Annotation in Java & Spring Boot.
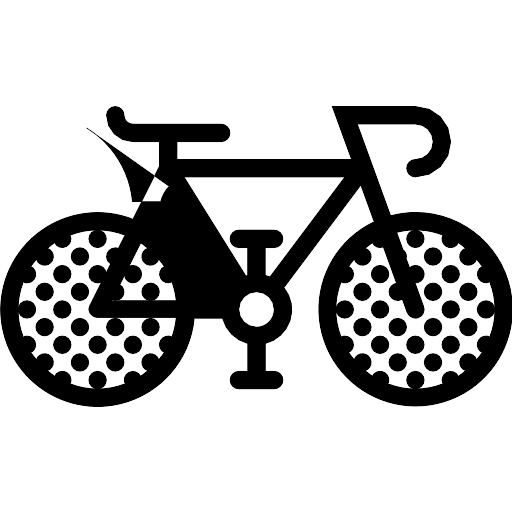
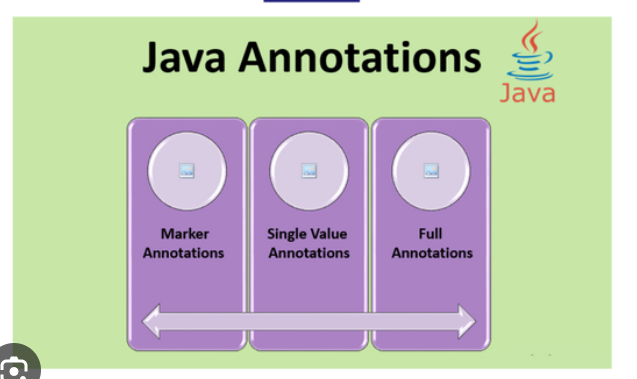
Contents of Article:
1.Definition of annotation.
2.Types of Annotation.
3.Built in Annotation vs Custom Annotation.
4.Examples of annotation used in Java And Spring Boot .
Annotation Definition: An Annotation is a form of metadata that can be added to Java code elements such as classes, methods, variables, and parameters.Annotations provide additional information about the code elements or modify their behavior.
They are used to convey instructions or configuration settings to the compiler, runtime.Embedding an extra (supplement) info to our source code act like an indication to the compiler, and developer that we added some supplement to our code.
Previously we used to have xml configuration but we can say annotation is a replacement of long xml files .
There are two types of annotation in java .
1)In built Annotation .
Examples : @Overrid,@Deprecated,@Bean etc
1.1)Marker Annotation
An annotation that has no method, is called marker annotation. For example:
@interface MarkerAnnotation{}
1.2)Single Value-Annotation :
An annotation that has one method, is called single-value annotation.
@interface SingleValueAnnotation
int value();
}
1.3)Multi-Value Annotation
An annotation that has more than one method, is called Multi-Value annotation.Inside a interface there will be number of methods.
@interface { MultipleValueAnnotation
int value1();
String value2();
String value3();
}
}
2)User defined annotation .
In this simple java code i created a simple coustom annotation :
package test;
import java.lang.annotation.Annotation;
import java.lang.annotation.ElementType;
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
import java.lang.annotation.Target;
//Simple functional Interface ->A interface which have only one abstract method is called functional interface .
interface Demo {
void display();
}
@Target({ElementType.TYPE,ElementType.FIELD,ElementType.METHOD})
@Retention(RetentionPolicy.RUNTIME)
@interface CricketPlayer {
int age() default 34;
String country() default "india";
}
@CricketPlayer
class Virat {
@CricketPlayer
int runs;
int innings;
public int getRuns() {
return runs;
}
public void setRuns(int runs) {
this.runs = runs;
}
public int getInnings() {
return innings;
}
public void setInnings(int innings) {
this.innings = innings;
}
}
public class AnnotationTest {
public static void main(String[] args) {
//Implementing functional interface using lamda expression .
Demo d= () -> {
System.out.println("This is functional interface");
};
Virat v = new Virat();
v.setRuns(1500);
v.setInnings(250);
System.out.println(v.getInnings());
System.out.println(v.getRuns());
System.out.println("Annotation values are fetched");
Class c = v.getClass();
//Down Casting .
Annotation an =c.getAnnotation(CricketPlayer.class);
CricketPlayer cp = (CricketPlayer)an;
System.out.println("AGE" +cp.age());
System.out.println("Country"+cp.country());
}
}
I am going to mention some important annotation that we generally use in our java or spring boot project .
Before getting started with type of annotation i would like to let you know that there is some pre defined access for In build annotation in javawhich java teams already fixed while developing the annotation .
a)Target: @Target Annotation is used to specify that the annotation can be applied to wether to a class or method or variables etc .
@Target({ElementType.TYPE,ElementType.FIELD,ElementType.METHOD})
ElementType.TYPE
: Annotations can be applied to classes, interfaces, and enums.ElementType.METHOD
: Annotations can be applied to methods.ElementType.FIELD
: Annotations can be applied to fields (instance variables).ElementType.PARAMETER
: Annotations can be applied to method parameters.ElementType.CONSTRUCTOR
: Annotations can be applied to constructors.ElementType.LOCAL_VARIABLE
: Annotations can be applied to local variables.ElementType.ANNOTATION_TYPE
: Annotations can be applied to other annotations.ElementType.PACKAGE
: Annotations can be applied to packages.
b)@Retention: The @Retention
annotation is itself a meta-annotation, used to annotate other annotations. It takes a single parameter called value
, which specifies the retention policy as one of the RetentionPolicy
enum values.
