Apache Beam Introduction

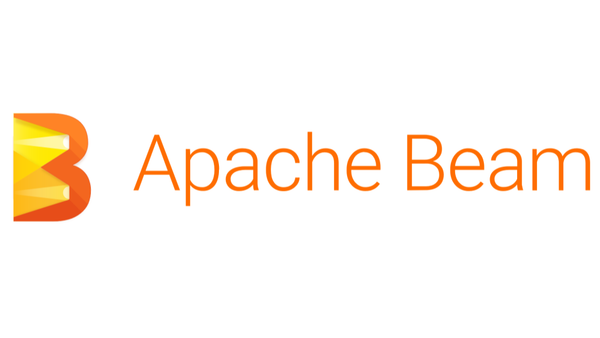
Apache Beam (Batch + strEAM) is an open-source, unified programming model for processing and analyzing large-scale data sets. It provides a simple and expressive way to implement data processing pipelines that can run on various distributed processing backends, such as Apache Flink, Apache Spark, and Google Cloud Dataflow.
Key Features of Apache Beam
1. Portability: Beam pipelines are designed to be vendor-agnostic, allowing them to run on multiple execution engines. This portability enables you to write your pipelines once and execute them on different distributed processing backends, such as Apache Flink, Apache Spark, and Google Cloud Dataflow.
2. Scalability: Apache Beam leverages the scalability of distributed processing frameworks to handle massive data sets. It can seamlessly scale your pipelines across a cluster of machines, enabling efficient processing of large volumes of data in parallel.
3. Flexibility: Beam supports both batch and streaming data processing, making it a versatile choice for building real-time and batch pipelines. You can implement continuous streaming applications as well as perform batch-processing tasks using the same programming model and APIs.
4. Extensibility: Apache Beam provides a rich set of connectors and transformations, making it easy to integrate with various data sources and sinks. Additionally, you can extend Beam by creating custom transformations tailored to your specific data processing requirements.
5. Fault Tolerance: Apache Beam incorporates built-in fault tolerance mechanisms to ensure reliable data processing. It provides automatic checkpointing and state management, allowing pipelines to recover from failures and continue processing without data loss. This feature enhances the reliability and robustness of your data pipelines.
6. Language-agnostic: Apache Beam offers language-agnostic APIs, allowing you to write pipelines in multiple programming languages, including Java, Python, and Go. This language-agnostic approach provides flexibility and enables teams to use their preferred programming languages while collaborating on data-processing projects. It also promotes code reuse and interoperability across different language ecosystems.
7. Unified Programming Model: Apache Beam provides a unified programming model that allows you to express your data processing logic consistently, regardless of the underlying execution engine. This model is based on the concept of data transformations, where you define a series of operations on your data to transform it from input to output. The unified API abstracts away the complexities of different execution engines, making it easier to write portable and reusable data processing pipelines. With Apache Beam, you can focus on the logic of your data transformations rather than the intricacies of the execution environment.
8. Open-Source Community: Apache Beam is an open-source project with a vibrant and active community. This means that the framework is constantly evolving, benefiting from contributions and improvements from a diverse group of developers. The open-source nature of Apache Beam ensures transparency, fosters innovation and allows users to participate in the development and enhancement of the framework. The community provides support through forums, mailing lists, and regular updates, making it easier to learn, troubleshoot, and collaborate with other users.
Example: Word Count Pipeline in Apache Beam (Java)
In this example, we create a pipeline that reads input from a text file, splits the lines into individual words by white space, counts the occurrences of each word, and writes the results to an output file.
import org.apache.beam.sdk.Pipeline;
import org.apache.beam.sdk.io.TextIO;
import org.apache.beam.sdk.options.PipelineOptions;
import org.apache.beam.sdk.options.PipelineOptionsFactory;
import org.apache.beam.sdk.transforms.Count;
import org.apache.beam.sdk.transforms.FlatMapElements;
import org.apache.beam.sdk.transforms.SerializableFunction;
import org.apache.beam.sdk.values.KV;
import org.apache.beam.sdk.values.PCollection;
import org.apache.beam.sdk.values.TypeDescriptors;
import java.util.Arrays;
public class WordCountPipeline {
public static void main(String[] args) {
// Create a pipeline options instance
PipelineOptions options = PipelineOptionsFactory.create();
// Create a pipeline
Pipeline pipeline = Pipeline.create(options);
// Step 1: Read input text file
PCollection<String> lines = pipeline.apply(TextIO.read().from("input.txt"));
// Step 2: Split each line into individual words
PCollection<String> words = lines.apply(
FlatMapElements.into(TypeDescriptors.strings())
.via((SerializableFunction<String, Iterable<String>>) line -> Arrays.asList(line.split("\\s"))));
// Step 3: Count the occurrences of each word
PCollection<KV<String, Long>> wordCounts = words.apply(Count.perElement());
// Step 4: Write the results to an output text file
wordCounts.apply("WriteCounts", TextIO.write().to("output.txt"));
// Run the pipeline
pipeline.run().waitUntilFinish();
}
}
Explanation of Each Step:
Step 1: Read input text file: In this step, the pipeline reads the content of an input text file using
TextIO.read
().from("input.txt")
. Thefrom()
method specifies the path or pattern of the input file(s). The result is aPCollection<String>
represents a collection of lines from the input file.Step 2: Split each line into individual words: The
FlatMapElements
transformation is applied to the linesPCollection
. It takes each line as input and outputs multiple elements, where each element represents a word from the line. The transformation uses a lambda function to split each line into words by splitting on whitespaces (\\s
). The result is aPCollection<String>
containing individual words.Step 3: Count the occurrences of each word: The
Count.perElement()
transformation is applied to the wordsPCollection
. It counts the occurrences of each word and produces aPCollection<KV<String, Long>>
, where each element represents a word and its corresponding count.Step 4: Write the results to an output text file: The
TextIO.write().to("output.txt")
writes the word count results to an output text file. Theto()
method specifies the path or pattern of the output file(s). Theapply()
method with the"WriteCounts"
argument is used to label this specific transform in the pipeline graph.Run the pipeline: The pipeline is executed by calling
pipeline.run
().waitUntilFinish()
. This triggers the execution of all the defined transformations in the pipeline and waits until the pipeline completes.
Conclusion
In a world driven by data, Apache Beam emerges as a game-changer, offering a unified, scalable, and flexible framework for processing large-scale data sets. With its powerful features and a vibrant community, Apache Beam opens up a realm of possibilities for developers and data enthusiasts alike. Embrace Apache Beam, and unlock the true potential of your data-driven ambitions.
Get ready for an exhilarating journey into the world of data processing! In our upcoming blog, we dive into the fascinating realm of Apache Beam, where we demystify essential terminologies and guide you through the exhilarating process of building your very first data pipeline. Stay tuned for a hands-on experience that will empower you to unleash the full potential of your data-driven endeavours. The adventure begins in our next blog!
Subscribe to my newsletter
Read articles from Syed Sarfaraz Ahammed directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Syed Sarfaraz Ahammed
Syed Sarfaraz Ahammed
I am passionate about leveraging cutting-edge technologies and advanced algorithms to unravel complex data challenges. Committed to building scalable and efficient solutions that empower businesses to harness the full potential of their data. Dedicated to continuous learning and staying at the forefront of data engineering to drive innovation and deliver exceptional results.