Mastering JavaScript Array and it methods
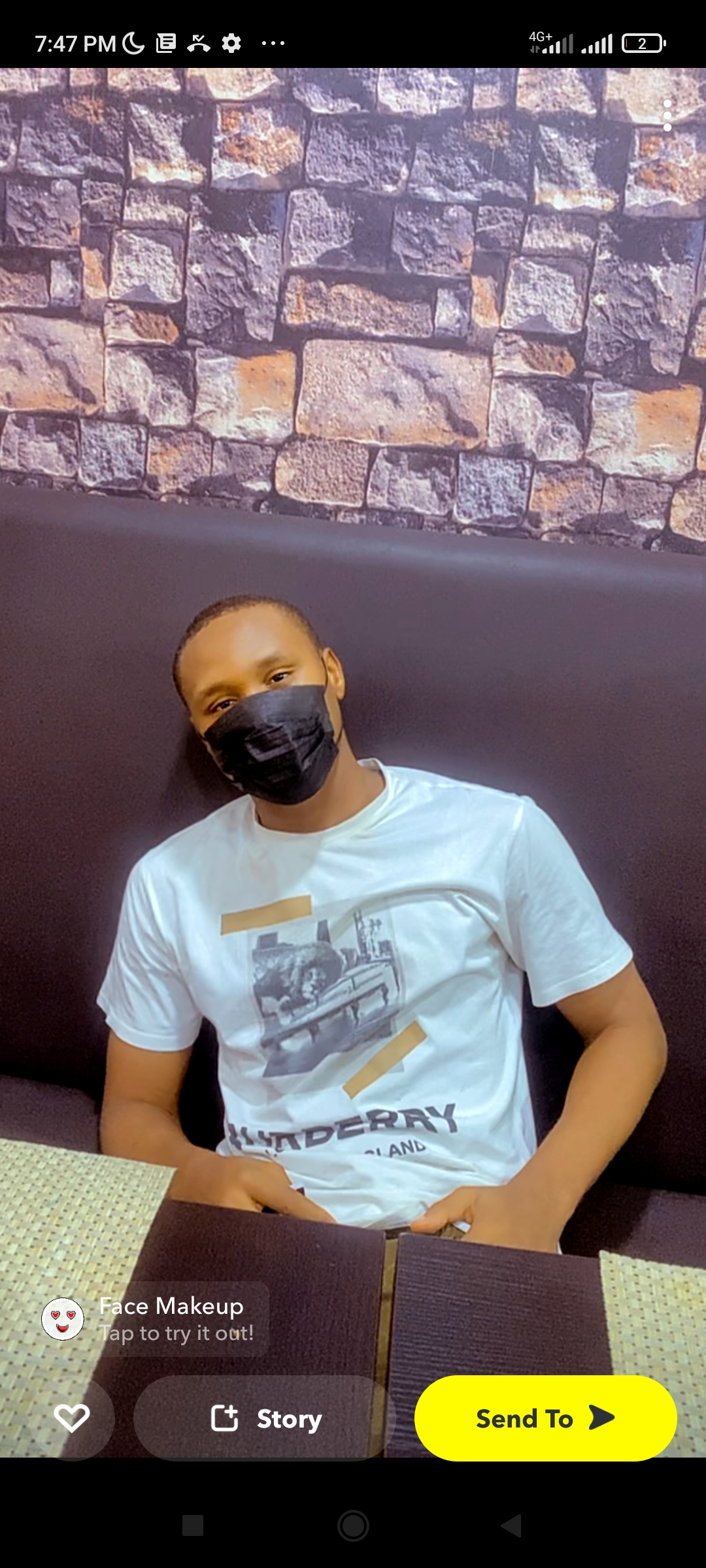
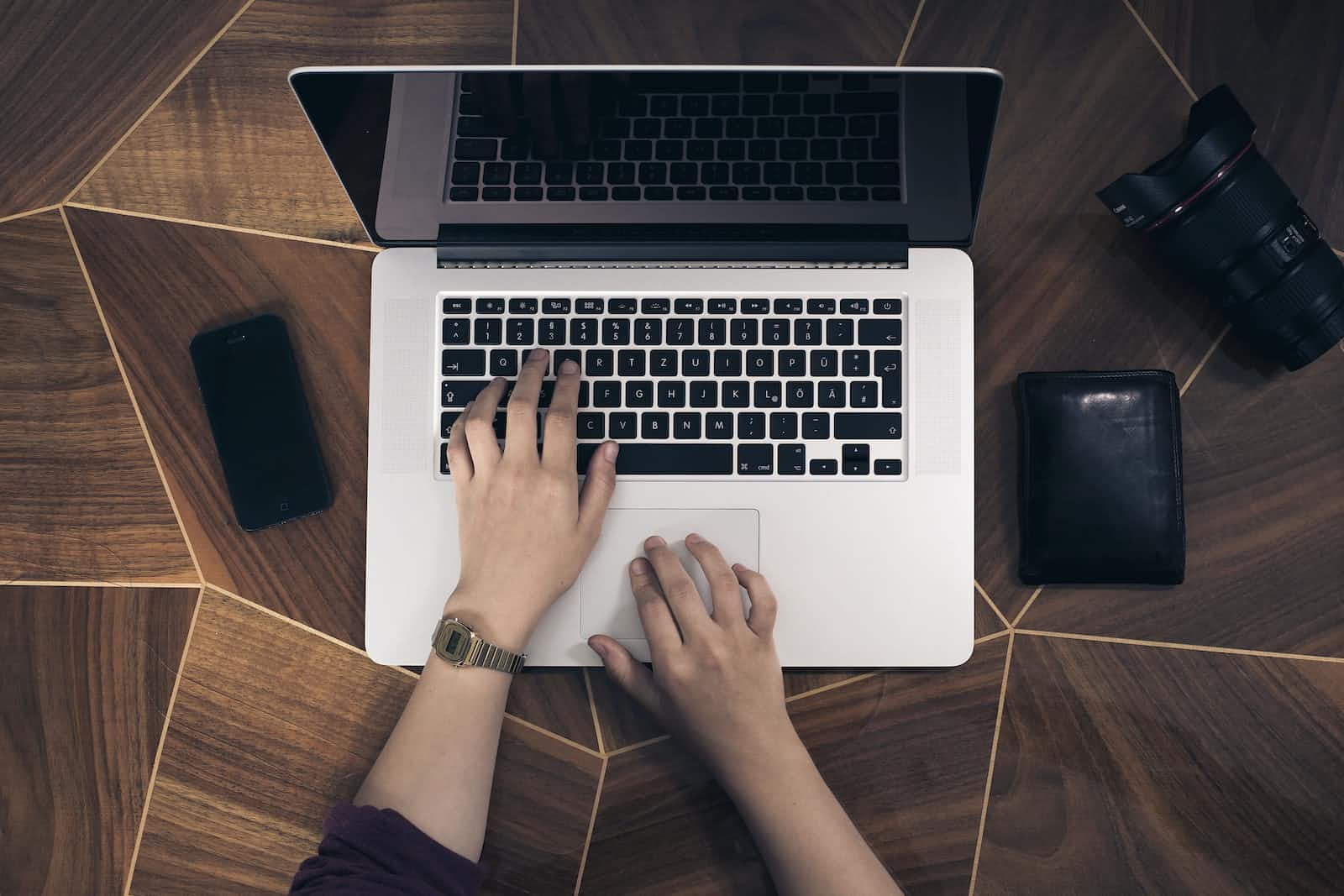
Arrays are variables that can hold multiple values, in this article, I will be discussing javascript array and it methods.
const myArray = ["Nigeria", "USA", "Canada", "Benin"]
The code above is an example of how an array looks like in javascript.
Congratulations, we just wrote our first array variable in javascript.
Why Arrays in javascript ?
we use an array in javascript in other to reduce the amount of repeated code in our program, instead of saving each value in a separate variable, we can just put them into an array.
Now let's focus on how we can manipulate our array to get our desired outcome.
