Angular Change Detection Strategy: A Guide
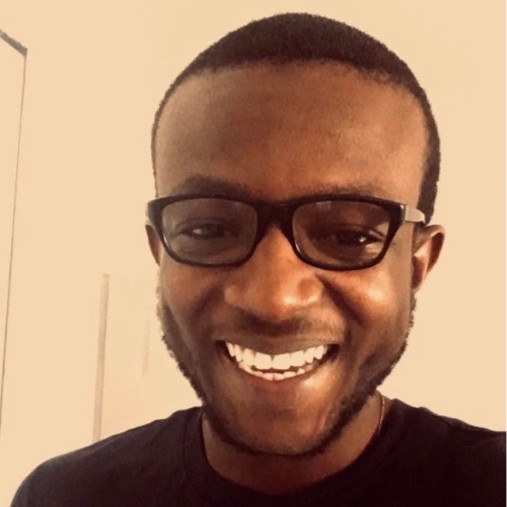
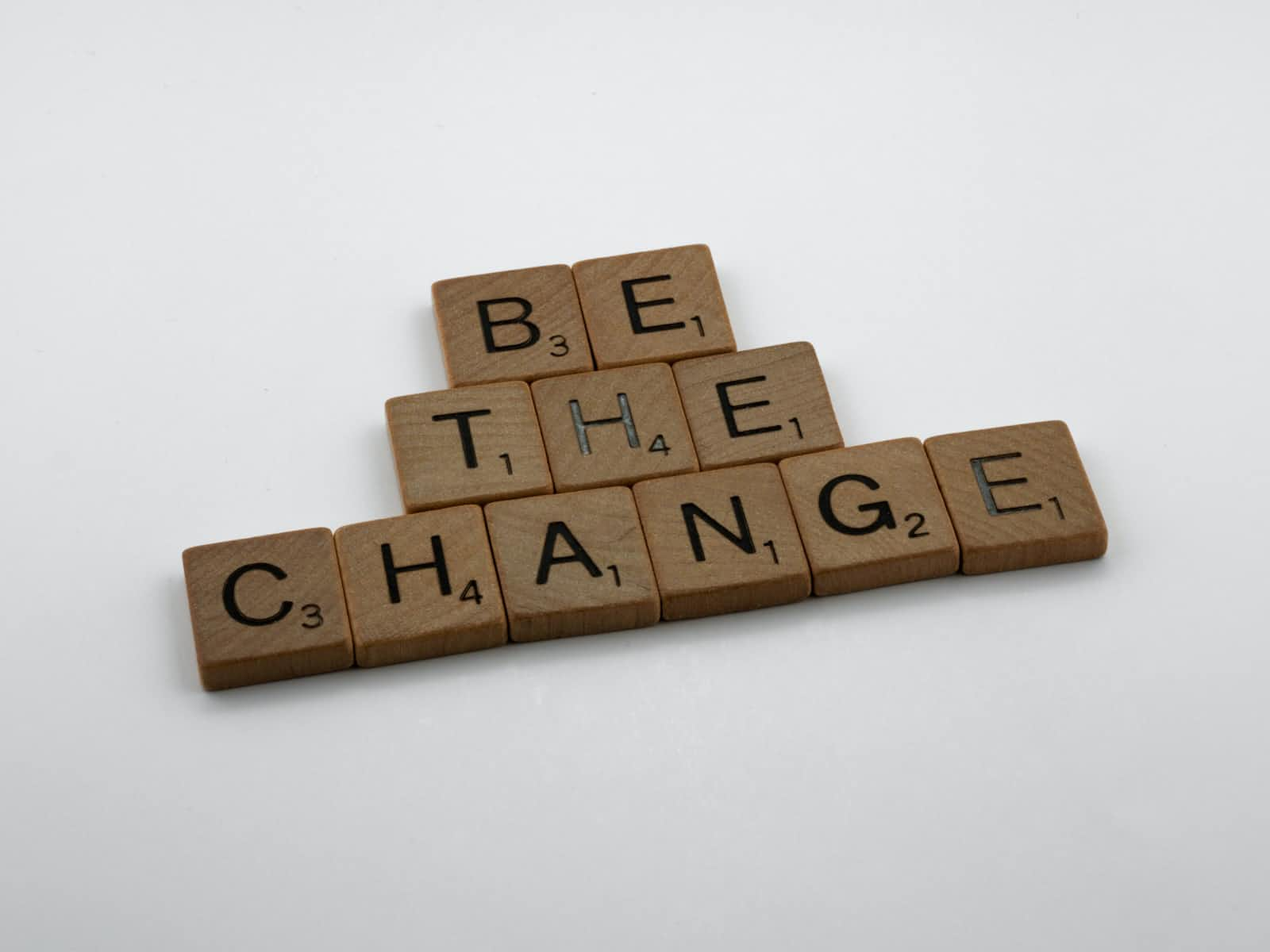
Angular is a powerful framework known for its robustness and ability to facilitate the development of large-scale applications. A key feature of Angular is its Change Detection Strategy, a mechanism that allows Angular to track changes in components and perform updates effectively. This blog post delves into this crucial aspect of Angular, explaining how to implement it and how it contributes to performance optimization.
