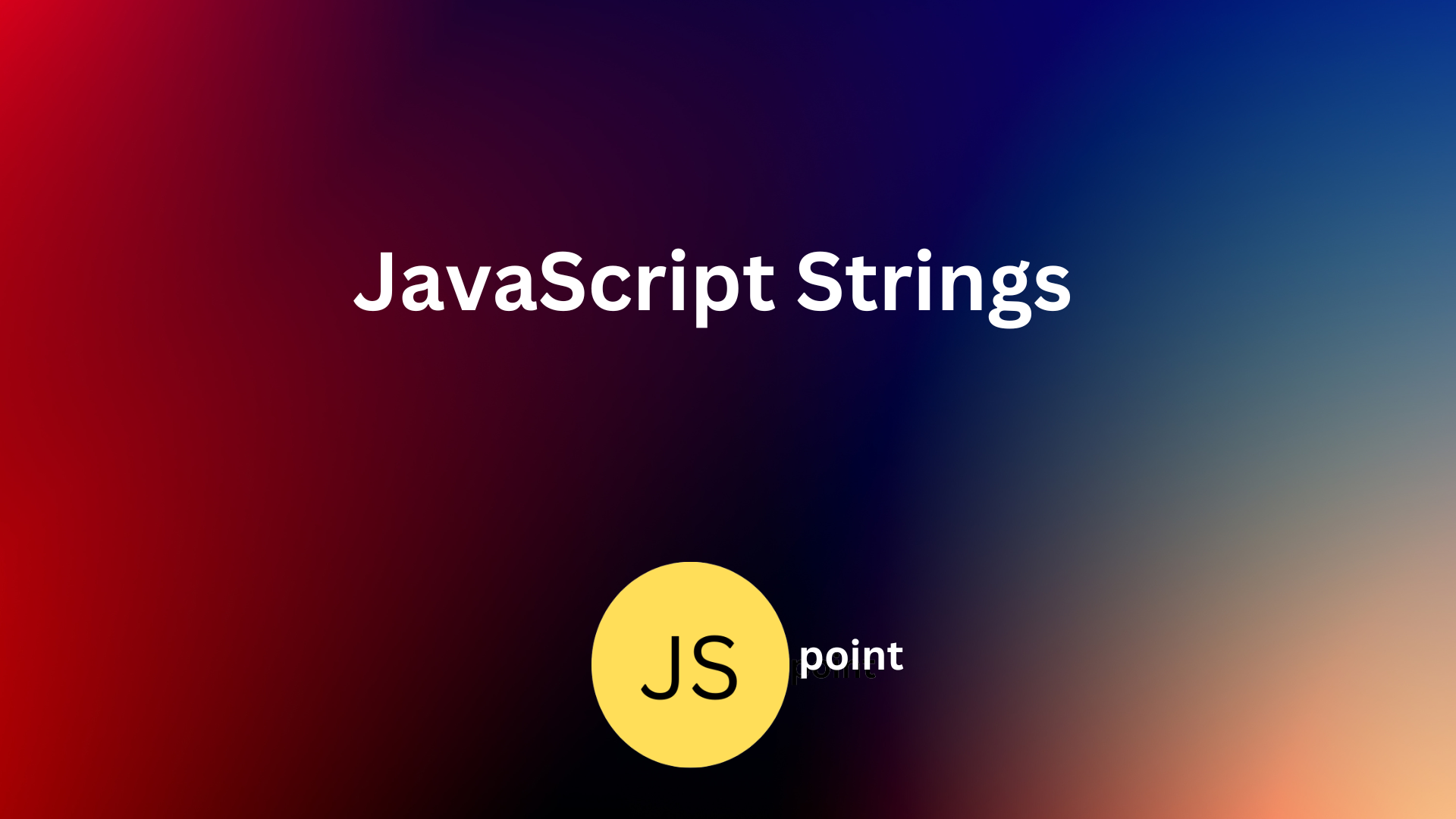
In this article, we learn about strings and their methods
What is String?
a string is a sequence of characters enclosed in single quotes (''), double quotes ("") or backticks (``).
Strings are immutable, meaning their values cannot be changed once created
Here are some commonly used string methods:
slice()
The slice()
method extracts a part of a string and returns it as a new string, without modifying the original string.
Syntax
slice(indexStart)
slice(indexStart, indexEnd)
const str = 'The quick brown fox jumps over the lazy dog.';
console.log(str.slice(31));
// Expected output: "the lazy dog."
console.log(str.slice(4, 19));
// Expected output: "quick brown fox"
console.log(str.slice(-4));
// Expected output: "dog."
console.log(str.slice(-9, -5));
// Expected output: "lazy"
substring()
The substring()
method returns the part of the string
from the start index up to and excluding the end index.