In javascript, there are several methods we can use in manipulating arrays, these are well-known as array methods
,however, without these methods, we can't work with arrays.
Array methods.
We have multiple arrays method in javascript, and I will explain each of them in this article.
Array slice ()
An array slice is used to select an element in an array and store it in a new array, however, the original array remains the same, unlike the pop and push method.
syntax:
array.slice(first, end).
Example:
cont myArray = const myArray = ["Nigeria", "USA", "Canada", "Benin"]
const newArray = myArray.slice(0, 1)
console.log(newArray) // this return in the console "Nigeria", "USA"
Code Explanation:
In our code above, we created an array that we called myArray
, then we use the slice method to select some elements in myArray
, which was the first and second value on myArray
element. in javascript, if we are to target a value or element in an array, we use number type
to get the index
of the element we are targeting, which means an index of 0
is the first element in myArray
and also index of 1
is the second element in myArray.
As we all know we do not count from an index of 1 in javascript, we start counting from an index of 0. Inconclusion, we then save the selected element in a new variable called newArray.
Array splice()
The array splice method removes or adds an element in an array and returns the element if any.
syntax:
array.splice(index, how many, item1, ....., itemX).
Example:
const myArray = ["Nigeria", "USA", "Canada", "Benin"];
myArray.splice(3, 1, "Spain", "zimbabwe")
console.log(myArray)// this returns "Nigeria", "USA", "Canada", "Spain", "zimbabwe"
Code Explanation:
In our example above we created an array, then we use the splice method to start adding more countries at the index of 3
, the 1
inside the splice object is the number of countries we want to remove to add our new countries, lastly, we added the countries we wanted to add inside our array.
Array sort ()
This method is used for sorting or organizing an array in a descending or ascending order.
syntax:
array.sort(compare function)
Example:
//sorting in ascending order
const myArray = ["Nigeria", "USA ", "Canada", "Spain", "Zimbabwe"];
myArray.sort(function (a, b){ return a-b});
console.log (myArray)// this returns "Canada", "Nigeria", "Spain", "USA", "Zimbabwe"
//sorting in descending order
const myArray = ["Nigeria", "USA ", "Canada", "Spain", "Zimbabwe"];
myArray.sort(function (a, b){ return b-a});
console.log (myArray)// this returns "Zimbabwe", "USA", "Spain", "Nigeria", "Canada"
Code Explanation:
In the code above, we created an array of countries, then we use the sort method to organize the code, we were able to achieve this using a nested function using it to compare our values. kindly take note, if you are sorting a number it targets the smallest to the biggest and vice versa, but if you are sorting a string it targets the first later not all the characters in the string. Finally, there are many ways to compare our codes to give us the same result, in this example I just decided to use the nested function technique.
Array shift() and unshift()
Now let's look into the shift
and unshift
method, we can use the shift
method to completely remove the first element in our array.
we can store the value which was removed entirely from our original array in a new array variable, if necessary.
The unshift
method is used to add elements at the beginning of the original array.
syntax:
array.shift()
array.unshift(item1, item2, ..., itemX)
Example:
const myArray = ["Nigeria", "USA ", "Canada", "Spain", "Zimbabwe"];
myArray.shift()
console.log(myArray)// this returns "USA ", "Canada", "Spain", "Zimbabwe"
//using the unshift method
myArray.unshift("Ethiopia")
console.log(myArray)//This return the "Ethiopia" into our array. "Ethiopia" "USA ", "Canada", "Spain", "Zimbabwe"
Code Explanation:
Using the .shift
and .unshift
methods is very simple because, we do not need to do any complex solution to get our desired outcome, we just have to use the .shift
or the .unshift
syntax on our array.
The .unshift
syntax added an element to the start of our original array and the .shift
element removed the first element.
Array pop() and array push()
This array method plays almost a small role as the shift
and Unshift
method. However, the array.pop()
method removes the last element from an array, while the array.push()
method can be used to add new elements to an array.
syntax :
array.pop()
array.push(item1, item2, ..., itemX)
Example:
const myArray = ["Nigeria", "USA ", "Canada", "Spain", "Zimbabwe"];
myArray.pop();
console.log(myArray)//This returns "Nigeria", "USA ", "Canada", "Spain".