Understanding Change Detection Strategy
Change detection is the process Angular uses to sync the state of an application view with the state of the components. The Change Detection Strategy controls this synchronization process.
Angular provides four change detection strategies:
Default
OnPush
Detached
CheckOnce
These strategies offer different levels of control over when change detection runs, and can be leveraged to optimize the performance of your application. Let's take a closer look at each one.
Default
By default, Angular uses the Default
strategy. This strategy checks the component and its children for changes during every change detection cycle. Here's an example of a component using the default strategy:
typescriptCopy codeimport { Component } from '@angular/core';
@Component({
selector: 'my-component',
templateUrl: './my-component.component.html',
styleUrls: ['./my-component.component.css'],
// No need to specify 'changeDetection' property as Default is used by default
})
export class MyComponent {
title = 'Hello, Angular';
}
This strategy ensures that the view stays updated, but can be resource-intensive for complex applications.
OnPush
The OnPush
strategy can significantly optimize performance by reducing unnecessary checks. With this strategy, Angular only checks for changes when new references are passed to the component's input properties, or when an event is emitted by the component.
Here's an example of a component using the OnPush
strategy:
typescriptCopy codeimport { Component, ChangeDetectionStrategy } from '@angular/core';
@Component({
selector: 'my-component',
templateUrl: './my-component.component.html',
styleUrls: ['./my-component.component.css'],
changeDetection: ChangeDetectionStrategy.OnPush
})
export class MyComponent {
title = 'Hello, Angular';
}
In this case, the title
property of the component will only trigger a change detection if its reference changes, leading to less frequent updates and better performance.
However, there may be cases where you might want to manually trigger change detection, even when using OnPush
. For example, if you're working with an Observable and you're sure the state has changed, but Angular hasn't detected it due to the OnPush
strategy. In such cases, calling this.cdr.detectChanges();
or this.cdr.markForCheck();
can be useful.
Here's an example of how you might use it:
typescriptCopy codeimport { Component, ChangeDetectionStrategy, ChangeDetectorRef } from '@angular/core';
import { DataService } from './data.service';
@Component({
selector: 'my-component',
templateUrl: './my-component.component.html',
styleUrls: ['./my-component.component.css'],
changeDetection: ChangeDetectionStrategy.OnPush
})
export class MyComponent {
data: any;
constructor(private dataService: DataService, private cdr: ChangeDetectorRef) {}
ngOnInit() {
this.dataService.getData().subscribe(response => {
this.data = response;
this.cdr.detectChanges(); // Manually trigger change detection
});
}
}
In this case, this.cdr.detectChanges();
is used to manually trigger change detection when new data is received from dataService
. This ensures that the view is updated with the new data, even though OnPush
strategy is being used. However, it's good practice to limit the use of detectChanges()
and rely on Angular's built-in mechanisms as much as possible to maintain performance and keep the code clean.
