Demystifying React WYSIWYG Editors: A Comprehensive Setup Guide
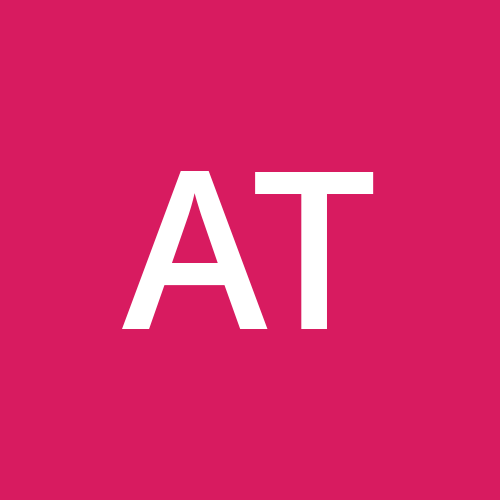
Table of contents
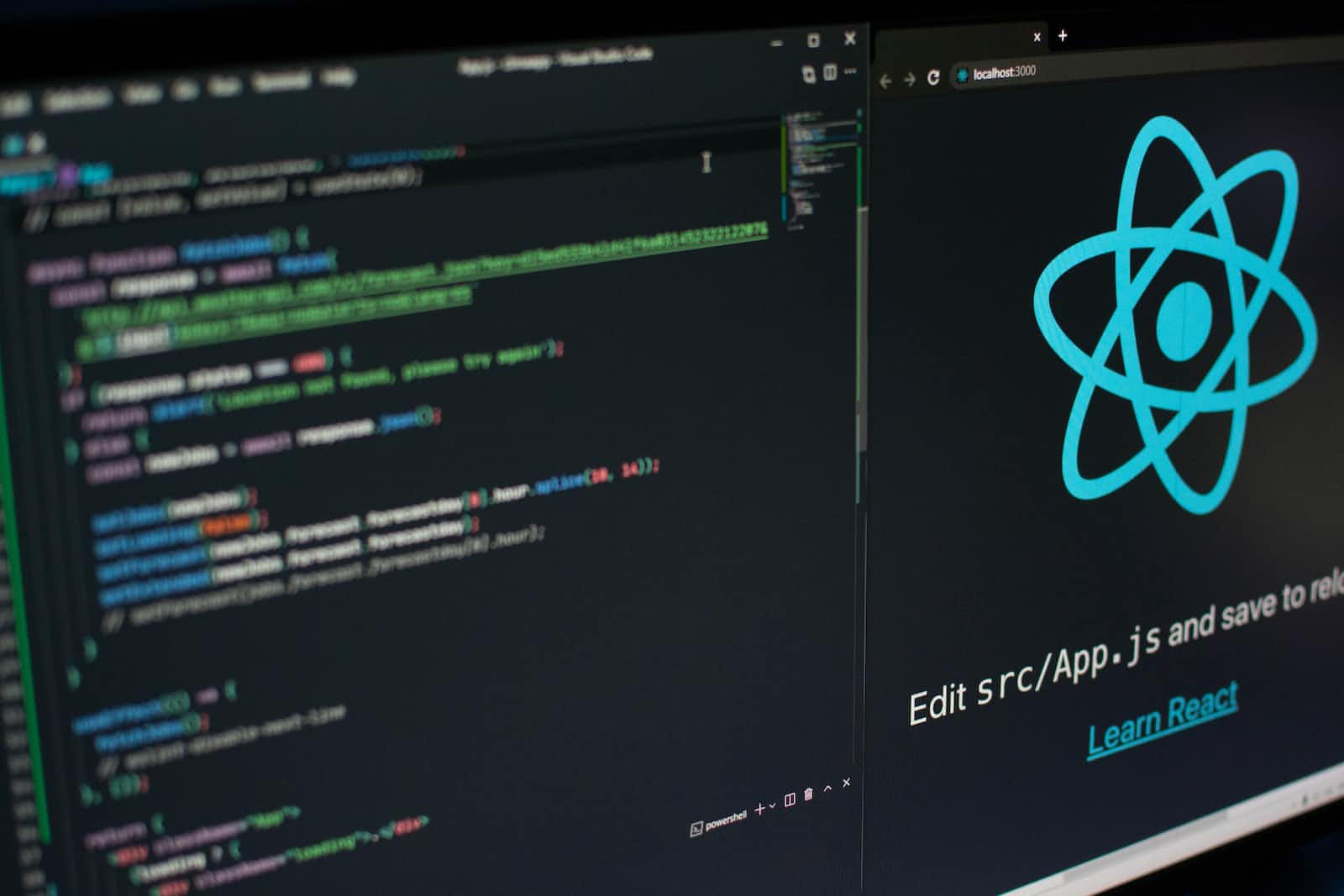
WYSIWYG Editors
They simplify the process of generating HTML markup by providing intuitive tools for applying styles, inserting media, and creating tables. These editors empower users to create visually appealing and well-formatted content without the need for extensive coding knowledge, making them valuable tools in web development and other applications
- offer a user-friendly visual interface for creating and formatting content
here we are taking up React-quill as an example and later exploring more...