//using the push method.
const myArray = ["Nigeria", "USA ", "Canada", "Spain"];
myArray.push("Ethiopia");
console.log(myArray)// This returns "Nigeria", "USA ", "Canada", "Spain", "Ethiopia".
Array delete()
Array.delete()
can be used to delete an element in an array, but I will advise you not to use this method, because, it leaves an undefined hole in your code, instead, you can use the pop
method to delete an element in an array.
syntax:
Array.delete()
Example:
const myCities = [ "Lagos", "Sacramento", "Tokyo", "Capetown", "Kampala"];
myCities.delete();
console.log(myCities);
Array join()
Array join('')
joins all elements together that are been separated by commas or any specific separators in an Array.
syntax:
array.join(separator)
Example:
const myCities = [ "Lagos", "Sacramento", "Tokyo", "Capetown", "Kampala"];
console.log(myCities.join('')); // this returns "LagosSacramentoTokyoCapetownKampala".
Array concaat().
This method joins two or more arrays together, moreover, it works just like the + operator.
syntax:
array1.concat(array2, array3, ..., arrayX)
Example:
const myCities1 = [ "Lagos", "Sacramento", "Tokyo", "Capetown", "Kampala"];
const myCities2 = ["Texas", "Addis Ababa", "Accra", "Cotonou"];
myCities1.concaat(myCities2)// this return "Lagos", "Sacramento", "Tokyo", "Capetown", "Kampala", "Texas", "Addis Ababa", "Accra", "Cotonou"
Code Explanation:
In the code above, we created our first array "myCities1"
and also created another array, which was called "myCities2"
, we then call for the concaat method on myCities1
, and later, we added "myCities2"
to the parenthesis of our concaat method, so this resulted in the first and second arrays joining together to form an array.
Array toString().
Array tostring()
works on turning all the string-type elements in an array into one string type.
syntax:
array.toString()
Example:
const = myCities ["Lagos", "Sacramento", "Tokyo", "Capetown", "Kampala"];
newArray = myCities.toString();
console.log(newArray)//This returns Lagos Sacramento Tokyo Capetown Kampala
Code Explanation.
we created an array in the code above, after that, we created a new variable called newArray, and then we assigned it to the original array "myCities
" and then called the toString()
method on myCities
, finally, we log newArray to our console and get our desired result.
Array length
The length method checks for the length of an array element and returns the actual number of elements in an array.
syntax:
array.length
Example:
const = myCities ["Lagos", "Sacramento", "Tokyo", "Capetown", "Kampala"];
newArray = myCities.length;
console.log(newArray)// 5
Bonus example:
const = myCities ["Lagos", "Sacramento", "Tokyo", "Capetown", "Kampala"];
myCities.length = 3
console.log(myCities)//This returns "Lagos", "Sacramento", "Tokyo".
Code Explanation:
This is a very simple code, we started by creating a new array, afterward, we also created a new array and assign it to our original array "mycities"
and called for the length method, after that, we console log newArray
, which resulted in 5
because the length of our myCities
array is 5
.
In our second example, myCities.length
return "Lagos", "Sacramento", and "Tokyo"
, because, we assigned myCities.length
to get the length of 3 elements.
Array flat()
Array flat methods are used to concatenate sub-arrays elements.
syntax:
array.flat()
Example:
const myArray = [[1,2], [3,4], [5,6]]
newArray = myArray.flat()
console.log(newArray)// This returns 1,2,3,4,5,6
Explanation:
The code above takes the nested array [[1,2], [3,4], [5,6]]
and transforms it into a single-dimensional array [1, 2, 3, 4, 5, 6]
by flattening it using the flat()
method.