@Retention(RetentionPolicy.RUNTIME)
The RetentionPolicy
enum provides the following retention policies:
RetentionPolicy.SOURCE
: Annotations are discarded by the compiler and are not included in the compiled bytecode. They are only available during the compilation process and are not accessible at runtime.RetentionPolicy.CLASS
: Annotations are included in the compiled bytecode, but they are not accessible at runtime. This is the default retention policy if@Retention
is not specified.RetentionPolicy.RUNTIME
: Annotations are included in the compiled bytecode and are accessible at runtime. They can be accessed and processed using reflection at runtime.
1.@Deprecated => @Deprecated
annotation is used to mark the class,Method as deprecated. This means that the class,Method is no longer recommended for use and might be removed in future versions.
2.@Override: Tthe @Override
annotation is used to indicate that the annotated method overrides a method from a superclass or implements an interface method. This annotation helps catch errors at compile-time if the annotated method does not actually override any method.
3.@Bean : This is the mostly asked interview Question that what is difference between @Autowire and @Bean.
@Configuration
public Class MyTest {
@Bean
@LoadBalanced
public RestTemplate restTemplate() {
return new RestTemplate();
}
@Bean
public ModelMapper modelMapper() {
return new ModelMapper();
}
3.a).@Bean is used to indicate that a method in a Spring configuration class produces a bean that should be managed by the Spring container.
3.b).@Bean
tells Spring 'Here is an instance of this class, please keep hold of it and give it back to me when I ask'.
3.c)@Autowire : @Autowired
is used to automatically wire dependencies into a Spring-managed bean.It can be applied to fields, constructors, or setter methods of a class.When a bean is created and @Autowired
is used, Spring automatically resolves and injects the appropriate dependency into the bean.
@Service
@Slf4j
public class BedServiceImpl implements BedService {
@Autowired
private BedRepository bedRepo;
@Autowired
private RoomRepository roomRepo;
}
3.d)@Autowired
says 'please give me an instance of this class, one that I created with an @Bean
annotation earlier'.
3.e)If there are multiple beans that match the dependency type, you can further specify the dependency using qualifiers or by using @Primary
annotation.
summary of @Bean and @Autowire: @Bean is use to Declare the method and store it in Spring container until unless user asked using spring dependency injection .
@Autowired
is used to inject dependencies into a bean. @Bean
is typically used in configuration classes to define beans, and @Autowired
is used within the beans to wire the dependencies.
4.@Configuration: @Configuration
is used to declare a class as a configuration class in Spring.
Configuration classes are responsible for defining beans and configuring the application context.
Annotated with
@Configuration
, a class can contain@Bean
methods that define individual beans. These beans are managed by the Spring container and can be retrieved and used by other components.Configuration classes are often used in conjunction with
@ComponentScan
to scan for other Spring components and configure the application context.We cant use @Service ,@Controller , @Repository with in Class annotated with
5.@Component:
@Component
is a generic annotation used to indicate that a class is a Spring-managed component.It is a base annotation that can be used as a marker for any Spring-managed class.
By default, Spring will scan for classes annotated with
@Component
or its specializations (@Service
,@Repository
,@Controller
) and create beans for them in the application context.
6.@Controller:
A class is annotated with @Controller
, it indicates that the class serves as a controller component responsible for handling HTTP requests and managing the flow of the application. Typically, controllers receive incoming requests, process them, and return an appropriate response, such as rendering a view or returning data in JSON format.
7.@RestController: To get Response in Json Format we must use @RestController annotation in our Controller Class in Spring boot .
8.@RestControllerAdviceIn:
Spring Boot, the @RestControllerAdvice
annotation is used to create global exception handlers and centralized error handling for RESTful web services. It is a combination of @ControllerAdvice
and @ResponseBody
annotations, specifically designed for building RESTful APIs.
Example Code:
import java.io.FileNotFoundException;
import java.util.HashMap;
import java.util.Map
import javax.validation.ConstraintViolationException;
import org.springframework.http.HttpStatus;
import org.springframework.web.bind.MethodArgumentNotValidException;
import org.springframework.web.bind.annotation.ExceptionHandler;
import org.springframework.web.bind.annotation.ResponseStatus;
import org.springframework.web.bind.annotation.RestControllerAdvice;
@RestControllerAdvice
public class ApplicationException {
@ResponseStatus(HttpStatus.BAD_REQUEST)
@ExceptionHandler(MethodArgumentNotValidException.class)
public Map<String,String> handleInvalidArgument(MethodArgumentNotValidException ex) {
Map<String,String> errorMap = new HashMap<>();
ex.getBindingResult().getFieldErrors().forEach(error -> {
errorMap.put(error.getField(), error.getDefaultMessage());
});
return errorMap;
}
}
Subscribe to my newsletter
Read articles from Md Azam directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
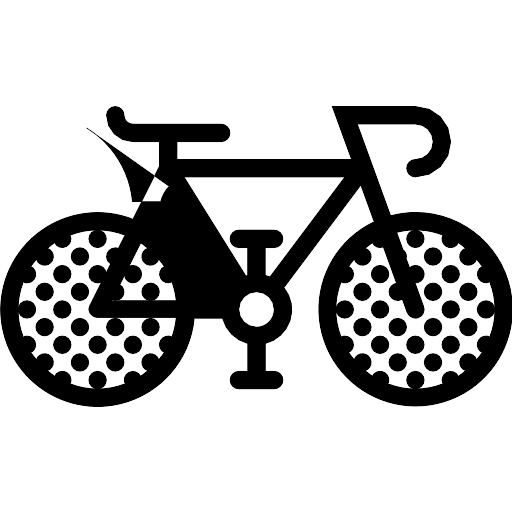
Md Azam
Md Azam
I am a full Stack developer ,Familiar with technologies like Java,Spring Boot,React,Redux,python.