Syntax
substring(indexStart)
substring(indexStart, indexEnd)
const str = 'Mozilla';
console.log(str.substring(1, 3));
// Expected output: "oz"
console.log(str.substring(2));
// Expected output: "zilla"
trim()
The trim()
method removes whitespace from both ends of a string and returns a new string, without modifying the original string.
Syntax
trim()
const greeting = ' Hello world! ';
console.log(greeting); // Expected output: " Hello world! ";
console.log(greeting.trim()); // Expected output: "Hello world!";
trimEnd()
The trimEnd()
method removes whitespace from the end of a string and returns a new string, without modifying the original string.
Syntax
trimEnd()
const greeting = ' Hello world! ';
console.log(greeting);
// Expected output: " Hello world! ";
console.log(greeting.trimEnd());
// Expected output: " Hello world!";
trimStart()
The trimStart()
method removes whitespace from the beginning of a string and returns a new string, without modifying the original string.
trimStart()
const greeting = ' Hello world! ';
console.log(greeting);
// Expected output: " Hello world! ";
console.log(greeting.trimStart());
// Expected output: "Hello world! ";
toLowerCase()
The toLowerCase()
method returns the calling string value converted to lower case
Syntax
toLowerCase()
const sentence = 'The quick brown fox jumps over the lazy dog.';
console.log(sentence.toLowerCase());
// Expected output: "the quick brown fox jumps over the lazy dog."
toUpperCase()
The toUpperCase()
method returns the calling string value converted to uppercase
Syntax
toUpperCase()
const sentence = 'The quick brown fox jumps over the lazy dog.';
console.log(sentence.toUpperCase());
// Expected output: "THE QUICK BROWN FOX JUMPS OVER THE LAZY DOG."
split()
The split()
method splits a string into an array of substrings based on a specified delimiter.
Syntax
split(separator)
split(separator, limit)
const str = 'The quick brown fox jumps over the lazy dog.';
const words = str.split(' ');
console.log(words[3]);
// Expected output: "fox"
const chars = str.split('');
console.log(chars[8]);
// Expected output: "k"
const strCopy = str.split();
console.log(strCopy);
// Expected output: Array ["The quick brown fox jumps over the lazy dog."]
startsWith()
The startsWith()
method determines whether a string begins with the characters of a specified string, returning true
or false
as appropriate.
Syntax
startsWith(searchString)
startsWith(searchString, position)
const str1 = 'Saturday night plans';
console.log(str1.startsWith('Sat'));
// Expected output: true
console.log(str1.startsWith('Sat', 3));
// Expected output: false
search()
The search()
method executes a search for a match between a regular expression and this String
object.
Syntax
search(regexp)
const paragraph = 'The quick brown fox jumps over the lazy dog. If the dog barked, was it really lazy?';
// Any character that is not a word character or whitespace
const regex = /[^\w\s]/g;
console.log(paragraph.search(regex));
// Expected output: 43
console.log(paragraph[paragraph.search(regex)]);
// Expected output: "."
replace()
The replace()
method returns a new string with one, some, or all matches of a pattern
replaced by a replacement
. The pattern
can be a string or a RegExp
, and the replacement
can be a string or a function called for each match. If pattern
is a string, only the first occurrence will be replaced. The original string is left unchanged.
Syntax
replace(pattern, replacement)
const p = 'The quick brown fox jumps over the lazy dog. If the dog reacted, was it really lazy?';
console.log(p.replace('dog', 'monkey')); // Expected output: "The quick brown fox jumps over the lazy monkey. If the dog reacted, was it really lazy?"
const regex = /Dog/i; console.log(p.replace(regex, 'ferret')); // Expected output: "The quick brown fox jumps over the lazy ferret. If the dog reacted, was it really lazy?"
replaceAll()
The replaceAll()
method returns a new string with all matches of a pattern
replaced by a replacement
. The pattern
can be a string or a RegExp
, and the replacement
can be a string or a function to be called for each match. The original string is left unchanged.
Syntax
replaceAll(pattern, replacement)
const p = 'The quick brown fox jumps over the lazy dog. If the dog reacted, was it really lazy?';
console.log(p.replaceAll('dog', 'monkey'));
// Expected output: "The quick brown fox jumps over the lazy monkey. If the monkey reacted, was it really lazy?"
// Global flag required when calling replaceAll with regex
const regex = /Dog/ig;
console.log(p.replaceAll(regex, 'ferret'));
// Expected output: "The quick brown fox jumps over the lazy ferret. If the ferret reacted, was it really lazy?"
padStart()
The padStart()
method pads the current string with another string (multiple times, if needed) until the resulting string reaches the given length. The padding is applied from the start of the current string.
Syntax
padStart(targetLength)
padStart(targetLength, padString)
const str1 = '5';
console.log(str1.padStart(2, '0'));
// Expected output: "05"
const fullNumber = '2034399002125581';
const last4Digits = fullNumber.slice(-4);
const maskedNumber = last4Digits.padStart(fullNumber.length, '*');
console.log(maskedNumber);
// Expected output: "************5581"
padEnd()
The padEnd()
method pads the current string with a given string (repeated, if needed) so that the resulting string reaches a given length. The padding is applied from the end of the current string.