source code available below
React-Quill
we can start using this package by running this command npm i react-quill
before installing there are some basic steps to set up your project
npx create-react-app quilleditor-react-demo
cd quilleditor-react-demo
npm i react-quill
that's it you complete the basic steps now we can start our battle.......
create a react-component and import ReactQuill
from react-quilll
there is no need to use useEffect
function, here it is just used for print loading
the output will be
we can add more configurations to this editor by mentioning modules in ReactQuill
const customToolbar = [
["bold", "italic", "underline", "strike"], // toggled buttons
["blockquote", "code-block"],
[{ header: 1 }, { header: 2 }], // custom button values
[{ list: "ordered" }, { list: "bullet" }],
[{ script: "sub" }, { script: "super" }], // superscript/subscript
[{ indent: "-1" }, { indent: "+1" }], // outdent/indent
[{ direction: "rtl" }], // text direction
[{ size: ["small", false, "large", "huge"] }], // custom dropdown
[{ header: [1, 2, 3, 4, 5, 6, false] }],
[{ color: [] }, { background: [] }], // dropdown with defaults from theme
[{ font: [] }],
[{ align: [] }],
["clean"], // remove formatting button
];
pass customToolbar value into ReactQuill
<div style={{ height: "135px", width: "800px" }}>
<ReactQuill theme="snow" style={{ height: "100px" }} value= {value} onChange={editorClick} modules={{ toolbar: customToolbar }} />;
</div>
the output will be...
there are a lot more configurations available for Explore here
TinyMCE
TinyMCE is another rich-text editor that allows users to create formatted content within a user-friendly interface.
we need to follow the same steps above mentioned in react-quill, only step 3 is different
npx create-react-app tinyeditor-react-demo
cd tinyeditor-react-demo
npm install --save @tinymce/tinymce-react
create a react-component named editor2.js
in react app and import it into app.js
in editor2.js
file we need to import the Editor
from @tinymce/tinymce-react
import { Editor } from "@tinymce/tinymce-react";
here we are using useRef (
useRef is a React Hook that provides a way to store and access mutable values that persist across renders. It returns a mutable ref object that can hold any value and does not trigger component re-rendering when its value changes. It is commonly used to access and modify DOM elements, manage focus, or preserve values between function calls.)