we have come to the end of this article, I hope you understand all concept that was explained in this article, as we all know, the array method is a very great tool to use while manipulating arrays. in this article, we looked at the frequently used array methods. Please note, there are more array methods in javascript that we didn't look into, you can read more on array methods here https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array
Subscribe to my newsletter
Read articles from Abanobi Emmanuel directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
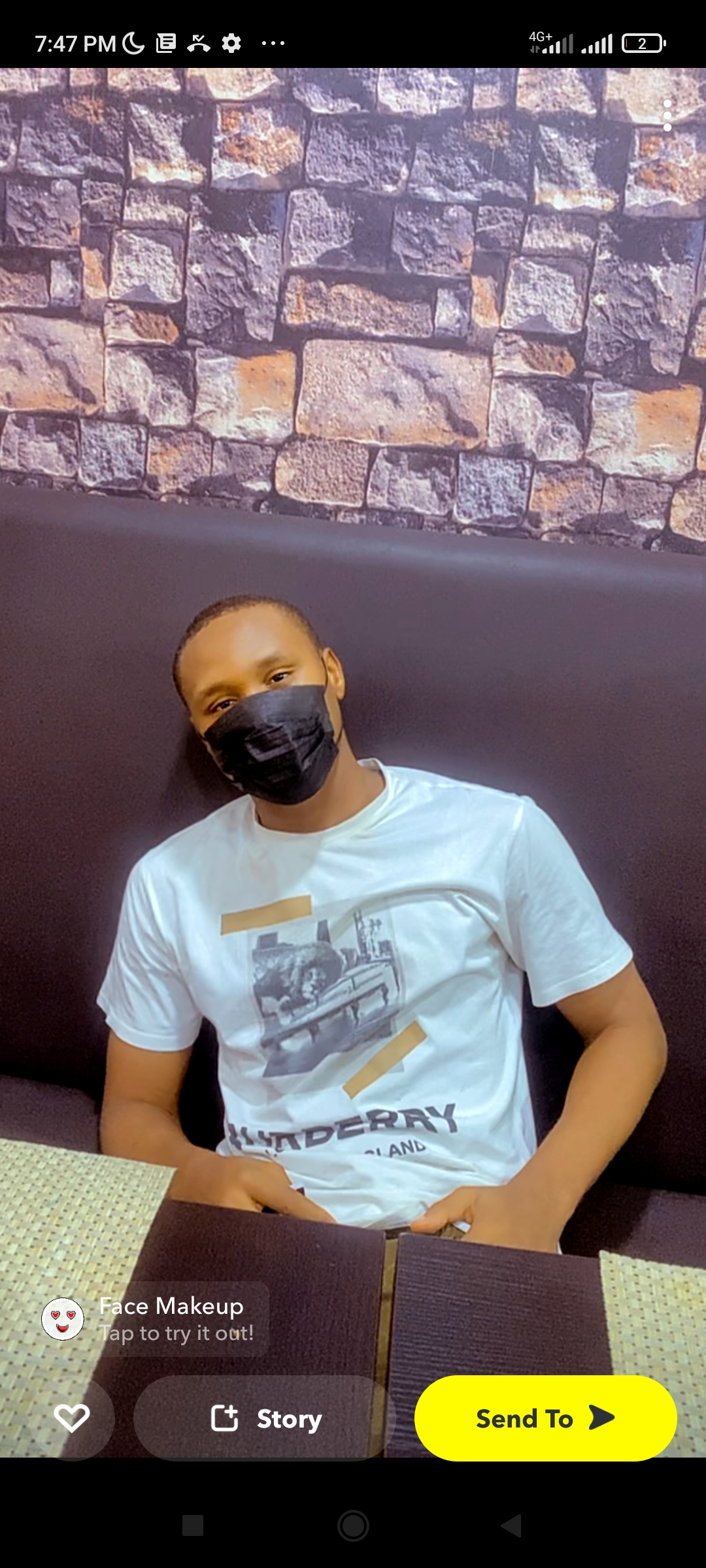
Abanobi Emmanuel
Abanobi Emmanuel
I am a skilled software engineer and technical writer with extensive experience in the field of computer science. With a passion for technology and a drive to stay up-to-date with the latest trend.