Syntax
padEnd(targetLength)
padEnd(targetLength, padString)
const str1 = 'Breaded Mushrooms';
console.log(str1.padEnd(25, '.'));
// Expected output: "Breaded Mushrooms........"
const str2 = '200';
console.log(str2.padEnd(5));
// Expected output: "200 "
match()
The match()
method retrieves the result of matching a string against a regular expression.
Syntax
match(regexp)
const paragraph = 'The quick brown fox jumps over the lazy dog. It barked.';
const regex = /[A-Z]/g;
const found = paragraph.match(regex);
console.log(found);
// Expected output: Array ["T", "I"]
matchAll()
The matchAll()
method returns an iterator of all results matching a string against a regular expression, including capturing groups.
Syntax
matchAll(regexp)
const regexp = /t(e)(st(\d?))/g;
const str = 'test1test2';
const array = [...str.matchAll(regexp)];
console.log(array[0]);
// Expected output: Array ["test1", "e", "st1", "1"]
console.log(array[1]);
// Expected output: Array ["test2", "e", "st2", "2"]
includes()
The includes()
method performs a case-sensitive search to determine whether one string may be found within another string, returning true
or false
as appropriate.
Syntax
includes(searchString)
includes(searchString, position)
const sentence = 'The quick brown fox jumps over the lazy dog.';
const word = 'fox';
console.log(`The word "${word}" ${sentence.includes(word) ? 'is' : 'is not'} in the sentence`);
// Expected output: "The word "fox" is in the sentence"
indexOf()
The indexOf()
method of String
values searches this string and returns the index of the first occurrence of the specified substring. It takes an optional starting position and returns the first occurrence of the specified substring at an index greater than or equal to the specified number.
Syntax
indexOf(searchString)
indexOf(searchString, position)
const paragraph = 'The quick brown fox jumps over the lazy dog. If the dog barked, was it really lazy?';
const searchTerm = 'dog';
const indexOfFirst = paragraph.indexOf(searchTerm);
console.log(`The index of the first "${searchTerm}" from the beginning is ${indexOfFirst}`);
// Expected output: "The index of the first "dog" from the beginning is 40"
console.log(`The index of the 2nd "${searchTerm}" is ${paragraph.indexOf(searchTerm, (indexOfFirst + 1))}`);
// Expected output: "The index of the 2nd "dog" is 52"
charAt()
The charAt()
method of String
values returns a new string consisting of the single UTF-16 code unit located at the specified offset into the string.
Syntax
charAt(index)
const sentence = 'The quick brown fox jumps over the lazy dog.';
const index = 4;
console.log(`The character at index ${index} is ${sentence.charAt(index)}`);
// Expected output: "The character at index 4 is q"
concat()
The concat()
method concatenates the string arguments to the calling string and returns a new string.
Syntax
concat(str1)
concat(str1, str2)
const str1 = 'Hello';
const str2 = 'World';
console.log(str1.concat(' ', str2));
// Expected output: "Hello World"
console.log(str2.concat(', ', str1));
// Expected output: "World, Hello"
endsWith()
The endsWith()
method determines whether a string ends with the characters of a specified string, returning true
or false
as appropriate.
Syntax
endsWith(searchString)
endsWith(searchString, endPosition)
const str1 = 'Cats are the best!';
console.log(str1.endsWith('best!'));
// Expected output: true
console.log(str1.endsWith('best', 17));
// Expected output: true
const str2 = 'Is this a question?';
console.log(str2.endsWith('question'));
// Expected output: false
lastIndexOf()
The lastIndexOf()
method of String
values searches this string and returns the index of the last occurrence of the specified substring. It takes an optional starting position and returns the last occurrence of the specified substring at an index less than or equal to the specified number.
Syntax
lastIndexOf(searchString)
lastIndexOf(searchString, position)
const paragraph = 'The quick brown fox jumps over the lazy dog. If the dog barked, was it really lazy?';
const searchTerm = 'dog';
console.log(`The index of the first "${searchTerm}" from the end is ${paragraph.lastIndexOf(searchTerm)}`);
// Expected output: "The index of the first "dog" from the end is 52"
Subscribe to my newsletter
Read articles from tarunkumar pal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

tarunkumar pal
tarunkumar pal
I'm a recent graduate with a degree in Mechanical Engineering but I have a passion for web development. I have hands on in ReactJS and I have experience building responsive, user-friendly web applications using this framework. I gained hands-on experience in web development through several projects . I'm proficient in HTML, CSS, JavaScript, and I have experience working with various front-end technologies such as ReactJS, Redux, and Bootstrap. I'm also familiar with back-end development such as Node.js and Express.js.