for values from the message box, useState
for storing values
In addition, we also need an API key for using this tinymce editor. This API key can be found in our account. We just need to signup for the TinyMCE cloud
let's look into the code
import React, { useRef, useState } from "react";
import { Editor } from "@tinymce/tinymce-react";
export default function Editor1() {
const [value, setValue] = useState("<p>This is the initial content of the editor.</p>");
const editorRef = useRef(null);
const log = () => {
if (editorRef.current) {
console.log(editorRef.current.getContent());
setValue(editorRef.current.getContent())
}
};
return (
<>
<h4>TINY MCE editor</h4>
<div style={{ height: "100px", width: "800px " }}>
<Editor
apiKey="eusbo0bxj4wuotu447kvqx1qx0x5ipf1cgze3rztbsxcyu7a"
onInit={(evt, editor) => (editorRef.current = editor)}
initialValue={value}
init={{
height: 200,
menubar: false,
plugins: [
"advlist autolink lists link image charmap print preview anchor",
"searchreplace visualblocks code fullscreen",
"insertdatetime media table paste code help wordcount",
],
toolbar:
"undo redo | formatselect | " +
"bold italic backcolor | alignleft aligncenter " +
"alignright alignjustify | bullist numlist outdent indent | " +
"removeformat | help",
content_style: "body { font-family:Helvetica,Arial,sans-serif; font-size:14px }",
}}
/>
<button onClick={log}>Log editor content</button>
</div>
</>
);
}
Import the necessary dependencies:
React
,useRef
,useState
, andEditor
from the@tinymce/tinymce-react
package.Define the
Editor1
component as a default function component.Initialize a state variable
value
using theuseState
hook. This state variable will hold the initial content of the editor and will be updated when the editor's content changes.Create a
ref
using theuseRef
hook and assign it to theeditorRef
constant. Thisref
will be used to reference the TinyMCE editor instance.Define a
log
function that logs the current content of the editor to the console. It accesses the editor content through theeditorRef.current
reference and updates thevalue
state with the current content.Inside the component's return statement, render the heading and a
<div>
element that wraps the TinyMCE editor.Configure the TinyMCE editor by providing necessary props:
apiKey
: Your unique TinyMCE API key for authentication.onInit
: A callback function that assigns the editor instance to theeditorRef.current
reference when the editor is initialized.initialValue
: The initial content of the editor.init
: An object containing various configuration options for the editor, such as height, plugins, toolbar options, and content styles.
Render a
<button>
element that triggers thelog
function when clicked.
above code demonstrates how to integrate and configure the TinyMCE editor in a React component, access its content using a ref
, and update the state based on the editor's content changes.
output will be
there are lot more configration available here
there are plenty of package available for this same purpose
Draft.js: Draft.js is a powerful and flexible framework for building rich text editors in React. It provides a set of customizable components and an extensive API for managing the editor state and rendering content.
CKEditor: CKEditor is a widely used WYSIWYG editor that offers a comprehensive set of features and a user-friendly interface. It supports plugins, content filtering, image handling, and many other advanced editing capabilities.
React-draft-wysiwyg: React-draft-wysiwyg is a wrapper component built on top of Draft.js. It provides a set of pre-designed toolbar options and allows for easy integration of Draft.js in a React application.
Slate.js: Slate.js is a powerful and customizable framework for building rich text editors. It offers a flexible data model, allowing you to define your own document structure and behavior. It also provides an extensive set of plugins and a robust API for handling editor operations.
React-Summernote: React-Summernote is a wrapper component for Summernote, a feature-rich WYSIWYG editor. It provides a simple integration with React and supports a wide range of editing options, including text formatting, media embedding, and code highlighting.
React-MDE: React-MDE is a Markdown editor built specifically for React applications. It provides a live preview of the rendered Markdown content and supports features like syntax highlighting, image uploading, and custom toolbar options.
SunEditor: SunEditor is a lightweight and customizable WYSIWYG editor for React. It offers a clean and intuitive interface, supports various formatting options, and provides plugins for additional functionality.
CONCLUTION
Overall, WYSIWYG editors empower developers and content creators to build dynamic and engaging web experiences by providing a user-friendly interface for editing rich text content. Whether it's for blog posts, documentation, or other content-driven applications, WYSIWYG editors play a vital role in enhancing the productivity and creativity of users.
EXPRESS YOUR THOUGHTS AND DONT HESITATE TO CONTACT ME IF YOU HAVE ANY DOUBT
SOURCE CODE OF BOTH EDITOR here
Subscribe to my newsletter
Read articles from Ashin Thankachan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
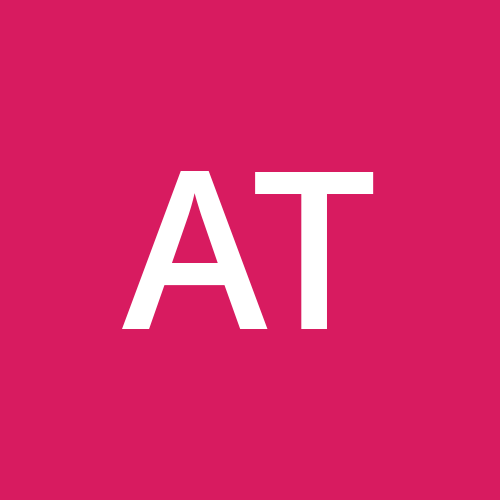