Detached
With the Detached
strategy, the component is entirely detached from Angular's change detection mechanism. Angular won't check for changes in the component or its children, regardless of whether new inputs are received or DOM events are triggered. This strategy can be implemented as follows:
typescriptCopy codeimport { Component, ChangeDetectorRef } from '@angular/core';
@Component({
selector: 'my-component',
templateUrl: './my-component.component.html',
styleUrls: ['./my-component.component.css'],
})
export class MyComponent {
constructor(private cdr: ChangeDetectorRef) {
this.cdr.detach();
}
//...
}
This strategy can be beneficial for components that don't need to be updated or are updated manually using the detectChanges()
method.
CheckOnce
Finally, the CheckOnce
strategy instructs Angular to check the component once and then detaches it. This strategy can be useful for components that are static or rarely change. Here is how to implement it:
typescriptCopy codeimport { Component, ChangeDetectorRef } from '@angular/core';
@Component({
selector: 'my-component',
templateUrl: './my-component.component.html',
styleUrls: ['./my-component.component.css'],
})
export class MyComponent {
constructor(private cdr: ChangeDetectorRef) {}
ngOnInit() {
this.cdr.detectChanges();
this.cdr.detach();
}
//...
}
In this example, detectChanges()
is called manually to trigger change detection, and then detach()
is called to detach the component from future change detection cycles.
Conclusion
The ever-evolving world of Angular's Change Detection Strategy - is as dynamic as the JavaScript ecosystem itself. If we were to compare Angular to a superhero, its change detection strategy would undoubtedly be its secret superpower, allowing it to keep up with changes faster than a speeding bullet. It's as if Angular took the phrase, "Be the change you want to see in the world," quite literally!
Just remember: with great power comes great responsibility. When working with the different strategies, make sure you don't detach when you should push, or default when you should check once. It can sometimes feel like a game of musical chairs, making sure each component has a chair (strategy) that suits its needs when the music (data changes) stops.
In all seriousness, understanding Angular's Change Detection Strategy is crucial for creating efficient and high-performing applications. By getting to grips with the different strategies, you can ensure that your components stay as updated as a teenager on social media, without draining resources like a cat-video marathon on your new 4K screen.
And while we're on the subject of jokes, here's a little developer humor for you:
Why don't Angular developers need to go out in the sunshine?
Because they're already used to a lot of change detection!
(Drumroll, please.)
In all fairness, jokes about change detection are like OnPush
strategy - they only hit when you didn't expect it!
Stay tuned for the next post, where we'll dive deeper into the intricacies of Angular, and who knows, we might even crack some more coding jokes while exploring ways to optimize your application's performance. Until then, keep detecting those changes!
Subscribe to my newsletter
Read articles from Mo' Claudius directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
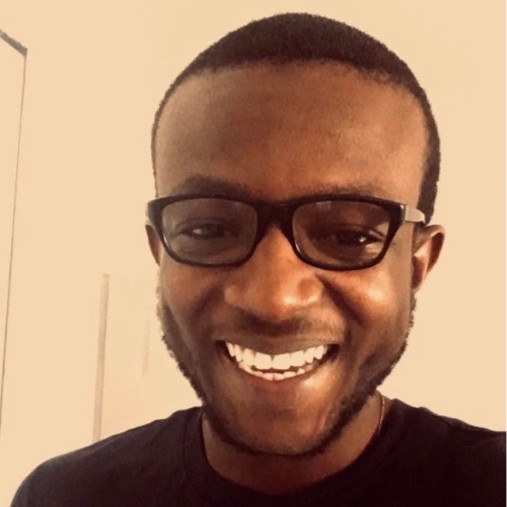
Mo' Claudius
Mo' Claudius
Hi there! I'm Mo' Claudius, a Software Developer with over 9 years of experience specializing in web development. I hold a Master's degree in Software Engineering, and I have a passion for working with Angular and Ruby on Rails. My expertise lies in creating efficient, user-friendly, and scalable web applications. When I'm not coding, I enjoy practicing kickboxing (savate) and dancing (kizomba). These hobbies keep me active, energized, and inspired to bring creativity and innovation to my work. As a member of the Hashnode community, my goal is to share my knowledge and experience with fellow developers in a "knowledge potluck" fashion. I believe that through collaboration and the exchange of ideas, we can all grow and improve in our respective fields. Feel free to connect with me, and let's learn from each other while creating amazing things together!